Common Mistakes When Using Volatile in Java Explained
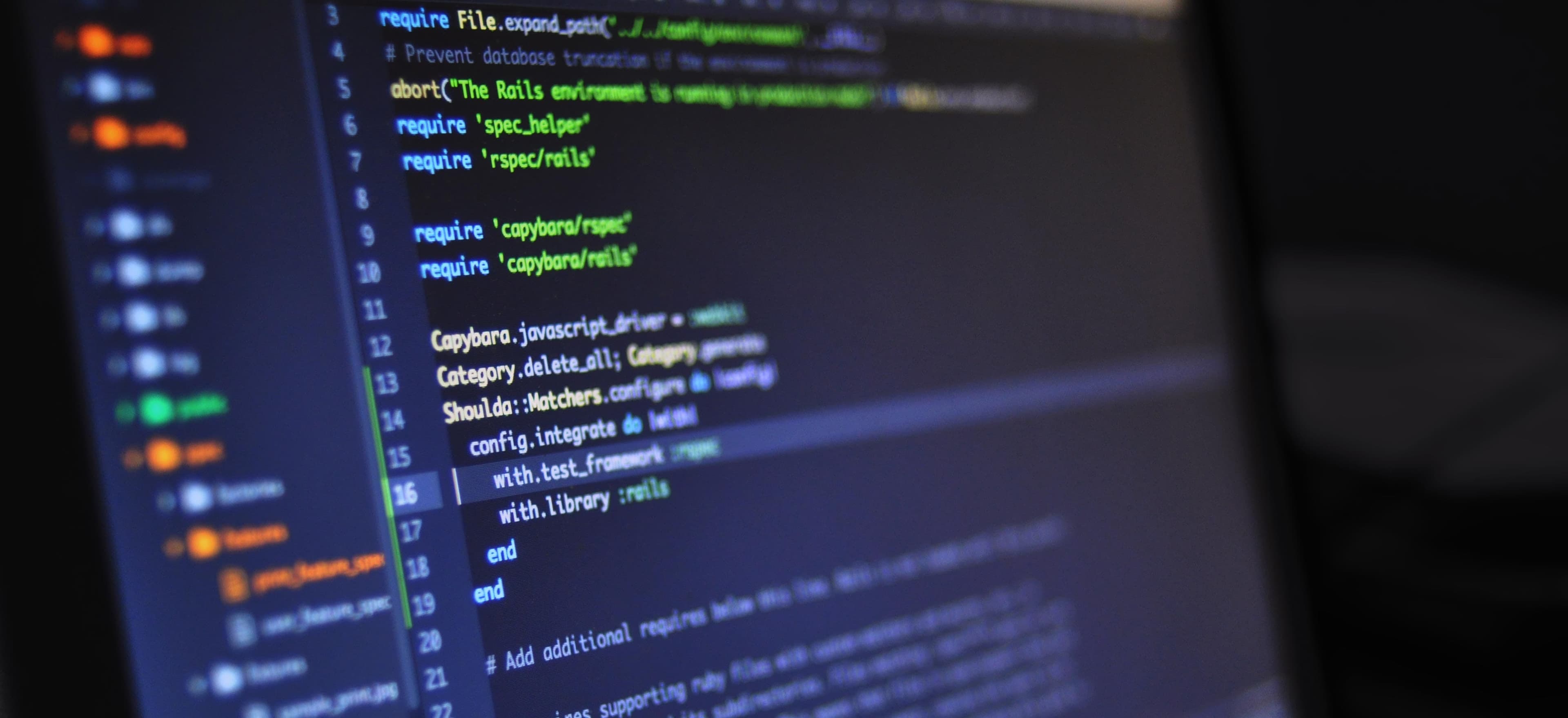
- Published on
Common Mistakes When Using Volatile in Java Explained
In the world of concurrent programming, ensuring data consistency while minimizing the risk of thread interference can be a daunting task. Java's volatile
keyword plays a vital role in the toolbox of concurrency controls, enabling developers to manage shared variables safely. However, misusing volatile
can lead to obscure bugs and instability. In this blog post, we will dive deep into what volatile
does, outline common pitfalls, and explore best practices when using this feature in your Java applications.
Understanding the volatile
Keyword
The volatile
keyword in Java is used to indicate that a variable's value will be modified by different threads. When a field is declared volatile
, it tells the Java compiler and runtime to read the variable from the main memory, rather than from the thread's local cache, ensuring visibility of changes to all threads.
Here's a simple example of how volatile
works:
public class VolatileExample {
private volatile boolean running = true;
public void stop() {
running = false; // other threads can see this change
}
public void run() {
while (running) {
// Do some work
}
}
}
Why Use volatile
?
