Troubleshooting Active Hotspot Issues in Garbage Collection
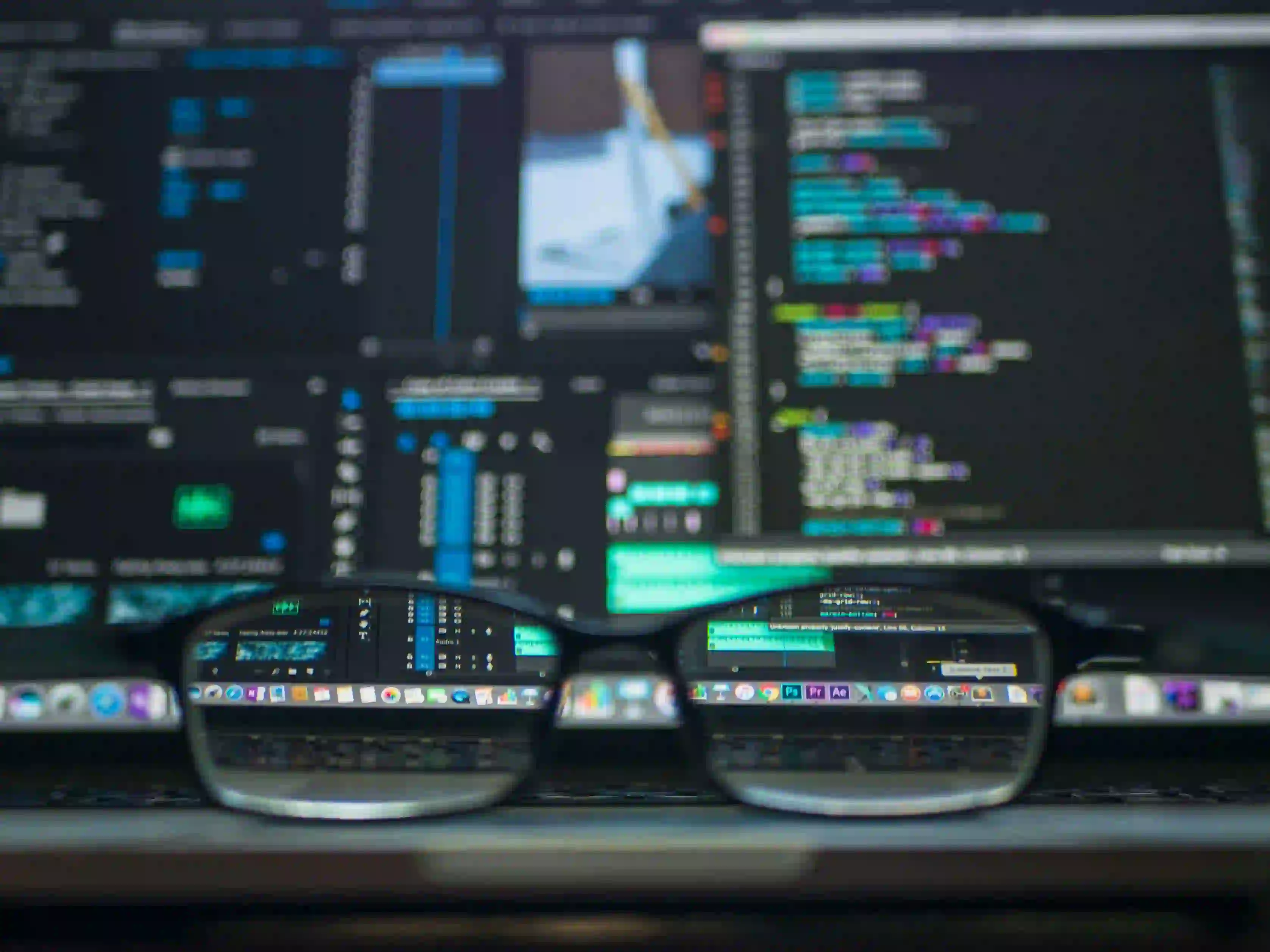
Troubleshooting Active Hotspot Issues in Garbage Collection
Java's Garbage Collection (GC) mechanism is a cornerstone of its memory management, allowing developers to concentrate on writing code rather than worrying about memory leaks and manual memory management. However, even the most robust systems encounter pitfalls, particularly when faced with Active Hotspot issues during Garbage Collection.
In this post, we will delve into what Active Hotspot issues are, how they can affect the performance of Java applications, and strategies for troubleshooting these problems. We will provide code snippets with explanations to illuminate the subject further, ensuring that you gain a comprehensive understanding.
