Common Spring Boot Mistakes and How to Avoid Them
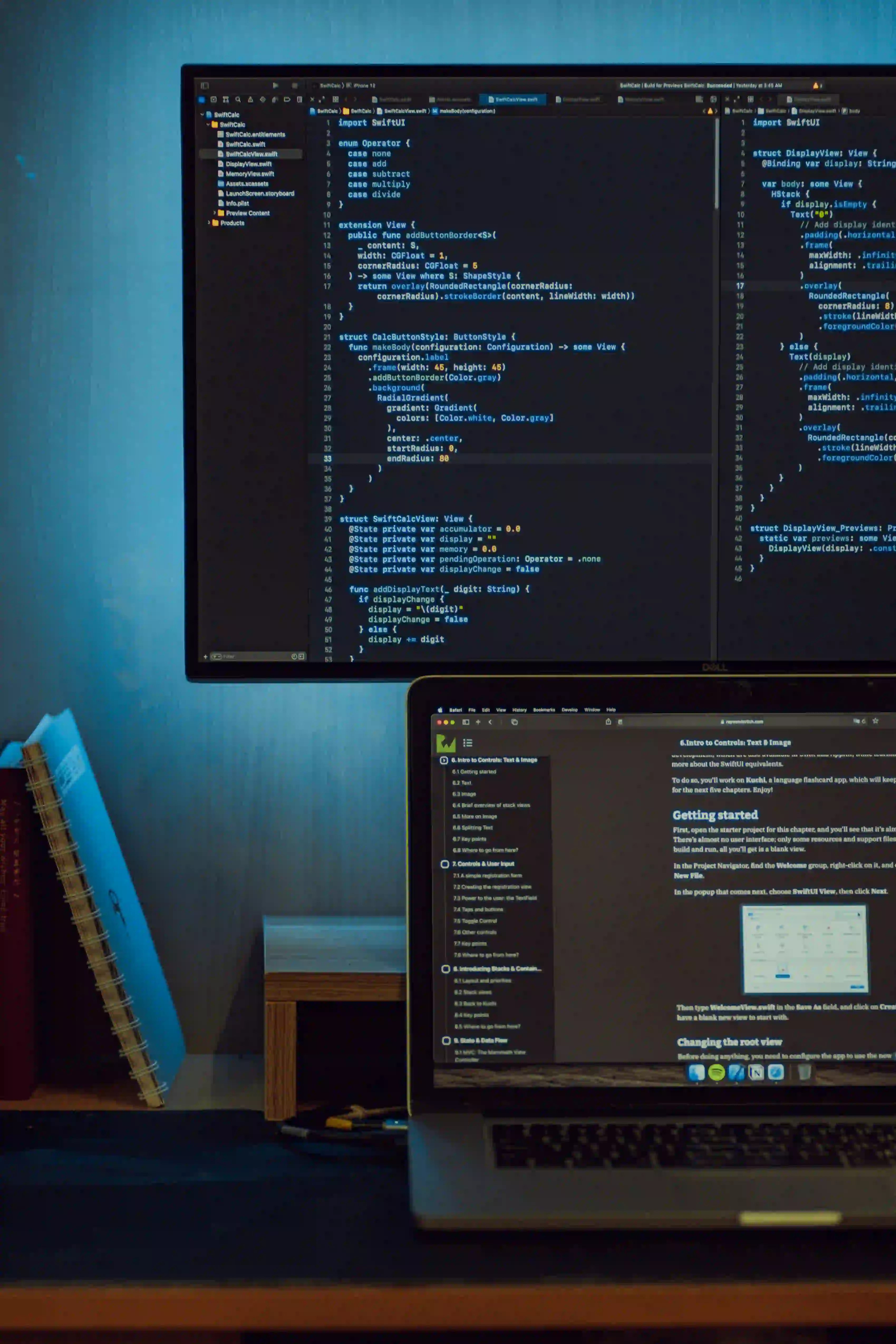
Common Spring Boot Mistakes and How to Avoid Them
Spring Boot is a powerful framework that simplifies the development of Java applications. However, even experienced developers can make mistakes when using it. This blog post aims to discuss common pitfalls in Spring Boot development and how to avoid them, ensuring you write cleaner, more maintainable code.
Why Use Spring Boot?