- Visibility: A write to a
volatile
variable happens-before subsequent reads of the same variable, ensuring visibility across threads. - Preventing Caching:
volatile
prevents the compiler from optimizing variable access by keeping data in local memory caches, which can delay updates.
Common Mistakes with volatile
While volatile
is a powerful feature, it is often misunderstood. Let's discuss several common mistakes developers make when using it.
1. Assuming volatile
Guarantees Atomicity
One of the biggest misconceptions about volatile
is that it guarantees atomic operations on the variable it modifies. However, volatile
does not provide atomicity for compound actions like incrementing a value.
Consider the following code snippet:
public class Counter {
private volatile int count = 0;
public void increment() {
count++; // This is NOT atomic
}
}
Explanation
Here, count++
consists of three operations: reading the value, incrementing it, and writing it back. The use of volatile
does not make this operation atomic, meaning if multiple threads call increment()
simultaneously, they might read the same value before any updates are written back, leading to lost updates.
Best Practice
For atomic operations, use AtomicInteger
from java.util.concurrent.atomic
package:
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet(); // This is atomic
}
}
2. Using volatile
Instead of Synchronization
Sometimes, developers use volatile
in place of proper synchronization to manage complex interactions between threads. This can lead to race conditions.
public class SharedObject {
private volatile String value;
public synchronized void updateValue(String newValue) {
value = newValue;
}
}
Explanation
In this case, volatile
alone does not manage the constructor's state or the value's consistency, which requires synchronization to ensure that the variable is both read and updated safely.
Best Practice
Use synchronized blocks or methods when you need mutual exclusion. Consider using ReentrantLock
for more complex locking strategies:
import java.util.concurrent.locks.ReentrantLock;
public class SafeSharedObject {
private String value;
private ReentrantLock lock = new ReentrantLock();
public void updateValue(String newValue) {
lock.lock();
try {
value = newValue;
} finally {
lock.unlock();
}
}
}
3. Incorrectly Combining volatile
with if
Statements
Another common mistake is using volatile
variables with if
statements to check conditions, which can lead to unexpected behavior.
public class ConditionExample {
private volatile boolean flag = false;
public void execute() {
while (true) {
if (flag) {
break; // This check might not see updates by other threads
}
// Do some work...
}
}
}
Explanation
In this scenario, if one thread flips the flag
to true
, there is no guarantee that the thread running execute()
will observe this change immediately. This happens because a while
loop does not provide an adequate safety mechanism for visibility or ordering guarantees.
Best Practice
Use volatile
in combination with synchronized blocks or leverage higher-level constructs, like CountDownLatch
or Semaphore
, to manage concurrency based on conditions:
import java.util.concurrent.CountDownLatch;
public class LatchExample {
private CountDownLatch latch = new CountDownLatch(1);
public void waitForFlag() throws InterruptedException {
latch.await(); // Waits until latch is counted down
// Proceed with execution
}
public void setFlag() {
latch.countDown(); // Notify waiting thread
}
}
4. Overusing volatile
for Performance
Developers are sometimes tempted to declare many fields as volatile
in hopes of improving performance without fully understanding the implications. While volatile
variables reduce cache coherence issues, they can introduce more frequent reads from main memory, potentially resulting in performance degradation rather than improvement.
Explanation
Each read or write to a volatile
variable incurs a performance penalty because of the additional synchronization with main memory. If most threads only need to write and read within their caches, using volatile
may needlessly complicate the design.
Best Practice
Use volatile
judiciously. Analyze whether it is truly necessary for the specific use case. Attempt to use concurrent data structures, which often encapsulate the necessary synchronization mechanisms:
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentDataExample {
private ConcurrentHashMap<String, String> map = new ConcurrentHashMap<>();
public void putValue(String key, String value) {
map.put(key, value); // Thread-safe without needing volatile
}
}
Wrapping Up
While the volatile
keyword serves as a useful tool in Java's concurrency toolkit, it is not a one-stop solution for thread safety. Developers must understand its limitations and use it correctly to avoid common pitfalls, such as assuming atomicity or using it improperly within control structures.
By combining volatile
with other synchronization mechanisms and high-level concurrency constructs, you can build more robust and thread-safe applications. When in doubt, consult the Java Concurrency in Practice book for deeper insights into threading and concurrency design strategies.
Feel free to share your thoughts or experiences using volatile
in your projects. How have you ensured data consistency in a multi-threaded environment? Let’s continue the conversation!
Checkout our other articles