Mastering Flexbox Alignment with Java-based Web Frameworks
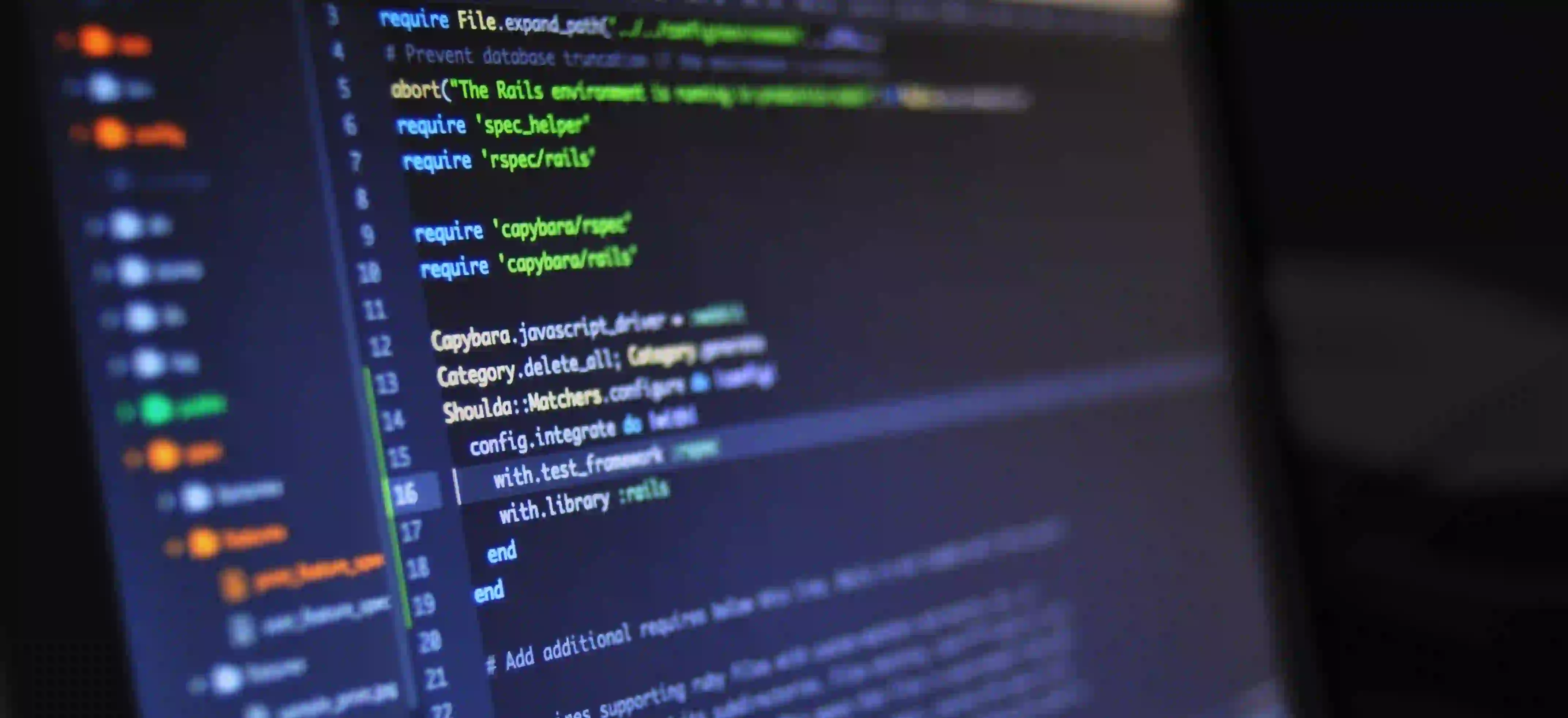
Mastering Flexbox Alignment with Java-based Web Frameworks
The modern web development landscape is ever-evolving, presenting developers with new tools and methodologies to enhance user experience. One such significant advancement is the Flexbox layout model, which greatly simplifies the alignment and distribution of space among items in a container. In this article, we'll explore how to master Flexbox alignment within Java-based web frameworks like Spring and JavaServer Faces (JSF). Our focus will be on practical examples and why these implementations matter, helping you create responsive layouts that are flexible and intuitive.
Understanding Flexbox: The Basics
Flexbox, or the Flexible Box Layout, is a CSS layout model that allows items in a container to be arranged efficiently, even when their size is unknown. The core tenets of Flexbox focus on simplifying alignment, spacing, and the overall flow of your layout.
