Mastering Versioning: Avoiding Common Release Pitfalls
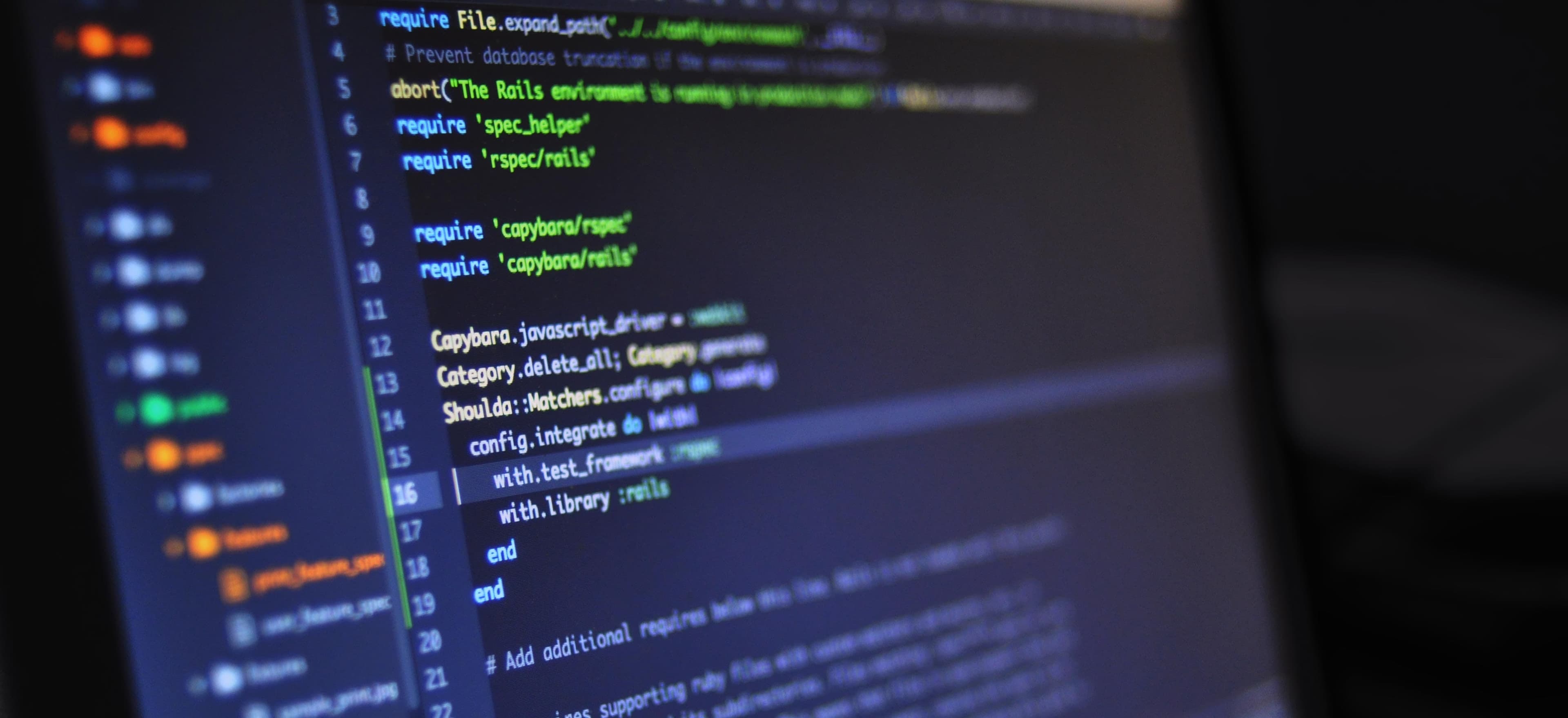
- Published on
Mastering Versioning: Avoiding Common Release Pitfalls
In the ever-evolving world of software development, versioning is a critical yet often overlooked aspect of project management. Having a solid versioning strategy helps maintain the integrity of your software, facilitates collaboration among developers, and provides clarity to end-users. In this blog post, we're diving deep into the ins and outs of versioning, discussing common release pitfalls, and providing practical guidance to help you navigate this essential process.
Why Versioning Matters
Versioning isn't just about numbering your software releases. It encompasses the following:
- Communication: Versioning conveys the changes made, ensuring that everyone - from developers to end-users - understands the current state of the software.
- Reproducibility: Having a versioned code base allows you to recreate specific states of your application for debugging or future development.
