Resolving Database Connection Issues in Java Web Apps
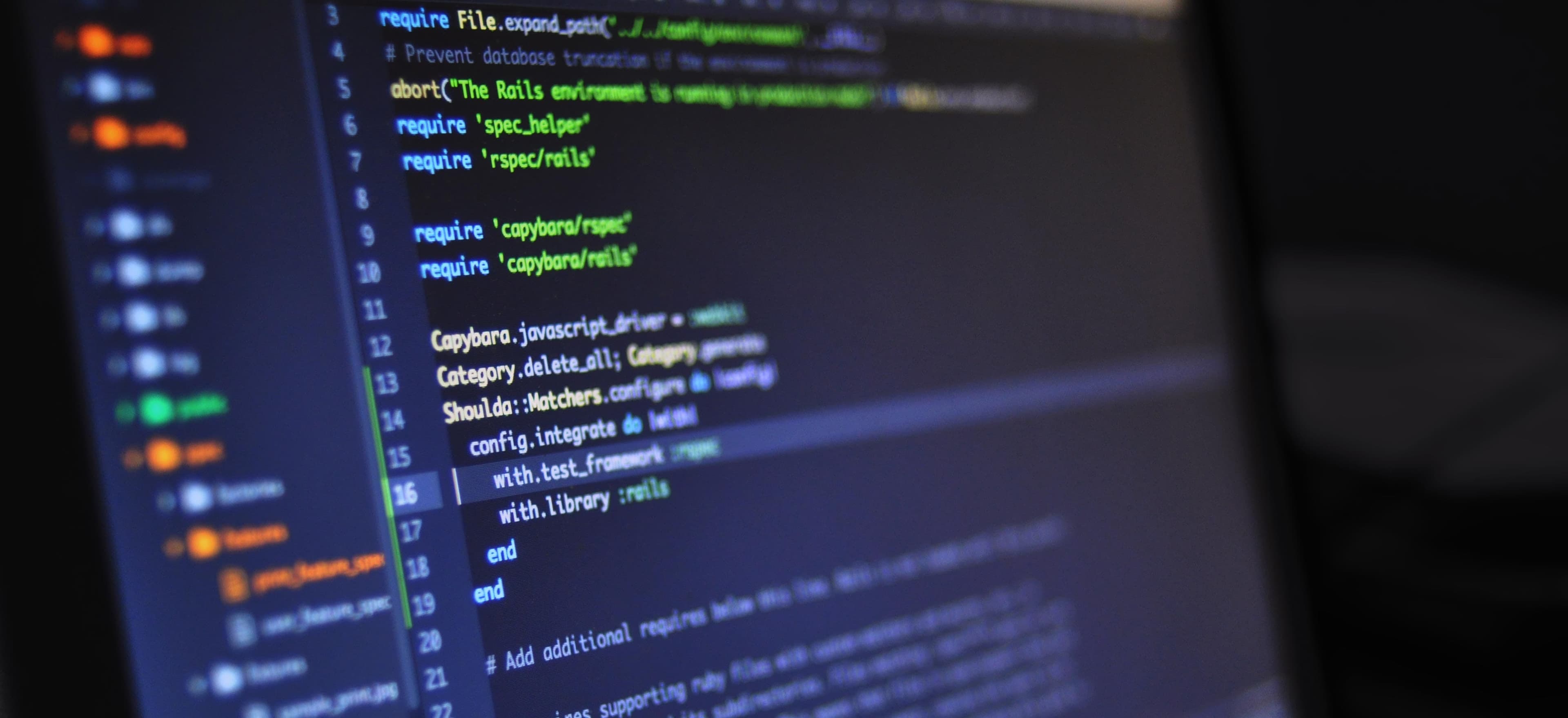
- Published on
Resolving Database Connection Issues in Java Web Apps
In today's digital landscape, building robust Java web applications requires a seamless connection with databases. A common hiccup developers encounter is establishing an effective database connection. Whether you're using MySQL, PostgreSQL, or another RDBMS, connection issues can be a hurdle that quickly slows down your project. In this article, we'll dive into common database connection issues in Java web applications and explore best practices for resolving them.
