Choosing the Fastest Serialization Format: Java vs JSON vs Kryo
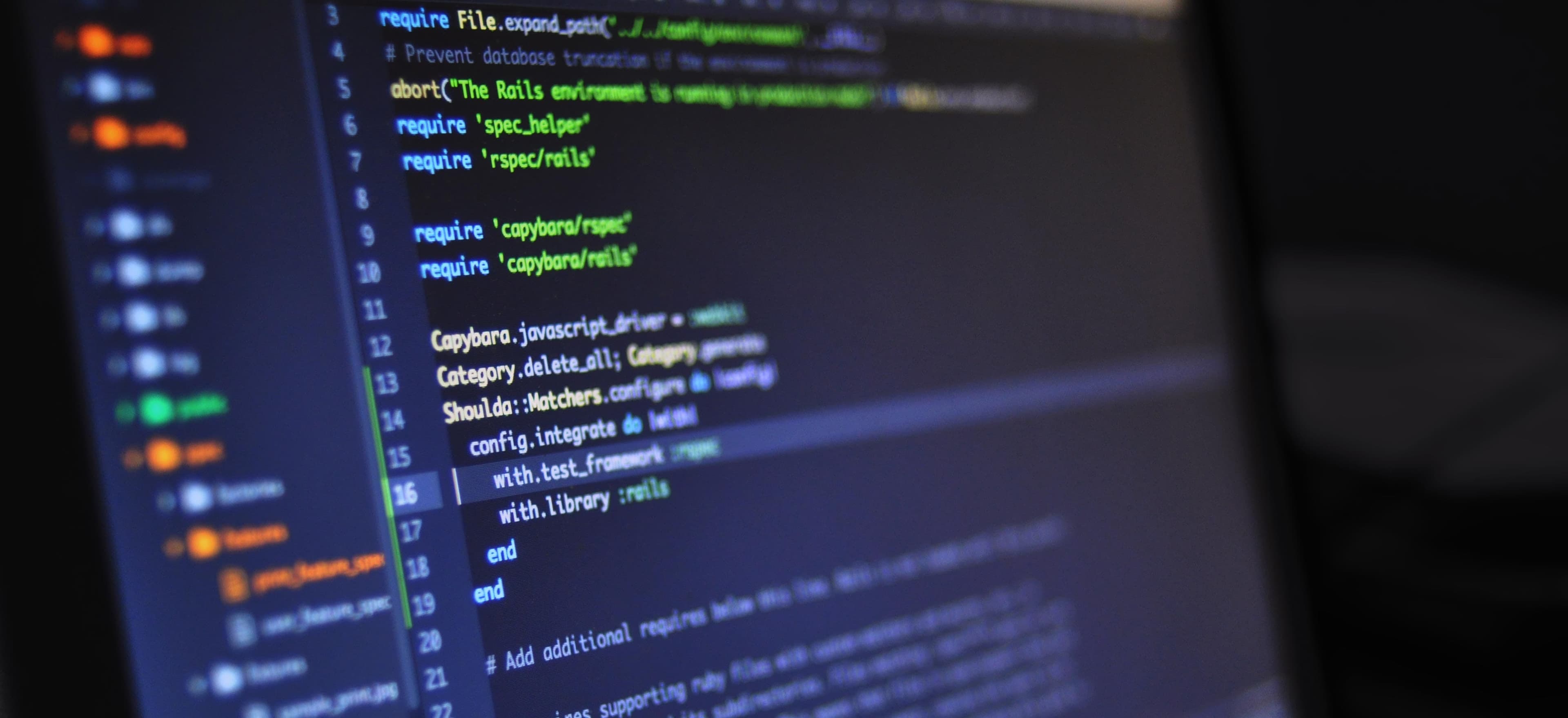
- Published on
Choosing the Fastest Serialization Format: Java vs JSON vs Kryo
Serialization is a crucial aspect of software development, primarily when dealing with data transmission and storage in distributed systems. A well-chosen serialization format can significantly impact performance, making it vital to understand your options. This article will discuss three popular serialization formats: Java Serialization, JSON, and Kryo. We will compare their performance and use cases, providing code snippets and commentary to illustrate their characteristics.
What is Serialization?
Serialization is the process of converting an object into a byte stream. This stream can be easily stored in a file or transmitted over a network. Deserialization is the reverse process, where the byte stream is transformed back into an object. Here’s why serialization matters:
- Data Transfer: To transmit object data over networks.
- Data Persistence: To store object states in files or databases.
- Interoperability: Different programming languages can read the same serialized format.
Overview of Java Serialization
Java offers its built-in serialization mechanism through the Serializable
interface. Objects that implement this interface can be converted into byte streams.
Pros of Java Serialization
- Integrated Support: Being a part of the Java language, it supports any Java object without additional dependencies.
- Ease of Use: Implementing
Serializable
is straightforward.
Cons of Java Serialization
- Performance: It is relatively slow due to the overhead of metadata and reflection.
- Versioning Issues: Modifications to classes can lead to
InvalidClassException
at runtime.
