Solving Bidirectional One-to-One Key Association Issues
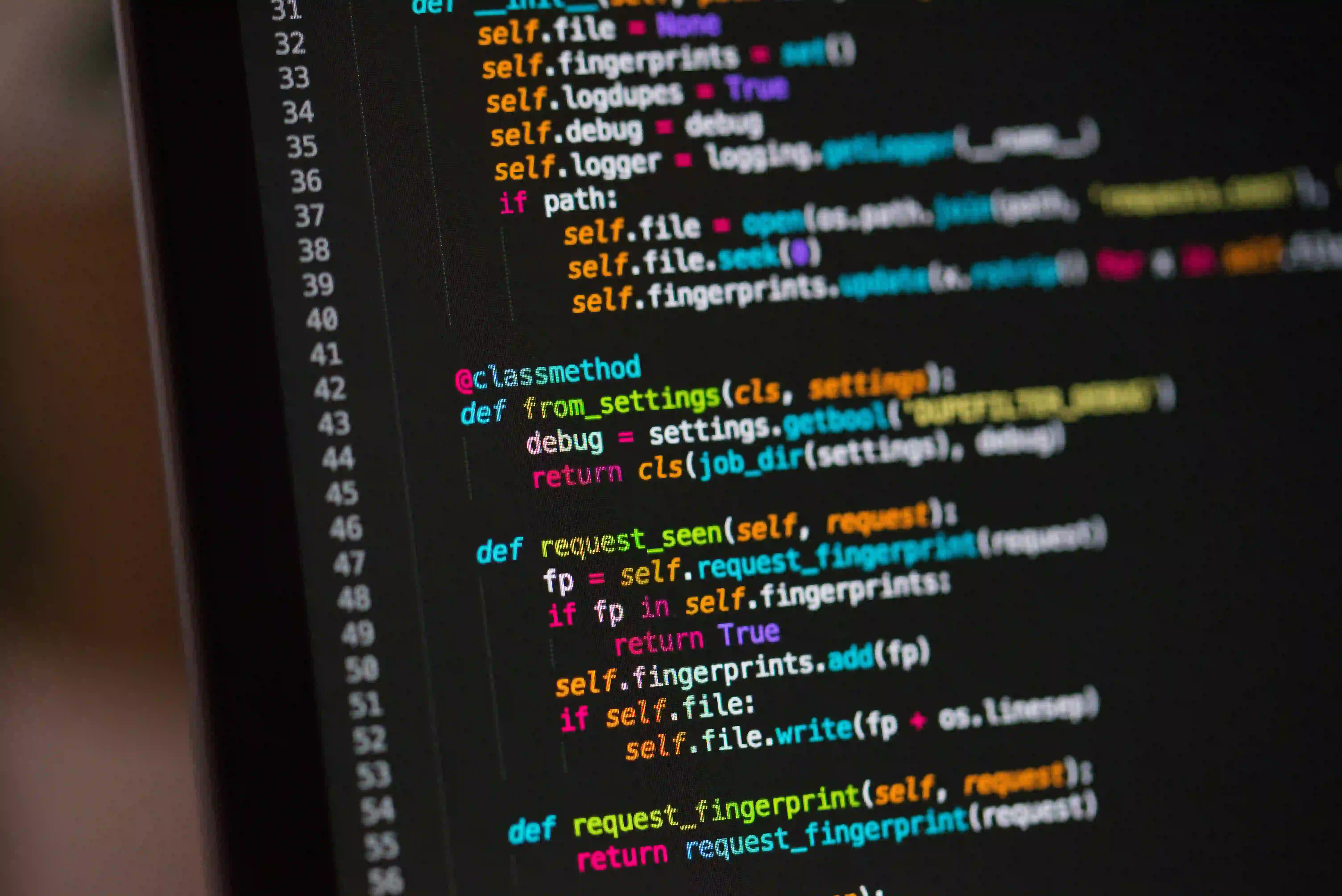
Solving Bidirectional One-to-One Key Association Issues in Java
Bidirectional One-to-One key associations are prevalent in Java, particularly when working with Object-Relational Mapping (ORM) frameworks like Hibernate and JPA. These associations allow you to establish a two-way relationship between two entities. However, implementing them can often lead to complexities and potential pitfalls. In this post, we will explore how to effectively manage bidirectional one-to-one associations while utilizing Hibernate ORM to ensure the integrity and efficiency of your data.
