Overcoming Latency Issues in Big Data Streaming Solutions
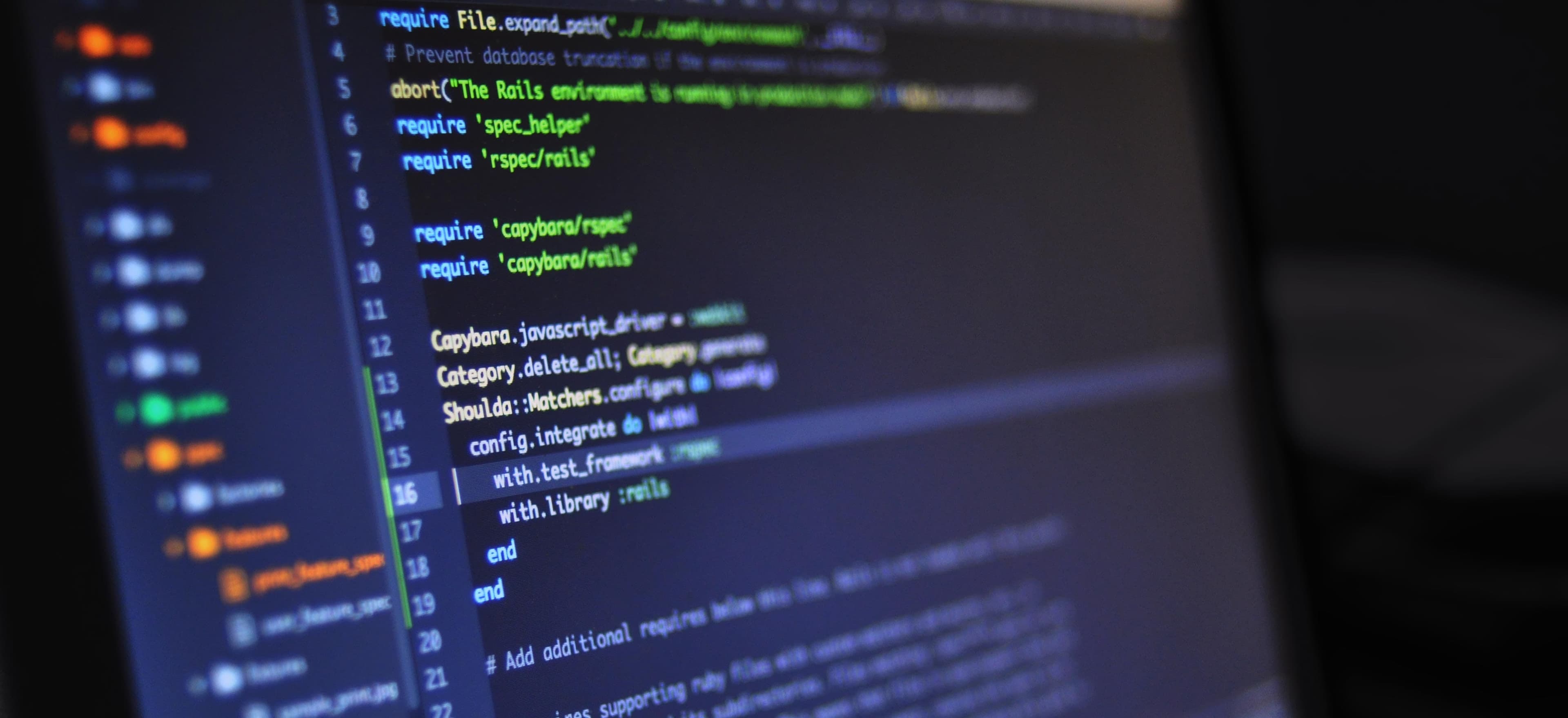
- Published on
Overcoming Latency Issues in Big Data Streaming Solutions
In today's rapidly evolving digital landscape, businesses are increasingly relying on big data streaming solutions to deliver real-time insights and drive decision-making. However, one significant challenge that often arises is latency. Latency can lead to delays in data processing, resulting in slower response times and less informed business decisions. This blog post explores the nature of latency issues in big data streaming, along with effective strategies to overcome them.
Understanding Latency in Big Data Streaming
Latency refers to the time it takes for data to travel from its source to its destination and be processed. In a big data streaming context, latency can stem from various factors, including network delays, data processing bottlenecks, and inefficient algorithms. Lowering latency is crucial because even a few milliseconds can have a substantial impact on performance, especially in applications like financial trading or real-time analytics.
Common Causes of Latency
Before we can address latency, it's essential to understand its common causes. The following factors can contribute to high latency in big data streaming solutions:
- Network Latency: Delay caused by data traveling through a network.
- Processing Delays: Time taken by the data processing engine to handle incoming data.
- Buffering Delays: Introduced when aggregating data before processing.
- Serialization Overhead: The process of converting data into a format suitable for transmission can add latency.
Impact of Latency
High latency can lead to:
- Poor user experience.
- Decreased operational efficiency.
- Loss of business opportunities.
- Inaccurate data insights.