Example: Java Serialization
Here's a sample code snippet demonstrating Java serialization.
import java.io.*;
class Person implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Person{name='" + name + "', age=" + age + '}';
}
}
public class JavaSerializationExample {
public static void main(String[] args) {
Person person = new Person("Alice", 30);
try {
// Serialize object
FileOutputStream fileOut = new FileOutputStream("person.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(person);
out.close();
fileOut.close();
// Deserialize object
FileInputStream fileIn = new FileInputStream("person.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Person deserializedPerson = (Person) in.readObject();
in.close();
fileIn.close();
System.out.println("Deserialized Person: " + deserializedPerson);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Commentary on the Code:
- Serializable Interface: The
Person
class implements theSerializable
interface, making it eligible for serialization. - Serialization Process: Using
ObjectOutputStream
, we serialize the object and store it in a file. - Deserialization Process: Using
ObjectInputStream
, we read the object back from the file.
Overview of JSON Serialization
JavaScript Object Notation (JSON) is a lightweight data interchange format that is easy for humans to read and write and for machines to parse and generate. It is language-independent, which promotes interoperability.
Pros of JSON Serialization
- Human-Readable: JSON is easy to read and understand, making it great for configuration files and debugging.
- Wide Language Support: Most programming languages have libraries for parsing and generating JSON.
Cons of JSON Serialization
- Performance: JSON can be slower than binary formats due to text-based nature.
- Type Limitations: JSON does not support all data types (e.g., Java-specific types).
Example: JSON Serialization with Jackson
Here's a snippet using the Jackson library for JSON serialization.
import com.fasterxml.jackson.databind.ObjectMapper;
class Person {
private String name;
private int age;
public Person() {} // Default constructor needed for JSON deserialization
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
public class JsonSerializationExample {
public static void main(String[] args) {
ObjectMapper objectMapper = new ObjectMapper();
Person person = new Person("Bob", 25);
try {
// Convert object to JSON
String jsonString = objectMapper.writeValueAsString(person);
System.out.println("JSON String: " + jsonString);
// Convert JSON back to object
Person deserializedPerson = objectMapper.readValue(jsonString, Person.class);
System.out.println("Deserialized Person: " + deserializedPerson.getName() + ", Age: " + deserializedPerson.getAge());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on the Code:
- Jackson Library: We use
ObjectMapper
from the Jackson library for JSON processing. Ensure you have it in yourpom.xml
. - Serialization and Deserialization: We convert the Java
Person
object to a JSON string and back seamlessly.
Dependency for Jackson:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.4</version>
</dependency>
Overview of Kryo Serialization
Kryo is an efficient serialization framework for Java that emphasizes speed and compactness.
Pros of Kryo Serialization
- Speed: Kryo is significantly faster than both Java Serialization and JSON.
- Compactness: It produces smaller byte streams.
Cons of Kryo Serialization
- Complexity: Requires configuration for complex types.
- Dependencies: You need to include Kryo as a dependency in your project.
Example: Kryo Serialization
Here’s a simple code snippet to demonstrate Kryo serialization.
import com.esotericsoftware.kryo.Kryo;
import com.esotericsoftware.kryo.io.KryoInput;
import com.esotericsoftware.kryo.io.KryoOutput;
import java.io.*;
class Person {
private String name;
private int age;
public Person() {} // Default constructor needed for Kryo deserialization
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Person{name='" + name + "', age=" + age + '}';
}
}
public class KryoSerializationExample {
public static void main(String[] args) {
Kryo kryo = new Kryo();
Person person = new Person("Charlie", 35);
try {
// Serialize object
Output output = new Output(new FileOutputStream("person.kryo"));
kryo.writeObject(output, person);
output.close();
// Deserialize object
Input input = new Input(new FileInputStream("person.kryo"));
Person deserializedPerson = kryo.readObject(input, Person.class);
input.close();
System.out.println("Deserialized Person: " + deserializedPerson);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary on the Code:
- Kryo Class: We create an instance of the
Kryo
class for serialization. - Output/Input Streams: Kryo uses its
Output
andInput
classes to handle serialized data operations.
Dependency for Kryo:
<dependency>
<groupId>com.esotericsoftware.kryo</groupId>
<artifactId>kryo</artifactId>
<version>5.0.6</version>
</dependency>
Performance Comparison
While all three serialization formats can handle object serialization, performance can vary:
- Java Serialization: Slower due to overhead; not recommended for high-performance applications.
- JSON: Slower than Kryo but easier to work with for data interchange.
- Kryo: Fastest and most efficient in terms of space; ideal for high-load systems.
Summary
When selecting a serialization format, consider factors such as speed, ease of use, and data requirements. Java Serialization might be suitable for smaller projects or where ease of implementation is key. JSON shines in API communications due to its human-readable format. Finally, Kryo is the best choice for performance-critical applications.
For further reading, check out Java Documentation on Serialization and the Kryo GitHub Repository.
Ultimately, the best serialization format will depend on the specific requirements of your application, so weigh the pros and cons carefully to make an informed decision.