Mastering Spring RESTTemplate: Super Type Tokens Simplified
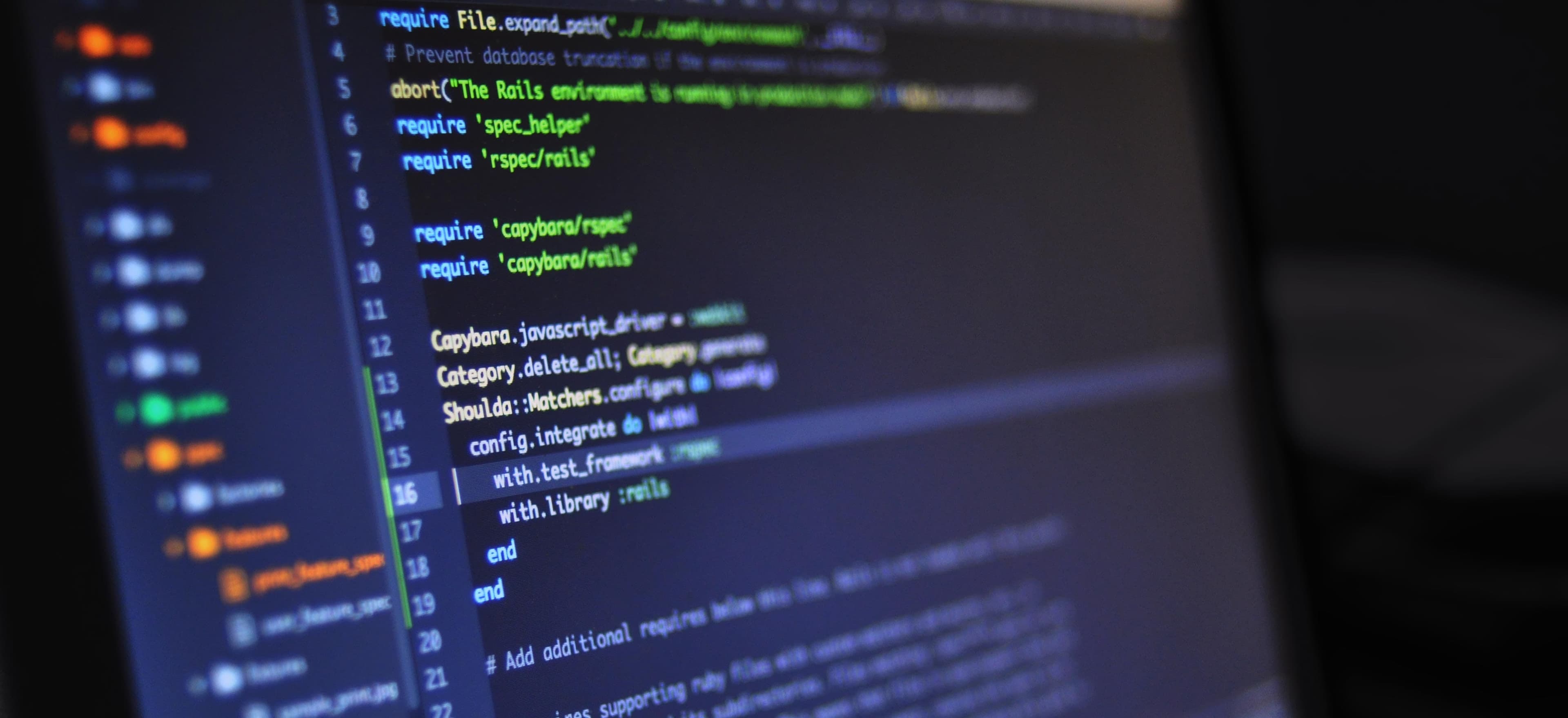
- Published on
Mastering Spring RESTTemplate: Super Type Tokens Simplified
Spring's RESTTemplate is a powerful tool for consuming RESTful services. While it simplifies communication between applications, developers often face challenges when working with complex data structures, particularly those involving generic types. In this blog post, we will dive deep into super type tokens and how they facilitate working with generics in Spring's RESTTemplate.
What is Spring RESTTemplate?
Spring’s RESTTemplate is part of the Spring Framework, designed for easy interaction with RESTful web services. It simplifies many tasks by providing high-level methods to perform HTTP requests and responses. From GET to POST, and even error handling, it provides built-in support for these actions.