Importance of Database Connections
Before delving into solutions, let's discuss why database connections are pivotal. Databases store vital information that your application relies on. Connecting to a database is the bridge through which an application interacts with this data. A weak or broken connection can result in application errors, performance bottlenecks, and user frustration. Understanding how to manage these connections effectively can enhance not just the efficiency of your app but also the overall user experience.
Common Database Connection Issues
1. Incorrect Database Credentials
One of the most frequent issues web developers face is providing incorrect database credentials, which includes the username, password, and database URL. If any of these details are incorrect, the application will fail to establish a connection.
Example Code Snippet:
String dbURL = "jdbc:mysql://localhost:3306/mydatabase";
String user = "myUser";
String password = "myPassword";
try {
Connection connection = DriverManager.getConnection(dbURL, user, password);
} catch (SQLException e) {
System.out.println("Database connection failed! " + e.getMessage());
}
In the above code, we attempt a connection to a MySQL database. If the credentials are incorrect, SQLException
will be thrown, providing us with the reason for the connection failure. This is a prime example of how critical accurate credentials are in database communication.
2. Database Driver Issues
Another challenge developers run into is using the wrong database driver. Each database comes with a specific JDBC driver that facilitates connections. If the driver is not included in your project’s dependencies, your application will not function properly.
Maven Dependency Example:
To include the MySQL driver in a Maven project, you can add the following dependency to your pom.xml
:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
Make sure the version matches the requirements of your database and Java version. Mismatched details can lead to driver-related exceptions that hinder connection establishment.
3. Network Issues
Sometimes the issue isn’t with credentials or drivers; it’s external, such as network connectivity. If your application is trying to access a remote database, ensure that the server is reachable and that there are no firewall or network obstacles hindering the connection.
Troubleshooting Steps:
- Ping the database server to check connectivity.
- Ensure that the database service is up and running.
- Verify network configurations, such as firewall rules.
4. Connection Pooling
For applications requiring multiple concurrent database connections, connection pooling is essential. Many developers neglect to implement pooling, which can lead to performance issues and connection timeouts.
Using libraries like HikariCP or Apache DBCP can help manage connection pools efficiently.
Example of HikariCP Configuration:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase");
config.setUsername("myUser");
config.setPassword("myPassword");
HikariDataSource dataSource = new HikariDataSource(config);
HikariCP allows your application to manage multiple connections efficiently, significantly improving performance and reducing wait times. A good connection pool can handle sustaining connections at peak times, leading to a better user experience.
5. Connection Timeout Issues
Connection timeout can occur when the database takes too long to respond. This usually happens when the database is under heavy load or experiencing latency issues.
To resolve this, you can set a connection timeout parameter in your JDBC URL or in your connection pooling configuration.
Example of Connection Timeout:
config.setConnectionTimeout(30000); // Set timeout to 30 seconds
Adjusting the timeout increases the chances of a successful connection, especially during high traffic periods.
Best Practices for Database Connections in Java
1. Use Environment Variables for Credentials
To maintain security, avoid hardcoding database credentials into your source code. Use environment variables to store sensitive information. This ensures that credentials are not exposed in your repository.
2. Implement Proper Exception Handling
Exception handling is vital for understanding where connections fail. Use robust logging to trace issues effectively.
try {
Connection connection = DriverManager.getConnection(dbURL, user, password);
} catch (SQLException e) {
logger.error("Database connection failed with error: ", e);
}
3. Optimize Connection Configuration
Adjust connection parameters based on your application's load and performance requirements. Experiment with pool sizes, timeout values, and other configurations for optimal performance.
4. Regularly Update Database Drivers
Ensure that you're using the latest versions of database drivers to leverage improved features and security patches. Outdated drivers can lead to incompatibility issues and performance hits.
Further Reading: Migrating Local WordPress Site to Live Server
For those transitioning a project from a local development environment to a live server, it's essential to address database connection concerns thoroughly. A comprehensive guide on the subject can be found in the article titled "Migrating Local WordPress Site to Live Server: Database Connection Concern" at infinitejs.com/posts/migrating-wordpress-site-live-server-database-connection-concern. This resource provides valuable insights into handling database connection issues during migration, which can also be relevant to Java developers.
The Closing Argument
Database connection issues can significantly impact the performance and reliability of Java web applications. By understanding the common pitfalls and implementing best practices, you ensure that your application maintains a strong connection with its database, leading to a smoother experience for users. Leverage the strategies discussed here and keep your applications running efficiently.