Common Spring Boot Mistakes That New Developers Make
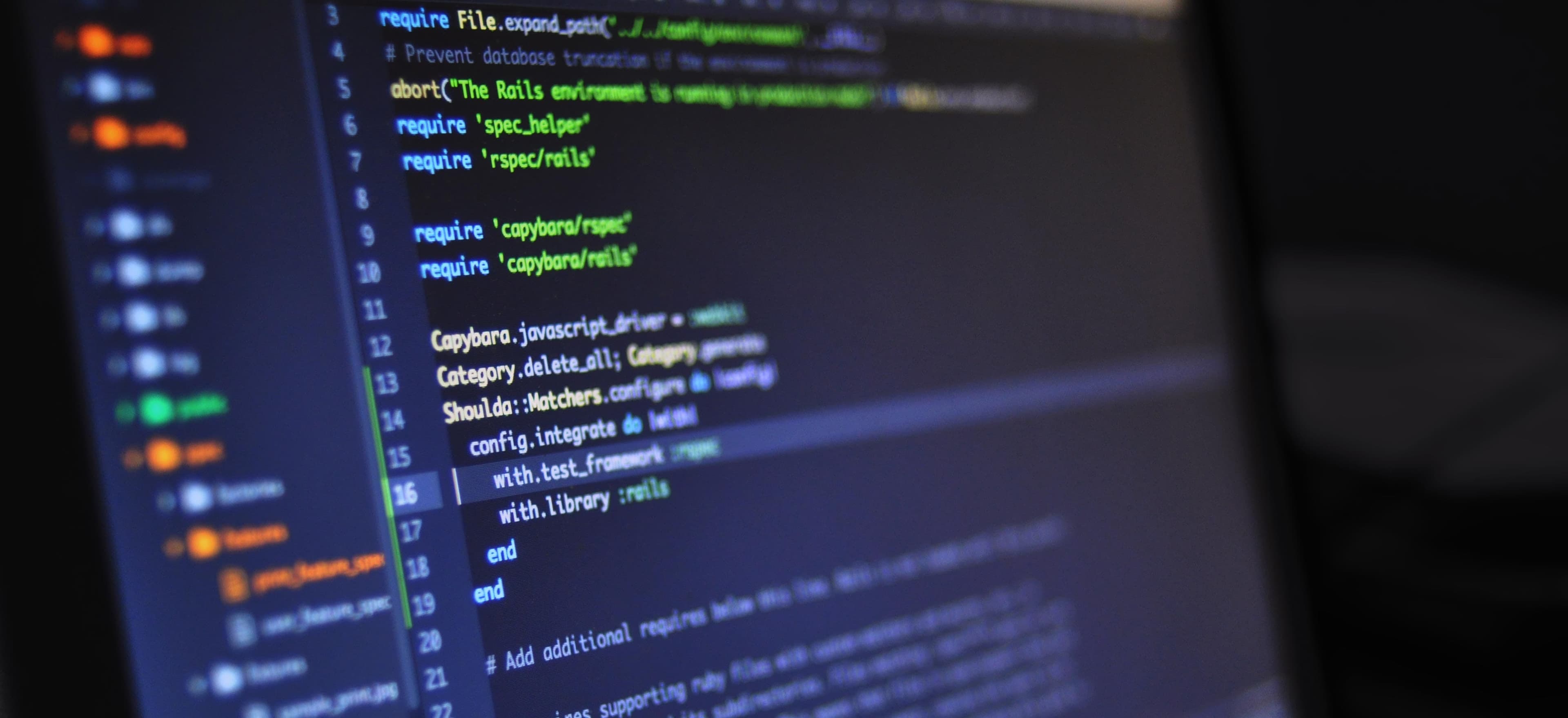
- Published on
Common Spring Boot Mistakes That New Developers Make
Spring Boot has quickly become one of the most popular frameworks for building Java applications. It simplifies the process of developing, deploying, and managing applications with a wide array of features like auto-configuration, an embedded server, and production-ready setups. However, even though Spring Boot aims to facilitate development, new developers often encounter pitfalls that can hinder their progress. In this article, we will discuss common mistakes made by novice Spring Boot developers and how to avoid them.
