Mastering Java 8: Overcoming Scala Type Challenges
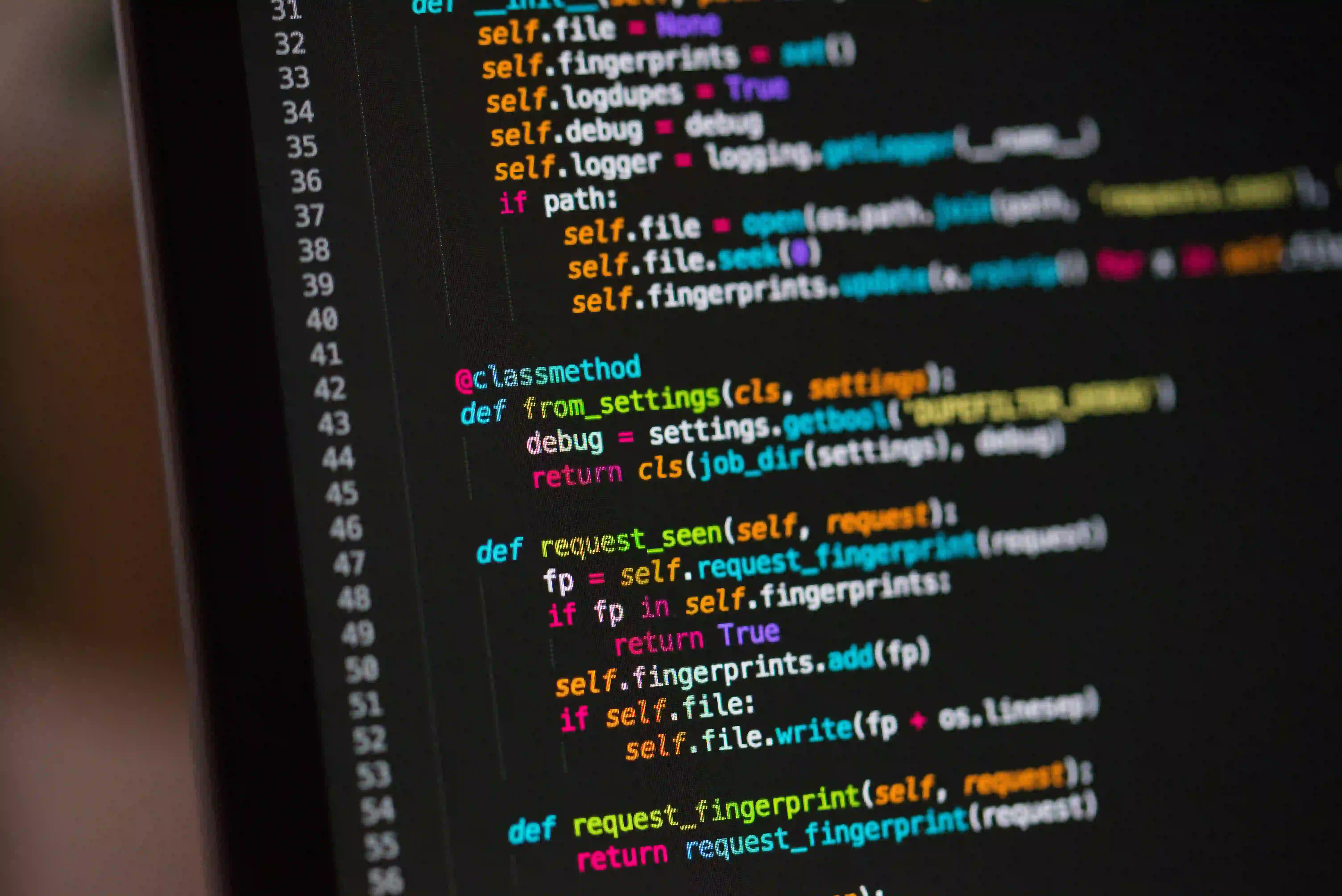
Mastering Java 8: Overcoming Scala Type Challenges
Java and Scala are two prominent languages in the JVM ecosystem, each with its unique strengths and challenges. As Java developers explore modern programming paradigms, they often encounter Scala's advanced type system. This blog post delves into how Java 8 features can help you overcome Scala's type challenges, enhancing code clarity while leveraging functional programming's power.
Understanding Type Challenges in Scala
Scala is known for its rich type system that combines object-oriented and functional programming concepts. This brings about several advantages—such as type inference and case classes—while adding complexity that Java developers might find challenging. Here are common Scala type issues:
-
Type Inference: Scala’s ability to infer types can lead to confusion. While it minimizes boilerplate, new Scala users might not understand the underlying types.
-
Implicits: Implicit parameters and conversions can obfuscate code reading. They allow Scala to be highly abstract, yet they can make the code less predictable.