Example Usage of RESTTemplate
Here’s a basic example of using RESTTemplate to perform a GET request:
import org.springframework.web.client.RestTemplate;
import org.springframework.http.ResponseEntity;
public class RestTemplateExample {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
String url = "https://api.example.com/data";
ResponseEntity<String> responseEntity = restTemplate.getForEntity(url, String.class);
String responseData = responseEntity.getBody();
System.out.println(responseData);
}
}
This snippet showcases a straightforward application that fetches data from an API. However, as we delve deeper into the world of APIs, we often encounter more complex data structures, such as lists or maps of custom types.
Understanding Super Type Tokens
One of the biggest challenges in using RESTTemplate arises when dealing with generics. For example, let’s say we want to fetch a list of objects from a REST API, where the objects are of a certain type, say User
. The method signature we might want to use looks something like this:
List<User> users = restTemplate.exchange(url, HttpMethod.GET, null,
new ParameterizedTypeReference<List<User>>() {}).getBody();
Why Do We Need Super Type Tokens?
In Java, type erasure at runtime means that generics information is not available. This can lead to challenges when performing operations with complex types, as type information is lost. Super type tokens provide a way to preserve this type information across method calls.
The ParameterizedTypeReference
class acts as a holder for the type information. It maintains the specific type, overcoming the limitations imposed by type erasure.
Implementing Super Type Tokens in RESTTemplate
Let me demonstrate a practical approach to using super type tokens with RESTTemplate.
Step 1: Define Your Domain Class
First, let's define a User
class:
public class User {
private int id;
private String name;
// Getters and Setters
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
This User
class has two properties: id
and name
. With this defined, we can now retrieve a list of User
objects from the API.
Step 2: Making the API Call Using Super Type Tokens
Now, let’s see how to retrieve a list of users from a RESTful service:
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.http.HttpMethod;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
import java.util.List;
public class UserService {
private final RestTemplate restTemplate;
public UserService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
public List<User> getAllUsers(String url) {
ResponseEntity<List<User>> responseEntity = restTemplate.exchange(
url,
HttpMethod.GET,
null,
new ParameterizedTypeReference<List<User>>() {}
);
return responseEntity.getBody();
}
}
Breakdown of the Code
- RestTemplate: Our main tool for making web requests.
- ParameterizedTypeReference: We utilize this to retain type information about the returned list of
User
. - ResponseEntity: This encapsulates the response, including the HTTP status and headers, along with the body.
Step 3: Invoking our Service
To utilize this service, one would write:
public class Application {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
UserService userService = new UserService(restTemplate);
String url = "https://api.example.com/users";
List<User> users = userService.getAllUsers(url);
users.forEach(user -> System.out.println(user.getName()));
}
}
Error Handling with RESTTemplate
When working with REST calls, you should anticipate potential errors. Here’s how to enhance the initial example to include error handling using try-catch
:
public List<User> getAllUsers(String url) {
try {
ResponseEntity<List<User>> responseEntity = restTemplate.exchange(
url,
HttpMethod.GET,
null,
new ParameterizedTypeReference<List<User>>() {}
);
return responseEntity.getBody();
} catch (HttpClientErrorException e) {
System.out.println("Error occurred: " + e.getStatusCode());
return Collections.emptyList();
}
}
Why Implement Error Handling?
Implementing robust error handling is vital in production applications to prevent crashes and to allow for graceful degradation of service. Having a fallback approach, like returning an empty list, assures that functions that depend on this method can proceed without interruption.
Bringing It All Together
In this article, we explored the power of Spring’s RESTTemplate and learned about super type tokens. By utilizing ParameterizedTypeReference
, you can effectively manage generics and retain type safety when consuming complex JSON APIs.
For further exploration, consider diving into Spring's official documentation on RESTTemplate or exploring JSON serialization with Jackson to see how it integrates with RESTTemplate. Understanding these concepts will not only enhance your RESTful service consumption skills but will also bolster your Java development toolkit.
By mastering such subtleties in frameworks, developers can become more efficient in creating reliable and scalable applications. Remember, the journey of learning is ongoing, so embrace every opportunity to expand your understanding of Java, Spring, and RESTful services!