Decoding Public Key Infrastructure: Common Pitfalls Revealed
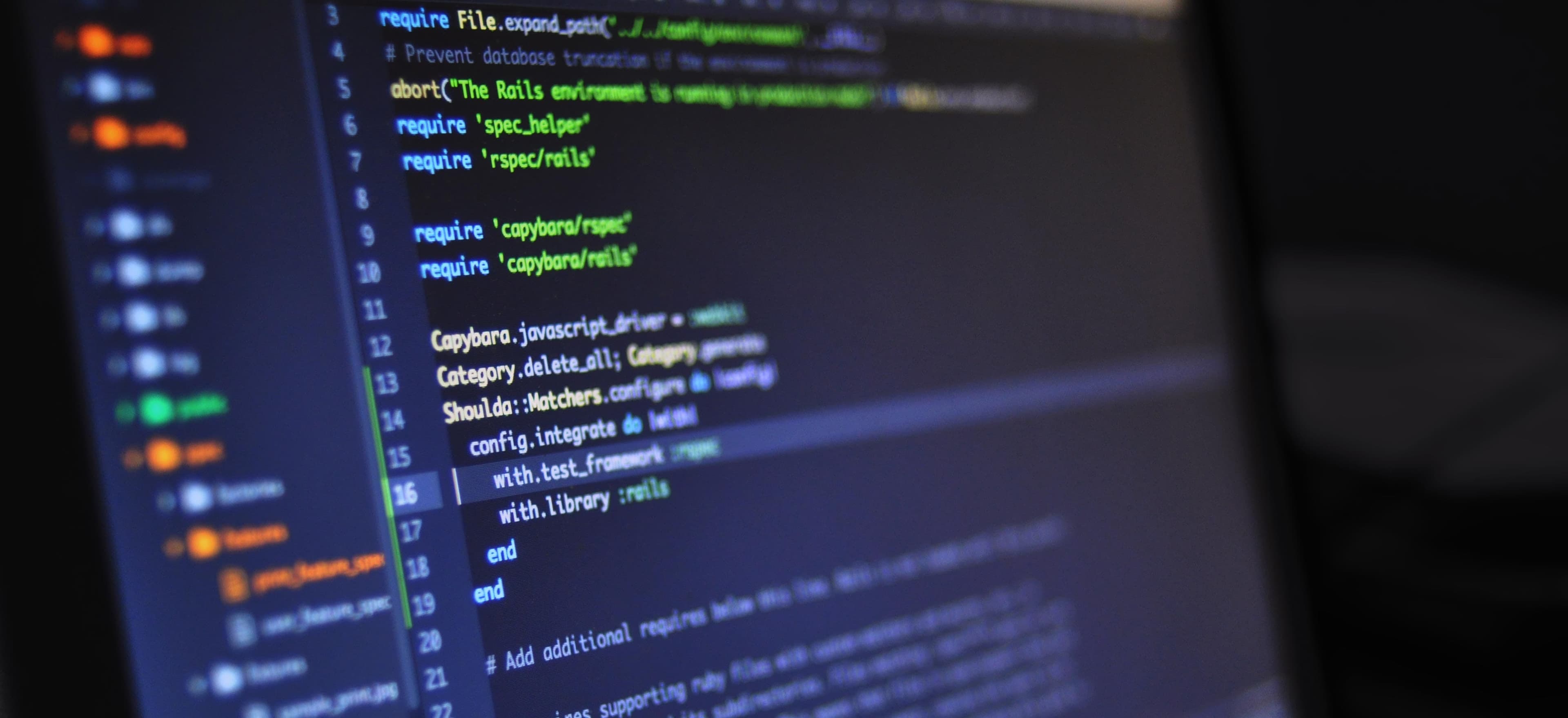
- Published on
Decoding Public Key Infrastructure: Common Pitfalls Revealed
Public Key Infrastructure (PKI) is crucial in today’s digital landscape. It allows for secure communication, authentication, and data integrity across various platforms. However, misunderstandings and misconfigurations can lead to severe vulnerabilities. This blog post will delve into the common pitfalls in PKI, helping you understand how to navigate this complex landscape effectively.
Understanding Public Key Infrastructure
Before we tackle the pitfalls, let’s briefly explain what PKI is.
PKI is a framework that enables secure data exchange through cryptographic keys. It typically consists of:
- Public Keys: These are widely distributed and used for encrypting messages or verifying signatures.
- Private Keys: These are kept secret and are used for decrypting messages or generating signatures.
- Certificate Authorities (CAs): Trusted entities that issue digital certificates to verify identities.
- Digital Certificates: These bind a public key to an individual or entity, establishing trust.
For more detailed information on PKI and its components, you can refer to the National Institute of Standards and Technology (NIST) guide on PKI.
Common Pitfalls in PKI
While PKI can enhance security, there are several pitfalls that organizations often encounter. Let’s explore the most common ones.
