Mastering Date Handling in Groovy: Common Pitfalls Explained
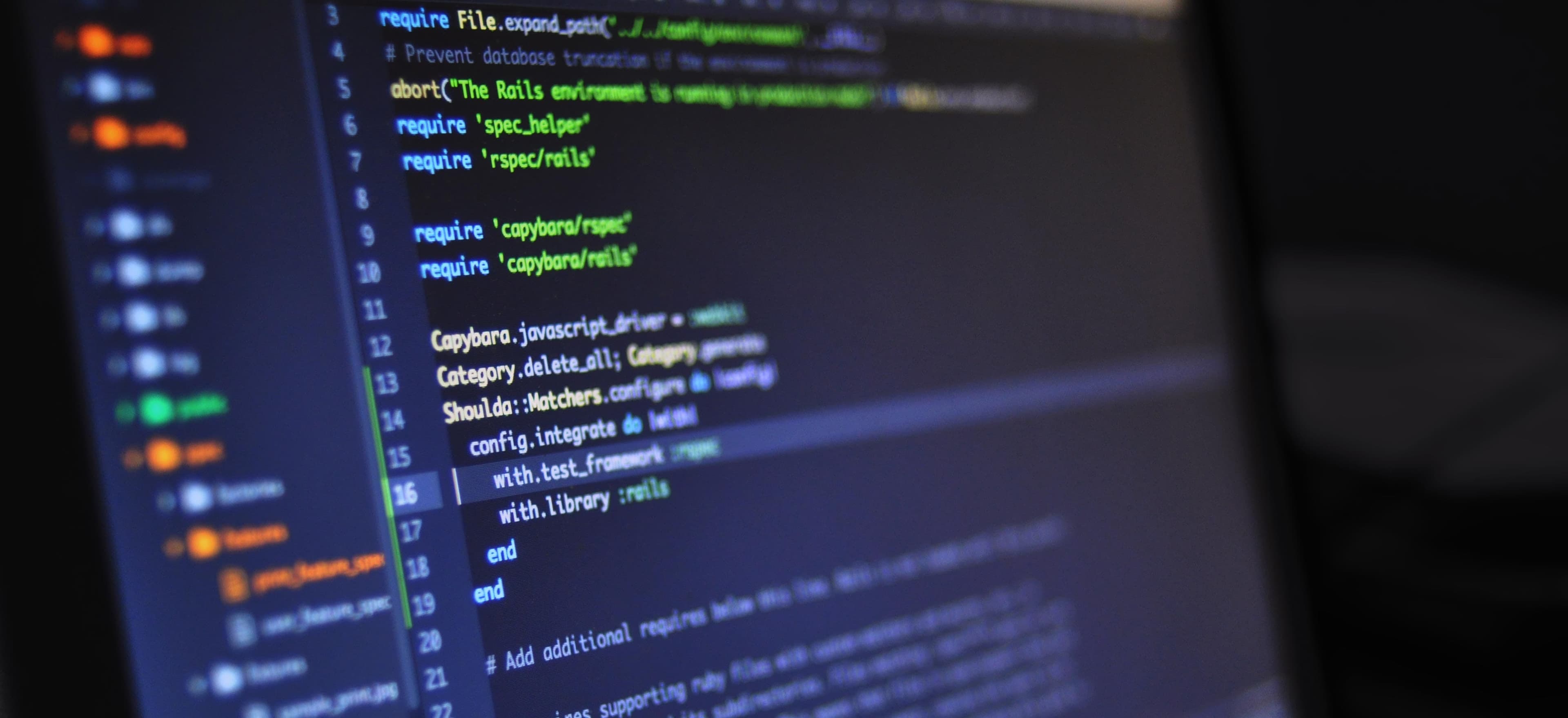
- Published on
Mastering Date Handling in Groovy: Common Pitfalls Explained
Date handling can often be a stumbling block for many developers, especially when it comes to using scripting languages like Groovy. With its dynamic nature and integration with the Java ecosystem, Groovy offers powerful features for handling date and time. However, this flexibility can also lead to common pitfalls that might trip up even seasoned developers.
In this blog post, we will explore Groovy's date handling capabilities, discuss common pitfalls, and provide best practices to avoid them. Let's dig in!
Understanding Groovy's Date Handling
Groovy extends the native java.util.Date
class and provides several methods to manipulate dates and times seamlessly. It also integrates well with Java's java.time
package introduced in Java 8, offering even more robust functionalities.
