Common Pitfalls in Adding Database Logging to JUnit 3
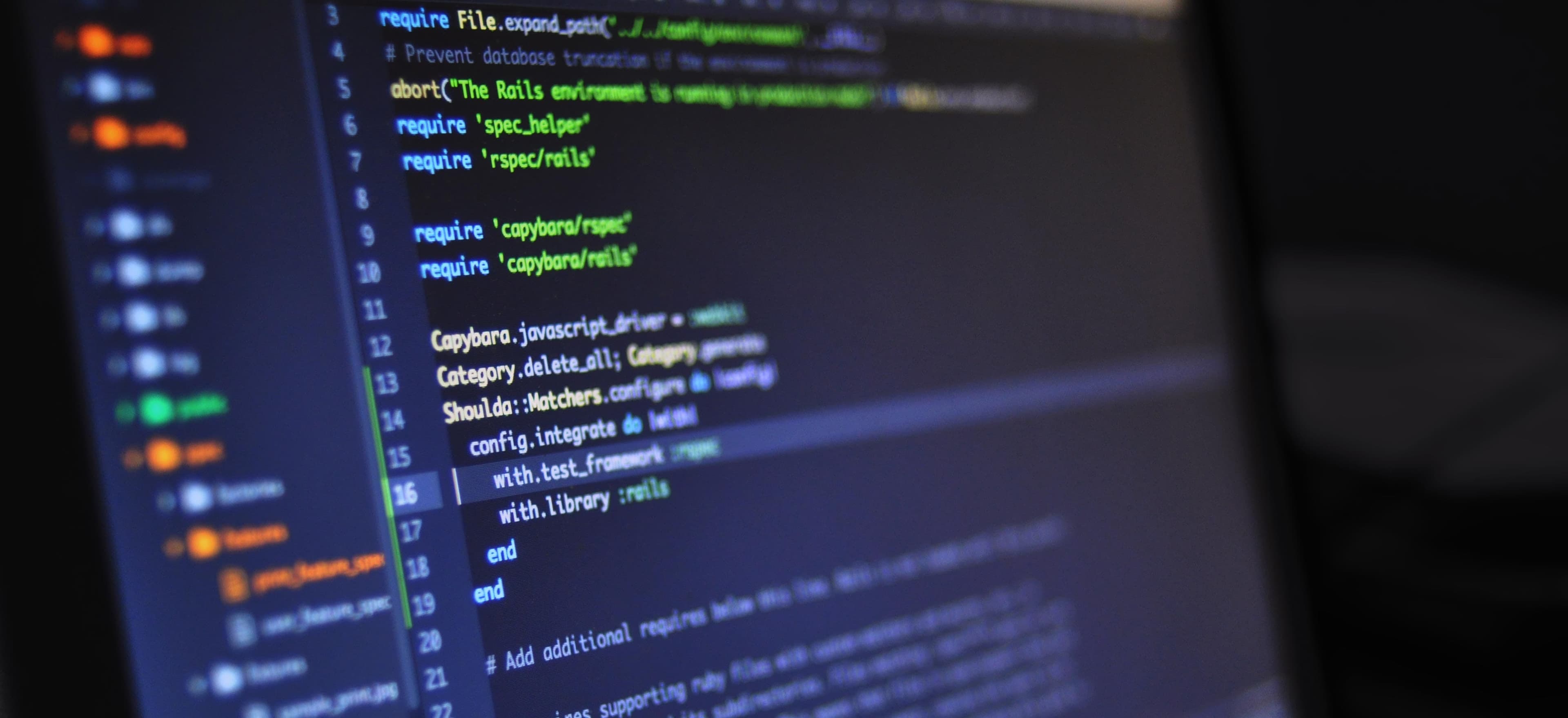
- Published on
Common Pitfalls in Adding Database Logging to JUnit 3
In software development, logging is vital for understanding application behavior, especially during testing. When using JUnit 3, integrating database logging can present unique challenges. This post deep dives into common pitfalls developers face when adding database logging to JUnit 3, providing code snippets to elucidate best practices.
Why Use Database Logging?
Database logging allows developers to persist logs, facilitating a detailed audit and easier bug tracking. Unlike traditional log files, database logs provide structured access to log data, enabling complex queries and reporting.
Pitfall 1: Not Configuring the Database Connection Properly
One of the most frequent errors is improper database connection configuration. This generally leads to connection timeouts or failed log insertions.
