Common WSO2 Identity Server Authentication Issues Explained
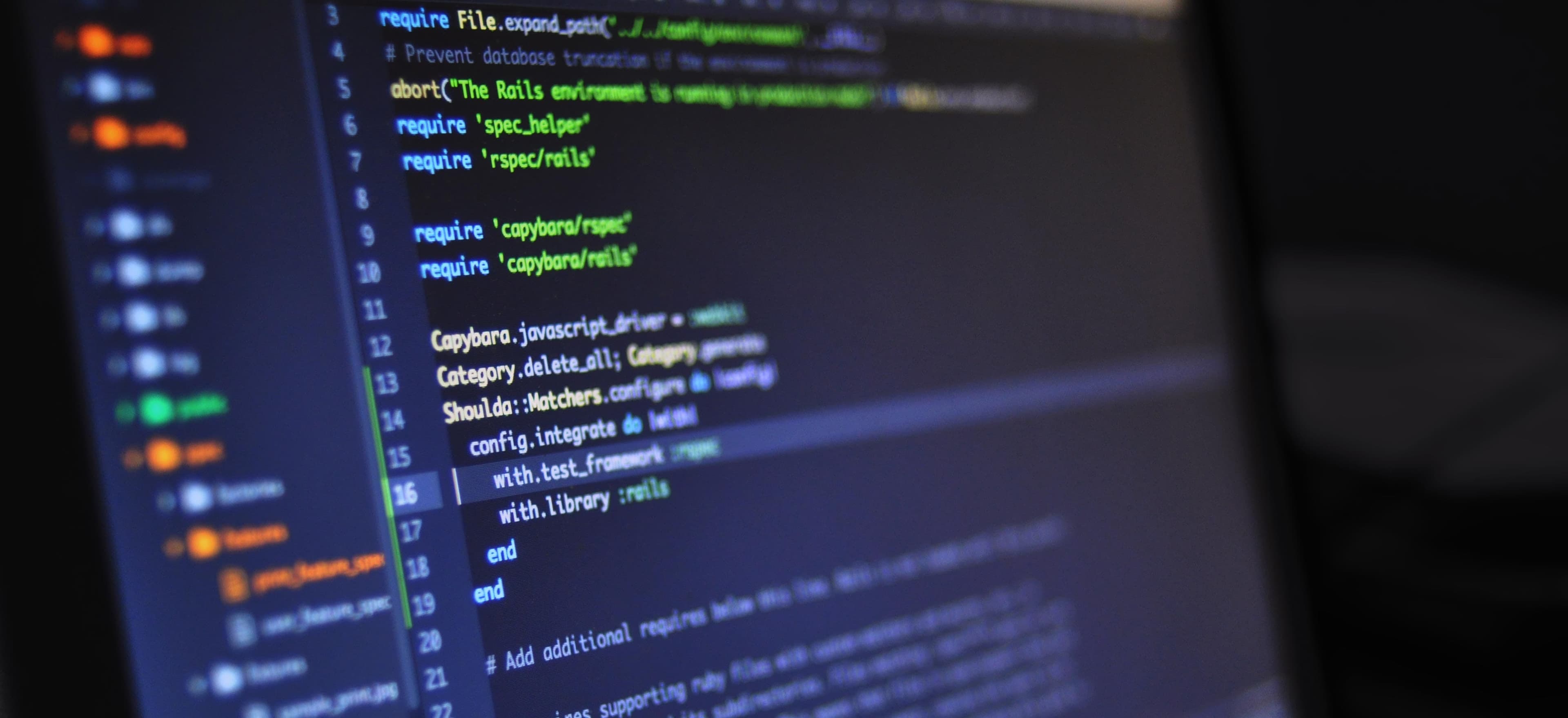
- Published on
Common WSO2 Identity Server Authentication Issues Explained
In today's digital landscape, secure identity management is paramount. WSO2 Identity Server (WSO2 IS) is a powerful open-source platform that enables organizations to manage user identities and authentication effectively. However, like any sophisticated technology, it comes with its own set of challenges. This blog post aims to elucidate common authentication issues encountered while using WSO2 Identity Server and provide solutions to these problems.
Understanding WSO2 Identity Server
Before diving into the issues, it's essential to understand what WSO2 Identity Server does. WSO2 IS is designed to create a single sign-on (SSO) system, handle identity federation, and manage user roles and permissions. It's equipped to handle various authentication protocols like OAuth2, OpenID Connect, and SAML.
