Common OAuth2 Token Issues and How to Fix Them
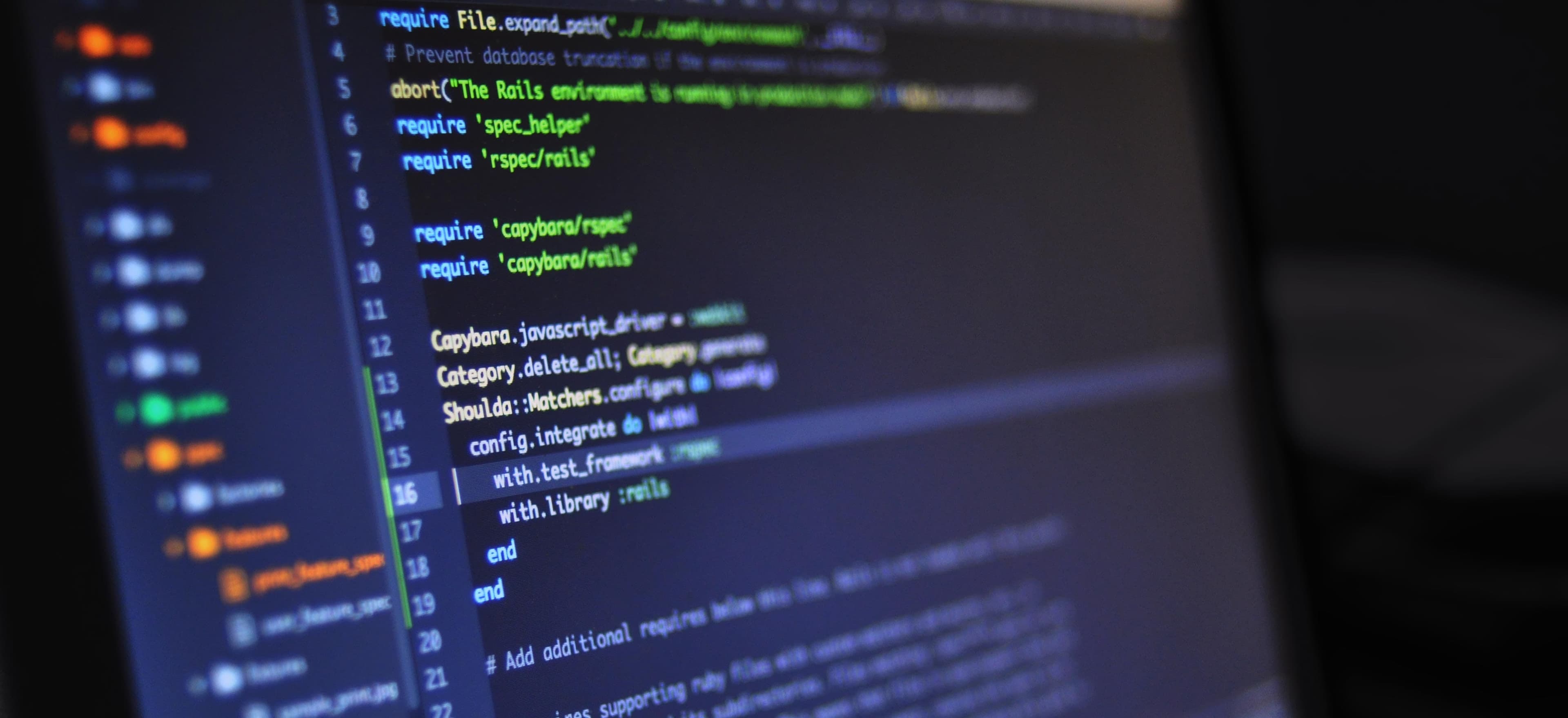
- Published on
Common OAuth2 Token Issues and How to Fix Them
OAuth2 is a widely adopted authorization framework that enables applications to obtain limited access to user accounts on an HTTP service, such as Facebook, Google, or GitHub. Despite its popularity, developers often encounter issues related to token management. Understanding these issues can save time and improve application security. In this blog post, we’ll explore common OAuth2 token issues and provide actionable solutions to overcome them.
Understanding OAuth2 Tokens
OAuth2 uses tokens to grant access permissions. There are primarily two types of tokens:
- Access Tokens: These are short-lived tokens that provide access to resources.
- Refresh Tokens: These are long-lived tokens used to obtain new access tokens without requiring user credentials.
Tokens are crucial in maintaining a seamless user experience and securing access to protected resources.
