Common Security Pitfalls in Microservices Architecture
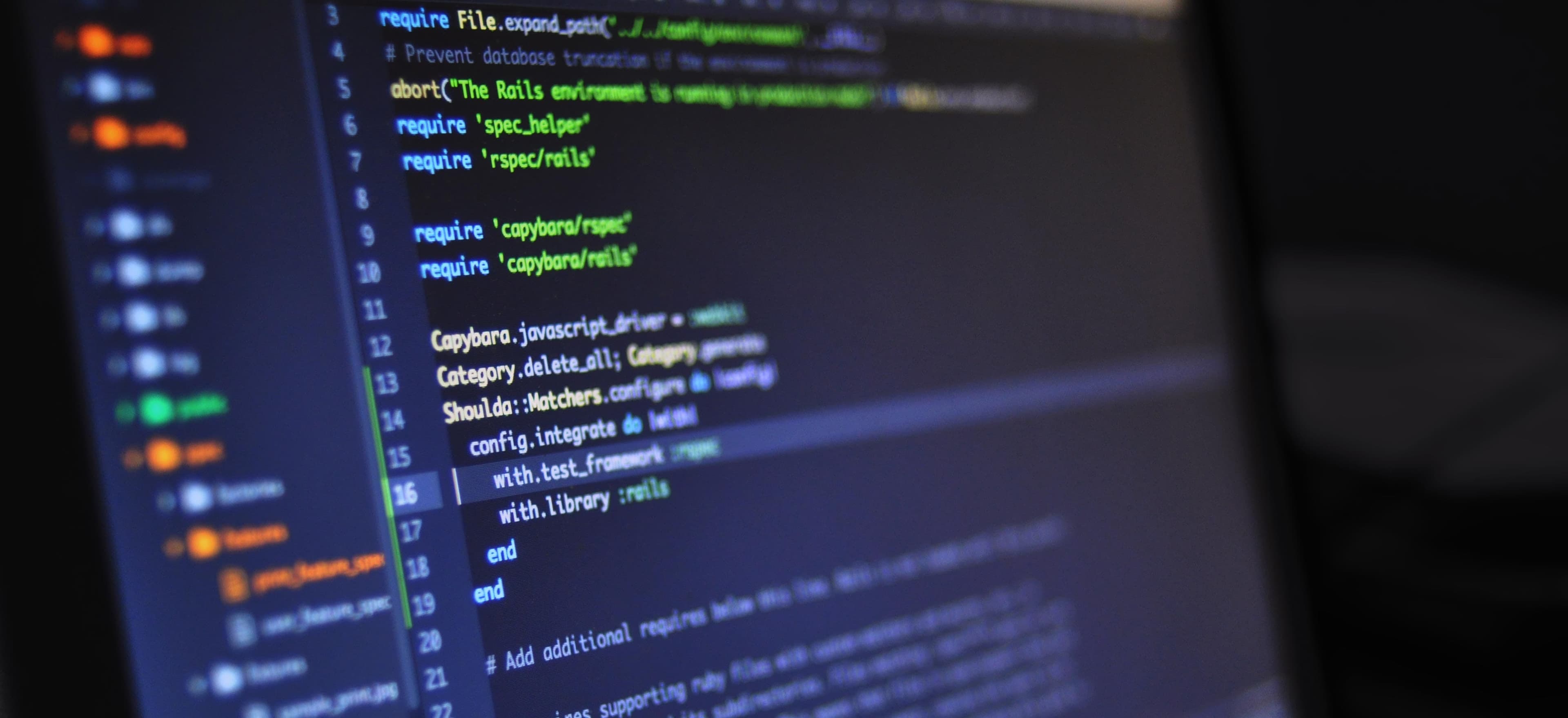
- Published on
Common Security Pitfalls in Microservices Architecture
In today's digital landscape, microservices architecture has garnered widespread adoption, offering the agility and scalability that modern applications require. However, with this architectural flexibility comes a complex web of security challenges that can expose vulnerabilities if not managed appropriately. This blog post will delve into common security pitfalls in microservices architecture, highlighting best practices and robust strategies to mitigate risks.
Understanding Microservices Architecture
Microservices architecture breaks down large applications into smaller, loosely coupled services that can be developed, deployed, and scaled independently. Each service typically performs a specific function and communicates over a network using APIs. While this design promotes faster development and resilience, it also introduces numerous security complexities.
