Understanding JVM, JRE, JDK: Common Confusions Explained
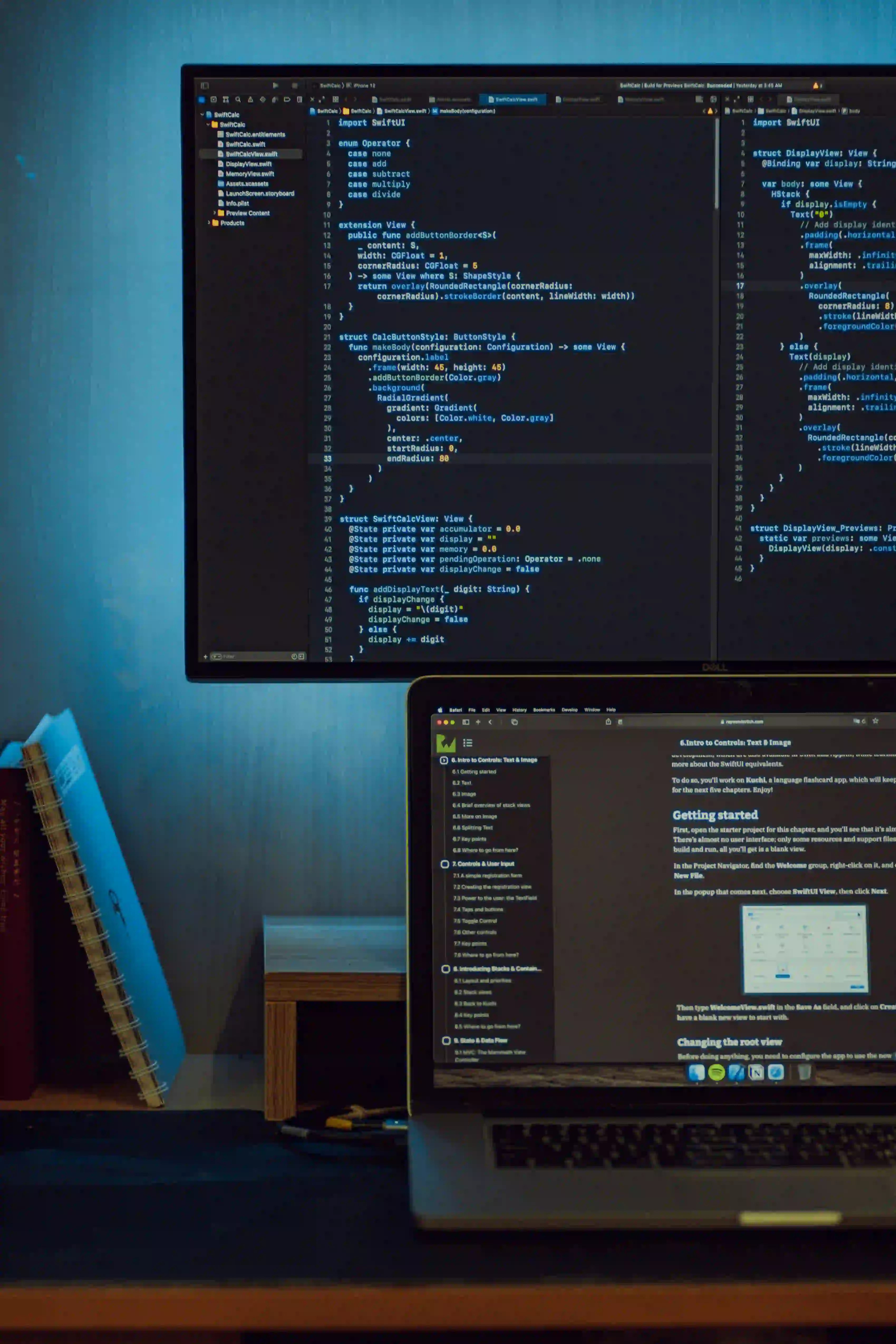
Understanding JVM, JRE, JDK: Common Confusions Explained
Java has long been a staple language in the world of programming. However, many beginners find themselves confused about the core components that make Java work: the Java Virtual Machine (JVM), Java Runtime Environment (JRE), and Java Development Kit (JDK). Each of these has a specific role in the Java ecosystem, and understanding them is vital for any programmer aspiring to work in Java. In this article, we will demystify these components and help you navigate the sometimes murky waters of Java.
What is JVM?
The Java Virtual Machine (JVM) is the heart of the Java ecosystem. It is an abstract computing machine that enables a computer to run Java programs and programs written in other languages that are also compiled to Java bytecode.
Key Responsibilities of JVM
- Loading Code: The JVM loads class files, which are outputted by the Java compiler.
