Mastering Test Naming: Boost Clarity and Efficiency
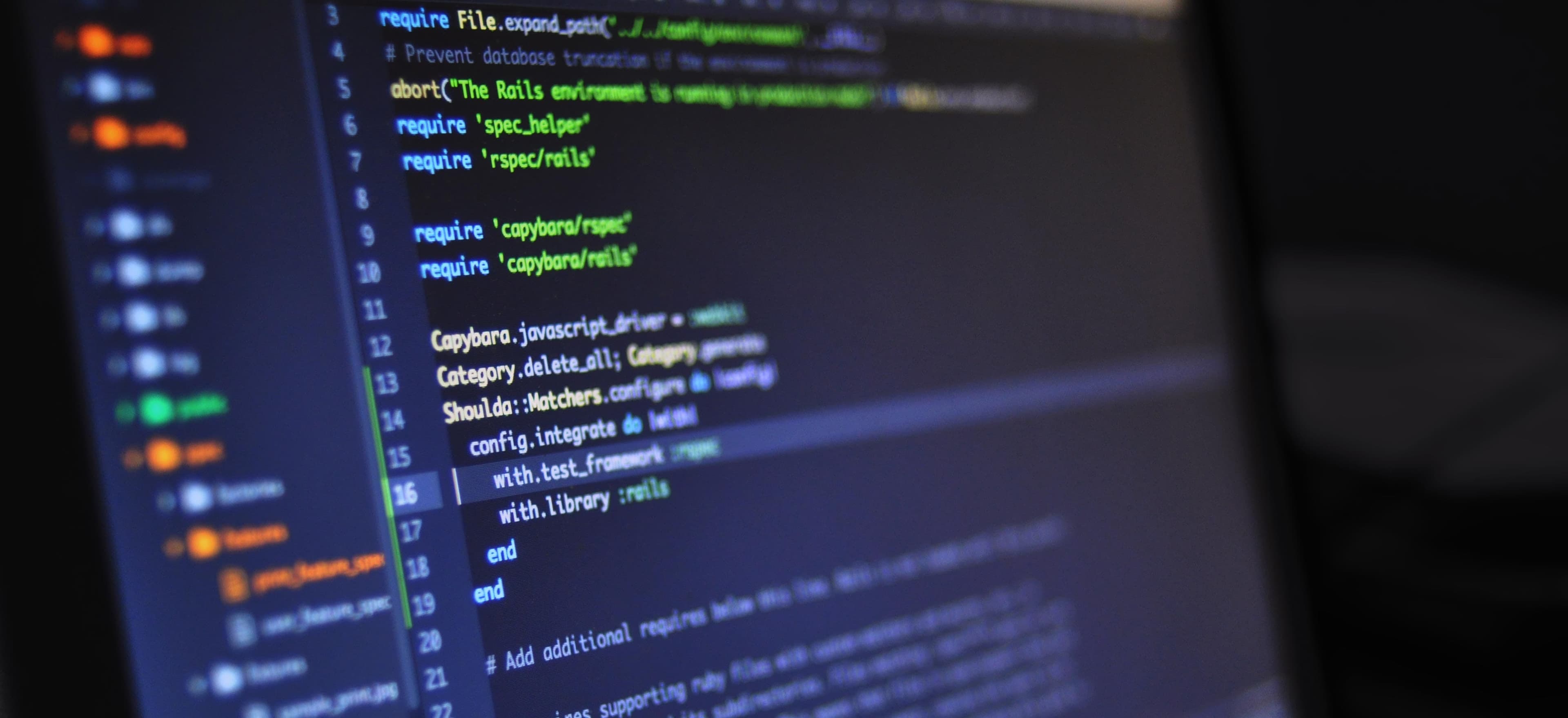
- Published on
Mastering Test Naming: Boost Clarity and Efficiency
In the world of software development, effective testing is a cornerstone of delivering a reliable product. Yet, one area that often gets overlooked is the way we name our tests. Clarity in naming is crucial not only for writing maintainable code but also for ensuring that the tests serve their purpose effectively. In this blog post, we'll explore the importance of naming tests correctly and how this can boost both clarity and efficiency in your development process.
