Micronaut REST APIs: Tackling Slow Startup Issues
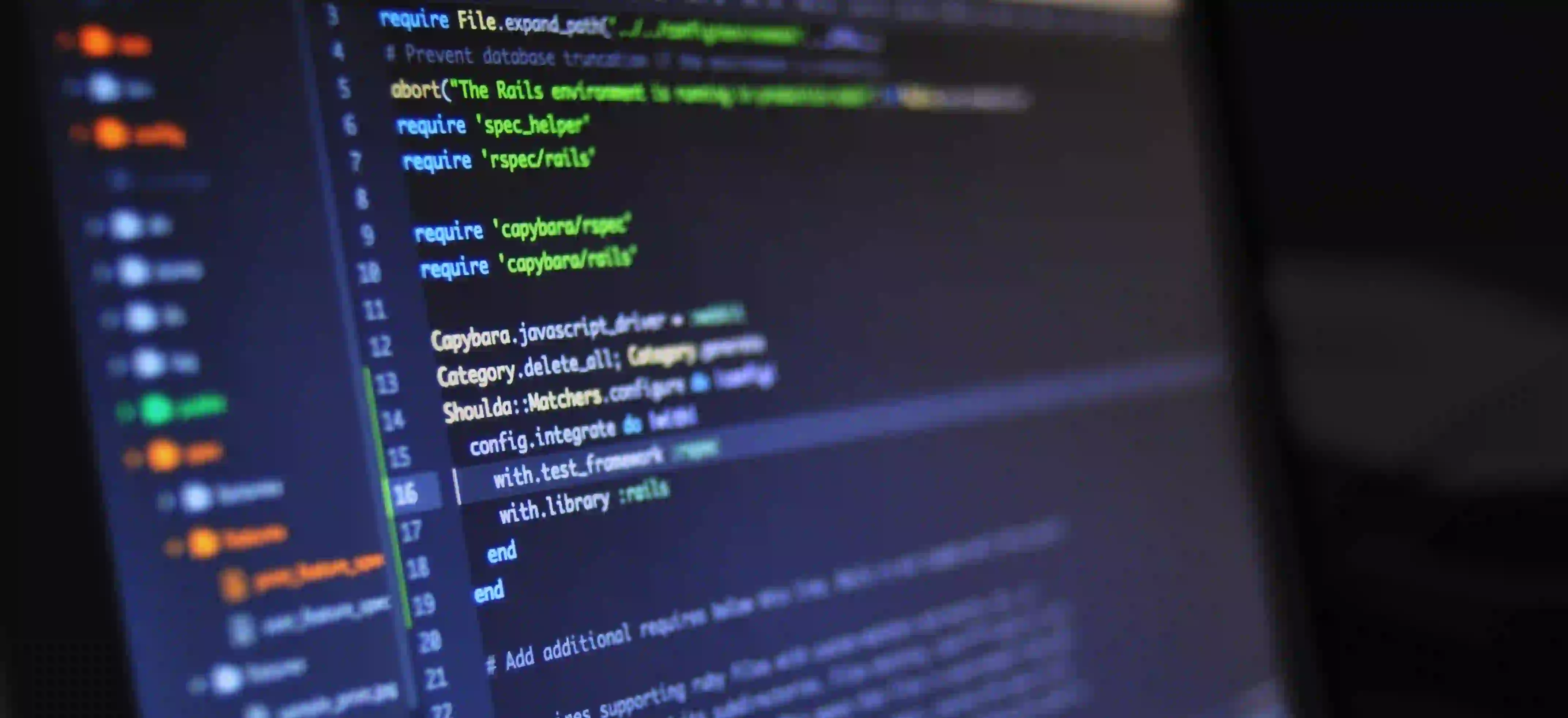
Micronaut REST APIs: Tackling Slow Startup Issues
Micronaut is a modern framework designed for building lightweight and high-performance applications, specifically optimized for microservices. One of its primary advantages is the minimal startup time compared to traditional frameworks like Spring Boot. However, you might still encounter slow startup issues under certain circumstances. In this blog post, we will explore common reasons for slow startup, identify potential bottlenecks, and provide solutions to enhance startup performance.
Understanding Micronaut
Micronaut is a JVM-based framework that utilizes compile-time dependency injection and AOT (Ahead of Time) compilation, allowing for faster startup times and lower memory consumption. It is particularly suited for microservices and serverless applications where resources are often constrained. For more details on Micronaut framework features, visit the Micronaut official documentation.
