Shadow Testing: Safeguarding Against Production Pitfalls
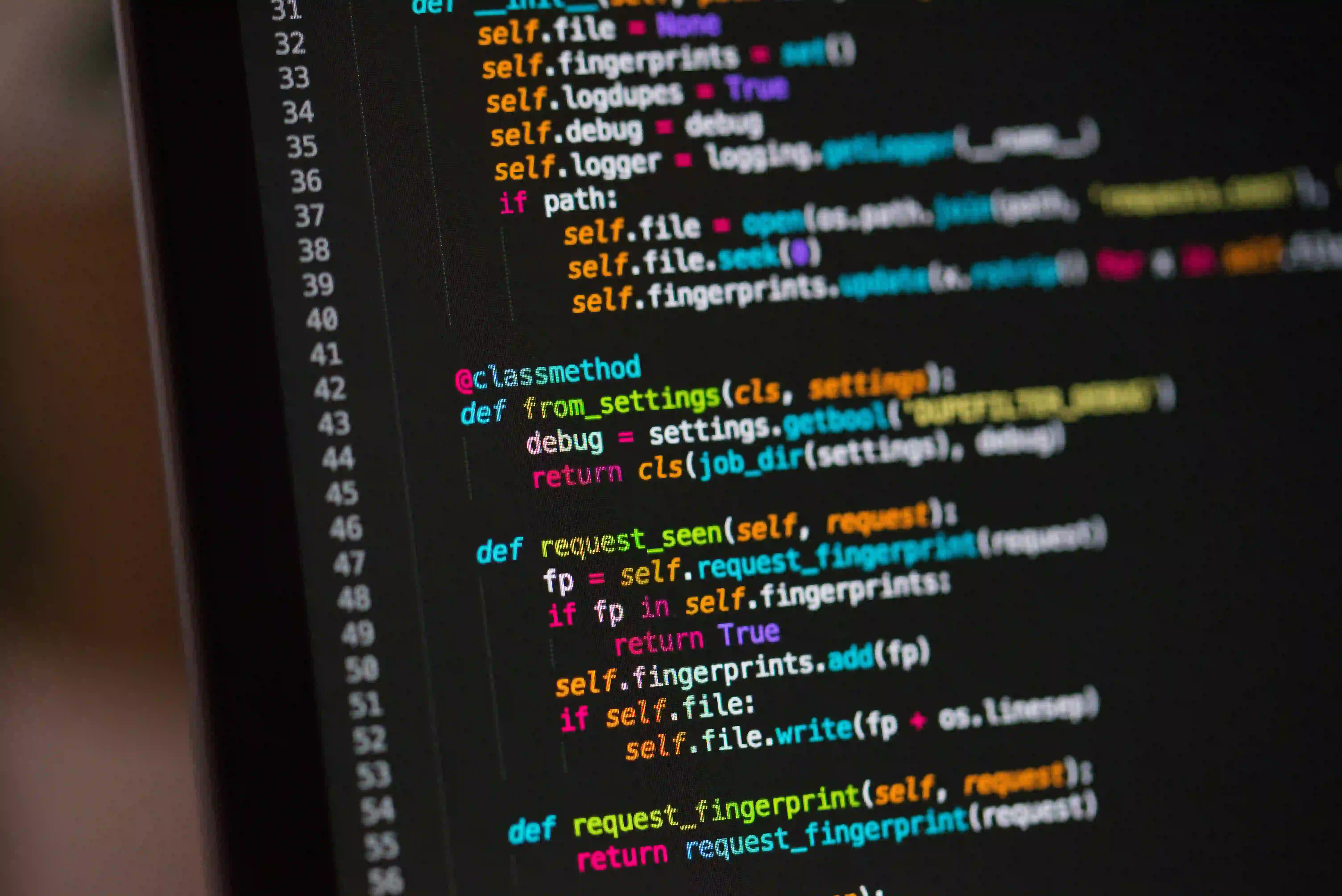
Shadow Testing: Safeguarding Against Production Pitfalls
In the fast-paced world of software development, the difference between success and the downfall of an application can often be traced back to how testing is conducted. In this context, shadow testing has emerged as a vital strategy for identifying issues before they manifest in a live environment. This blog post explores shadow testing, its methodology, its benefits, and how it integrates seamlessly with modern development practices.
What is Shadow Testing?
Shadow testing, often referred to as “dark launch” or “canary deployment,” involves running a new version of software alongside the current version without exposing it to end-users. Instead of performing testing in a staging environment, shadow testing allows developers to operate a production-level test on real data, providing a clearer picture of how the new system performs under real-world conditions.
