Overcoming Cold Start Delays in Serverless Spring Apps
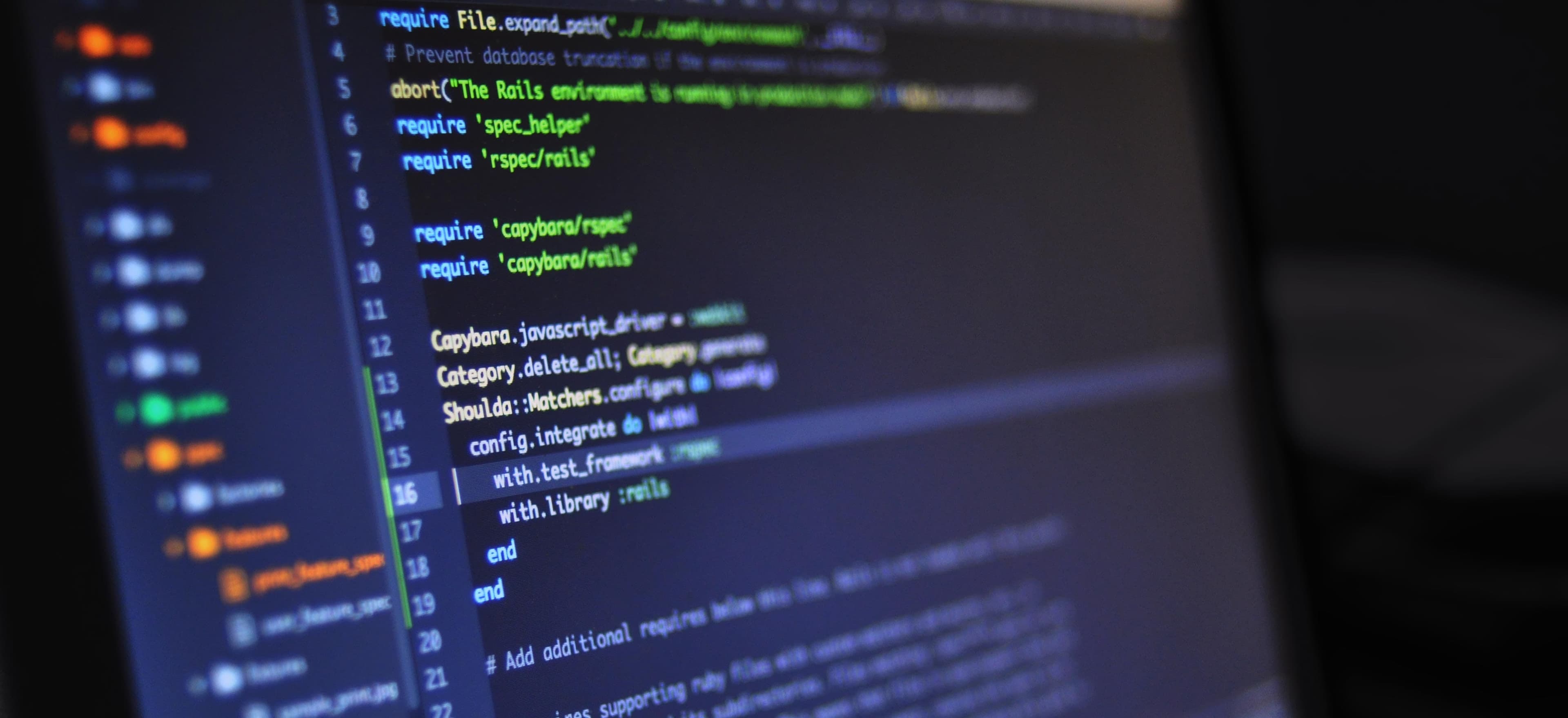
- Published on
Overcoming Cold Start Delays in Serverless Spring Apps
As more developers embrace serverless architectures to boost the efficiency of their applications, they often encounter a common hurdle: the dreaded cold start delay. This challenge is particularly evident in Spring applications, given their extensive reliance on various libraries and configurations. In this post, we will explore what cold starts are, why they occur in serverless Spring apps, and how to mitigate these delays effectively.