1. Insufficient Authentication and Authorization
The Pitfall
One of the most glaring vulnerabilities in microservices is insufficient authentication and authorization. Each microservice may implement its own authentication mechanism, leading to inconsistent and often insecure implementations.
Best Practice
Implement a centralized authentication solution, such as OAuth 2.0 or OpenID Connect, to streamline authentication across services. This approach allows for unified user identity management, reducing the risk of unauthorized access.
Example Code
Here’s a simple example of using the Spring Security OAuth2 library to secure a microservice:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.oauth2Login();
}
}
Why? This code snippet configures Spring Security to require authentication for any request except those made to the public endpoints. By relying on OAuth2, we can ensure that services authenticate requests effectively and securely.
2. Overly Permissive Network Policies
The Pitfall
Microservices architectures often allow services to communicate with one another dynamically, which can lead to overly permissive network policies. If not configured correctly, this may expose sensitive services to unintended access.
Best Practice
Enforce the principle of least privilege when defining network policies. Configure firewalls or service meshes to restrict communications to only necessary services.
Example Code
If you’re using Kubernetes, you can set Network Policies to control pod communication:
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: allow-database-access
namespace: example
spec:
podSelector:
matchLabels:
app: database
ingress:
- from:
- podSelector:
matchLabels:
app: important-service
Why? This YAML file defines a network policy allowing only the specified service to communicate with the database service. By doing this, you minimize unnecessary exposure and potential attack vectors.
3. Lack of Data Encryption
The Pitfall
Many developers neglect to focus on data encryption in transit and at rest. Ensuring that sensitive data remains protected while being transmitted or stored is critical for maintaining confidentiality.
Best Practice
Use HTTPS for all service communications and encrypt sensitive data at rest using robust algorithms (like AES).
Example Code
For encrypting data at rest in a Java application:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class EncryptionUtil {
public static byte[] encrypt(String data, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(data.getBytes());
}
public static SecretKey generateKey() throws Exception {
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(128); // or 256
return keyGen.generateKey();
}
}
Why? This code uses AES for data encryption, securing sensitive information before it is stored. Encrypting data at rest helps to protect it from unauthorized access, thereby enhancing security.
4. Inadequate Logging and Monitoring
The Pitfall
Microservices can result in dispersed logs across services, making it challenging to monitor system performance or security events effectively.
Best Practice
Implement a centralized logging solution such as ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk to aggregate logs from all services. This allows you to monitor events and detect anomalies in real-time.
Example Configuration (Elasticsearch)
output:
elasticsearch:
hosts: ["http://localhost:9200"]
Why? By configuring your logging to output to Elasticsearch, you create a single source of truth for all logs. This helps in identifying unusual patterns that could indicate security threats.
5. Ignoring Service-to-Service Communication Security
The Pitfall
Microservices often communicate over HTTP, potentially exposing sensitive information to interception if not implemented securely. This can be particularly problematic if services are not within a secured network.
Best Practice
Utilize service mesh technologies such as Istio or Linkerd for secure service-to-service communication, including mutual TLS (mTLS) to encrypt traffic.
Example Command to Install Istio
kubectl apply -f istio-1.7.0/samples/addons
Why? By employing mTLS, you encrypt communication between services and authenticate service identities. This drastically reduces the possibility of man-in-the-middle attacks.
6. Poor Exception Handling
The Pitfall
Inadequate exception handling can disclose sensitive information. Microservices that expose stack traces or unexpected behavior can inadvertently leak critical data to attackers.
Best Practice
Always handle exceptions gracefully and avoid exposing stack traces or detailed error information in production environments.
Example Code
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception ex) {
// Log the exception with a logger without revealing sensitive information
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An unexpected error occurred. Please try again later.");
}
}
Why? This global exception handler provides a uniform way to handle errors in your microservices without exposing sensitive information, thus securing your application.
Final Considerations
Navigating the myriad of security pitfalls in microservices architecture requires a strategic approach and strong adherence to security best practices. By implementing centralized authentication and logging, enforcing network policies, securing data, and maintaining consistent communication protocols, you can significantly mitigate risks associated with your microservices.
For further reading on secure microservices architecture, consider exploring these resources:
- OWASP Microservices Security
- Kubernetes Network Policies
- Spring Security Reference
Investing in security from the ground up enables organizations to fully harness the advantages of microservices without compromising on safety.