Common Redis Configuration Issues in Spring Boot Explained
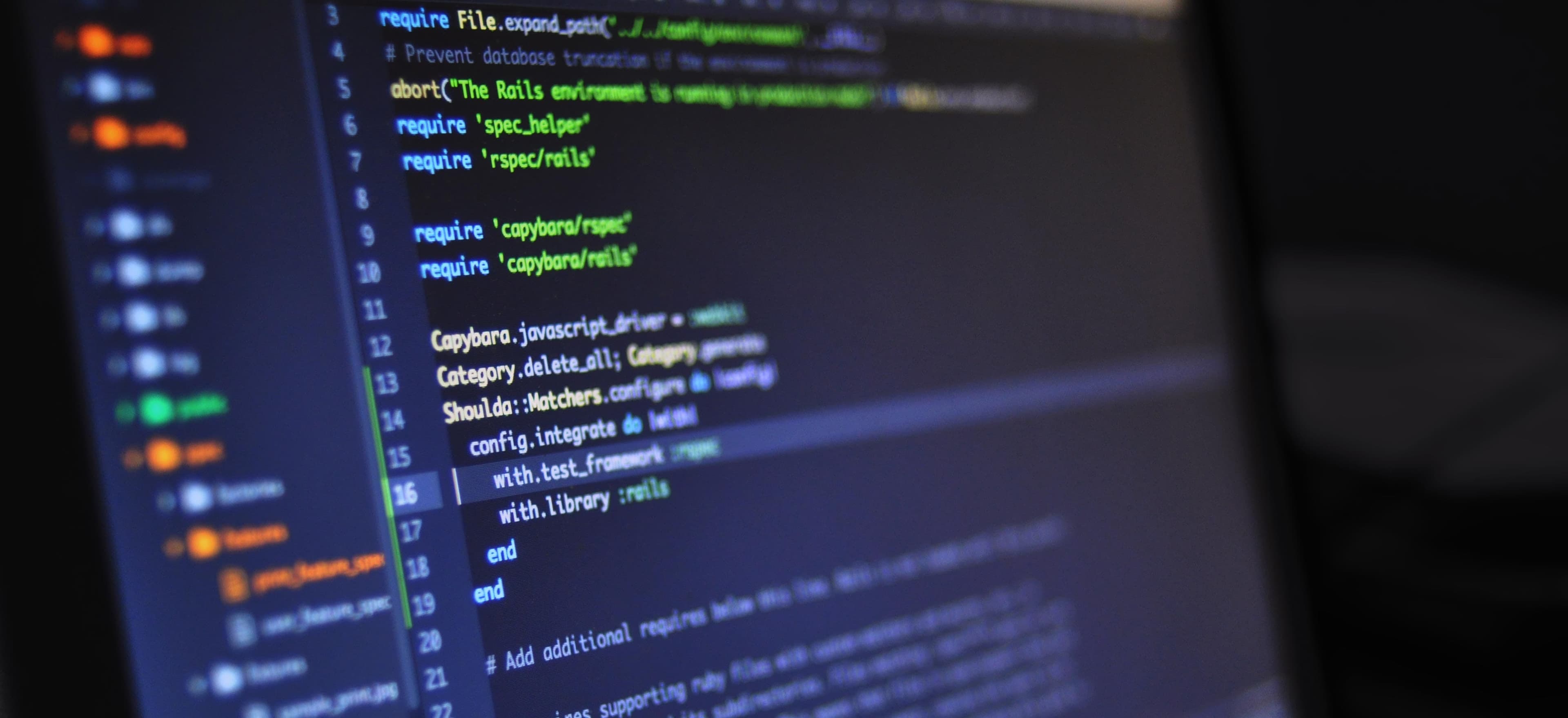
- Published on
Common Redis Configuration Issues in Spring Boot Explained
Redis is a powerful in-memory data structure store that can be used as a cache, message broker, and more. In the context of Spring Boot, integrating Redis can significantly enhance application performance by storing frequently accessed data in memory. However, improper configuration can lead to a range of issues that might impact your application’s efficiency. This blog post will explore common Redis configuration issues in Spring Boot and provide practical solutions for each problem.
