Common Pitfalls in Designing Domain Layers of Multilayered Architectures
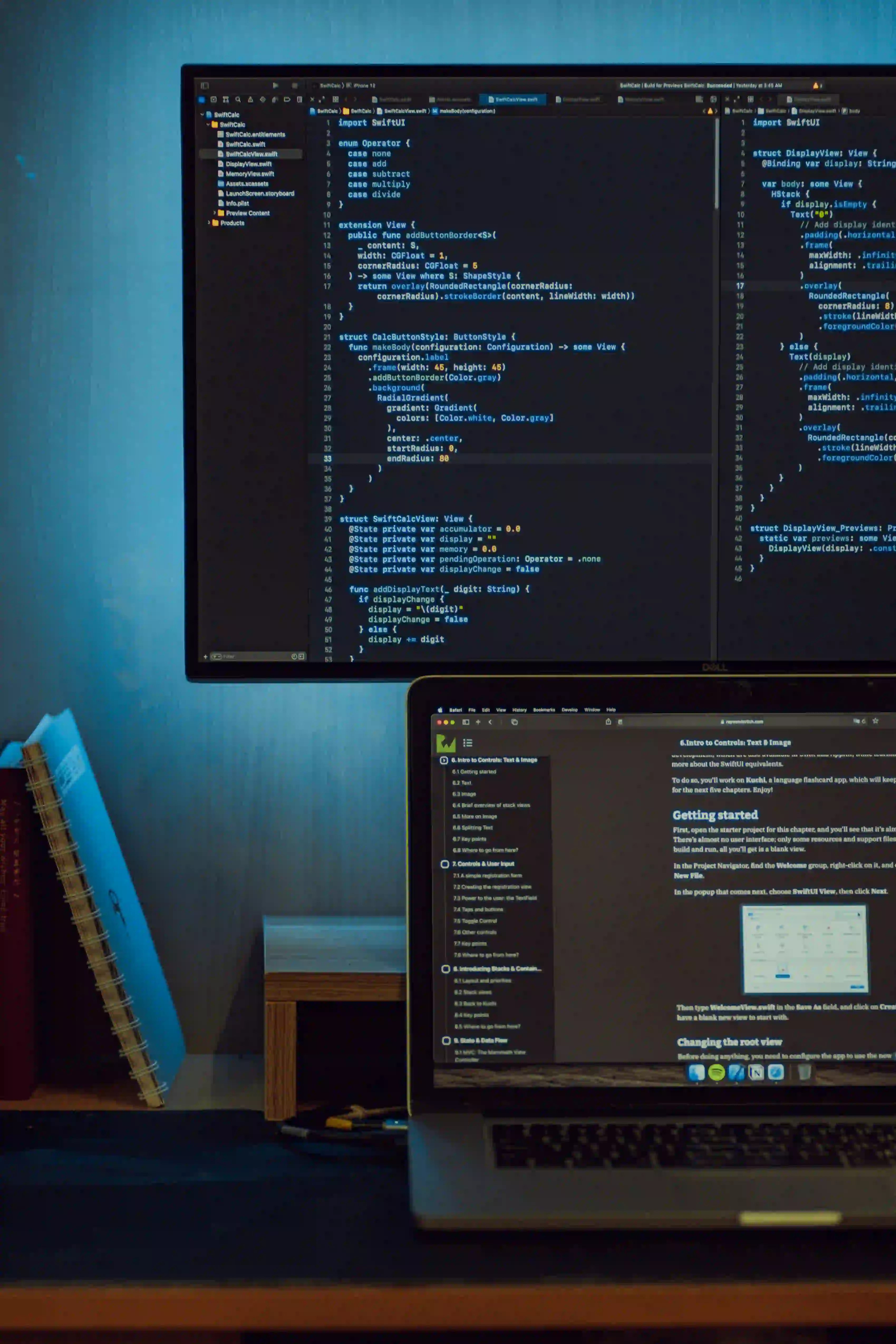
Common Pitfalls in Designing Domain Layers of Multilayered Architectures
Designing a multilayered architecture involves a careful balancing act, especially within the domain layer. This layer serves as the heart of your application, where business logic, validation, and rules reside. Ensuring that this layer is robust and well-structured is paramount for the success of any software project. In this article, we will discuss common pitfalls while designing the domain layer of multilayered architectures, offering solutions and code snippets to highlight best practices in Java.
Understanding the Architecture Layers
Before diving into pitfalls, let's clarify the typical layers present in a multilayered architecture:
- Presentation Layer: Responsible for handling user interactions. It communicates with the domain layer to retrieve and present data.