Why Use Shadow Testing?
- Real-World Data: Unlike synthetic tests, shadow testing utilizes real-world traffic and data, resulting in more accurate feedback.
- Immediate Feedback: Developers can monitor metrics in real-time. This immediate feedback loop helps in identifying bottlenecks or errors quickly.
- Risk Mitigation: Because the new feature is not directly visible to users, organizations can reduce risks associated with deploying new code.
How Does Shadow Testing Work?
Shadow testing primarily involves duplicating requests made to the live application to the new version without affecting the user experience. Here’s a high-level breakdown of the process:
- Clone Requests: The new application receives the same requests as the live version, processes them, but doesn’t return responses to users.
- Collect Metrics: All responses, errors, and performance data from the new application are logged for analysis.
- Analyze Results: Developers review metrics to identify any anomalies or issues present in the new version.
By shadowing requests, teams can ensure that their applications behave as expected without the risk of user impact.
Example Code Snippet
Here's an example of how you might implement a simple shadow testing mechanism in Java using Spring Boot:
@RestController
@RequestMapping("/api")
public class MyController {
@Autowired
private NewService newService;
@Autowired
private CurrentService currentService;
@GetMapping("/data")
public ResponseEntity<DataResponse> getData() {
// The original request is processed
DataResponse currentResponse = currentService.getData();
// Simulate routing the same request to the new service
CompletableFuture<DataResponse> shadowResponse = CompletableFuture.supplyAsync(() -> {
return newService.getData();
});
// Log the response for analysis later
shadowResponse.thenAccept(response -> {
logShadowResponse(response);
});
return ResponseEntity.ok(currentResponse);
}
private void logShadowResponse(DataResponse response) {
// Code to log shadow response - can include metrics, errors, etc.
System.out.println("Shadow response: " + response);
}
}
Commentary on the Code
In the snippet above, we create an API endpoint that serves data. When the endpoint is hit, it processes the request through the current service and simultaneously delegates the same request to the new service. This allows us to capture the response from the new service without affecting the live user experience.
Using CompletableFuture
, we handle asynchronous execution so that logging the shadow response doesn’t block the original response. This is crucial for maintaining performance, especially under high traffic.
Benefits of Shadow Testing
Improved Quality Assurance
Shadow testing allows teams to conduct quality assurance in a risk-free manner. By observing how the new service handles live traffic, teams can quickly spot performance bottlenecks or unexpected behaviors.
Decreased Downtime
When deployed successfully, shadow testing contributes significantly to reducing downtime. By identifying potential issues before fully transitioning to the new system, teams minimize the likelihood of a catastrophic failure.
Enhanced User Experience
With a smoother rollout of features and the ability to manage issues proactively, user experience remains uninterrupted. End-users are seldom aware of the complexities happening behind the scenes, fostering trust in the system.
Integrating Shadow Testing with CI/CD
In today's development landscape, Continuous Integration/Continuous Deployment (CI/CD) pipelines are paramount for operational efficiency. Shadow testing can be seamlessly integrated into these pipelines.
Here’s how you might incorporate shadow testing within a CI/CD framework:
- Automated Tests: Along with unit and integration tests, shadow testing can be automated to run on every deployment, evaluating new features against live traffic.
- Monitoring Systems: Deploy performance monitoring tools that can instantly flag abnormalities stemming from the shadow service.
- Control Thresholds: Set thresholds on performance metrics from the shadow service to determine if the deployment should proceed to the next stage.
Potential Challenges
Resource Intensive
Shadow testing can require additional infrastructure resources. Running two services simultaneously can drive up costs, especially for large-scale applications.
Complexity in Data Management
Handling data privacy is essential. Using real user data for testing introduces potential GDPR and other compliance issues. Teams must ensure that they anonymize or abstract sensitive data when necessary.
Reliability of Shadow Responses
The reliability of shadow responses can vary under load. Misconfiguration in routing or staggering user loads between environments may result in less actionable feedback.
To Wrap Things Up
In conclusion, shadow testing proves to be an effective safety net in the development lifecycle, offering insights that traditional testing lacks. By leveraging real-world data and real-time monitoring, development teams can build high-quality applications that are robust and reliable.
As software development continues to evolve, integrating methodologies like shadow testing will be critical in safeguarding user experience and aligning with the fast-paced nature of real-world applications.
For further reading on testing methodologies, you may find this article and this link insightful. Let the age of informed development begin, and may your deployments keep sailing smoothly!
Feel free to share your thoughts on shadow testing in the comments section below, or let us know your experiences with integrating various testing techniques in your workflow!