Table of Contents:
Before We Begin to Spring Boot and Redis
Spring Boot’s ease of use and seamless integration with Redis via the Spring Data Redis abstraction makes it a natural choice for developers looking to implement caching solutions. However, efficient configurations are essential. Issues with Redis setup can lead to performance bottlenecks, incorrect data retrieval, or even application crashes.
This post will help identify some common pitfalls in Redis configuration and how to resolve them. Whether you are just starting or are an experienced developer looking to optimize your application, you'll find valuable tips here.
Common Configuration Issues
1. Misconfigured Redis Connection
Issue: A frequent issue arises from incorrect Redis connection settings, which can lead to connection timeouts and availability problems.
Solution: Ensure that the connection properties in application.properties
or application.yml
are correctly set. Here's an example configuration:
spring:
redis:
host: localhost
port: 6379
password: yourpassword # Optional, if your Redis server requires authentication
Why: The host
and port
settings tell Spring Boot where to find your Redis instance. If these values don’t match your actual Redis server configuration, your Spring Boot application won't be able to connect. Additionally, ensure that your Redis server is up and running by trying to connect to it using a Redis client.
2. Serialization Problems
Issue: Redis stores data in binary format. If your application tries to serialize and deserialize objects improperly, it could lead to runtime exceptions.
Solution: Use a proper serializer, such as the Jackson2JsonRedisSerializer
. Here is how you can configure it:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer;
@Configuration
public class RedisConfig {
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
// Use JSON serialization
Jackson2JsonRedisSerializer<Object> serializer = new Jackson2JsonRedisSerializer<>(Object.class);
template.setValueSerializer(serializer);
return template;
}
}
Why: The default serializer may not effectively handle complex objects. By switching to Jackson2JsonRedisSerializer
, you ensure that data is stored in a more flexible JSON format, which can help avoid common serialization issues.
If you want to learn more about serialization, check out this article.
3. Network Configurations
Issue: Sometimes, firewalls or network policies restrict access to the Redis server.
Solution: Ensure that your application can communicate with Redis over the appropriate ports. Here are a few steps to check:
- Confirm that the Redis server is accessible from the application server.
- Check your firewall settings to ensure that port 6379 is open.
- If using Docker or cloud solutions like AWS, ensure that the security group settings allow inbound traffic on the Redis port.
Why: Network issues can result in Connection refused
errors or timeouts. By confirming your network settings, you can eliminate one of the most common sources of confusion.
4. Cache Eviction Strategy
Issue: Depending on your application's requirements, the default eviction strategy may not suffice.
Solution: You can define a custom eviction strategy or use existing strategies like LRU (Least Recently Used). Here is a configuration example to set a maximum memory size:
spring:
redis:
cache:
type: redis
redis:
key-prefix: cache:
max-size: 1000 # Maximum number of entries
expire-after-write: 10m # Expiration time
Why: Configuring a sensible eviction strategy can help manage memory usage efficiently. Without these settings, your cache could grow without bounds, leading to performance degradation.
5. Expiration Policy
Issue: Redis allows for expiry times to be set on stored data, but setting these incorrectly can lead to losing necessary data prematurely.
Solution: When using @Cacheable
, ensure you're aware of the default expiration times and configure them as necessary:
@Service
public class MyService {
@Cacheable(value = "myCache", key = "#id", unless = "#result == null")
public MyObject getObjectById(String id) {
// Method implementation
}
}
Why: The @Cacheable
annotation caches the return value of the method based on the specified key. If data expires too quickly, repeated calls to the method will happen, potentially leading to excessive back-end calls. Monitoring and setting an appropriate expiration policy will optimize performance.
The Last Word
Configuring Redis with Spring Boot can significantly improve your application's performance when done properly. However, as outlined above, various pitfalls could arise if configurations are not set correctly. By understanding the common misconfigurations—ranging from connection issues to serialization problems—you can troubleshoot effectively and create a more robust application.
Redis is a powerful tool available to developers, and with the right configuration, it can provide enormous benefits. For more information on Redis and its configuration, check out the official Spring Data Redis documentation.
With the knowledge shared in this post, you're better equipped to address common Redis configuration issues. Happy coding!