Understanding Bidirectional One-to-One Associations
In a bidirectional one-to-one association, two entities reference each other. This relationship can be illustrated through an example involving a User
and a Profile
. Every user has a unique profile, and each profile directly corresponds to a single user.
Consider the following structure:
- A
User
entity containing a reference to aProfile
. - A
Profile
entity containing a reference back to theUser
.
This relationship can be beneficial for encapsulation and minimizes redundancy, but the implementation can be error-prone if not handled correctly.
Setting Up the Entities
Let us define the User
and Profile
entities using Java Persistence API (JPA) annotations:
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
@OneToOne(mappedBy = "user", cascade = CascadeType.ALL, fetch = FetchType.LAZY, optional = false)
private Profile profile;
// Constructors, Getters and Setters
}
Commentary:
- The
@OneToOne(mappedBy = "user")
annotation defines the bidirectional relationship, making theUser
the non-owning side. - The
cascade = CascadeType.ALL
means changes (like persist, merge, etc.) onUser
will propagate to theProfile
. - The
optional = false
attribute ensures that aUser
must have an associatedProfile
.
Now, let’s define the Profile
entity.
import javax.persistence.*;
@Entity
@Table(name = "profiles")
public class Profile {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String bio;
@OneToOne
@JoinColumn(name = "user_id", nullable = false)
private User user;
// Constructors, Getters and Setters
}
Commentary:
- The
@JoinColumn(name = "user_id")
specifies the foreign key in theprofiles
table that links to theusers
table. - This setup reflects a one-to-one relationship, effectively linking a profile back to its corresponding user.
Managing Changes in Bidirectional Relationships
When dealing with bidirectional relationships, maintaining synchronization between the two entities often presents challenges. If a relationship is altered from one side, it is crucial to ensure the other side reflects that change.
Example of Synchronizing Both Ends
Let's say you want to add or update a user's profile. It is essential to synchronize both entities. Here is how you can accomplish this:
public void updateUserProfile(User user, Profile profile) {
user.setProfile(profile);
profile.setUser(user);
// Assume entityManager is provided and is managed transactionally
entityManager.merge(user);
}
Commentary:
- In the method
updateUserProfile
, both theUser
andProfile
references are set, ensuring they remain in sync. - It is highly recommended to handle such changes within a transaction to maintain data integrity.
Avoiding Common Pitfalls
1. Circular Dependency Issues
When deleting or merging entities, ensure that removal of an entity does not result in a circular reference. Consider the following during transactions:
public void deleteUser(User user) {
if (user.getProfile() != null) {
user.getProfile().setUser(null); // Break the link
}
entityManager.remove(user);
}
Commentary:
- This code snippet demonstrates how to properly handle deletion by breaking the bidirectional link.
- Always ensure that the reference is broken to avoid exceptions when attempting to delete.
2. Lazy Loading Concerns
Using FetchType.LAZY
can lead to LazyInitializationException
when accessing the associated profile outside the transactional context. Always ensure the session is active when accessing lazily-loaded entities.
Utilizing DTOs (Data Transfer Objects) can be a viable strategy to prevent this exception and avoid loading unnecessary data.
Business Logic Considerations
In a real-world application, you might want to consider using a service layer that encapsulates the logic of handling users and profiles. For example:
@Service
public class UserProfileService {
@Autowired
private UserRepository userRepository;
public void createUserWithProfile(String username, String bio) {
User user = new User();
user.setUsername(username);
Profile profile = new Profile();
profile.setBio(bio);
user.setProfile(profile);
profile.setUser(user);
userRepository.save(user);
}
}
Commentary:
- This method encapsulates the logic required to create a user with a profile while effectively managing both sides of the relationship.
- Keeping business logic in a dedicated service layer enhances readability and maintainability.
The Last Word
Solving bidirectional one-to-one key association issues in Java can be a challenging yet rewarding endeavor. By leveraging JPA and Hibernate correctly, you can establish a robust relationship between your entities while preserving the integrity and efficiency of your database operations.
To further your understanding of JPA and Hibernate, consider reading the following resources:
Implementing these best practices can significantly reduce debugging time and improve the functionality of your applications. Remember, the essence of maintaining data integrity lies in the careful management of relationships between entities. Happy coding!