1. Poor Key Management
What’s the issue?
Key management is the backbone of PKI security. Mismanagement can lead to key compromise, rendering the entire system vulnerable. Common pitfalls include:
- Using Default Keys: Many systems come with preset keys. Using these keys is risky as they can be easily compromised.
- Inconsistent Key Rotation: Not rotating keys regularly can lead to prolonged exposure of a compromised key.
How to mitigate?
Implement a comprehensive key management policy, including:
- Regular key rotation.
- Unique key generation for each application.
Here’s an exemplary snippet to illustrate key generation using Java's KeyPairGenerator
:
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.NoSuchAlgorithmException;
public class KeyPairExample {
public static void main(String[] args) {
try {
// Initialize KeyPairGenerator for RSA
KeyPairGenerator keyGen = KeyPairGenerator.getInstance("RSA");
keyGen.initialize(2048); // Using a 2048-bit key
KeyPair pair = keyGen.generateKeyPair();
// Print key details
System.out.println("Public Key: " + pair.getPublic().toString());
System.out.println("Private Key: " + pair.getPrivate().toString());
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
}
}
Why? This ensures that you always use strong, unique keys tailored for your security needs.
2. Misconfigured Certificates
What’s the issue?
Incorrect configurations during the issuance of digital certificates can lead to trust issues. Common misconfigurations include:
- Expired Certificates: Using a certificate that has expired can lead to loss of trust.
- Incomplete Certificate Chains: Not providing the entire certificate chain can cause verification failures.
How to mitigate?
Always validate the configuration of your certificates:
- Check expiration dates.
- Use tools to validate your certificate chain, like SSL Labs’ SSL Test.
Here’s how to check a certificate expiration in Java:
import java.security.cert.CertificateFactory;
import java.security.cert.X509Certificate;
import java.io.FileInputStream;
public class CertificateExpirationCheck {
public static void main(String[] args) throws Exception {
FileInputStream fis = new FileInputStream("path/to/certificate.crt");
CertificateFactory cf = CertificateFactory.getInstance("X.509");
X509Certificate cert = (X509Certificate) cf.generateCertificate(fis);
// Check if the certificate is expired
try {
cert.checkValidity();
System.out.println("Certificate is valid.");
} catch (Exception e) {
System.out.println("Certificate has expired: " + e.getMessage());
}
}
}
Why? Regularly checking the status of your certificates can prevent unauthorized access and maintain trust.
3. Lack of Awareness and Training
What’s the issue?
Many organizations overlook the importance of educating their staff about PKI. Lack of knowledge can lead to incorrect implementations or security lapses.
How to mitigate?
- Training Sessions: Regularly conduct training sessions focused on PKI basics and best practices.
- Documentation: Provide clear documentation regarding PKI processes and protocols.
4. Ignoring Revocation
What’s the issue?
Certificate revocation is often neglected, leading organizations to trust keys or certificates that should no longer be valid. Common practices that fall short include:
- Failing to use the Online Certificate Status Protocol (OCSP).
- Not updating the Certificate Revocation List (CRL).
How to mitigate?
Always ensure that revocation mechanisms are operational:
- Integrate OCSP into your applications where possible.
- Regularly update and monitor the CRL.
Example code snippet to check the status of a certificate using OCSP in Java:
import java.security.cert.X509Certificate;
import org.bouncycastle.cert.ocsp.OCSPReqBuilder;
import org.bouncycastle.cert.ocsp.OCSPResp;
import org.bouncycastle.cert.ocsp.OCSPReq;
public class OCSPCheck {
public static boolean checkCert(X509Certificate cert) {
try {
OCSPReqBuilder reqBuilder = new OCSPReqBuilder();
OCSPReq request = reqBuilder.addRequest(cert).build();
// Send the request to OCSP responder (omitted for brevity).
OCSPResp response = sendRequestToOCSP(request);
return response.getStatus() == 0; // 0 indicates a valid response
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
}
Why? Regularly confirming revocation status helps maintain a strong security posture.
5. Over-reliance on PKI
What’s the issue?
PKI is a powerful tool, but it should not be the only line of defense. Over-reliance can lead to complacency regarding other security measures.
How to mitigate?
Adopt a holistic security approach by layering additional security mechanisms such as:
- Multi-factor authentication (MFA).
- Intrusion detection systems (IDS).
Wrapping Up
Public Key Infrastructure is a significant asset for organizations aiming to secure digital communications. However, pitfalls abound. By understanding and actively mitigating these issues—like poor key management, misconfigured certificates, lack of awareness, ignoring revocation, and over-reliance—you can bolster your organization's security posture.
For more technical information on implementing PKI solutions, you might explore resources from Microsoft's documentation on PKI, which offers insights applicable across various platforms.
By adopting best practices and fostering a culture of awareness, you can ensure that your PKI framework serves your organization effectively while minimizing risks.