Overcoming Challenges in Fine-Grained Authorization Implementation
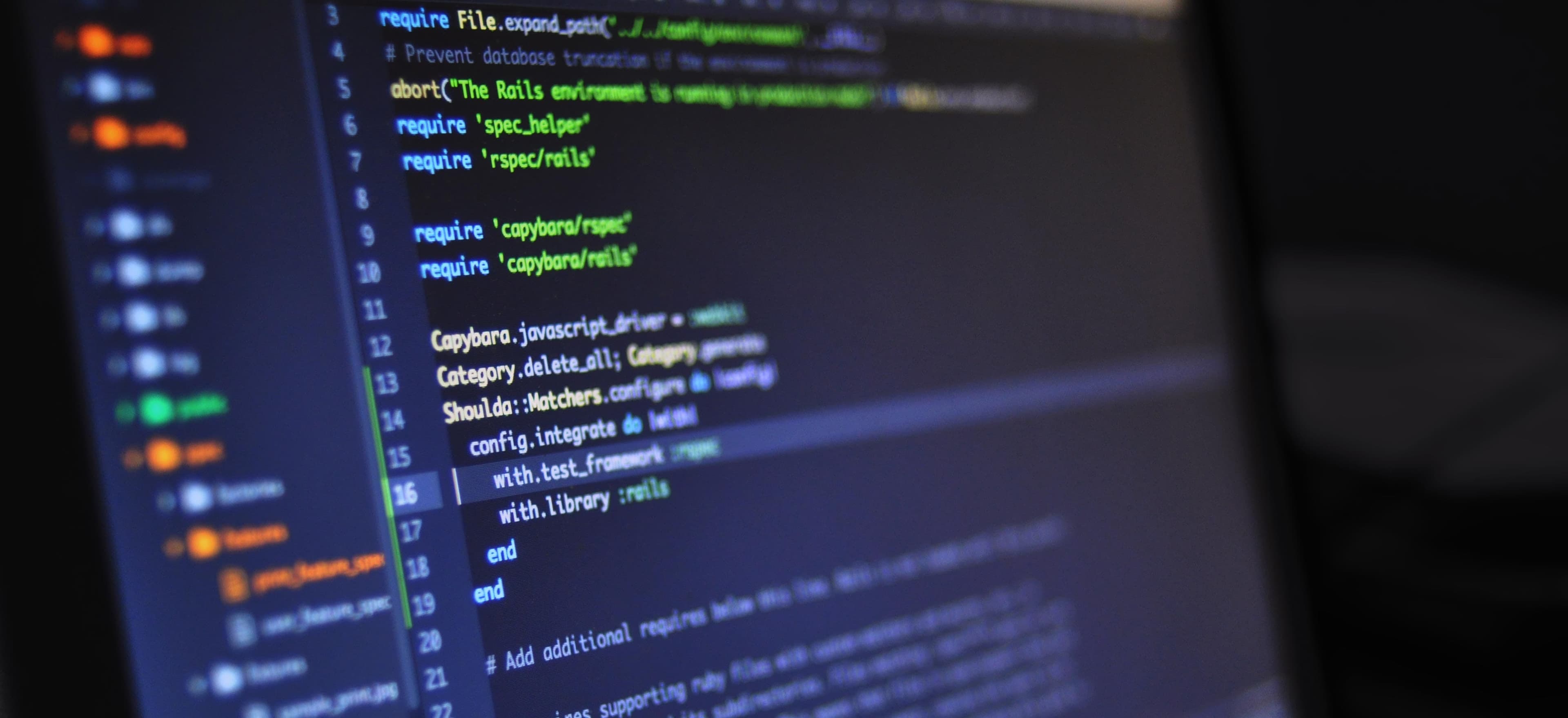
- Published on
Overcoming Challenges in Fine-Grained Authorization Implementation
Fine-grained authorization is an essential aspect of securing applications, particularly in today’s data-rich digital environment. As systems scale and become increasingly complex, the need for detailed access control arises. This blog post delves into the challenges that come with implementing fine-grained authorization and discusses how to effectively overcome them.
What is Fine-Grained Authorization?
Fine-grained authorization allows for specific access control at a very detailed level, enabling developers to set up rules that define what users can access based on countless criteria—like roles, attributes, or even real-time conditions. This granularity is key for ensuring that users only have access to the information they require, thereby enhancing security.
The Importance of Fine-Grained Authorization
- Data Security: Protects sensitive information by limiting access.
- Compliance: Helps organizations meet regulatory requirements.
- User Trust: Builds confidence in the application’s security posture.