-
Variance Annotations: Scala uses variance annotations, which can be a double-edged sword. Using
Covariant
,Contravariant
, orInvariant
in type definitions can lead to flexibility but also confusion for developers from strongly typed backgrounds.
Java 8 provides several features, such as lambda expressions, the Stream API, and new interfaces, enabling developers to tackle these challenges effectively.
Leveraging Java 8 Features
1. Lambda Expressions
Java 8 introduced lambda expressions to allow developers to write clearer, more concise code. They serve a similar purpose to Scala's anonymous functions but within Java's type-safe framework.
Example: Filtering a list using a lambda expression.
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class LambdaExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Filtering names that start with 'C'
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("C"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [Charlie]
}
}
Why the Code Matters: In the above example, lambda expressions provide clear and concise syntax for filtering collections. You can immediately see the conditions under which elements are retained, providing clarity similar to Scala's functional expressions but without the potential obscurity from implicit conversions or type inference.
2. The Stream API
The Stream API allows for functional-style operations on streams of data. It enables parallel processing and lazy evaluations, similar to Scala's collections.
Example: Summing up even numbers from a list.
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
int sumOfEvens = numbers.stream()
.filter(n -> n % 2 == 0)
.mapToInt(Integer::intValue)
.sum();
System.out.println("Sum of even numbers: " + sumOfEvens); // Output: 12
}
}
Why the Code Matters: The Stream API transforms complex operations into a chain of methods that are easy to read and maintain. In a Scala context, this is similar to using filter
, map
, and reduce
, but Java developers can rely on their familiar type system and avoid unexpected behavior caused by Scala's type inference.
3. Optional Class
One of the most significant introductions in Java 8 is the Optional
class, which serves as a container for potentially null values, reminiscent of Scala’s Option
.
Example: Using Optional
to avoid null references.
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
Optional<String> optionalName = getName();
// Using ifPresent to handle the value safely
optionalName.ifPresent(name -> System.out.println("The name is: " + name));
// Providing a default value
String defaultName = optionalName.orElse("Default Name");
System.out.println("The name is: " + defaultName);
}
public static Optional<String> getName() {
return Optional.ofNullable(null); // Simulating a null return
}
}
Why the Code Matters: The Optional
class provides a robust solution to avoid NullPointerException
, a common culprit in Java. It pushes developers to think proactively about null values, similar to how Scala’s Option
requires conscious handling of possible empty states.
4. Default Methods
Java 8's introduction of default methods allows you to add new functionalities to interfaces without breaking existing implementations. This feature brings a more Scala-like feel to Java interfaces by allowing for more flexible extension.
Example: Defining a default method in an interface.
interface Vehicle {
void drive();
default void honk() {
System.out.println("Beep beep!");
}
}
class Car implements Vehicle {
public void drive() {
System.out.println("Car is driving.");
}
}
public class DefaultMethodsExample {
public static void main(String[] args) {
Vehicle car = new Car();
car.drive(); // Output: Car is driving.
car.honk(); // Output: Beep beep!
}
}
Why the Code Matters: Default methods enable developers to define standard behavior that all subclasses can inherit. This functionality is similar to Scala traits and provides a more seamless evolution of interfaces.
Reducing Complexity
By using Java 8 features, developers can avoid some of the complexities encountered in Scala, while still leveraging powerful programming constructs. Here are some strategies to keep your code clean and maintainable:
-
Favor Clearer Syntax: Use lambda expressions and the Stream API, keeping the syntax straightforward. Avoid overly complex constructs as they can detract from code readability.
-
Utilize Optional Wisely: Always consider whether your methods might return null. Instead of allowing nulls, encapsulate them in an
Optional
. This practice eliminates ambiguity about the existence of a value. -
Implement Interfaces with Default Methods: Default methods can simplify code maintenance, allowing changes in interfaces without affecting dependent classes.
-
Break Down Large Functions: Adhere to the single responsibility principle by breaking down large functions into smaller, reusable utilities.
-
Document the ‘Why’ Approach: Comments should focus on why the code does what it does, helping future developers (and you!) understand the rationale behind design decisions.
Key Takeaways
Java 8 introduced several features that significantly improve the way we write code, especially when tackling challenges faced by Scala developers. Understanding and utilizing lambda expressions, the Stream API, the Optional class, and default methods can empower you to write clearer, more maintainable Java code.
While Scala's type system provides flexibility, it can also create confusion, particularly for developers accustomed to Java's stricter type constraints. By embracing the robust features of Java 8, developers can navigate these complexities while enjoying the benefits of modern programming paradigms.
For additional reading on Java versus Scala's type systems and their respective libraries, explore:
- Java 8 Documentation
- Scala Type System
Master the art of writing clean, clear, and functional Java code, and watch your programming prowess flourish as you bridge the gap between Java and Scala. Happy coding!