- Verifying Code: The JVM performs validation checks to ensure that the bytecode adheres to the rules of Java.
- Executing Code: At runtime, the JVM interprets or compiles bytecode to machine-level instructions that the hardware can execute.
- Memory Management: The JVM is responsible for memory allocation and garbage collection, ensuring that your application doesn't run out of memory.
Code Example: Hello World using JVM
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
When you compile this code using the Java compiler (javac
), it generates a class file called HelloWorld.class
. The JVM then executes this bytecode when you run the program using the java
command.
Why is JVM Important?
Understanding the JVM is crucial because it defines how Java achieves "Write Once, Run Anywhere" (WORA). The JVM abstracts the underlying operating system, providing a consistent environment for Java applications. Whether you run this program on Windows, macOS, or Linux, as long as the appropriate JVM is installed, the program will execute in the same way.
What is JRE?
The Java Runtime Environment (JRE) is a part of the Java Development Kit but specifically designed to allow the execution of Java applications. It consists of the JVM along with the libraries and other files needed to run Java applications.
Key Components of JRE
- JVM: The engine that runs Java bytecode, as described above.
- Set of Libraries: The JRE includes essential libraries, commonly used by applications, such as the Java Class Library (JCL).
- Other Components: It also includes the necessary files that support core Java functionalities, such as the security tools and management modules.
When to Use JRE?
If you are merely looking to run Java applications and have no intention of developing your own applications, the JRE is sufficient. It provides you everything you need to execute Java programs but doesn't include the tools for development like compilers.
Code Example: Using JRE
Imagine you want to run a pre-compiled Java application stored in MyApp.jar
. You would execute it using:
java -jar MyApp.jar
This command uses the JRE to invoke the JVM, which in turn runs the application coded inside the JAR file.
What is JDK?
The Java Development Kit (JDK) is a software development environment used for developing Java applications. It includes everything the JRE has, along with tools to develop, compile, debug, and document Java programs.
Key Components of JDK
- JRE: The JDK includes the full JRE so you can run applications.
- Compiler (
javac
): This is the tool responsible for converting Java source code files (.java
) into bytecode (.class
files). - Debugging Tools: These tools assist in tracking down code issues, helping developers debug effectively.
- JavaDoc: A tool for generating documentation from Java source code.
When Should You Use JDK?
If you are a developer seeking to write and compile your own Java applications, you need the JDK. It bundles everything together, ensuring that you have the necessary components to build and run your Java applications seamlessly.
Code Example: Compiling and Running a Java Program with JDK
Let's go back to our HelloWorld
example. If you have saved this code in a file named HelloWorld.java
, you would compile and run it as follows:
javac HelloWorld.java # Compiles Java source code to bytecode
java HelloWorld # Runs the Java application
With javac
, the Java compiler translates your source code into bytecode, which is then executed by the JVM when you use the java
command.
JVM, JRE, JDK: A Quick Comparison
| Feature | JVM | JRE | JDK | |------------------------------|---------------------|----------------------------------------|-------------------------------------------------------------------------------------------------------------------------------------| | Purpose | Runs Java programs | Provides runtime environment | Development toolkit enforcing standards for building Java applications | | Components | Core of Java | JVM + Libraries + Other supporting files | JRE + Compiler + Debugger + Tools for documentation like JavaDoc | | Users | Anyone executing Java apps | End-users wanting to run Java apps | Developers looking to create, compile, and debug Java applications | | Installation Size | Small | Small to medium | Larger due to additional development tools | | Java Version | Specific | Matches JVM | Matches JRE but with additional features |
Common Confusions Explained
-
Can I run Java applications without JDK? Yes, you can run Java applications using just the JRE, as it includes the JVM. The JDK is necessary for development only.
-
Is JRE and JVM the same? No, the JRE encompasses the JVM but also includes libraries and other components necessary for running Java applications.
-
What if I only install JDK? Installing the JDK also gives you the JRE. This makes it a popular choice for developers, as they can compile, debug, and run applications all in one kit.
-
Do I need separate JVM installations? Most cases don’t require separate installations. The JVM comes packaged with both the JDK and the JRE.
Closing the Chapter
Understanding the distinction between JVM, JRE, and JDK is an essential step in mastering Java. Each component plays a specific, vital role in the Java ecosystem.
- JVM allows execution of bytecode and provides an abstraction layer.
- JRE is tailored for running Java applications.
- JDK is a comprehensive suite for developing and compiling Java programs.
It is this interconnectedness that forms the backbone of Java's platform-independent philosophy. For further reading on these facets, you can explore Oracle’s official Java documentation and Java's architecture overview.
By comprehensively understanding these components, you will not only sharpen your Java skills but also equip yourself to tackle more complex programming challenges ahead. Happy coding!