Common Performance Issues in Jersey RESTful Applications
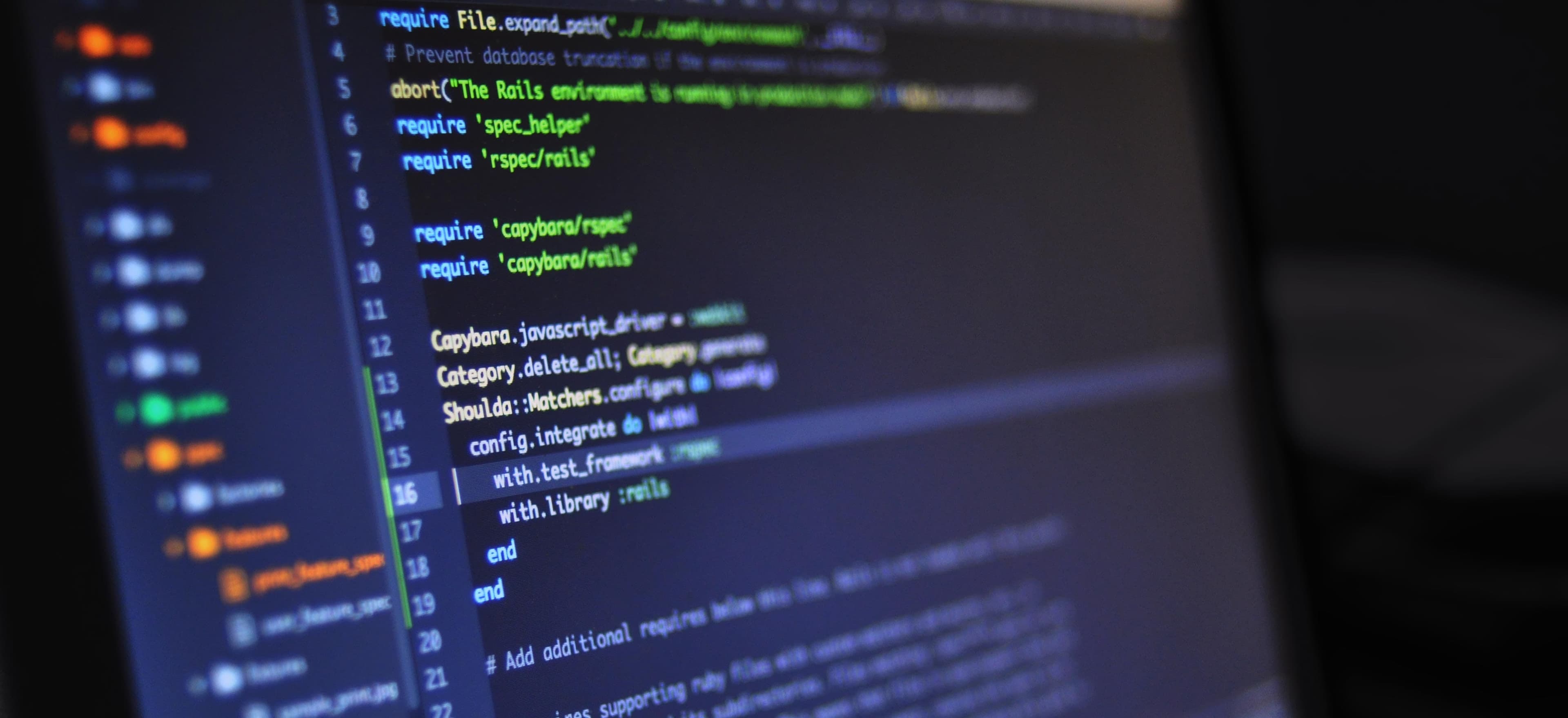
- Published on
Common Performance Issues in Jersey RESTful Applications
Creating RESTful applications using the Jersey framework can significantly enhance your software's scalability and maintainability. However, as with any technology stack, issues may arise that can degrade application performance. In this blog post, we will explore common performance problems in Jersey RESTful applications and how to address them.
Table of Contents
- Understanding Jersey Framework
- Common Performance Issues
- 2.1 Slow API Response Times
- 2.2 Inefficient Resource Handling
- 2.3 Lack of Caching
- 2.4 Unoptimized Database Queries
- Best Practices for Performance Optimization
- Conclusion
- Further Reading
Understanding Jersey Framework
Jersey is a powerful framework for developing RESTful web services in Java. It implements the Java API for RESTful Web Services (JAX-RS) and offers various features, such as content negotiation, support for multiple data formats (like JSON and XML), and built-in support for dependency injection.