Why is Startup Time Important?
Measuring startup time is crucial for cloud-native applications, especially those running in serverless environments. A slow startup can lead to increased latency, higher costs, and in multi-tier architectures, this can affect the entire system’s performance. Optimizing startup time not only improves user experience but also reduces operational costs.
Common Causes of Slow Startup
Before diving into solutions, let’s identify some common factors that might contribute to slow startup times in a Micronaut application:
- Heavy Dependency Injection: If your application is utilizing a large number of dependencies, it can lead to increased initialization time.
- Large Codebase: A huge codebase, especially with excessive reflection or runtime processing, can hinder startup speed.
- Configuration Loading: Misconfigured YAML or properties files may lead to unnecessary overhead during application startup.
- Database Connections: Establishing connections to databases or external services can add significant latency.
Solutions to Improve Startup Time
Let’s go through some strategies to tackle slow startup issues in your Micronaut REST APIs:
1. Reduce Dependency Injection Overhead
Micronaut provides compile-time dependency injection, which is generally faster than runtime injection. However, if you’re still encountering slow startup times, consider the following:
- Minimize Transitive Dependencies: Regularly review and reduce unnecessary dependencies in your
build.gradle
orpom.xml
files. If you can avoid dependencies that significantly increase startup time, do so.
Example: Gradle File with Reduced Dependencies
dependencies {
implementation("io.micronaut:micronaut-runtime")
implementation("io.micronaut.http:micronaut-http-client")
// Remove unused dependencies
// implementation("io.micronaut.data:micronaut-data-hibernate-jpa") // Uncomment if really needed
}
2. Leverage the @Singleton
Annotation
Using @Singleton
for beans can ensure that these components are created only once, reducing the overall initialization overhead.
Code Example:
import jakarta.inject.Singleton;
@Singleton
public class CacheService {
// Cache initialization logic here
public void cacheData() {
// Cache data handling
}
}
In the example above, CacheService
is created just once, which means subsequent calls do not incur the cost of its instantiation.
3. Lazy Initialization
Utilizing lazy initialization can also reduce startup time. Micronaut supports async initialization through the @Produces
and @Singleton
annotations.
Code Example:
import jakarta.inject.Singleton;
import jakarta.inject.Provider;
@Singleton
public class UserService {
private final Provider<DatabaseConnection> dbConnectionProvider;
public UserService(Provider<DatabaseConnection> dbConnectionProvider) {
this.dbConnectionProvider = dbConnectionProvider;
}
public void performAction() {
DatabaseConnection connection = dbConnectionProvider.get(); // Retrieved when needed.
// Perform actions with the connection
}
}
In this example, DatabaseConnection
will only be instantiated when performAction()
is called, making startup faster.
4. Profile Your Application Startup
Micronaut offers built-in support for application profiling. Using the Micronaut Launch or Gradle, you can evaluate which beans are taking the most time to initialize.
Command Example:
./gradlew run -Dmicronaut.profiles=dev
After profiling, look for packages or classes that are taking longer than expected to load, and strategically refactor them.
5. Optimize Configuration Files
Ensure your application.yml
or application.properties
files are lightweight. Heavy and convoluted configuration files can lead to longer parsing times during startup.
Example:
Keep it simple:
micronaut:
application:
name: MyService
server:
port: 8080
6. Use Native Compilation
Micronaut supports native compilation using GraalVM, which can significantly speed up startup times due to ahead-of-time compilation.
Example:
To compile your application to a native image:
./gradlew nativeCompile
Ensure you have GraalVM installed and properly set up in your environment. This approach also optimizes memory usage, making it even more efficient for microservices.
7. Increase Kubernetes Pod Readiness Time
If you are deploying your Micronaut application on Kubernetes, be sure to set appropriate readiness and liveness probes. A higher timeout setting can prevent premature failures.
readinessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 5
periodSeconds: 10
Wrapping Up
Optimizing startup times in your Micronaut REST APIs involves a combination of reducing dependency overhead, optimizing configuration files, and wisely managing bean initialization. By following the strategies outlined above, you can ensure a more efficient and responsive application, ultimately leading to improved user experiences and cost savings.
For more information, delve into the official Micronaut documentation and consider contributing to Micronaut’s community for shared insights and updates on best practices.
By embracing these optimization techniques, you not only enhance performance but also position your application for success in a competitive landscape where speed and efficiency are critical. Happy coding!