Understanding Active Hotspot Issues
An Active Hotspot occurs when the Garbage Collector is spending an excessive amount of time managing memory for certain long-lived objects or heavily accessed data. This can lead to performance degradation, causing applications to exhibit high latency or even timeouts.
Hotspots can occur for various reasons:
- Incorrect GC Strategy: Not choosing the right Garbage Collector for your application's needs.
- Memory Leaks: Excessive retention of objects that should otherwise be garbage collected.
- Insufficient Heap Space: Not providing enough memory to accommodate your application's workload.
Before diving into troubleshooting, it's essential to understand how Java’s Garbage Collection works and the variety of collectors available, such as G1, CMS (Concurrent Mark-Sweep), and Parallel GC.
Basic Troubleshooting Approach
-
Observe GC Logs: Start analyzing Garbage Collection logs. GC logging provides essential insights about memory usage, collection times, and the frequency of collections.
-
Heap Dump Analysis: Utilize heap dumps to inspect living objects in memory. This helps identify retained objects that contribute to hotspots.
-
Profiling and Monitoring: Use tools like VisualVM or JConsole to monitor application performance live.
Enabling GC Logging
To enable GC logging, you can add the following JVM parameters when starting your application:
-XX:+PrintGCDetails
-XX:+PrintGCDateStamps
-Xloggc:<file_path>
Code Snippet: Sample GC Logging
Here’s a simple example that illustrates enabling GC logging:
public class GCDemo {
public static void main(String[] args) {
System.out.println("Java Garbage Collection Demo");
for (int i = 0; i < 100000; i++) {
String str = new String("Active Hotspot Issue " + i);
}
}
}
In this code, we are flooding the heap with Strings. You can monitor how often garbage collection occurs by examining the GC log file generated by the parameters above.
Why This Matters
By enabling GC logging, you'll gather insights critical for troubleshooting. You'll notice patterns such as Full GCs typically pointing to larger datasets or memory leaks.
Identifying Hotspots
Once you have enabled logging, the next step is to identify hotspots.
-
Look at GC Pause Times: Longer pauses can indicate that your application may be struggling with memory management.
-
Monitor Object Allocation: Use heap analysis tools to observe the allocation rates.
Analyzing Heap Dumps
To analyze heap dumps, use the jmap utility to generate a heap dump:
jmap -dump:live,format=b,file=<file_path>.hprof <pid>
You can open the generated .hprof file in tools like Eclipse Memory Analyzer (MAT) to identify memory leaks and objects retained in memory.
Code Snippet: Generating a Heap Dump
Here’s a simple method I’ve implemented to create a heap dump programmatically:
import java.lang.management.ManagementFactory;
import java.lang.management.MemoryMXBean;
import java.lang.management.MemoryPoolMXBean;
public class MemoryMonitor {
public static void main(String[] args) {
createHeapDump();
}
public static void createHeapDump() {
MemoryMXBean mxBean = ManagementFactory.getMemoryMXBean();
for (MemoryPoolMXBean pool : ManagementFactory.getMemoryPoolMXBeans()) {
System.out.println("Memory Pool: " + pool.getName() + " - Usage: " + pool.getUsage());
}
}
}
Why Analyze Heap Dumps?
Heap dump analysis helps in isolating cords of memory leaks and identifies which object types are taking up most of the heap. It lets you pinpoint what could potentially create Active Hotspots in your application.
Optimizing the Garbage Collector
Depending on what you've discovered, optimizing the GC settings or even refactoring portions of your code may be necessary. Below are some common GC tuning parameters that you can tweak:
- Heap Size:
-Xms
and-Xmx
- Garbage Collector Choice:
-XX:+UseG1GC
,-XX:+UseParallelGC
- Adjusting Size of Young Generation:
-XX:NewSize
and-XX:MaxNewSize
Example: Tuning G1 Garbage Collector
If you find that your application is delayed by too many Minor GCs, consider configuring G1 with larger young generation sizes:
-XX:NewRatio=3 -XX:MaxGCPauseMillis=200 -XX:G1HeapRegionSize=16M
Why Optimize?
Adjusting these parameters may lead to reduced pause times, improving overall application performance and responsiveness.
Closing the Chapter
Garbage Collection in Java is a powerful feature that, when used appropriately, can elevate application performance. However, when Active Hotspot issues arise, troubleshooting becomes essential to maintain performance.
Start by enabling GC logging, analyzing heap dumps, and monitoring your application. From there, identify hotspots and optimize your garbage collector settings.
For a deeper dive into Garbage Collector strategies, check out the official Oracle documentation on Java Garbage Collection and consider examining a tutorial on Java Memory Management for practical examples.
Arming yourself with the knowledge and tools to manage GC effectively, you can keep your Java applications running smoothly. Happy coding!