Understanding Cold Starts
A cold start occurs when a serverless function is invoked after being idle for a period, requiring the cloud provider to allocate resources, initialize the runtime, and load any necessary libraries before executing the function. This process can result in higher latencies that could degrade user experience.
Why Cold Starts Matter
In applications that require high performance and quick response times, every millisecond counts. Cold starts can dramatically affect:
- User Experience: Prolonged loading times can frustrate users.
- Application Performance: Increased latencies can lead to inefficiencies.
- Cost: Resources not utilized effectively can lead to higher operational costs.
Why Cold Starts Happen in Serverless Spring Apps
If you're using a serverless framework like AWS Lambda or Azure Functions with Spring, several factors contribute to cold starts:
- Dependency Loading: Spring applications often load numerous dependencies, leading to longer initialization times.
- Framework Overhead: The Spring ecosystem adds significant overhead due to dependency injection, which can delay function execution.
- JVM Warm-up: The Java Virtual Machine itself requires time to initialize.
Understanding these factors is essential for implementing strategies to reduce cold start delays effectively.
Strategies to Mitigate Cold Starts
Here are several effective strategies to avoid cold start delays in your serverless Spring applications.
1. Use Graviton Instances on AWS Lambda
AWS Graviton processors, based on the Arm architecture, offer improved performance and lower cost. They particularly help in reducing cold starts. When configuring your AWS Lambda function, opt for Graviton for faster startup times.
# Example of defining a Graviton function in AWS SAM template
Resources:
MyFunction:
Type: AWS::Serverless::Function
Properties:
Architectures:
- arm64
2. Reduce Application Size
Minimizing the application size can significantly reduce cold start times. You can achieve this by:
-
Removing Unused Dependencies: Use tools like Maven’s dependency tree to identify unused libraries.
mvn dependency:tree
-
Optimizing Spring Boot configurations: Exclude certain auto-configurations that are not necessary for your application.
@SpringBootApplication(exclude = { DataSourceAutoConfiguration.class })
public class MySpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringBootApplication.class, args);
}
}
By eliminating unnecessary components, you minimize the initialization overhead.
3. Use Provisioned Concurrency
Provisioned Concurrency keeps your function ‘warm’ by maintaining a specified number of instances of your function running, regardless of traffic. It ensures low latency even during peak loads.
# Example of enabling Provisioned Concurrency
Resources:
MyFunction:
Type: AWS::Serverless::Function
Properties:
ProvisionedConcurrencyConfig:
ProvisionedConcurrentExecutions: 5
While this approach may increase costs, it ensures a more responsive application.
4. Implement Lazy Loading
Lazy loading allows you to defer the initialization of certain components until they are actually needed. This can help speed up the initial startup of your application.
@Configuration
public class MyConfiguration {
@Bean
@Lazy
public MyService myService() {
return new MyService();
}
}
This technique delays the load of certain services, reducing the initial cold start impact.
5. Optimize JVM Startup
The Java Virtual Machine can be fine-tuned to reduce startup time. Some options include:
- Using Native Images: Tools like Spring Boot’s native image feature enable you to compile into a native executable, drastically improving startup time.
- JVM Options: Tuning garbage collection settings and other JVM parameters can sometimes lead to improvements.
The Bottom Line
Cold starts in serverless Spring applications can pose significant challenges. However, by employing strategies such as using Graviton instances, reducing application size, implementing lazy loading, and configuring provisioned concurrency, you can mitigate these delays effectively.
Additional resources to explore:
- AWS Lambda and Cold Starts
- Spring Native
Incorporating these practices will not only enhance your serverless Spring applications' performance but also improve overall user satisfaction. Don't settle for slow response times; adapt your application to meet the demands of modern web services!