Why Java 8's Release Still Leaves IDEs in the Dark
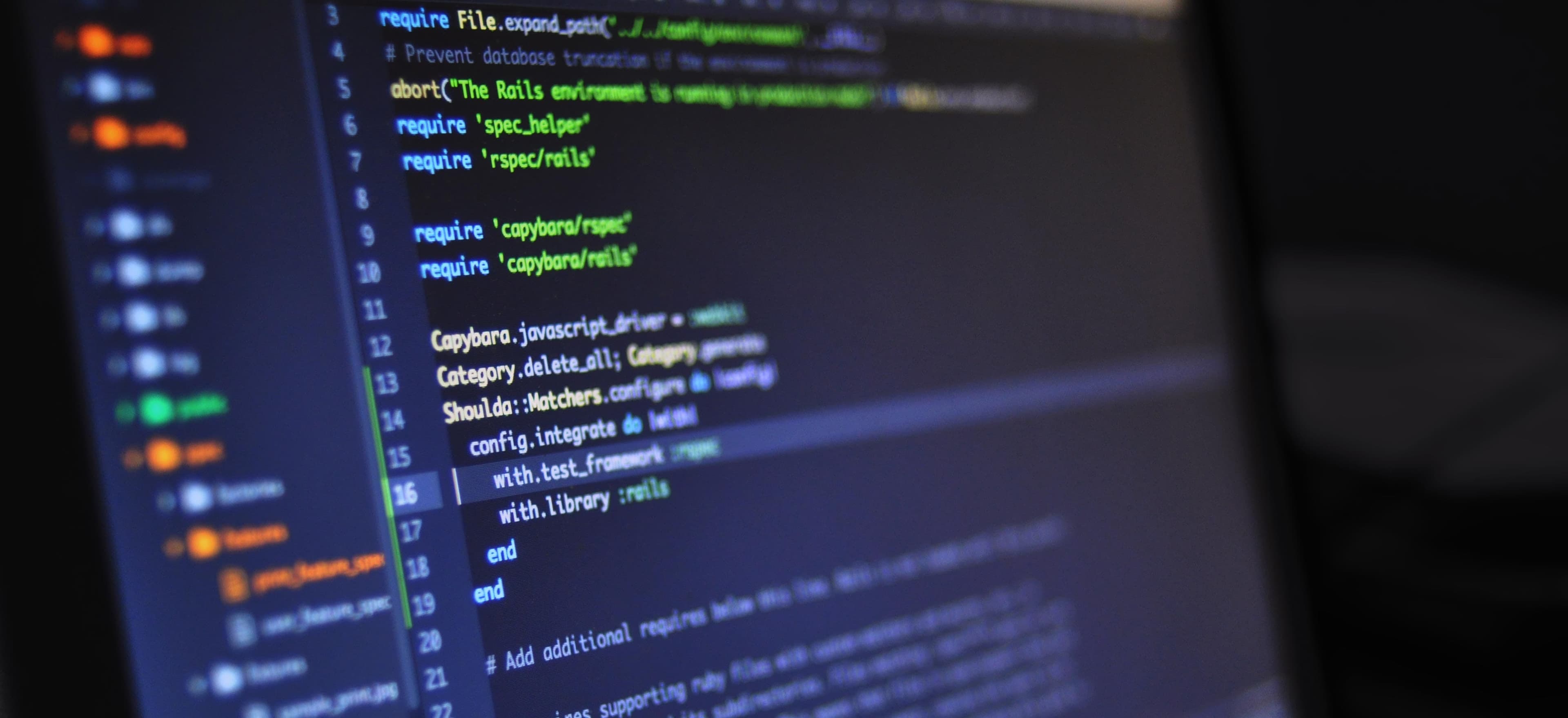
- Published on
Why Java 8's Release Still Leaves IDEs in the Dark
Java 8, released in March 2014, brought a slew of features that transformed the landscape of Java programming. Features like lambda expressions, the Stream API, and the new Date and Time API were revolutionary. However, despite its advancements, the complexities introduced can sometimes leave Integrated Development Environments (IDEs) struggling to keep up with best practices and features.