Key Components of OAuth2 Token Flow
Before diving into common issues, it’s important to understand the OAuth2 token flow. The process typically involves:
- Authorization Request: The client requests authorization from the resource owner.
- Authorization Grant: The resource owner grants or denies the request.
- Token Request: The client exchanges the authorization grant for an access token.
- Token Response: The resource server returns the access token, and optionally, a refresh token.
The above flow ensures that users only need to authenticate once, allowing for smoother interactions with applications.
Common OAuth2 Token Issues
1. Expired Tokens
Problem: Access tokens have a limited lifespan, typically ranging from a few minutes to a couple of hours. When an access token expires, the application cannot access protected resources, leading to failures.
Solution: Implement retry logic with refresh tokens to obtain a new access token when the current one expires. Here's a simple code sample to illustrate this:
public class TokenManager {
private String accessToken;
private String refreshToken;
public void accessResource() {
if (isTokenExpired(accessToken)) {
accessToken = refreshAccessToken(refreshToken);
}
// Proceed to call the resource with accessToken
}
private boolean isTokenExpired(String token) {
// Logic to check if the token is expired
return false;
}
private String refreshAccessToken(String refreshToken) {
// Logic to use refreshToken to get a new accessToken
return "new_access_token";
}
}
Why: The above implementation ensures that your application continues to have access to resources, even after token expiration. The accessResource
method checks token validity and refreshes as needed.
2. Invalid Token
Problem: Sometimes, the access token can be invalid due to incorrect generation, tampering, or premature expiration. This can result in authorization errors from the server.
Solution: Always validate tokens before using them and implement proper error handling for scenarios where the token is invalid.
public void useToken(String token) {
try {
if (!isTokenValid(token)) {
throw new InvalidTokenException("Token is invalid");
}
// Make API request using the token
} catch (InvalidTokenException e) {
// Handle the error (e.g., prompt user to log in again)
System.out.println(e.getMessage());
}
}
private boolean isTokenValid(String token) {
// Logic to verify token validity
return true; // Return false for invalid tokens
}
Why: Validating tokens prior to usage helps avoid unexpected errors and enhances user experience. This also provides a quick direction on what to do if the token is invalid.
3. Missing Scopes
Problem: Access tokens are created with specific scopes. If your application requests a resource outside the given scopes, access will be denied.
Solution: Ensure that the requested scopes encompass all required resources. You can define the necessary scopes upon requesting the token as follows:
String requestScopes = "read_user write_user";
String authorizationUrl = "https://authorization.server/auth?scope=" + requestScopes;
// Redirect the user to the authorization URL
Why: Properly defining scopes ensures that your application can access all necessary APIs without any hiccups. It’s essential for maintaining user security by limiting access to only what’s necessary.
4. Token Revocation Issues
Problem: Tokens can be revoked by users or administrators at any time. Attempting to use a revoked token can lead to access errors.
Solution: Keep track of token states and handle revocation gracefully. Ensure that you inform users accordingly when they try to use a revoked token.
public void checkTokenStatus(String token) {
if (isTokenRevoked(token)) {
// Inform the user their session has been revoked
System.out.println("Your session has been revoked. Please log in again.");
}
}
private boolean isTokenRevoked(String token) {
// Logic to check if the token has been revoked
return false;
}
Why: Handling token revocation correctly enhances the security of your application and provides a better user experience.
5. Not Handling Token Storage Properly
Problem: Poor token management can lead to token leakage, which poses a risk to user data and application integrity.
Solution: Store access tokens securely, such as in memory or utilizing secure storage solutions (like a secure cookie or local storage with encryption).
public class SecureTokenStorage {
private String encryptedAccessToken;
public void storeToken(String token) {
this.encryptedAccessToken = encryptToken(token);
}
public String retrieveToken() {
return decryptToken(encryptedAccessToken);
}
private String encryptToken(String token) {
// Encryption logic
return "encrypted_token";
}
private String decryptToken(String encryptedToken) {
// Decryption logic
return "decrypted_token";
}
}
Why: Securing token storage reduces the chance of unwanted access and ensures that sensitive information is not exposed.
Wrapping Up
Dealing with OAuth2 tokens can be challenging, but understanding common issues and implementing the provided solutions can enhance your application's stability and security. By following best practices in token management, developers can create a seamless and secure user experience.
For more in-depth understanding of OAuth2, consider visiting OAuth2 Simplified and What is OAuth? for additional insights. Always strive to stay updated with OAuth2 standards and recommendations to ensure the highest levels of security in your applications.