To get started, let’s look at the most critical properties of Flexbox:
display: flex;
- Enables the flex context for the containerflex-direction
- Specifies the direction flex items are placed in the flex container (row, column, etc.)justify-content
- Aligns items horizontally and manages spacing in the flex containeralign-items
- Aligns items vertically within the flex containerflex-wrap
- Controls whether flex items should wrap onto multiple lines
Understanding these properties will set the foundation for effective Flexbox implementations in your Java-based web applications.
Implementing Flexbox in Java-based Web Frameworks
1. Spring Boot with Thymeleaf
Overview:
Spring Boot is a robust framework designed to create Java-based enterprise applications. Combined with Thymeleaf, a Java library for rendering web views, we can easily integrate Flexbox into our web templates.
Step-by-step Example:
Let’s create a simple layout with three divs, applied with Flexbox for alignment.
HTML - Thymeleaf Template
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Flexbox Layout</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="flex-container">
<div class="flex-item item-1">Item 1</div>
<div class="flex-item item-2">Item 2</div>
<div class="flex-item item-3">Item 3</div>
</div>
</body>
</html>
CSS - style.css
.flex-container {
display: flex; /* Create a flex container */
justify-content: space-around; /* Distribute space evenly */
align-items: center; /* Center items vertically */
height: 100vh; /* Full height */
}
.flex-item {
background-color: lightblue; /* Background color for visibility */
padding: 20px;
width: 100px; /* Set width for items */
border: 1px solid blue;
}
Explanation:
display: flex;
makes the.flex-container
a flex container, allowing its direct children (the items) to be aligned and spaced logically.justify-content: space-around;
creates equal spacing around each flex item, providing a clean, balanced layout.align-items: center;
ensures that all items are vertically centered regarding the flex container's height.
2. JavaServer Faces (JSF)
Overview:
JavaServer Faces (JSF) is a Java specification for building component-based user interfaces. You can easily implement Flexbox layouts within JSF views.
Example Layout in JSF:
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html">
<head>
<title>JSF Flexbox Example</title>
<link rel="stylesheet" type="text/css" href="style.css" />
</head>
<body>
<h:panelGroup layout="block" styleClass="flex-container">
<h:panelGroup styleClass="flex-item item-1">Item 1</h:panelGroup>
<h:panelGroup styleClass="flex-item item-2">Item 2</h:panelGroup>
<h:panelGroup styleClass="flex-item item-3">Item 3</h:panelGroup>
</h:panelGroup>
</body>
</html>
CSS - style.css
The same CSS applies here, ensuring a consistent layout across both frameworks.
Why Use Flexbox in Java-Based Frameworks?
The innovative abilities of Flexbox transform your coding approach, enhancing organization and responsiveness. When using Java frameworks like Spring Boot or JSF:
- Responsive Design: Flexbox adapts to various screen sizes, providing a superior experience without writing extensive CSS.
- Ease of Use: The declarative nature of Flexbox allows developers to write cleaner, maintainable code.
- Alignment and Distribution: Simplifies complicated layouts through simple properties, reducing the need for complex calculations.
For more insights on how to arrange elements within your designs and understanding the intricacies of CSS layout models, refer to the article titled Struggling to Arrange Three Divs Side by Side in Desktop View, which explains layout challenges in depth.
Advanced Flexbox Techniques
Once you're comfortable with basic Flexbox properties, consider diving deeper into some advanced techniques:
- Nested Flex Containers: You can create a more complex layout by nesting flex containers within each other. This is useful when you require different alignment settings for various sections of a layout.
.parent {
display: flex;
}
.child {
display: flex; /* Child becomes a flex container */
justify-content: flex-end; /* Alternative alignment */
}
- Flex Item Properties: You can control how individual flex items grow and shrink.
.flex-item {
flex-grow: 1; /* Allow items to grow equally */
flex-basis: 100px; /* Start width*/
}
These properties allow for better control of how flex items behave in different screen sizes and can greatly enhance your user interface design.
Final Thoughts
Mastering Flexbox alignment in Java-based web frameworks introduces you to a powerful technique for creating responsive web layouts. By leveraging frameworks like Spring and JSF, you can simplify your coding experience, enhance design consistency, and ensure your applications are user-friendly.
As the world of web development continues to grow and evolve, staying updated with tools like Flexbox will ensure you can craft layouts that are not only visually appealing but also adaptable to the modern user experience. Don’t hesitate to explore more complex scenarios, and continue to refine your understanding of CSS alongside Java. Happy coding!