Struggling with Arquillian? Master Secured EJB Testing Now!
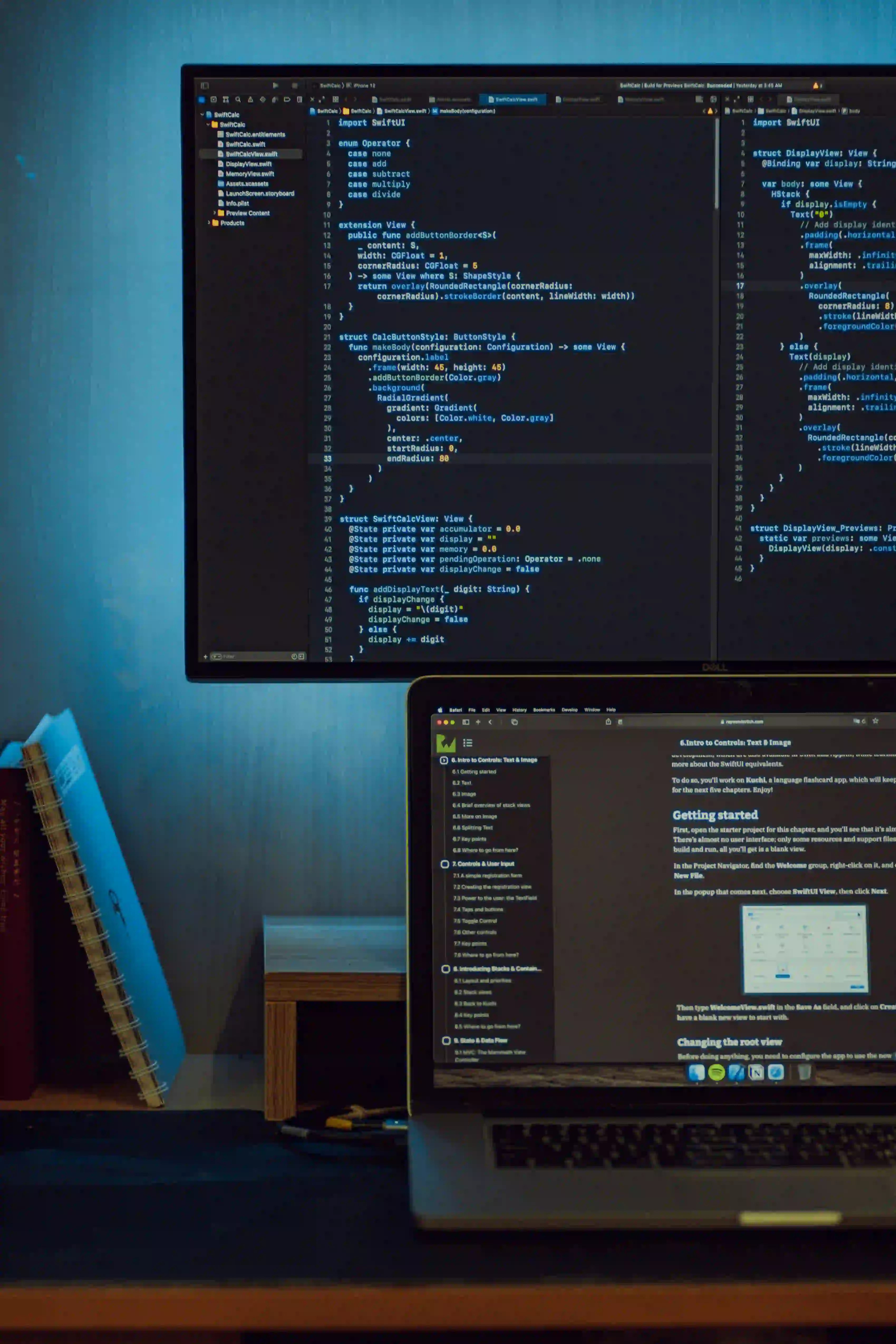
Mastering Secured EJB Testing with Arquillian
In the realm of Java Enterprise applications, testing plays a crucial role in ensuring code reliability and integrity. Among the plethora of testing frameworks, Arquillian stands out, especially for its ability to test Enterprise JavaBeans (EJBs) in a realistic container environment. While using Arquillian for secured EJB testing can seem daunting at first, mastering it can significantly enhance your development process. This blog will guide you through the essential concepts and practices for implementing secured EJB testing using Arquillian.
What is Arquillian?
Arquillian is an open-source testing platform that allows you to run your integration tests in the actual environment where your code will run. Unlike traditional unit tests, Arquillian performs tests in the container, enabling you to test components like EJBs under real-world conditions.