For more in-depth insights on authorization models, you may explore the NIST Cybersecurity Framework that outlines best practices for securing applications.
Challenges in Implementing Fine-Grained Authorization
While the necessity of fine-grained authorization is clear, the path to successful implementation is often riddled with hurdles. Let's break down the most common challenges and their solutions.
1. Complexity of Rules
Challenge
Creating and managing intricate rule sets can become overwhelming. As the number of users and data points grows, so does the complexity of the corresponding authorization rules.
Solution
Make use of policy-based access controls. Utilize a framework like XACML (eXtensible Access Control Markup Language) to define rules in a more manageable way.
Example Code Snippet
import org.w3c.dom.Document;
import org.w3c.dom.Element;
public class PolicyExample {
public Document createPolicy() {
// Create a new document for the XACML Policy
DocumentBuilderFactory docFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder docBuilder = docFactory.newDocumentBuilder();
Document doc = docBuilder.newDocument();
// Create the root element
Element policy = doc.createElement("Policy");
policy.setAttribute("PolicyId", "SamplePolicy");
policy.setAttribute("Version", "1.0");
doc.appendChild(policy);
return doc;
}
}
Commentary
In this snippet, we create an XML document representing a policy. XACML allows you to easily adjust and scale your policies without manually altering each rule individually. Thus, maintaining clarity becomes simpler.
2. Performance Implications
Challenge
Fine-grained authorization can lead to performance degradation. Each access decision requires intricate checks, consuming time and resources.
Solution
Implement caching strategies. Utilize a cache to store frequently accessed permission checks, thereby minimizing unnecessary computation.
Example Code Snippet
import java.util.HashMap;
import java.util.Map;
public class AuthorizationCache {
private Map<String, Boolean> cache = new HashMap<>();
public boolean checkPermission(String userId, String resource) {
String key = userId + ":" + resource;
if (cache.containsKey(key)) {
return cache.get(key); // Return cached result
}
boolean hasAccess = performAccessCheck(userId, resource);
cache.put(key, hasAccess); // Cache the result
return hasAccess;
}
private boolean performAccessCheck(String userId, String resource) {
// Perform complex authorization checks here
return true; // Placeholder logic
}
}
Commentary
In the above example, we employ a simple caching mechanism for authorization checks. By storing results, we reduce the overhead of repeatedly performing the same checks. A robust caching solution can significantly enhance performance, enabling faster response times.
3. User Management
Challenge
Managing user roles and permissions in a fine-grained system is often resource-intensive. Changes in user roles can lead to cascading changes in permissions.
Solution
Use an automated process for role management. Consider implementing a Role-Based Access Control (RBAC) system that allows for bulk changes to user roles and permissions.
Example Code Snippet
import java.util.ArrayList;
import java.util.List;
public class RoleManager {
private List<UserRole> userRoles = new ArrayList<>();
public void assignRole(String userId, String newRole) {
for (UserRole ur : userRoles) {
if (ur.getUserId().equals(userId)) {
ur.setRole(newRole); // Update role
break;
}
}
}
// Additional methods for role methods, checks, etc.
}
Commentary
This code snippet introduces a simple system where roles can be easily assigned to users. It’s essential to maintain this modularity so that adjusting roles won’t require altering everything across your application.
4. Inadequate Documentation
Challenge
Fine-grained authorization mechanisms can lead to confusion if not well documented. Developers may face challenges understanding the intricacies of the rules.
Solution
Invest time in creating comprehensive documentation that explains each rule, its purpose, and how it fits into the overall security mechanism of the application.
Example Documentation Structure
- Overview: Introduction to fine-grained authorization and its importance.
- Rules: Detailed explanation of each authorization rule.
- User Roles: Descriptions of user roles and associated permissions.
- Implementation Guide: Step-by-step instructions for adding new rules.
Establishing clear documentation will help team members understand and apply fine-grained authorization effectively.
5. Regulatory Compliance
Challenge
Staying in compliance with data protection laws (e.g., GDPR, HIPAA) is a continuous challenge that requires meticulous attention to authorization rules.
Solution
Regularly audit your system's permissions against compliance requirements. Automated tools can help streamline this process by flagging non-compliant roles or access rules.
Closing Remarks
Implementing fine-grained authorization is not without its challenges, but these hurdles can be effectively managed with thoughtful strategies. By employing policy-based frameworks, optimizing performance, and maintaining informative documentation, you can ensure your authorization system is both effective and efficient.
For more about the context of fine-grained authorization, check out OWASP's guidelines for application security best practices.
Enhancing your application's security through fine-grained authorization is a worthwhile investment. Your data deserves it.