Top Java Projects to Boost Your Programming Skills
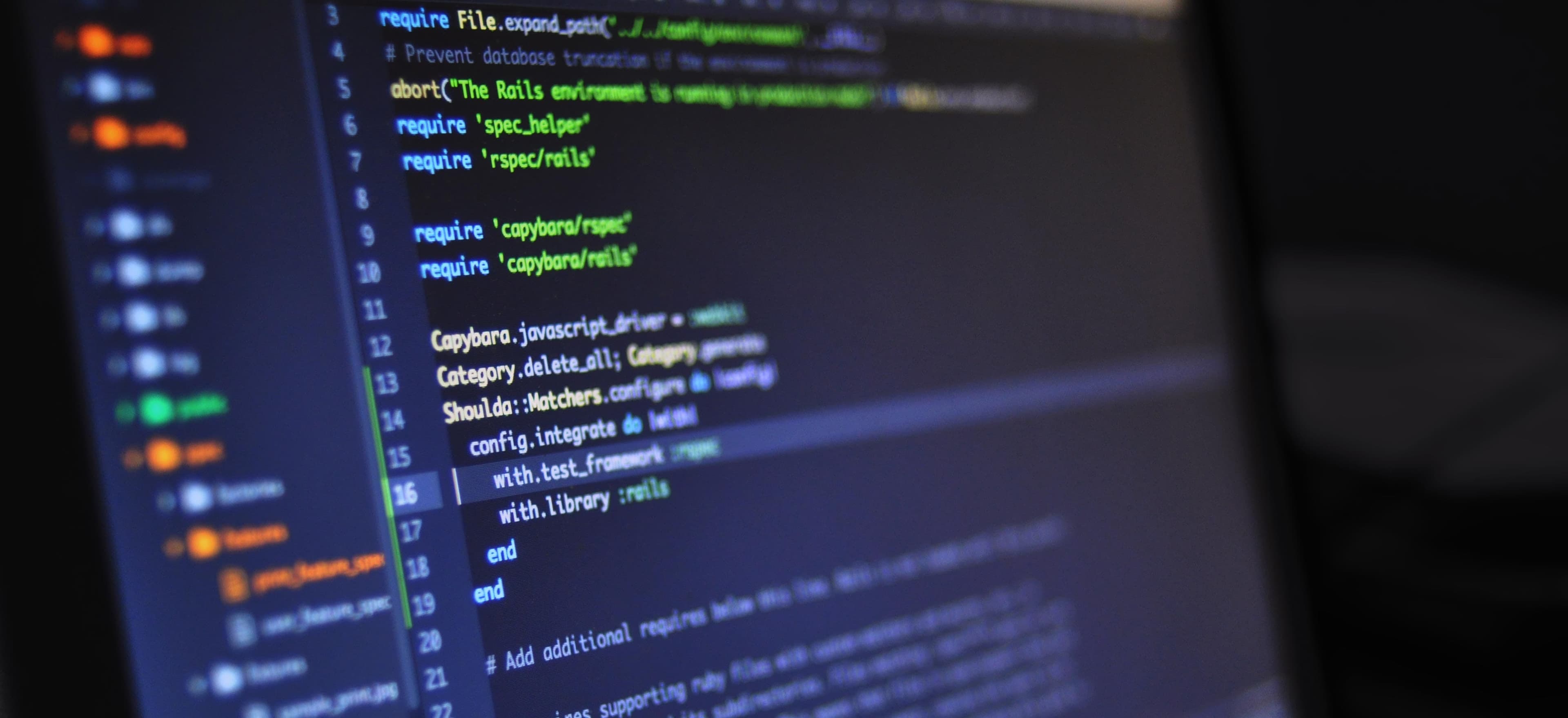
- Published on
Top Java Projects to Boost Your Programming Skills
Java is one of the most widely used programming languages in the world, known for its portability, performance, and vibrant community. Whether you are a beginner honing your skills or an advanced programmer looking to deepen your expertise, working on real-world projects is essential. In this blog post, we will explore some exciting project ideas that will help you improve your Java skills significantly.
Why Work on Projects?
Projects allow you to move beyond tutorials and theoretical concepts. They provide a platform to implement what you have learned, encounter real-world challenges, and cultivate problem-solving skills. Moreover, projects can enhance your portfolio, making you more appealing to potential employers.
1. Personal Expense Tracker
Overview
A personal expense tracker is a simple application to record and manage your expenses. Users can add, edit, and delete transactions, making it an excellent project to understand CRUD (Create, Read, Update, Delete) operations.
