Mastering Backoff: Optimize Your Apache Camel Polling
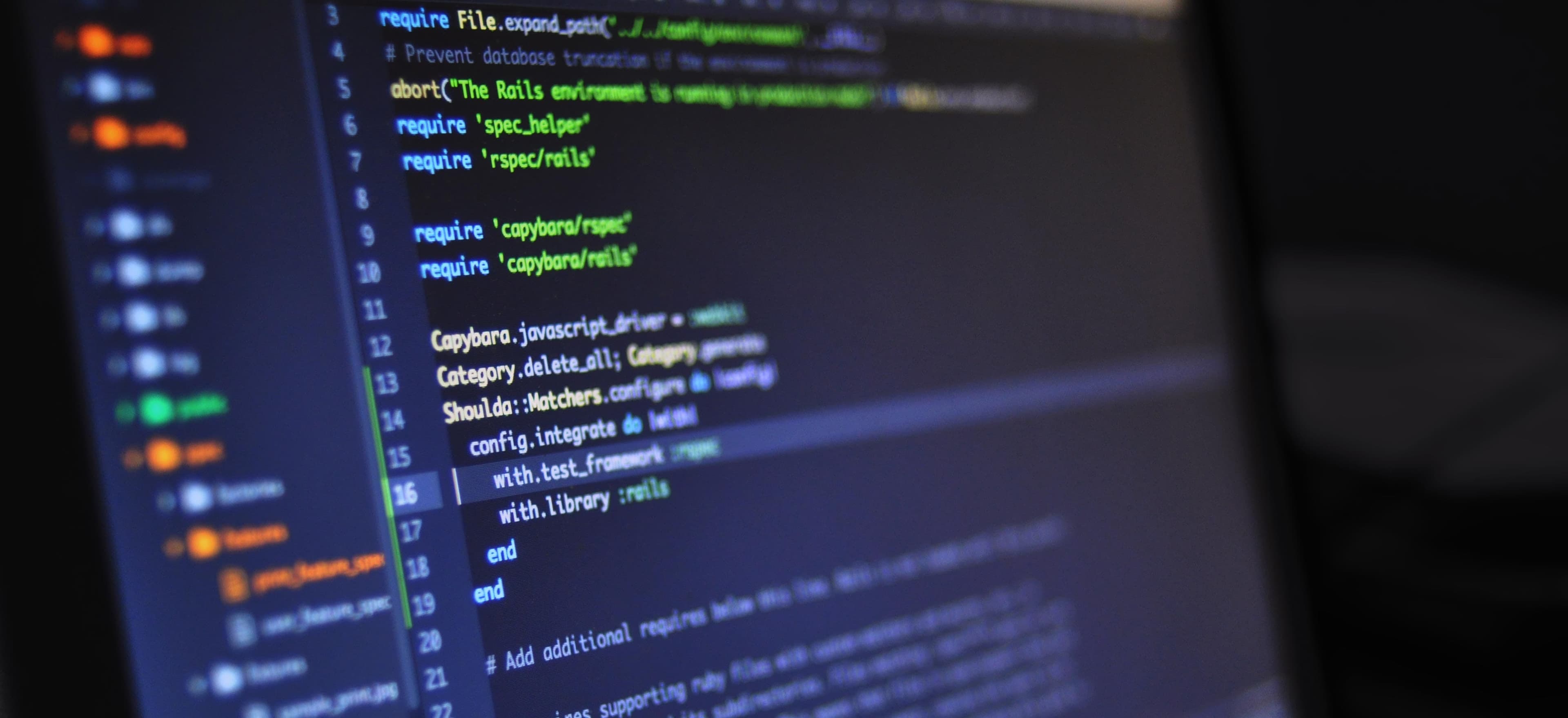
- Published on
Mastering Backoff: Optimize Your Apache Camel Polling
Apache Camel is a powerful open-source integration framework that provides a wide range of features for building robust and flexible integration solutions. However, one area that often requires careful consideration is the polling mechanism when working with external systems. In this blog post, we will explore how to effectively implement backoff strategies to optimize your Apache Camel polling and ensure efficient resource utilization.
Understanding the Need for Polling
Polling is a common pattern in integration solutions, especially when dealing with external systems that do not provide push notifications. In these scenarios, your system needs to continually check for new data or changes. While this can be an effective approach, it also comes with challenges, such as:
- Increased Resource Usage: Frequent polling can lead to excessive resource consumption, potentially overwhelming both your application and the external systems.