Why Test Naming Matters
Good test names serve several key functions:
-
Clarity: A well-named test communicates what is being tested and under what conditions. This is crucial for anyone reading the test in the future, including your future self.
-
Efficiency: Developers spend less time deciphering what a test does, thus speeding up onboarding and collaboration.
-
Documentation: Well-named tests act as a form of documentation. They can help in understanding the intended functionality of the code without diving deep into it.
-
Debugging: When tests fail, a clear name can provide immediate context, making it easier to pinpoint the problem.
Guidelines for Effective Test Naming
Be Descriptive
The most fundamental principle of test naming is to be descriptive. Use a naming convention that allows anyone reading the test name to understand its purpose.
Here's an example of a poorly named test:
@Test
public void test1() {
// This test checks the sum
assertEquals(4, add(2, 2));
}
And here’s a better version:
@Test
public void shouldReturnSumOfTwoNumbers() {
assertEquals(4, add(2, 2));
}
In the second version, we clearly state what the test does, making it easier to understand.
Incorporate Context
Provide context in the test name to indicate the conditions under which it is valid. This is particularly useful for complex functionalities.
Example of a test naming that lacks context:
@Test
public void testProduct() {
// Test for product calculation
assertEquals(15, product(3, 5));
}
This can be improved by providing conditions under which the test is valid:
@Test
public void shouldReturnProductOfPositiveIntegers() {
assertEquals(15, product(3, 5));
}
Use the "Given-When-Then" Approach
A popular approach for structured naming of tests is the Given-When-Then format. This structure can enhance both readability and understanding.
- Given: The initial context.
- When: The action taken.
- Then: The expected outcome.
For instance:
@Test
public void givenTwoPositiveIntegers_whenMultiplied_thenReturnCorrectProduct() {
assertEquals(15, product(3, 5));
}
This name succinctly conveys the initial conditions, action, and expected result, improving clarity.
Keep it Concise
While being descriptive is crucial, overelaboration can lead to overly long test names. Aim for a balance. A concise yet informative test name can be more impactful.
For example, instead of:
@Test
public void testIfTheOutputIsEqualToTheExpectedValueForTwoPositiveIntegerInputs() {
assertEquals(15, product(3, 5));
}
A simpler version would be:
@Test
public void shouldReturnCorrectProductForPositiveIntegers() {
assertEquals(15, product(3, 5));
}
Avoid Ambiguity
Avoid using terms that could mean different things in different contexts. For example, if your test involves database interactions, specify it in your test name.
Consider this ambiguous name:
@Test
public void testSave() {
// Save operation
}
Instead, try:
@Test
public void shouldSaveUserInformationToDatabase() {
// Save operation
}
This leaves no room for confusion regarding what is being tested.
Code Snippet: A Complete Example
Let's put all these principles into practice with a complete example that showcases effective test naming.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void givenTwoPositiveNumbers_whenAdded_thenReturnCorrectSum() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result, "The sum of 2 and 3 should be 5");
}
@Test
public void givenNegativeAndPositiveNumber_whenAdded_thenReturnCorrectSum() {
Calculator calculator = new Calculator();
int result = calculator.add(-2, 3);
assertEquals(1, result, "The sum of -2 and 3 should be 1");
}
@Test
public void givenZeroAndNumber_whenAdded_thenReturnNumber() {
Calculator calculator = new Calculator();
int result = calculator.add(0, 5);
assertEquals(5, result, "Adding 0 to any number should return the number itself");
}
}
In the code snippet above, each test is named following the "Given-When-Then" structure. They are brief, comprehensive, and informative, making it evident what the objective of each test is.
Wrapping Up
Mastering test naming isn’t just a matter of personal preference; it's a critical aspect of ensuring your codebase remains understandable and efficient over time. Descriptive, contextual, concise, and unambiguous test names significantly enhance clarity, making day-to-day development tasks smoother.
For further reading about testing best practices, you may want to check the resources from JUnit's official website and the Clean Code book by Robert C. Martin.
By implementing the strategies discussed in this post, you’ll improve not just the quality of your tests, but the overall maintainability of your code. Remember that writing tests should be just as intentional and meticulous as writing your application code. Happy coding!