Overcoming SWT's Challenges for Truly Responsive UIs
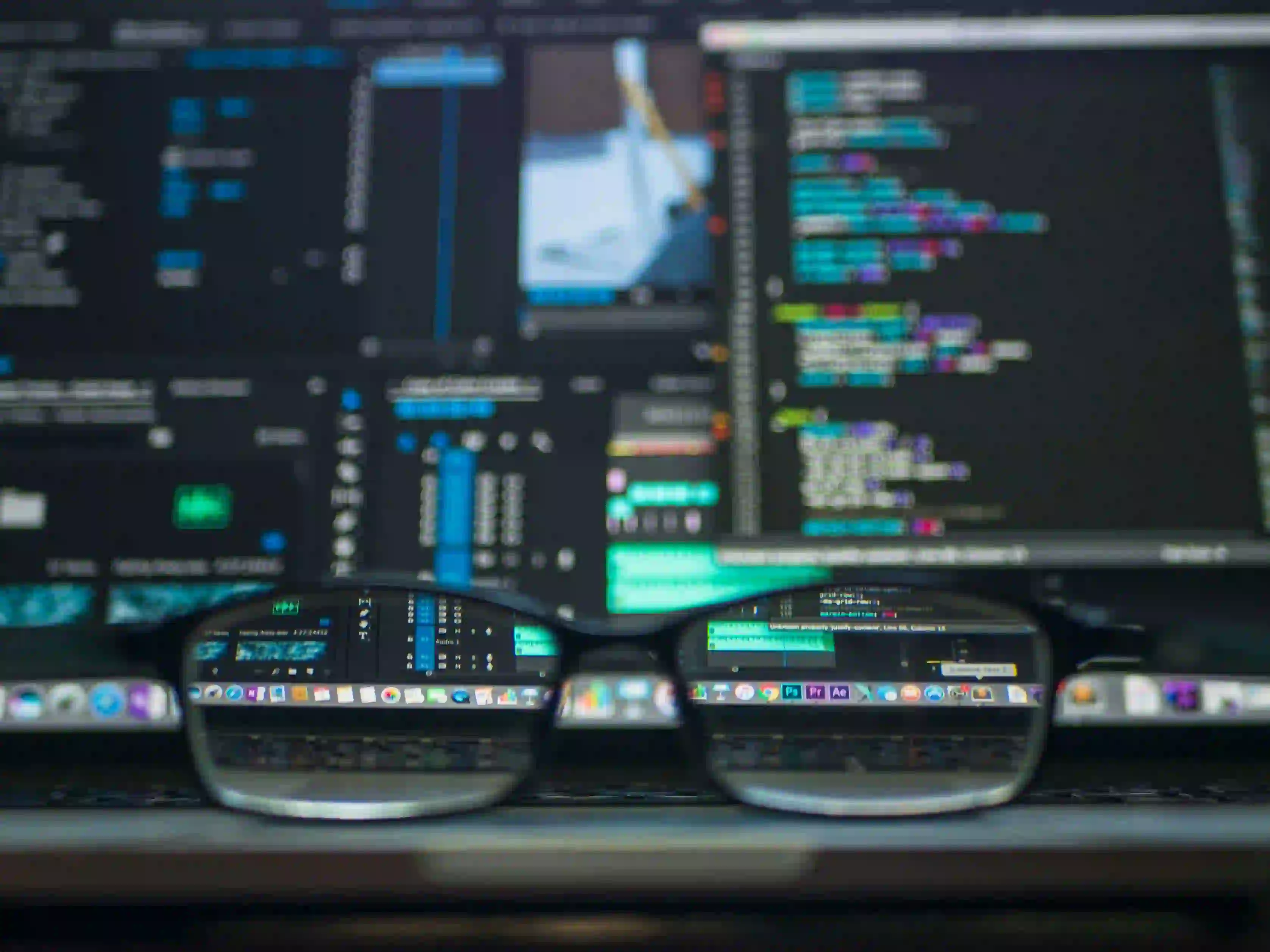
Overcoming SWT's Challenges for Truly Responsive UIs
Creating responsive user interfaces (UIs) is a critical aspect of modern software development. Java's Standard Widget Toolkit (SWT) is a popular choice for building rich applications because it provides native look and feel across different platforms. However, developers often encounter challenges that can hinder the development of truly responsive UIs. This post will explore common SWT challenges and provide solutions that can help you deliver a smooth and responsive user experience.
Understanding SWT
SWT is a graphical widget toolkit for use with Java. It provides a set of components that are used to create user interfaces, allowing developers to harness native functionalities with ease. One of the reasons SWT has a strong following is its ability to integrate with native windowing systems, leading to a look and feel that is consistent with the target platform.