- Latency Issues: Polling too often can introduce latency between when data is available and when it is processed.
In order to mitigate these challenges, implementing an effective backoff strategy is crucial.
What is Backoff?
Backoff is a strategy used to reduce the frequency of polling attempts following a failure or a non-responsive state. Instead of continuously trying to poll an external system, backoff allows your application to wait for an extended period before retrying. This approach can help optimize resource use, enhance system responsiveness, and maintain a good relationship with external systems.
Types of Backoff Strategies
There are several backoff strategies, but we will focus on two of the most common:
- Fixed Backoff: Waits for a predetermined amount of time before each retry. This is simple to implement but does not adapt to the situation.
- Exponential Backoff: Increases the wait time exponentially with each failed attempt. This can significantly reduce load on the external system under strain and is generally more efficient.
Implementing Backoff in Apache Camel
The Basic Polling Camel Route
To start, let's create a basic Apache Camel route that polls a database for new records:
from("quartz://poll?cron=0/10+*+*+*+*+?")
.to("jdbc:dataSource?useHeadersAsParameters=true&sql=SELECT * FROM records WHERE processed=false")
.process(new RecordProcessor());
In this route, we use the Quartz component to poll every 10 seconds. However, this does not implement a backoff strategy.
Adding Fixed Backoff
To implement a fixed backoff, we can use the errorHandler
to configure the delay
property for retries. Here's how to modify the route:
from("quartz://poll?cron=0/10+*+*+*+*+?")
.errorHandler(noErrorHandler())
.to("jdbc:dataSource?useHeadersAsParameters=true&sql=SELECT * FROM records WHERE processed=false")
.process(new RecordProcessor())
.onException(SQLException.class)
.maximumRedeliveries(3)
.redeliveryDelay(10000) // Wait 10 seconds before retrying
.log("Error occurred, retrying in 10 seconds...")
.end();
In this example, if an error occurs while processing a record, Camel will retry up to three times, waiting 10 seconds between each retry. This is a simple fixed backoff approach that can prevent overwhelming an external database.
Implementing Exponential Backoff
Exponential backoff can be more effective in scenarios where the probability of success increases with longer wait times. To implement exponential backoff in Apache Camel, we can enhance our error handling logic:
from("quartz://poll?cron=0/10+*+*+*+*+?")
.errorHandler(noErrorHandler())
.to("jdbc:dataSource?useHeadersAsParameters=true&sql=SELECT * FROM records WHERE processed=false")
.process(new RecordProcessor())
.onException(SQLException.class)
.maximumRedeliveries(5)
.redeliveryDelay(5000) // Initial Delay of 5 seconds
.redeliveryDelayStrategy(new ExponentialBackoffStrategy())
.log("Error occurred, will retry in ${exchangeProperty.CamelRedeliveryDelay} milliseconds...")
.end();
Custom Exponential Backoff Strategy
To customize the redelivery delay, you can create an exponential backoff strategy like this:
import org.apache.camel.Exchange;
import org.apache.camel.processor.RedeliveryPolicy;
public class ExponentialBackoffStrategy extends RedeliveryPolicy {
@Override
public long calculateDelay(Exchange exchange, int attempt) {
// Base delay in milliseconds
long baseDelay = 5000; // 5 seconds
// Exponential factor
long delay = (long) (baseDelay * Math.pow(2, attempt));
return delay;
}
}
Benefits of Backoff Strategies
Implementing a backoff strategy can lead to:
- Reduced Resource Usage: By avoiding continuous polling, your application consumes fewer resources and decreases load on external systems.
- Increased Agility: Fewer requests to the external system allow it to recover from overload, enhancing overall stability and performance.
- Error Handling: A well-defined backoff strategy helps manage error handling smoothly, giving you better control over failure scenarios.
Key Takeaways
Optimizing the polling mechanism in Apache Camel is essential for building efficient integration solutions. By mastering backoff strategies, you can ensure that your application does not overwhelm external systems while improving resource utilization.
Utilizing fixed and exponential backoff strategies can immensely help in handling transient failures and improving overall application resilience. Implement these strategies in your Camel routes and see the benefits for yourself, whether you are polling databases, message queues, or any other external system.
For more advanced features and capabilities, take a moment to explore the Apache Camel Documentation, which contains a wealth of information to further enhance your integration skills.
As you move forward, remember to review your logging and monitoring strategies to gain insights into the performance of your polling mechanisms, further enhancing your ability to fine-tune and optimize as necessary.