Before we dive into the mistakes, let's briefly explore why Spring Boot is so popular. It enables rapid application development by providing the following features:
- Convention over Configuration: Spring Boot follows sensible defaults, allowing developers to focus on business logic rather than configurations.
- Embedded Servers: With built-in support for Tomcat, Jetty, and Undertow, you can run your applications standalone without needing a separate server.
- Microservices Ready: It’s easy to create microservices with Spring Boot thanks to its modular architecture and powerful dependency management.
However, let’s now transition into the common mistakes developers make.
1. Ignoring Dependency Management
Mistake
One common mistake is neglecting Maven or Gradle dependency management. This can lead to issues such as version conflicts or unnecessary bloat due to securing multiple versions of a library.
Solution
Opt for a well-defined dependency management strategy. Utilize the spring-boot-dependencies
BOM (Bill of Materials) which helps manage the versions of shared dependencies efficiently.
Example using Maven:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.5.4</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Why? This way, Maven handles transitive dependencies for you, reducing the risk of version conflicts.
2. Excessive Use of @Autowired
Mistake
Using the @Autowired
annotation for dependency injection everywhere can lead to tightly-coupled classes, making unit testing difficult.
Solution
Prefer constructor injection whenever possible. This promotes immutability and makes the dependency requirements of a class explicit.
Example:
@Service
public class MyService {
private final MyRepository myRepository;
@Autowired
public MyService(MyRepository myRepository) {
this.myRepository = myRepository;
}
}
Why? Constructor injection makes it clear what dependencies are required for a class, improving readability and maintainability.
3. Not Utilizing Spring Profiles
Mistake
Many developers hard-code configurations directly into their applications, disregarding the benefits of Spring Profiles. This results in the lack of flexibility when deploying to different environments such as development, testing, or production.
Solution
Use Spring Profiles to separate configuration for different environments. You can define profile-specific properties in application-{profile}.yml
files.
Example:
# application-dev.yml
server:
port: 8081
# application-prod.yml
server:
port: 80
Why? This approach allows you to manage configurations cleanly and keep your application adaptable to various environments.
4. Ignoring Actuator Endpoints
Mistake
Spring Boot includes a powerful feature set in the form of Actuator endpoints that provide insights into your application's health and metrics. Neglecting these can lead to missed opportunities for system monitoring.
Solution
Integrate the Spring Boot Actuator and configure the endpoints that suit your needs.
Example:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
In application.yml
, enable specific endpoints:
management:
endpoints:
web:
exposure:
include: health, info
Why? Knowing the health of your application and important metrics allows for proactive troubleshooting and performance optimization.
5. Overriding Methods in Configuration Classes
Mistake
Overriding methods in configuration classes without proper understanding can lead to unintended consequences in the application context.
Solution
Ensure you fully understand the lifecycle of Spring beans and the impact of method overriding before applying it to configuration classes.
Example:
@Configuration
public class MyConfig {
@Bean
public MyService myService() {
return new MyService();
}
@Bean
public MyRepository myRepository() {
return new MyRepository(myService());
}
}
Why? When writing configuration, clarity in the creation of beans is essential in avoiding unexpected behavior in your application.
6. Not Leveraging Spring Data JPA Properly
Mistake
Many developers try to write queries manually rather than leveraging Spring Data JPA's repository functionalities, which can lead to code duplication and maintenance challenges.
Solution
Utilize Spring Data JPA repositories for CRUD operations.
Example:
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByLastName(String lastName);
}
Why? This abstraction helps you avoid boilerplate code while also giving you the ability to customize queries through method naming conventions.
7. Dismissing Exception Handling
Mistake
Neglecting proper exception handling can make your application prone to crashes and less user-friendly.
Solution
Implement global exception handling using @ControllerAdvice
to catch errors across your application.
Example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<Object> handleResourceNotFound(ResourceNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
Why? This promotes a standardized response to exceptions, improving user experience and simplifying debugging.
Closing Remarks
Spring Boot can tremendously streamline your Java application development, but avoiding common pitfalls ensures you're maximizing its potential. By understanding and circumventing these common mistakes—like ignoring dependency management, not using Spring Profiles, and neglecting exception handling—you can build more robust, maintainable applications.
For more on Spring Boot best practices, check out Spring Boot Official Documentation and consider exploring additional resources such as Baeldung's Spring Boot Tutorials.
By implementing these strategies, you’ll sharpen your development skills and foster an environment that encourages clean, efficient code. Happy coding!