However, despite its advantages, SWT presents unique challenges, especially when it comes to responsiveness. This article will discuss these hurdles and equip you with techniques to overcome them.
Common Challenges in SWT
1. Event Dispatch Thread (EDT) Management
In SWT, all UI updates must occur on the UI thread, also known as the Event Dispatch Thread (EDT). Performing long-running operations on the EDT can freeze the UI, making it unresponsive.
Solution: Use Display.asyncExec()
or Display.syncExec()
to handle UI updates effectively. Here is how:
Display.getDefault().asyncExec(new Runnable() {
public void run() {
// Code to update UI components goes here
myButton.setEnabled(true);
myLabel.setText("Task Completed");
}
});
Why: This code snippet places UI updates on the EDT, ensuring that the UI remains responsive while long-running tasks are executed in the background.
2. Handling Long-running Tasks
One of the most common pitfalls in SWT applications is running time-consuming tasks directly on the EDT.
Solution: Use a background thread to handle these tasks. Here’s an example using Thread
:
new Thread(() -> {
// Simulating a long-running operation
try {
Thread.sleep(5000); // Simulate work
} catch (InterruptedException e) {
e.printStackTrace();
}
// Update UI from the EDT
Display.getDefault().asyncExec(() -> {
myButton.setEnabled(true);
myLabel.setText("Task Completed");
});
}).start();
Why: By placing the long-running operation in a separate thread, we allow the EDT to remain free for user interactions. This greatly increases the responsiveness of your application.
3. Optimizing Layouts and Painting
SWT's native controls can sometimes be a challenge when it comes to custom layout and painting, which can lead to responsiveness issues.
Solution: Use layout managers like GridLayout
or FillLayout
wisely. Here’s an example of using GridLayout
:
Composite composite = new Composite(shell, SWT.NONE);
GridLayout layout = new GridLayout(2, false);
composite.setLayout(layout);
Label label = new Label(composite, SWT.NONE);
label.setText("Enter a Value:");
Text text = new Text(composite, SWT.BORDER);
GridData data = new GridData(SWT.FILL, SWT.CENTER, true, false);
text.setLayoutData(data);
Why: Using a layout manager ensures that your UI elements are placed efficiently and correctly. Well-structured UIs are easier to manage and respond better to resizing and user inputs.
4. Resource Management
Improper management of resources can lead to memory leaks and affect application performance. SWT often requires explicit control over resource allocation and disposal.
Solution: Always dispose of resources when they are no longer needed. For example:
Image image = new Image(display, "path/to/image.png");
// Use the image
image.dispose(); // When done, dispose of the image
Why: By disposing of resources, you free up memory, which can lead to a more responsive application, especially for long-running applications or those that frequently utilize graphical assets.
Best Practices for Developing Responsive UIs in SWT
-
Use Worker Threads: Always offload heavy computations to worker threads and use
asyncExec()
for updating UI components. -
Minimize UI Updates: Instead of refreshing the UI on every data input, batch updates to reduce the frequency of UI redraws.
-
Debounce User Input: Implement a debounce mechanism for user input fields to avoid excessive binding or loading calls.
-
Profile Performance: Use tools such as VisualVM or JProfiler to monitor the performance of your SWT application, identifying bottlenecks.
-
Keep It Simple: Sometimes, simplicity can be the best approach. Avoid complex UI elements that could lead to performance issues unless necessary.
Example Application
To illustrate the discussed concepts, let's create a simple SWT application that performs a long-running task and updates the UI.
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Label;
public class ResponsiveSWTApp {
private static boolean isRunning = false;
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setSize(300, 200);
shell.setText("Responsive SWT App");
Label statusLabel = new Label(shell, SWT.NONE);
statusLabel.setBounds(10, 10, 280, 25);
statusLabel.setText("Idle");
Button startButton = new Button(shell, SWT.PUSH);
startButton.setBounds(10, 50, 80, 25);
startButton.setText("Start Task");
startButton.addListener(SWT.Selection, e -> {
if (!isRunning) {
isRunning = true;
statusLabel.setText("Running...");
startLongRunningTask(statusLabel);
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
private static void startLongRunningTask(Label statusLabel) {
new Thread(() -> {
try {
Thread.sleep(5000); // Simulate a long task
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
Display.getDefault().asyncExec(() -> {
statusLabel.setText("Task Completed");
isRunning = false;
});
}
}).start();
}
}
The Closing Argument
Developing responsive UIs using the SWT framework does come with challenges, but they are manageable with the right techniques and best practices. By effectively managing the EDT, employing background threads for long-running tasks, optimizing layouts, and practicing resource management, developers can create applications that provide a smooth user experience.
For more information on SWT, you can refer to the official documentation here.
Arming yourself with these strategies will empower you to tackle SWT challenges and build applications that not only meet user expectations but exceed them. Happy coding!