Common Authentication Issues
1. Incorrectly Configured Identity Providers
One of the most frequent issues with WSO2 Identity Server is the misconfiguration of identity providers (IdPs). This often leads to failed login attempts and a poor user experience.
Solution
Ensure that the IdP configuration is accurate. Check the following:
- Client ID and Secret: Match the values set in WSO2 IS and your application.
- Redirection URI: Ensure that this URI is consistent across the IdP and your application.
Here’s a sample configuration for connecting an external IdP using OAuth2:
<identityProvider>
<identityProviderId>exampleIdP</identityProviderId>
<name>Example IdP</name>
<protocol>oauth2</protocol>
<customAuthenticator>oauth2Authenticator</customAuthenticator>
<oauth2Config>
<clientId>1234567890</clientId>
<clientSecret>secretKey</clientSecret>
<callbackUrl>https://yourapp.com/callback</callbackUrl>
<authorizationEndpoint>https://idp.com/auth</authorizationEndpoint>
</oauth2Config>
</identityProvider>
Why this matters: This configuration allows your WSO2 IS to interact correctly with the IdP.
References:
2. Token Expiration Issues
Token expiration can create significant disruptions in user authentication. By default, access tokens in WSO2 IS can expire quickly.
Solution
Manage your token lifetimes effectively according to your application needs. Consider using refresh tokens to maintain user sessions without requiring re-authentication.
Here's an example of how you can adjust the token expiration settings:
<OAuth>
<AccessTokenExpiryTime>3600</AccessTokenExpiryTime> <!-- 1 hour -->
<RefreshTokenExpiryTime>7200</RefreshTokenExpiryTime> <!-- 2 hours -->
</OAuth>
Why this matters: Proper token management keeps users logged in and reduces the frequency of authentication prompts.
3. Failed User Login Attempts
Many users experience login failures due to incorrect credentials or configuration mismatches between your application and WSO2 IS.
Solution
Implement logging to capture failed login attempts. This will allow you to diagnose reasons for user login failures. Additionally, consider implementing a user-friendly error message that can help users rectify their input.
public void authenticateUser(String username, String password) {
try {
// Authenticating user against WSO2 Identity Server
AuthenticationResult result = identityProvider.authenticate(username, password);
if (!result.isAuthenticated()) {
logFailedLoginAttempt(username);
throw new AuthenticationFailedException("Invalid credentials. Please try again.");
}
} catch (Exception e) {
// Handle the exception
e.printStackTrace();
}
}
Why this matters: This provides clear feedback to the user and keeps track of potential system abuses.
4. CORS Issues with API Authentication
Cross-Origin Resource Sharing (CORS) issues can arise when trying to authenticate through APIs hosted on different domains.
Solution
Enable CORS in your WSO2 IS configuration. You can control CORS settings in the deployment.toml
file.
[CORS]
enabled = true
allow_origins = ["https://yourapp.com"]
allow_headers = ["Authorization", "Content-Type"]
allow_methods = ["GET", "POST", "OPTIONS"]
Why this matters: Proper CORS settings ensure that your API requests are not blocked, allowing seamless interaction between services.
5. Missing User Roles and Permissions
Sometimes, users may log in successfully but have restricted access due to missing roles or permissions, impacting their experience.
Solution
Revisit your role and permission configurations within WSO2 IS. Ensure that users are assigned the correct roles upon creation or via an automated process such as group membership.
Here’s a Java snippet to check and assign roles:
public void assignUserToRole(String username, String roleName) {
try {
if (!userHasRole(username, roleName)) {
getUserStoreManager().addRole(roleName, new String[]{username}, null);
}
} catch (UserStoreException e) {
e.printStackTrace();
}
}
Why this matters: Assigning correct roles ensures users have the necessary access rights to your applications, maintaining an optimal user experience.
In Conclusion, Here is What Matters
WSO2 Identity Server is a robust solution for managing user authentication and identity. However, as with any powerful tool, common issues can arise if configurations are not handled properly. By understanding these challenges and their solutions such as IdP configurations, token management, CORS settings, and role assignments, you can create a more seamless and secure authentication experience for your users.
For more information on common issues in WSO2 Identity Server, you can also refer to the WSO2 Identity Server Community.
By addressing these common authentication issues in WSO2 Identity Server, organizations can enhance user satisfaction and ensure a more secure digital environment. If you've faced challenges not covered here, feel free to comment below or share your experience!