Creating Date Instances
Creating date instances in Groovy is straightforward:
// Creating a date instance for the current date and time
def currentDate = new Date()
println "Current Date: ${currentDate}"
// Creating a specific date
def specificDate = Date.parse("yyyy-MM-dd", "2023-10-01")
println "Specific Date: ${specificDate}"
Why Use Date.parse
?
The Date.parse
method allows you to create a date object from a string representation easily. This is very helpful when dealing with data coming from external sources, such as databases or user inputs.
Common Pitfalls in Date Handling
1. Using Default Time Zones
One common pitfall is not accounting for time zones. If your application deals with users across different locations, you need to ensure that times are consistent.
def date = new Date() // Uses the default time zone
println "Local date: ${date.format('yyyy-MM-dd HH:mm:ss Z')}"
Solution: Specify Time Zones
You can specify a specific time zone when creating or manipulating dates:
import java.util.TimeZone
def tz = TimeZone.getTimeZone("UTC")
def dateInUtc = new Date().format('yyyy-MM-dd HH:mm:ss Z', tz)
println "UTC Date: ${dateInUtc}"
2. Date Comparison Issues
When comparing dates, developers sometimes inadvertently compare date instances directly, leading to unexpected results.
def date1 = Date.parse("yyyy-MM-dd", "2021-09-01")
def date2 = Date.parse("yyyy-MM-dd", "2021-09-02")
if (date1 < date2) {
println "date1 is before date2"
}
Why is This Problematic?
This works for direct comparisons, but it might lead to unexpected results when considering time components. Always ensure you are focusing on the proper granularity (date vs. date-time).
Better Approach with compareTo
:
if (date1.compareTo(date2) < 0) {
println "date1 is before date2"
}
3. Misunderstanding of Leap Years
Another common mistake is failing to account for leap years when performing date arithmetic.
def leapYearDate = Date.parse("yyyy-MM-dd", "2020-02-29")
println "Leap Year Date: ${leapYearDate}"
What to Keep in Mind?
Always validate your date logic when adding days or months that might land on February 29th. In Groovy, the Date class handles leap years effectively, but your application logic should be aware of these nuances.
4. Formatting Dates Incorrectly
Formatting dates for display can also be a source of confusion.
def today = new Date()
def formattedDate = today.format("MM/DD/YYYY")
println "Formatted Date: ${formattedDate}"
The Issue?
Using the incorrect date format can lead to ambiguous outputs. The above code may not behave as expected because "YYYY" represents the week year, not the calendar year.
Correct Format Approach:
def correctFormattedDate = today.format("MM/dd/yyyy") // Use lowercase 'yyyy'
println "Correctly Formatted Date: ${correctFormattedDate}"
5. Parsing Issues with Locale
When parsing dates from strings, failing to account for locale can lead to failures or incorrect date objects.
def dateString = "03/10/2023"
def parsedDate = Date.parse("MM/dd/yyyy", dateString)
println "Parsed Date: ${parsedDate}"
Potential Pitfall
If the format is not aligned with the system's locale, you might get unexpected results.
Solution: Specify Locale Explicitly
If you are sure of the date format, it's better to manage the locale programmatically when necessary:
import java.text.SimpleDateFormat
import java.util.Locale
def formatter = new SimpleDateFormat("dd/MM/yyyy", Locale.US)
def date = formatter.parse("10/03/2023")
println "Locale-aware Parsed Date: ${date}"
Best Practices for Date Handling in Groovy
-
Always Use Time Zones Explicitly: When working with dates, always set and be aware of the time zones you are dealing with.
-
Utilize
java.time
: If you're using Java 8 or later, consider using thejava.time
classes, such asLocalDate
,LocalDateTime
, andZonedDateTime
, for clearer code. -
Test Edge Cases: Always test leap years, daylight saving time changes, and year boundaries to ensure your date logic holds under various scenarios.
-
Format with Care: When formatting dates, ensure that you are using the appropriate format patterns and be mindful of the locale to avoid ambiguities.
-
Use Libraries When Necessary: For complex date operations, consider using libraries like Joda-Time or the new
java.time
package to simplify your code.
Bringing It All Together
Mastering date handling in Groovy requires attention to detail and understanding the pitfalls that can arise. By being aware of common challenges and implementing best practices, developers can create robust applications that handle dates and times seamlessly.
For additional reading on date handling in Java and Groovy, consider checking the following resources:
By avoiding the common pitfalls discussed in this article, you’ll save time and headaches down the road. Happy coding!
Checkout our other articles