Unlocking Java Concurrency: Common Pitfalls to Avoid
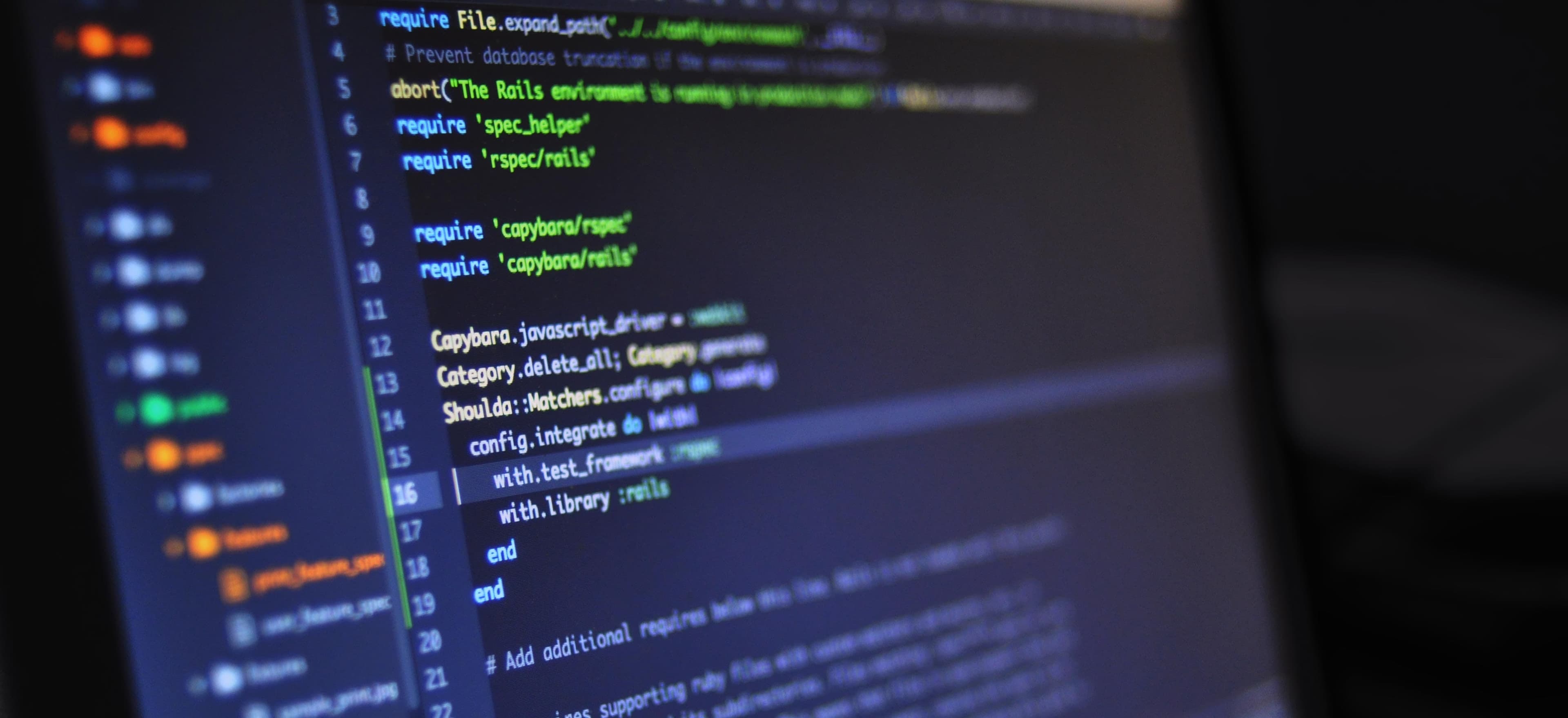
- Published on
Unlocking Java Concurrency: Common Pitfalls to Avoid
Concurrency in Java can unleash the power of multi-threading and improve application performance dramatically. However, diving into the world of Java concurrency without understanding its nuances can lead to disastrous results. In this blog post, we will explore common pitfalls in Java's concurrency model and provide best practices to help you write safe, efficient, and clean multi-threaded applications.
The Basics of Java Concurrency
Java offers robust support for concurrent programming, primarily through the java.lang.Thread
class and the java.util.concurrent
package. Threads allow multiple paths of execution within a program, enabling tasks to run concurrently, which can significantly speed up processing times.
