Handling CORS Issues in Java: A Developer's Guide
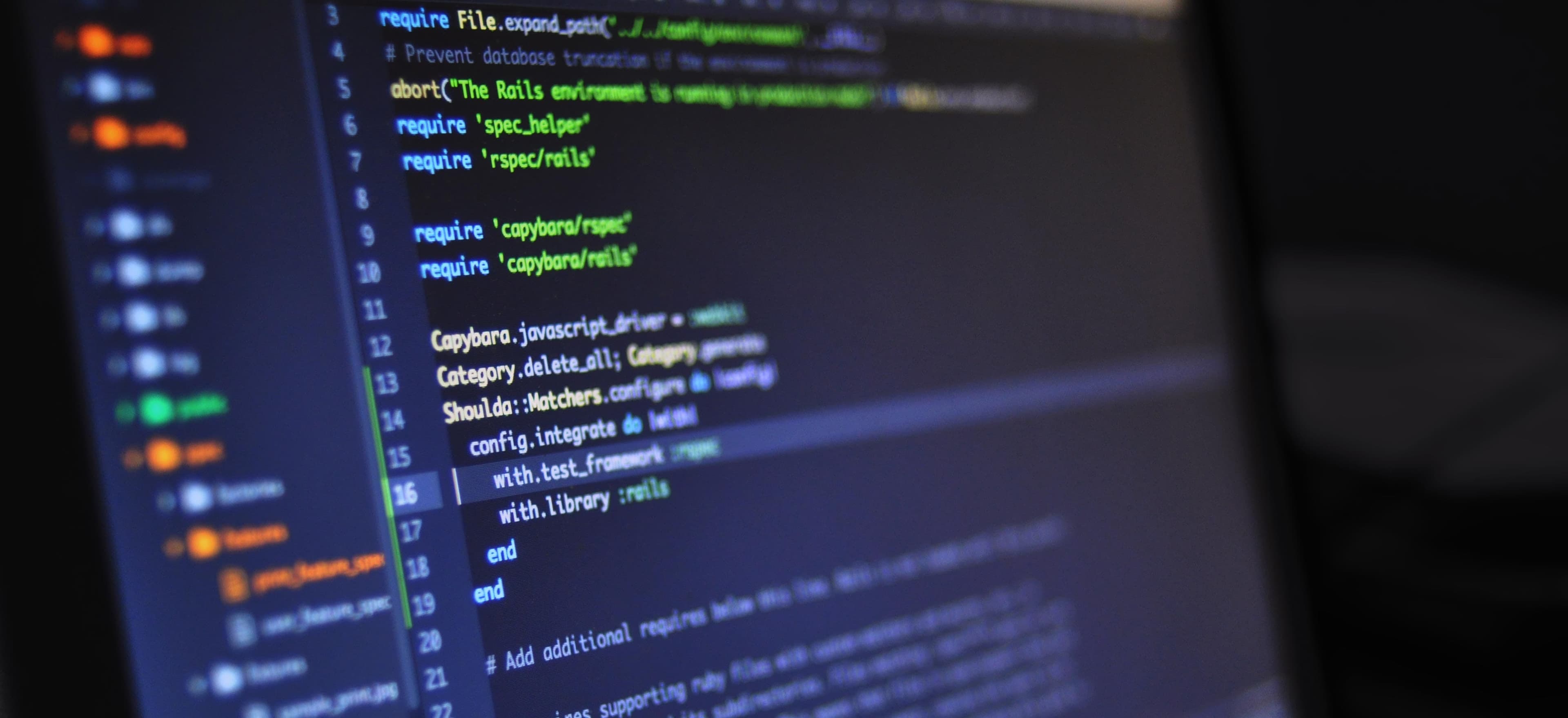
- Published on
Handling CORS Issues in Java: A Developer's Guide
Cross-Origin Resource Sharing (CORS) is a crucial mechanism that enables web applications to request resources from different domains. While it enhances flexibility, CORS can also pose significant challenges to developers. This article aims to explore how Java developers can effectively handle CORS issues. Along the way, we’ll reference key operations, clear explanations, and examples to provide a comprehensive understanding of the subject.
What is CORS?
CORS is a security feature implemented by web browsers to control access to resources hosted on different origins. An origin is defined by a combination of protocol, domain, and port. Without CORS, a web page can only request resources from the same origin. When a request is made to a different origin, the browser blocks it unless the target server includes the necessary CORS headers in its response.
For an in-depth understanding of CORS, consider reading the article Demystifying CORS: Solving Cross-Origin Resource Sharing.
Why Handling CORS is Important
When developing applications, especially with Java backend frameworks like Spring or Jakarta EE, CORS issues often emerge. Developers may face problems when frontend applications attempt to access APIs or resources from a server that resides on a different origin.
Handling CORS correctly is vital for several reasons:
- User Experience: Smooth integration between frontends and backends ensures the user experience is seamless.
- Security: Correctly implemented CORS policies bolster security while allowing legitimate requests to proceed.
- Interoperability: Ensuring different origins can communicate effectively is essential in a distributed architecture.