It's essential to understand Jersey's architecture to diagnose performance issues effectively. The framework utilizes servlets that can become performance bottlenecks if not configured properly. Moreover, poorly structured application logic can lead to inefficient resource utilization.
Common Performance Issues
2.1 Slow API Response Times
One of the most prevalent performance issues is slow API response times. This can occur due to several factors, including:
- Large Payloads: Sending excessively large JSON or XML payloads may slow down response times.
- Inefficient Endpoints: Some endpoints may perform unnecessary computations or perform operations that block other requests.
Example
@GET
@Path("/users/{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response getUser(@PathParam("id") String userId) {
// Potentially heavy logic here could lead to slow API responses
User user = userService.getUserById(userId);
return Response.ok(user).build();
}
Solution
Optimize your API by reducing the size of the payload. Use pagination for large dataset responses. Also, ensure that business logic inside your endpoint methods does not perform unnecessary calculations.
2.2 Inefficient Resource Handling
Improper management of resources like connections and threads can lead to application sluggishness. Common issues include:
- Too Many Connections: Opening too many HTTP connections can overwhelm your server.
- Thread Blocking: Synchronous processing can block threads and delay executions.
Example
@POST
@Path("/users")
@Consumes(MediaType.APPLICATION_JSON)
public Response createUser(User user) {
// Blocking call might cause delays
userService.saveUser(user);
return Response.status(Response.Status.CREATED).build();
}
Solution
Implement asynchronous processing using @Asynchronous
in EJB or CompletableFutures in Java. This will allow you to return responses without waiting for the entire processing cycle to complete.
2.3 Lack of Caching
Failing to utilize caching techniques results in constant hits to the backend services or databases which could be avoided. There are various types of caching strategies, including:
- Response Caching: Store the results of expensive service calls.
- Entity Caching: Cache database entities to reduce the time taken to fetch repeated entities.
Example
@GET
@Path("/products/{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response getProduct(@PathParam("id") String productId) {
Product product = productService.getProductById(productId);
return Response.ok(product).build();
}
Solution
Use annotations like @CacheControl
in Jersey to specify the caching policies. Set a time-to-live (TTL) for cached endpoints to ensure the data does not become stale.
@GET
@Path("/products/{id}")
@Produces(MediaType.APPLICATION_JSON)
@CacheControl(maxAge = 60) // Cache for 60 seconds
public Response getProduct(@PathParam("id") String productId) {
Product product = productService.getProductById(productId);
return Response.ok(product).build();
}
2.4 Unoptimized Database Queries
Inefficient database queries can significantly slow down your applications, especially when handling large datasets. They might include:
- N+1 Query Problem: Querying related data one-by-one rather than in a single batch.
- Missing Indexes: Queries without proper indexes will not perform well.
Solution
Optimize your database queries to fetch related entities in a single query and ensure your database is properly indexed. Consider using JPA features such as fetching strategies to load only required data.
public List<Product> getAllProducts() {
// Inefficient: fetching product details one-by-one
return entityManager.createQuery("SELECT p FROM Product p", Product.class).getResultList();
}
Best Practices for Performance Optimization
- Monitoring and Logging: Use metrics and logging to identify bottlenecks.
- Load Testing: Regularly test your application under load to understand how it behaves under stress.
- Use Profilers: Tools like VisualVM can help identify CPU and memory bottlenecks.
- Keep Dependencies Up-To-Date: Regularly update your libraries and possibly switch to more efficient ones when necessary.
Final Thoughts
Performance issues in Jersey RESTful applications can stem from various factors, ranging from slow API response times to the absence of caching. Understanding and mitigating these common performance pitfalls can dramatically enhance your application’s efficiency and responsiveness.
By following best practices and incorporating thoughtful optimizations, developers can create robust, high-performing RESTful applications that meet user expectations without lag or delay.
Further Reading
- Jersey Documentation
- Spring’s Best Practices for REST API
- JPA Fetch Strategies
Incorporating these strategies will help ensure that your Jersey RESTful application performs optimally, providing a seamless experience for its users.