- Dependency Management: Proper versioning allows other software that relies on your application to make informed decisions regarding compatibility.
Semantic Versioning: The Gold Standard
The most widely accepted convention for versioning is Semantic Versioning (SemVer). This methodology uses a three-part version number with the format MAJOR.MINOR.PATCH. Let’s break down each component:
- MAJOR: Increased for incompatible changes.
- MINOR: Increased for backward-compatible features.
- PATCH: Increased for backward-compatible bug fixes.
For a more comprehensive understanding of this versioning system, check out the Semantic Versioning Specification.
Common Release Pitfalls
Despite the clarity provided by a proper versioning strategy, many teams fall into significant pitfalls. Let’s discuss some of these pitfalls and how to avoid them.
Pitfall 1: Ignoring Incremental Changes
The Problem
One of the most frequent mistakes teams make is failing to increment the version number for minor changes or patches. This can lead to confusion about the state of the software and unforeseen compatibility issues.
The Solution
Make it a practice to update your version number every time you make a change, no matter how small. This ensures that your team – and your users – can trust that they are working with the most recent version of the software.
// Example: Incrementing the PATCH version number for a minor fix
public class Version {
private int major;
private int minor;
private int patch;
public Version(int major, int minor, int patch) {
this.major = major;
this.minor = minor;
this.patch = patch;
}
public void incrementPatch() {
this.patch++;
}
@Override
public String toString() {
return major + "." + minor + "." + patch;
}
}
// Usage
Version version = new Version(1,0,0);
version.incrementPatch(); // Version becomes 1.0.1
System.out.println("New Version: " + version); // Output: New Version: 1.0.1
Pitfall 2: Not Using Version Control Systems Properly
The Problem
Many developers overlook the importance of version control systems (VCS) like Git. It is common to see teams not tagging their releases, rendering branches difficult to navigate and manage.
The Solution
Utilize the tagging feature of your version control system. Tags provide an easy way to generate snapshots of your project at particular points, providing clarity and structure.
Git Tagging Example:
# Tagging a release in Git
git tag -a v1.0.0 -m "Release version 1.0.0"
git push origin v1.0.0
This simple command creates a tag in your repository, helping everyone keep track of specific releases.
Pitfall 3: Failing to Communicate Changes
The Problem
Assuming everyone knows what each version includes can lead to misunderstandings. This is especially harmful if the changes include backward-incompatible modifications.
The Solution
Implement a CHANGELOG.md in your project to document changes between versions. Clarity is key; providing explicit information on what has changed in each version can save a lot of confusion.
Example of a CHANGELOG.md entry:
# Changelog
## [1.0.1] - 2023-10-01
### Fixed
- Resolved a bug in user authentication that caused session expiration.
## [1.0.0] - 2023-09-15
### Added
- Initial launch with basic authentication flow.
With this approach, your stakeholders know precisely what to expect from each version.
Pitfall 4: Lack of Testing Between Releases
The Problem
One of the worst pitfalls you can encounter is releasing code without thorough testing. It can result in broken features and a frustrating experience for users.
The Solution
Incorporate a robust testing strategy within your CI/CD pipeline. Ensure that every change undergoes unit tests, integration tests, and end-to-end tests before deployment.
Example of a simple unit test using JUnit in Java:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class VersionTest {
@Test
public void testIncrementPatch() {
Version version = new Version(1, 0, 0);
version.incrementPatch();
assertEquals("1.0.1", version.toString());
}
}
By including automated testing, you raise the bar for quality assurance, effectively minimizing the risk of releasing buggy code.
Pitfall 5: Forgetting to Update Documentation
The Problem
Releases can quickly outpace documentation. When updates happen, it is easy to forget to update relevant documents, such as user manuals, API docs, or setup instructions.
The Solution
Adopt a documented process for releasing versions that includes updating related documentation. Consistency in documentation ensures that all users understand how to interact with the software as it evolves.
Lessons Learned
Mastering versioning is indeed a multifaceted endeavor. By adopting a consistent and clear versioning strategy, staying aware of potential pitfalls, and implementing robust practices, you can significantly improve not only your development process but also your end-users' experience.
With the guidelines discussed in this article, from the principles of semantic versioning to the practical recommendations for managing releases, you should be well-equipped to avoid common mistakes and navigate the complexities of software versioning effectively.
For a deeper dive into version control, check out the Pro Git Book.
Happy coding!