- Domain Layer: Encapsulates business logic. It's the core of your application where you define entities, value objects, and business rules.
- Data Access Layer: Manages how data is persistently stored and retrieved. This layer interacts with databases and other data sources.
Pitfall 1: Overcomplicating the Domain Model
When developers attempt to create a domain model that represents every conceivable rule, they often end up with an overly complex design.
Solution: Keep It Simple
Aim to create a domain model that reflects the essential business rules without adding unnecessary complexity. Review business requirements regularly and focus on encapsulating only the most critical rules in your domain layer.
public class User {
private String username;
private String password;
public User(String username, String password) {
validateUsername(username);
validatePassword(password);
this.username = username;
this.password = password;
}
private void validateUsername(String username) {
if (username == null || username.isEmpty()) {
throw new IllegalArgumentException("Username cannot be empty.");
}
}
private void validatePassword(String password) {
if (password.length() < 6) {
throw new IllegalArgumentException("Password must be at least 6 characters long.");
}
}
// Getters and additional methods
}
In this example, the User
class encapsulates only the essential behaviors necessary for maintaining user integrity without being overloaded by extraneous logic.
Pitfall 2: Ignoring the Separation of Concerns
Developers sometimes conflate different concerns within the domain layer, which can lead to difficulty in managing code and dependencies.
Solution: Use Value Objects and Entities Effectively
By clearly distinguishing between entities and value objects, you can maintain clean boundaries. Entities represent objects with identity (like a User), while value objects are immutable and defined by their attributes (like an Address).
public class Address {
private final String street;
private final String city;
private final String zipCode;
public Address(String street, String city, String zipCode) {
this.street = street;
this.city = city;
this.zipCode = zipCode;
}
// Getters for fields
}
This Address
class prevents changes once it's created, thereby ensuring that the integrity of the object's state is maintained.
Pitfall 3: Insufficient Validation of Business Rules
Failing to enforce business rules can lead to inconsistent states and unexpected behaviors. Often, developers leave important checks to the presentation layer, which is not ideal.
Solution: Enforce Business Rules in the Domain Layer
Always validate your business rules within your domain model. This ensures that no incorrect data can propagate through your application.
public class Order {
private List<Product> products;
public Order(List<Product> products) {
validateProducts(products);
this.products = new ArrayList<>(products);
}
private void validateProducts(List<Product> products) {
if (products == null || products.isEmpty()) {
throw new IllegalArgumentException("Order must contain at least one product.");
}
}
// Additional methods
}
By placing validation logic directly in the domain layer, you safeguard against invalid state, making your application more resilient.
Pitfall 4: Tight Coupling Between Layers
Coupled layers reduce flexibility and make your application harder to maintain and test. Coupling may occur when entities from the domain layer directly depend on infrastructure concerns like the database.
Solution: Use Interfaces and Dependency Injection
Introducing interfaces and dependency injection decouples your boundaries. This ensures that the domain layer remains independent of data access details, promoting flexibility.
public interface UserRepository {
User findByUsername(String username);
void save(User user);
}
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Methods using userRepository
}
In this architecture, UserService
interacts with an abstract UserRepository
. The actual implementation can be swapped in and out without changing the service layer.
Pitfall 5: Neglecting to Write Tests
Insufficient testing can lead to fragile applications. Developers often prioritize feature implementation over establishing a suite of tests for the business logic.
Solution: Implement Comprehensive Unit Tests
Commit to writing unit tests covering various scenarios in your domain layer. This practice not only safeguards against regression but also ensures that the business rules are correctly implemented.
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class UserTest {
@Test
public void testUserCreationWithEmptyUsername() {
Exception exception = assertThrows(IllegalArgumentException.class, () -> {
new User("", "password123");
});
assertEquals("Username cannot be empty.", exception.getMessage());
}
// Additional test cases
}
Unit tests serve as both documentation and a safety net for future modifications. They instill confidence when modifying the domain layer code.
The Closing Argument
Designing the domain layer within a multilayered architecture comes with its challenges. By avoiding these common pitfalls and implementing best practices, you can create a robust and effective domain layer.
- Keep your domain model simple and focused.
- Adhere to separation of concerns with clear data structures.
- Validate business rules within the domain layer itself.
- Decouple layers using interfaces and dependency injection.
- Prioritize writing comprehensive unit tests.
As you refine your domain layer, consider exploring Martin Fowler's Domain-Driven Design for additional insights. By understanding these concepts, you can elevate your application's design and ensure a strong foundation for future growth and complexity. Happy coding!