How CORS Works
CORS operates through a system of HTTP headers that determine whether a cross-origin request should be permitted. Here is a typical flow:
- Preflight Request: For some requests (like
PUT
orDELETE
), the browser sends a preflight request to determine if the actual request is safe. - CORS Headers: The server responds with appropriate headers such as
Access-Control-Allow-Origin
, which specifies trusted origins. - Final Request: If the preflight checks pass, the actual request is sent.
CORS Headers
Below are the most common CORS headers:
- Access-Control-Allow-Origin: Specifies which origins are allowed to access resources.
- Access-Control-Allow-Methods: Lists the HTTP methods the server supports.
- Access-Control-Allow-Headers: Lists the headers the browser can use in the actual request.
- Access-Control-Max-Age: Indicates how long the results of a preflight request can be cached.
Configuring CORS in Java with Spring Boot
Setting Up CORS in Spring Boot
Spring Boot makes it relatively straightforward to configure CORS through its built-in support. Here’s a step-by-step illustration:
-
Add Dependencies: Ensure you have the required Spring dependencies in your
pom.xml
.<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
-
Global CORS Configuration: Implement a CORS configuration class. This example demonstrates how to allow all origins while limiting methods.
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.web.servlet.config.annotation.CorsRegistry; import org.springframework.web.servlet.config.annotation.WebMvcConfigurer; @Configuration public class WebConfig implements WebMvcConfigurer { @Override public void addCorsMappings(CorsRegistry registry) { registry.addMapping("/**") .allowedOrigins("*") // Allows all origins .allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS") // HTTP methods allowed .allowedHeaders("*"); // Allows all headers } }
In this configuration, we use
addMapping("/**")
to apply the CORS settings to all endpoints. TheallowedOrigins("*")
specifies that requests from any origin are permissible, which is useful during development but should be restricted in production.
CORS Configuration for Specific Endpoints
In production environments, it’s best practice to limit access to specific origins and endpoints. Here’s how to do that:
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**") // Only for paths starting with api
.allowedOrigins("https://example.com") // Allow only this origin
.allowedMethods("GET", "POST");
}
This setup ensures that requests are only accepted from https://example.com
for endpoints that start with /api/
.
CORS in Jakarta EE
For those working with Jakarta EE, handling CORS manually in your JAX-RS resources is necessary.
Custom CORS Filter
Here’s how to create a custom CORS filter:
import javax.ws.rs.container.ContainerResponseFilter;
import javax.ws.rs.container.ContainerRequestContext;
import javax.ws.rs.container.ContainerResponseContext;
import javax.ws.rs.ext.Provider;
import javax.ws.rs.core.Response;
import javax.ws.rs.HttpMethod;
@Provider
public class CORSFilter implements ContainerResponseFilter {
@Override
public void filter(ContainerRequestContext requestContext, ContainerResponseContext responseContext) {
responseContext.getHeaders().add("Access-Control-Allow-Origin", "*");
responseContext.getHeaders().add("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS");
responseContext.getHeaders().add("Access-Control-Allow-Headers", "Content-Type, Authorization, X-Requested-With");
if (requestContext.getMethod().equalsIgnoreCase(HttpMethod.OPTIONS)) {
responseContext.setStatus(Response.Status.OK.getStatusCode());
}
}
}
This filter intercepts all responses and modifies them to include CORS headers. We also check if the request method is OPTIONS
and respond with HTTP 200 OK
, which is crucial for preflight requests.
CORS Configurations for Web Applications
Often, Java applications are combined with Angular, React, or Vue.js frontends. These applications require CORS handling for seamless operation.
- Configure Client-Side Tools: Set up client-side tools like Axios or Fetch to make cross-origin calls properly.
- Backend Adjustments: Remember to adjust the backend accordingly to allow those origins.
Debugging CORS Issues
Despite proper configurations, developers may still encounter issues. Here are a few debugging tips:
- Browser Console: Utilize the browser's developer tools to inspect network requests. Look for CORS-related errors.
- Log Responses: Log server responses to ensure correct headers are being sent.
- Test with Multiple Browsers: Different browsers may have subtle differences in CORS support.
Example Debugging Scenario
Assume a frontend application running on http://localhost:3000
tries to access a backend on http://localhost:8080/api/data
. If CORS is not properly configured, the browser will block the request, leading to an error message like this:
Access to XMLHttpRequest at 'http://localhost:8080/api/data' from origin 'http://localhost:3000' has been blocked by CORS policy:
No 'Access-Control-Allow-Origin' header is present on the requested resource.
Reviewing your backend setup will help determine why the headers are missing or incorrect, thus guiding you to a solution.
In Conclusion, Here is What Matters
CORS is a vital concept for web developers aimed at enabling cross-origin requests while ensuring security. In Java, whether using Spring Boot or Jakarta EE, handling CORS elegantly will lead to more robust, secure applications that provide an excellent user experience.
By understanding how CORS works and applying the configurations discussed here, developers can resolve most CORS issues effectively.
For additional insights, check out the article on CORS Demystifying CORS: Solving Cross-Origin Resource Sharing.
Additional Resources
- Spring Documentation on CORS
- Jakarta EE Tutorials
With these guidelines, you can become proficient at handling CORS in your Java applications, boosting both functionality and security. Happy coding!
Checkout our other articles