Mastering Rest Assured: Simplifying API Testing in Java
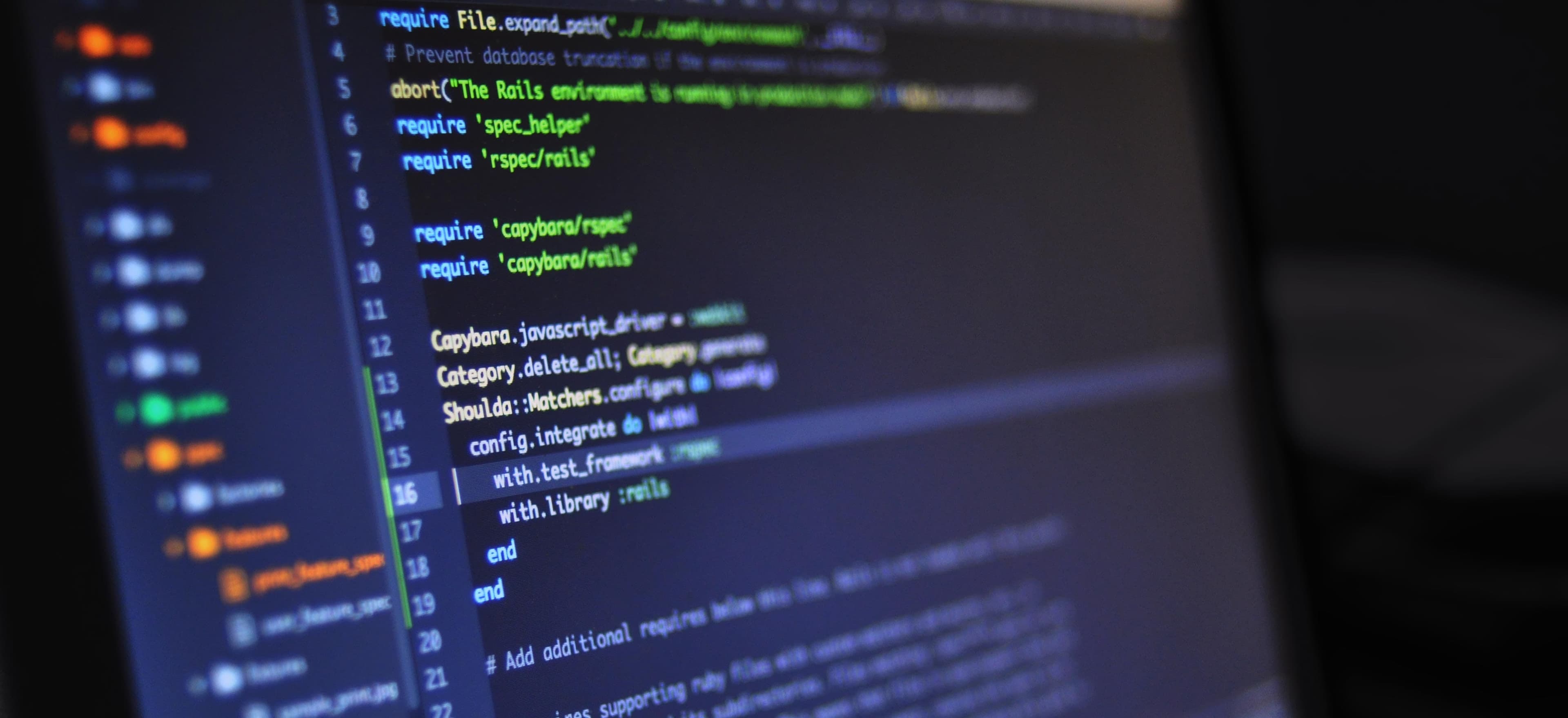
- Published on
Mastering Rest Assured: Simplifying API Testing in Java
API testing is a crucial part of modern software development. With the rise of microservices and application programming interfaces (APIs), ensuring that these components function correctly has become ever more important. This is where Rest Assured comes in—a Java library that simplifies the task of testing REST APIs. In this post, we will explore the ins and outs of Rest Assured, how to get started, and best practices to ensure you're testing efficiently and effectively.
Setting the Stage to Rest Assured
Rest Assured is an open-source Java library specifically focused on testing RESTful APIs. Its fluent interface allows developers to write readable tests quickly, making it easier to validate the behavior and output of APIs without excessive boilerplate code.
