Mastering Java: Resolving Iframe JavaScript Load Issues
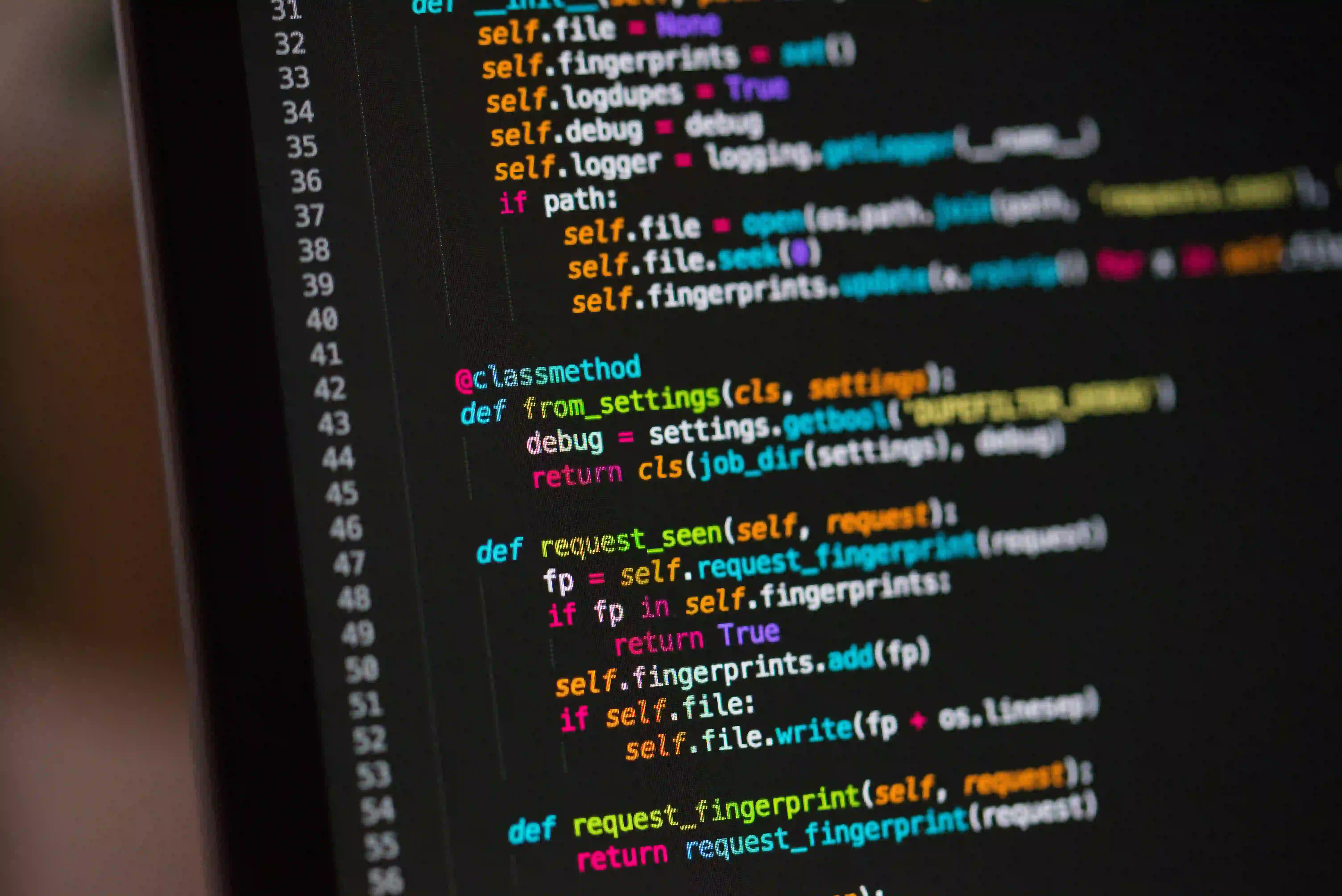
Mastering Java: Resolving Iframe JavaScript Load Issues
In the realm of web development, Iframes provide an efficient way to embed content from one webpage onto another. However, they can come with their own set of challenges—especially when it comes to JavaScript loaded within them. In this blog post, we will explore how to troubleshoot and resolve Java issues related to Iframe JavaScript load failures. This is an extension of the article "Troubleshooting Iframe JavaScript Load Failures" available at infinitejs.com/posts/troubleshooting-iframe-js-load-failures.