Given its implications, reducing latency should be a priority for any organization leveraging big data streaming solutions.
Strategies for Reducing Latency
1. Optimize Network Configuration
A well-optimized network can significantly lower latency. Here are a couple of approaches:
- Use Quality of Service (QoS): Prioritize streaming data over less critical network traffic. This ensures timely delivery of important data streams.
- Deploy a Content Delivery Network (CDN): By caching data closer to users, you can lower the distance that data must travel, reducing latency.
// Example demonstrating QoS implementation
import java.net.*;
public class QosExample {
public static void main(String[] args) {
try {
DatagramSocket socket = new DatagramSocket();
socket.setTrafficClass(0x10); // Setting the IP type of service
byte[] buffer = "Hello, Stream!".getBytes();
InetAddress address = InetAddress.getByName("localhost");
DatagramPacket packet = new DatagramPacket(buffer, buffer.length, address, 9999);
socket.send(packet);
System.out.println("Packet sent with QoS.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
In the code snippet above, we create a DatagramSocket
and set the Traffic Class. This QoS implementation will prioritize the packet being sent in the network.
2. Use Efficient Data Serialization Formats
Serialization formats can greatly influence latency. Consider using more efficient formats for data serialization, such as Avro or ProtoBuf, which are designed for performance.
// Example showing Avro data serialization
import org.apache.avro.Schema;
import org.apache.avro.generic.GenericData;
import org.apache.avro.io.*;
import org.apache.avro.specific.SpecificDatumWriter;
public class AvroSerializationExample {
public static void main(String[] args) {
String schemaString = "{\"type\":\"record\",\"name\":\"User\",\"fields\":[{\"name\":\"name\",\"type\":\"string\"}]}";
Schema schema = new Schema.Parser().parse(schemaString);
GenericData.Record user = new GenericData.Record(schema);
user.put("name", "Alice");
try {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
EncoderFactory encoderFactory = EncoderFactory.get();
Encoder encoder = encoderFactory.binaryEncoder(outputStream, null);
SpecificDatumWriter<GenericData.Record> writer = new SpecificDatumWriter<>(schema);
writer.write(user, encoder);
encoder.flush();
System.out.println("User serialized successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this snippet, we use Apache Avro for efficient data serialization. Avro's compact binary encoding can lead to lower latency compared to JSON.
3. Minimize Buffering and Batch Processing Delays
Sometimes, developers buffer the data before processing it in bulk to improve throughput. However, large buffer sizes can introduce latency. Use smaller buffer sizes or implement a stream processing architecture instead.
// Example of using Apache Kafka for stream processing
import org.apache.kafka.clients.consumer.ConsumerConfig;
import org.apache.kafka.clients.consumer.KafkaConsumer;
import org.apache.kafka.clients.consumer.ConsumerRecords;
import org.apache.kafka.clients.consumer.ConsumerRecord;
import org.apache.kafka.common.serialization.StringDeserializer;
import java.time.Duration;
import java.util.Collections;
import java.util.Properties;
public class KafkaConsumerExample {
public static void main(String[] args) {
Properties properties = new Properties();
properties.put(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
properties.put(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
properties.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
properties.put(ConsumerConfig.GROUP_ID_CONFIG, "group1");
KafkaConsumer<String, String> consumer = new KafkaConsumer<>(properties);
consumer.subscribe(Collections.singletonList("stream-data"));
try {
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
System.out.println("Received: " + record.value());
}
}
} finally {
consumer.close();
}
}
}
Using Apache Kafka here allows for low-latency stream processing. Messages can be consumed in real-time without large buffers holding them back.
4. Choose the Right Processing Framework
Using frameworks designed for low-latency data processing can make a significant difference. Apache Kafka, Apache Flink, and Apache Storm are excellent choices when low latency is a priority.
- Apache Flink: Stream processing framework known for its low-latency capabilities.
- Apache Storm: Offers real-time computation and can handle massive data streams efficiently.
5. Implement Dynamic Scaling
Cloud-based solutions can benefit from dynamic scaling, allowing your system to respond to changes in data traffic. This ensures resources are allocated effectively based on current processing needs.
6. Monitor and Optimize Continuously
Monitoring your streaming solution's performance is key to identifying latency issues. Consider implementing APM (Application Performance Management) tools for real-time monitoring and analytics.
Closing the Chapter
Latency issues in big data streaming solutions can significantly impact business operations and decision-making. By understanding the causes of latency and implementing robust strategies such as optimizing network configurations, using efficient serialization, minimizing buffering, leveraging the right frameworks, dynamic scaling, and continuous monitoring, organizations can overcome these challenges and unlock the full potential of their big data initiatives.
For further reading on optimizing big data solutions, check out this detailed guide.
By adopting the right strategies and tools tailored to your unique requirements, you can ensure that your applications remain responsive and effective in today's data-driven world.
This post offers an in-depth discussion on latency issues in big data streaming solutions, coupled with practical Java code examples that illustrate the concepts discussed. Implementing these strategies can help organizations mitigate latency repercussions in their systems.