Key Features
- User Authentication
- Expense Categorization
- Monthly Reports and Graphs
Key Technologies
- Java with Spring Boot for the backend
- MySQL database for storing transactions
- Thymeleaf or React for the frontend
Sample Code Snippet
Here’s a simple method to add a new transaction:
public void addTransaction(Transaction transaction) {
if(transaction != null && transaction.getAmount() > 0) {
transactionRepository.save(transaction);
} else {
throw new IllegalArgumentException("Invalid transaction");
}
}
Why This Code?
The method above checks if the transaction is valid before saving it to the database. Validation helps maintain data integrity and prevents erroneous entries.
2. Library Management System
Overview
This project digitizes a library's operations, allowing users to check out, return, and manage books. This is ideal for grasping class relationships and data structures in Java.
Key Features
- Book Catalog Management
- User Registration
- Book Search Functionality
Key Technologies
- Java with JavaFX for the desktop application
- SQLite or MySQL for data storage
Sample Code Snippet
Below is a method to search for a book:
public List<Book> searchBooks(String query) {
List<Book> results = new ArrayList<>();
for (Book book : bookList) {
if (book.getTitle().toLowerCase().contains(query.toLowerCase())) {
results.add(book);
}
}
return results;
}
Why This Code?
This method iteratively checks each book's title against the search query. It highlights how loops and collections work in Java, reinforcing fundamental programming constructs.
3. Chat Application
Overview
Creating a chat application will deepen your understanding of networking and multithreading in Java. You'll learn how to communicate between client and server.
Key Features
- Private and Group Chats
- User Online Status
- Chat History
Key Technologies
- Java Sockets for network communication
- Swing or JavaFX for the GUI
Sample Code Snippet
To establish a connection with a client, you might use:
try (ServerSocket serverSocket = new ServerSocket(12345)) {
while (true) {
Socket clientSocket = serverSocket.accept();
new ClientHandler(clientSocket).start();
}
} catch (IOException e) {
e.printStackTrace();
}
Why This Code?
This code snippet sets up a server that listens for incoming connections. It demonstrates the fundamental concepts of sockets in Java, essential for any networking-related project.
4. E-Commerce Platform
Overview
An e-commerce platform can be a complex project that incorporates several aspects of software development like UI/UX design, database management, and server-side handling.
Key Features
- Product Listings
- Shopping Cart and Checkout Process
- User Accounts and Order History
Key Technologies
- Java with Spring Boot for backend
- React or Angular for frontend
- MySQL or MongoDB for database management
Sample Code Snippet
To handle product addition:
public void addProduct(Product product) {
if (product != null && product.getPrice() > 0) {
productRepository.save(product);
} else {
throw new IllegalArgumentException("Product must be valid and have a positive price");
}
}
Why This Code?
Before saving the product, the method checks its validity, ensuring that only proper data gets stored in the database, which is a good practice in any application.
5. Weather Application
Overview
Developing a weather application is not only fun but also provides a practical understanding of APIs and JSON parsing.
Key Features
- Real-time Weather Data
- Location-Based Services
- Weather Forecast
Key Technologies
- OpenWeatherMap API for weather data
- Java with Spring Boot for backend
- Android or JavaFX for the user interface
Sample Code Snippet
Here is how you can fetch weather data using an API:
public Weather getWeather(String location) throws IOException {
URL url = new URL("https://api.openweathermap.org/data/2.5/weather?q=" + location + "&appid=YOUR_API_KEY");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return parseWeatherData(response.toString());
}
Why This Code?
This method demonstrates how to communicate with external APIs in Java. Parsing JSON data is essential for working with numerous web services available today.
6. Task Management App
Overview
A task management app helps users keep track of tasks and deadlines, perfect for practicing Java collections and date handling.
Key Features
- Task Creation and Reminders
- Categories and Prioritization
- Deadline Tracking
Key Technologies
- Java with Spring Boot
- MySQL for database storage
- Angular or React for a dynamic front-end
Sample Code Snippet
A method to mark a task as complete:
public void completeTask(int taskId) {
Task task = taskRepository.findById(taskId);
if (task != null) {
task.setCompleted(true);
taskRepository.save(task);
} else {
throw new NoSuchElementException("Task not found");
}
}
Why This Code?
The method exemplifies the importance of checking for null objects before proceeding. It showcases good defensive programming practices, vital in application development.
A Final Look
Working on these Java projects will not only improve your programming skills but also give you insights into real-world application development. As you implement these ideas, you’ll face challenges that will stretch your capabilities as a developer. Remember to document your progress and share your projects online, possibly on platforms like GitHub, to showcase your skills to future employers.
For more inspiration, check out GitHub for open-source projects or Codewars for coding challenges that can further refine your skills.
Happy coding!
Checkout our other articles