Understanding Iframes and JavaScript Interaction
Before diving into solutions, it is crucial to understand how Iframes work. An Iframe (Inline Frame) creates a nested browsing context, where one HTML document is embedded within another. When JavaScript is loaded into an Iframe, it faces unique constraints, primarily due to Same-Origin Policy and cross-domain issues.
The Same-Origin Policy
The Same-Origin Policy (SOP) is a security mechanism implemented in web browsers. It restricts how a document or script loaded from one origin can interact with resources from another origin. In simple terms, if your parent document and Iframe content reside on different domains, browsers will block JavaScript from manipulating Iframe elements or accessing its data.
Why JavaScript Load Failures Occur?
- Cross-Domain Restrictions: Attempting to manipulate or access an Iframe from a different domain triggers SOP violations.
- Timing Issues: JavaScript loaded inside the Iframe might not be executed if the parent document hasn’t completely loaded or if necessary resources aren’t ready.
- Resource Availability: If required JavaScript files or dependencies are missing, the load will fail.
Common Strategies for Resolution
Now that we’ve established a basic understanding, let’s delve into actionable strategies for resolving Iframe JavaScript load issues.
1. Confirming Same-Origin Policies
Before troubleshooting, determine if the content in the Iframe and the parent document share the same origin. Use the following code snippet to check origins:
String frameOrigin = iframe.getLocation().getHost();
String parentOrigin = parent.getLocation().getHost();
if (!frameOrigin.equals(parentOrigin)) {
System.out.println("Different origins: " + frameOrigin + " vs " + parentOrigin);
}
Why Check Origins?
This check is essential because if they don’t match, any attempt to communicate or manipulate the Iframe content using JavaScript will fail due to the SOP.
2. Utilizing PostMessage API
When dealing with cross-origin Iframes, the best solution is to utilize the postMessage()
API. This method provides a secure way to communicate between the parent window and the Iframe.
// In the parent window
const iframe = document.getElementById("myIframe");
iframe.contentWindow.postMessage('Hello from parent!', 'https://example.com');
// In the Iframe
window.addEventListener("message", function(event) {
if (event.origin !== "https://your-parent-domain.com") return;
console.log("Received message:", event.data);
});
Why Use postMessage?
The postMessage()
API allows interaction between different origins safely. You can send messages to the Iframe and vice versa without violating the Same-Origin Policy.
3. Using "load" Event Listeners
Another common source of load failures is timing issues. By using event listeners for the "load" event, you ensure that your JavaScript code executes only after the Iframe has fully loaded.
const iframe = document.getElementById('myIframe');
iframe.addEventListener('load', function() {
const iframeDocument = iframe.contentDocument || iframe.contentWindow.document;
// Run your code here
});
Why Load Event Listeners?
Using a load event listener guarantees that the entire content is ready for manipulation before executing your JavaScript code, therefore reducing errors due to unavailability of elements.
4. Debugging Tools for Load Failures
If JavaScript within your Iframe is still struggling to load, leverage debugging tools such as browser developer tools. Watch for console errors and verify that all resources are properly linked.
Control errors with Java's logging framework:
Logger logger = Logger.getLogger(getClass().getName());
try {
// Sensitive iframe loading code
loadIframe();
} catch (Exception e) {
logger.severe("Iframe load failed: " + e.getMessage());
}
5. Implementing Fallback Content
In instances where Ifames fail to load properly, provide fallback content. This could be an error message or alternative content that ensures users can still engage with your application.
<iframe src="https://example.com" onload="this.style.display='';" style="display:none;">
<p>Your browser does not support Iframes. Here's an alternative content.</p>
</iframe>
Why Fallback Content?
Fallback content helps enhance user experience. Users are promptly informed when something goes wrong, providing clarity about potential issues.
My Closing Thoughts on the Matter
Troubleshooting and resolving Iframe JavaScript load failures can be challenging, but with the right understanding and techniques, you can efficiently manage these issues. Always remember to check for the Same-Origin Policy, utilize the postMessage API for secure communication, implement load event listeners, debug properly, and provide fallback content.
By mastering these strategies, you'll not only improve the reliability of your web applications but also enhance user experience. For more in-depth discussions and examples, refer to the article "Troubleshooting Iframe JavaScript Load Failures" at infinitejs.com/posts/troubleshooting-iframe-js-load-failures. Happy coding!