However, concurrent programming can be tricky. Issues like race conditions, deadlocks, and thread starvation can make your application unpredictable and difficult to debug.
Pitfall #1: Race Conditions
What is a Race Condition?
A race condition occurs when two or more threads access shared resources simultaneously, and the final result depends on the sequence or timing of the threads' execution. This can lead to inconsistent or corrupt data.
Example Code
public class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
In the above snippet, the increment
method is not thread-safe. If multiple threads call increment
simultaneously, the count
variable may not increment correctly.
Solution: Synchronization
To avoid race conditions, you can use the synchronized
keyword to make methods or blocks of code thread-safe.
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
Using synchronized
ensures that only one thread can access the increment
method at any given time, thus preventing race conditions. However, do note that overusing synchronization can lead to performance bottlenecks.
When to Use Synchronization
- When accessing shared mutable state, it's crucial to synchronize the access to prevent data inconsistency.
- Use tools like Java Concurrency in Practice to understand best practices.
Pitfall #2: Deadlocks
What is a Deadlock?
A deadlock occurs when two or more threads are blocked forever, each waiting for a resource that the other holds. This can cripple an application, leading to frustrating user experiences.
Example of a Deadlock
public class DeadlockExample {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void method1() {
synchronized (lock1) {
synchronized (lock2) {
// critical section
}
}
}
public void method2() {
synchronized (lock2) {
synchronized (lock1) {
// critical section
}
}
}
}
In this example, if method1
is invoked by thread A and method2
is invoked by thread B, they can end up waiting indefinitely for each other to release their locks.
Solution: Lock Ordering
To prevent deadlocks, establish a global order for acquiring locks.
public class DeadlockAvoidanceExample {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void method1() {
lock(lock1, lock2);
}
public void method2() {
lock(lock1, lock2);
}
private void lock(Object firstLock, Object secondLock) {
synchronized (firstLock) {
synchronized (secondLock) {
// critical section
}
}
}
}
Best Practices
- Always acquire locks in a consistent order across your application.
- Keep the lock scope small, focusing only on the state you need to protect.
For more on this topic, consider reading Effective Java by Joshua Bloch.
Pitfall #3: Thread Starvation
What is Thread Starvation?
Thread starvation occurs when a thread is perpetually denied access to resources it needs for execution because other threads are consuming those resources.
Example Leading to Starvation
public class StarvationExample {
public void run() {
synchronized (this) {
// Long running task
}
}
}
If run()
is held for a long time by one thread, other threads requesting access to the synchronized block could be starved.
Solution: Use Fair Locks
Using fair locks can help mitigate starvation. The ReentrantLock
class allows you to create a lock that grants access to the longest waiting thread.
import java.util.concurrent.locks.ReentrantLock;
public class FairLockExample {
private final ReentrantLock lock = new ReentrantLock(true); // set fair to true
public void run() {
lock.lock();
try {
// critical section
} finally {
lock.unlock();
}
}
}
Summary of Best Practices
- Use locks judiciously and prefer higher-level abstractions such as
ExecutorService
over manual thread management. - Always assess the potential for starvation in your application architecture.
Pitfall #4: Not Using the Right Tools
Why Tools Matter
Java provides a suite of tools in the java.util.concurrent
package that can help manage concurrency without the overhead of manual thread management.
Tools like ExecutorService
, CountDownLatch
, Semaphore
, and more provide a framework for handling threading issues more effectively.
Example of ExecutorService
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ExecutorServiceExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 100; i++) {
executor.submit(() -> {
// task execution logic
});
}
executor.shutdown();
}
}
Benefits of Using Concurrent Tools
- Improved readability and maintainability.
- Enhanced scalability and performance.
- Built-in mechanisms that handle many common threading problems.
Wrapping Up
Concurrency in Java opens up numerous opportunities for optimizing application performance. By avoiding common pitfalls like race conditions, deadlocks, thread starvation, and neglecting tool utilization, developers can create robust and efficient multi-threaded applications.
Further Learning Resources
- Explore the Java Concurrency API.
- Read Java Concurrency in Practice for deeper insights.
By understanding these pitfalls and applying the solutions and best practices mentioned, you can unlock the full potential of Java concurrency and create high-performance, reliable applications. Happy coding!
Checkout our other articles