1. Ignoring Dependency Management
One of the primary features of Spring Boot is its ability to manage dependencies through the use of Maven or Gradle. New developers sometimes overlook the importance of proper dependency management.
Why It Matters
Not managing dependencies can lead to version conflicts, overlapping classes, and ultimately, application failures.
Example
When creating a Spring Boot application using Maven, you might start with a pom.xml
file that looks like this:
<properties>
<java.version>17</java.version>
<spring-boot.version>2.5.4</spring-boot.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Ensuring that you're using compatible versions across your dependencies is crucial. For instance, if you're using Spring Boot 2.5.4, do not mix it with libraries that expect Spring Boot 1.x.
2. Underestimating Configuration Properties
Spring Boot offers extensive configuration options through properties files (application.properties
or application.yml
). New developers often neglect to leverage these configuration capabilities effectively.
Why It Matters
Improper configuration can lead to issues that are hard to pinpoint and can complicate debugging.
Example
Setting up your application properties correctly can help manage environments:
# application.properties
# Server settings
server.port=8080
# DataSource configuration
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=myuser
spring.datasource.password=mypass
# JPA settings
spring.jpa.hibernate.ddl-auto=update
Utilizing environment-specific properties can lead to a more robust application. Consider using environment variables or profiles to load different configurations in development and production.
3. Not Using Spring Profiles
Many newcomers tend to hard-code configurations rather than separating them using Spring Profiles, such as dev
, test
, and prod
.
Why It Matters
Hard-coded configurations make it challenging to switch environments; you may inadvertently leak credentials or sensitive configurations.
Example
To utilize profiles, you can create separate properties files:
application-dev.properties
application-prod.properties
And activate them with a simple command:
java -jar myapp.jar --spring.profiles.active=dev
By leveraging Spring profiles, you maintain cleaner, more manageable code.
4. Failing to Handle Exceptions Properly
New developers often mishandle exceptions or skip them altogether in their applications.
Why It Matters
Unhandled exceptions can generate confusing error messages and degrade user experience.
Example
Instead of generic exception handling, employ @ControllerAdvice
for global exception handling:
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<Object> handleResourceNotFoundException(ResourceNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
This approach helps in providing a consistent error response to users.
5. Not Leveraging Spring Data JPA Properly
Many new developers misuse Spring Data JPA or don't utilize it at all, opting instead for JDBC template coding.
Why It Matters
Spring Data JPA simplifies data access and significantly reduces boilerplate code. Ignoring it means more repetitive coding and perplexing queries.
Example
Instead of writing boilerplate methods, create a repository interface:
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByEmail(String email);
}
By extending JpaRepository
, you inherit methods like save()
, delete()
, and findAll()
, thus significantly reducing code complexity.
6. Overusing @Autowired
While @Autowired
is a powerful annotation that simplifies dependency injection, its overuse can make code difficult to test and maintain.
Why It Matters
Over-reliance on @Autowired
can lead to hidden dependencies in your classes.
Example
Instead of injecting dependencies via fields, prefer constructor injection:
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
This approach enhances testability and clearly defines your dependencies.
7. Skipping Unit Tests
Quality assurance is often an afterthought for new developers, who may skip unit tests entirely.
Why It Matters
Not testing your code can lead to bugs surfacing late in the development process.
Example
Here's a simple unit test using JUnit and Mockito:
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserServiceTest {
@MockBean
private UserRepository userRepository;
@Autowired
private UserService userService;
@Test
public void testFindUserByEmail() {
when(userRepository.findByEmail("test@example.com")).thenReturn(Optional.of(new User()));
User user = userService.findByEmail("test@example.com");
assertNotNull(user);
}
}
This unit test ensures your service behaves as expected when interacting with the repository.
Closing the Chapter
Spring Boot streamlines Java application development, but common pitfalls can complicate the process for new developers. By focusing on proper dependency management, effective configuration, better exception handling, and the proper use of Spring features, developers can create more robust applications.
Always strive for clean, maintainable code and make use of the extensive features Spring Boot offers. For additional insights into making the most of Spring Boot, you can explore the official Spring Boot Documentation.
By avoiding these common mistakes, you'll be well on your way to mastering Spring Boot and becoming a proficient Java developer. Happy coding!
Checkout our other articles