Example Code:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
private static final String DB_URL = "jdbc:mysql://localhost:3306/testdb";
private static final String USER = "root";
private static final String PASS = "password";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(DB_URL, USER, PASS);
}
}
Why This Matters: The connection string must be correct, and the database must be accessible. If these parameters are incorrect, it results in SQL exceptions that could get tricky to debug. Always keep your connection credentials secure and leverage environment variables for sensitive data.
Pitfall 2: Forgetting to Use Transactions
When logging to the database, it’s crucial to ensure that log entries are atomic. Failure to handle transactions properly can leave the database in an inconsistent state.
Example Code:
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class LogToDatabase {
public void logEvent(String eventMessage) {
String insertSQL = "INSERT INTO logs(event_message) VALUES (?)";
try (Connection connection = DatabaseConnection.getConnection()) {
connection.setAutoCommit(false);
PreparedStatement preparedStatement = connection.prepareStatement(insertSQL);
preparedStatement.setString(1, eventMessage);
preparedStatement.executeUpdate();
connection.commit(); // Ensure that the log is committed to the database
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Why This Matters: Proper transaction management ensures either all log entries are written or none are, protecting data integrity. It prevents losing logging data in case of an error.
Pitfall 3: Not Cleaning up Resources
Another common mistake is neglecting to close database resources. Failing to close connections or statements can lead to resource leaks, exhausting database connections over time.
Example Code:
public void logEvent(String eventMessage) {
String insertSQL = "INSERT INTO logs(event_message) VALUES (?)";
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = DatabaseConnection.getConnection();
preparedStatement = connection.prepareStatement(insertSQL);
preparedStatement.setString(1, eventMessage);
preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
Why This Matters: Using finally
blocks to ensure resources are closed is crucial for performance. Java’s automatic garbage collection doesn’t suffice for managing database connections.
Pitfall 4: Poorly Designed Database Schema
Another hurdle lies in the database schema design. A lack of indexing or incorrect data types can hinder performance and complexity when querying logs.
Example Schema:
CREATE TABLE logs (
id INT AUTO_INCREMENT PRIMARY KEY,
event_message TEXT NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Why This Matters: Efficient schema design can drastically reduce query times when analyzing logs. Make sure to consider the types of queries you’ll need when designing schema for logging.
Pitfall 5: Not Capturing Enough Contextual Information
When logging events, it’s easy to overlook the importance of detailed contextual information. As a result, logs may lack essential details for troubleshooting.
Effective Logging Example:
public void logEvent(String eventMessage, String username, int userId) {
String insertSQL = "INSERT INTO logs(event_message, username, user_id) VALUES (?, ?, ?)";
// Similar connection handling as shown above
try (Connection connection = DatabaseConnection.getConnection();
PreparedStatement preparedStatement = connection.prepareStatement(insertSQL)) {
preparedStatement.setString(1, eventMessage);
preparedStatement.setString(2, username);
preparedStatement.setInt(3, userId);
preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
Why This Matters: Logging more contextual data helps in post-mortem analyses. Including user identifiers, timestamps, and stack traces will empower you to troubleshoot and optimize the system.
Pitfall 6: Ignoring Asynchronous Logging
In high-traffic applications, synchronous logging can become a bottleneck. This leads to performance degradation; hence, it is essential to implement asynchronous logging.
Example Using Executors:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class AsyncLogger {
private ExecutorService executor = Executors.newSingleThreadExecutor();
public void logEvent(String eventMessage) {
executor.submit(() -> {
// Call the synchronous log method here
new LogToDatabase().logEvent(eventMessage);
});
}
public void shutdown() {
executor.shutdown();
}
}
Why This Matters: Asynchronous logging improves performance by decoupling logging from the main application flow. However, ensure that this does not lead to lost logs during unexpected crashes.
Bringing It All Together
Integrating database logging into JUnit 3 requires careful consideration of various factors ranging from connection handling to schema design. By avoiding common pitfalls, you can create a robust logging system that enhances your application's maintainability and audit capabilities.
For more details on JUnit 3, visit the official JUnit documentation. Also consider reading up on best practices for logging, which can provide additional insights into effective logging strategies.
As always, testing is crucial. Make sure to write tests that cover both the success scenarios and edge cases for your logging logic.
Incorporating these best practices will not only make your logging more effective but also pave the way for smoother debugging and enhanced system performance. Happy coding!