In this blog post, we will explore why some established IDEs might still find it challenging to support Java 8's functionalities adequately. We will discuss features that pose challenges and offer some recommendations on how developers can navigate these issues.
Understanding Java 8 Features
Java 8 introduced significant enhancements to the language, substantially changing the way developers write Java code. To understand the potential pitfalls, we should first look at the critical features:
1. Lambda Expressions
Lambda expressions allow you to express instances of single-method interfaces (functional interfaces) more concisely. Here's a simple example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
Why: Instead of using an anonymous inner class for the forEach
method, you can now write cleaner, more readable code using lambda syntax. However, many IDEs were not initially equipped to provide autocompletion and type inference for lambda expressions.
2. The Stream API
The Stream API allows for functional-style operations on collections. With streams, you can express complex data manipulations in a declarative manner. Here's how easy it can be to filter a list:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Why: While the code is straightforward, the underlying implementation often makes IDEs work overtime to understand and display the types correctly, especially when combining filtering, mapping, and reducing.
3. New Date and Time API
Java 8 introduced a new Date and Time API that is significantly more sophisticated than the old java.util.Date
and java.util.Calendar
. The new API addresses many long-standing issues with date-time manipulation and offers an intuitive approach.
LocalDate today = LocalDate.now();
LocalDate tomorrow = today.plusDays(1);
Why: Although the new API simplifies date management, IDEs need to adapt their libraries and features to fully support operations like time zone conversions correctly.
IDEs Facing Challenges
Despite the exciting enhancements in Java 8, many IDEs initially struggled with providing adequate support for these features. Here are some potential reasons:
1. Increased Complexity
The introduction of lambda expressions and the Stream API resulted in a more functional programming style. Many established IDEs, which traditionally catered to an object-oriented programming paradigm, faced challenges in adapting their features to effectively support functional programming constructs.
2. Autocompletion and Type Inference
IDE features like autocompletion and type inference depend massively on the static analysis of code. The dynamic nature of lambda expressions and the Stream API sometimes led to incorrect type suggestions or failures to recognize certain constructs.
3. Documentation and Resources
Although Java 8 documentation was released with clear guidelines, not all IDEs were quick to integrate this information into their interfaces. This left developers grappling with how to effectively utilize new features without adequate IDE support.
Best Practices for Working with Java 8 in IDEs
While the challenges are clear, there are some best practices developers can adopt to maximize their efficiency while coding in Java 8.
1. Stay Updated
Ensure that you are using the latest version of your IDE. Development teams often release updates that include enhancements for newer Java features. Popular IDEs like IntelliJ IDEA and Eclipse frequently update their support for new Java versions.
2. Utilize Plugins
Enable or install plugins that may enhance your IDE's support for Java 8 features. For instance, plugins can improve autocompletion for lambda expressions or offer templates that streamline coding practices.
3. Leverage External Tools
Sometimes, external tools like Sonarqube or Checkstyle can help enforce coding standards and best practices. These tools can supplement an IDE's built-in capabilities, especially in environments where the IDE falls short in understanding lambda expressions or the Stream API.
4. Use Alternative IDEs
Consider trying out IDEs explicitly built with modern language constructs in mind. For instance, Eclipse has continued to improve its functionality, and IntelliJ IDEA quickly adapted to the changes introduced in Java 8, offering robust support.
Wrapping Up
Java 8 has undeniably advanced the Java programming landscape with features that champion functional programming. However, the complexity introduced by these features can leave some IDEs struggling to maintain adequate support.
By understanding both the enhancements and the challenges posed by Java 8, developers can navigate the hurdles effectively. Staying updated, utilizing plugins, leveraging external tools, and, if necessary, exploring alternative IDEs can optimize the Java development experience.
Although there are lingering issues for some IDEs, those willing to adapt will find that Java 8 significantly enhances their programming capabilities.
For further reading on Java 8's features, check out Oracle’s official documentation and consider learning more about functional programming in Java via Baeldung’s Java Functional Programming Guide.
By leveraging the right strategies, you can ensure a productive and fulfilling programming experience in Java 8, despite the challenges that may arise. Happy coding!