With Rest Assured, you can:
- Send HTTP requests
- Parse responses
- Validate status codes and response bodies
- Authenticate and manage headers easily
Why Choose Rest Assured?
- Simplicity: The concise syntax makes it easy to read and write tests.
- Integration: It integrates seamlessly with popular testing frameworks like JUnit and TestNG.
- Rich Features: Offers powerful built-in assertions, supports JSON and XML responses, and can handle complex authentication schemes.
If you want to dive deeper into the advantages of using Rest Assured over other REST testing libraries, you can check this comparison.
Getting Started with Rest Assured
To start using Rest Assured in your Java project, you will need to include the Rest Assured dependency in your pom.xml
if you're using Maven:
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>5.1.1</version>
<scope>test</scope>
</dependency>
If you're using Gradle, add the following line to your build.gradle
file:
testImplementation 'io.rest-assured:rest-assured:5.1.1'
Once that is set up, you are ready to start testing APIs. Let’s consider an example of a simple API that provides user data.
Example API
Consider the following simple REST API that fetches user information:
- Endpoint:
https://api.example.com/users
- Method: GET
Writing Your First Test
Here's how you can write a simple test case with Rest Assured to validate the response from the above API.
import io.restassured.RestAssured;
import io.restassured.response.Response;
import org.junit.jupiter.api.Test;
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
public class UserApiTest {
@Test
public void testGetUser() {
RestAssured.baseURI = "https://api.example.com";
Response response = given()
.header("Content-Type", "application/json")
.when()
.get("/users/1")
.then()
.statusCode(200)
.body("name", equalTo("John Doe"))
.body("age", greaterThan(20))
.extract().response();
System.out.println("Response: " + response.asString());
}
}
Commentary
-
RestAssured.baseURI
: This sets the base URI for subsequent calls. It helps maintain clarity in your tests by not repeating the base URL. -
given()
: Here, you can define your request specifications, such as headers, query parameters, etc. -
when().get("/users/1")
: This line actually makes the GET request to the specified endpoint. -
.then().statusCode(200)
: This validates that the response status code is 200, indicating success. -
.body(...)
: Here we validate specific fields in the response body using matchers. It's concise and expressive. -
Response Extraction: Using
.extract().response()
allows you to save the response object for further exploration or assertions if necessary.
Running Your Test
To run your tests, you can use your IDE's built-in capabilities or command line tools suited to your project (Maven, Gradle). The assertions will let you know if anything deviates from your expectations.
Advanced Features of Rest Assured
Authentication
Many APIs require authentication. Rest Assured provides methods to easily include various authentication forms, such as Basic Auth, OAuth, and more.
Here's how to include Basic Auth:
given()
.auth().preemptive().basic("username", "password")
.when()
.get("/secure-data")
.then()
.statusCode(200);
Working with JSON and XML
Rest Assured excels at parsing and validating JSON and XML responses. You can effortlessly switch between formats. Here’s how you can validate a JSON response:
.when()
.get("/users")
.then()
.body("users.size()", is(5))
.body("users[0].name", equalTo("John Doe"));
Validating Headers
Sometimes, it is crucial to validate headers in the response.
.then()
.header("Content-Type", "application/json; charset=utf-8");
Logging and Debugging
To ease the debugging process, Rest Assured provides logging capabilities.
.log().all()
Chaining Requests
Another powerful aspect is chaining methods for a cleaner and more efficient testing experience.
The following code snippet demonstrates chaining:
given()
.auth().oauth2("your_oauth_token")
.when()
.post("/users")
.then()
.statusCode(201)
.log().all();
Integrating with CI/CD Pipelines
Integrating your Rest Assured tests into Continuous Integration and Continuous Deployment (CI/CD) pipelines can help in automating the testing process. Tools like Jenkins, Travis CI, or GitHub Actions can trigger these tests, ensuring your API continues to function as intended after every commit.
A Final Look
Mastering Rest Assured can significantly simplify your API testing in Java. Its readability, flexibility, and robust features allow you to write powerful tests that align with modern development practices. Whether you're validating responses, parsing data, or ensuring proper authentication, Rest Assured offers a straightforward approach.
To further your understanding, explore the official documentation and practice writing tests for different RESTful services.
By adopting Rest Assured, you are not only ensuring your APIs function as expected but also embracing best practices in software testing that will benefit your entire development lifecycle.
Happy testing!
Checkout our other articles