Why Use Arquillian for Secured EJB Testing?
- Realistic Environment: Test your EJBs in an environment that closely resembles production.
- Simplified Configuration: Arquillian abstracts much of the complexity associated with managing container setups.
- Powerful Integration Testing: It seamlessly integrates with various testing frameworks like JUnit and TestNG, allowing you to leverage existing test structures.
Getting Started with Arquillian
To get started, ensure you have the following tools installed:
- Java JDK (at least version 8)
- Maven or Gradle for dependency management
- Your preferred IDE (IntelliJ IDEA, Eclipse, etc.)
Step 1: Set Up Your Maven Project
Create a new Maven project and include the necessary dependencies in your pom.xml
. Here is an example configuration:
<dependencies>
<dependency>
<groupId>org.jboss.arquillian.junit</groupId>
<artifactId>arquillian-junit-container</artifactId>
<version>1.7.0.Final</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.jboss.arquillian.container</groupId>
<artifactId>arquillian-wildfly-embedded</artifactId>
<version>1.0.0.Final</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>javax.ejb</groupId>
<artifactId>jboss-ejb-api</artifactId>
<version>3.2.3.Final</version>
</dependency>
</dependencies>
Commentary: Adding the Arquillian dependencies allows the testing framework to manage a container automatically. The WildFly container dependency ensures that the EJBs are run in a lightweight instance of the application server for testing.
Step 2: Writing Your EJB
Here’s a simple secured EJB for demonstration purposes. This EJB adds user authentication functionality using @RolesAllowed
.
import javax.annotation.security.RolesAllowed;
import javax.ejb.Stateless;
@Stateless
public class UserService {
@RolesAllowed({"ADMIN"})
public String getUserDetails(String userId) {
// Simulating fetching user details
return "Details of user: " + userId;
}
}
Commentary: The @RolesAllowed
annotation restricts access to the getUserDetails()
method, ensuring that only users in the ADMIN role can invoke this method. This setup is fundamental for securing EJBs within Java EE applications.
Step 3: Writing Tests with Arquillian
Let’s write a test for the UserService
EJB. Begin by setting up an Arquillian test class.
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.fail;
import javax.inject.Inject;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import org.jboss.arquillian.container.test.api.Deployment;
import org.jboss.arquillian.junit.Arquillian;
import org.jboss.shrinkwrap.api.ShrinkWrap;
import org.jboss.shrinkwrap.api.spec.JavaArchive;
import org.junit.Test;
import org.junit.runner.RunWith;
@RunWith(Arquillian.class)
public class UserServiceTest {
@Inject
private UserService userService;
@Deployment
public static JavaArchive createDeployment() {
return ShrinkWrap.create(JavaArchive.class)
.addClasses(UserService.class)
.addAsManifestResource("META-INF/beans.xml"); // Required by CDI
}
@Test
public void testGetUserDetails() {
String expected = "Details of user: 123";
String actual = userService.getUserDetails("123");
assertEquals(expected, actual);
}
}
Commentary: In this test class, we:
- Use
@RunWith(Arquillian.class)
to integrate JUnit with Arquillian. - Create a deployment method annotated with
@Deployment
, which packages our EJB for testing. - Leverage
@Inject
to inject theUserService
EJB. - Finally, we assert the expected output against the actual result using JUnit's
assertEquals
.
Step 4: Security Context in Tests
To effectively test secured methods, it’s important to configure a security context. Arquillian provides the ability to simulate the security context. For this purpose, consider using the @WithSecurity
annotation or a custom method to set up security roles depending on your container.
Running Your Tests
To execute your tests, simply run the Maven test goal:
mvn test
Monitor the output for test results, and ensure that your secured EJB behaves as expected under the defined security constraints.
Additional Considerations
Best Practices for Secured EJB Testing:
- Modular Testing: Focus on testing each EJB in isolation.
- Role-based Testing: Structure tests to cover various roles and their access to EJB methods.
- Error Handling: Test for expected exceptions when users without the proper roles try to access secured methods.
- Integration Tests: Use Arquillian’s capabilities to perform integration tests with other components and services.
Useful Resources:
- Arquillian Documentation provides a solid foundation for understanding Arquillian’s capabilities.
- Check out the Java EE Security Overview for more context on securing your applications.
Closing the Chapter
Mastering secured EJB testing with Arquillian requires an understanding of both the framework and EJB security features. By following the steps outlined in this blog, you will be well-equipped to test your Java EE applications in a secure and robust manner. Testing is not merely a means to catch bugs; it is an integral part of delivering quality software that meets user expectations.
Now go ahead and try integrating Arquillian into your projects. Happy testing!