Common Pitfalls When Starting with Apache Thrift in Java
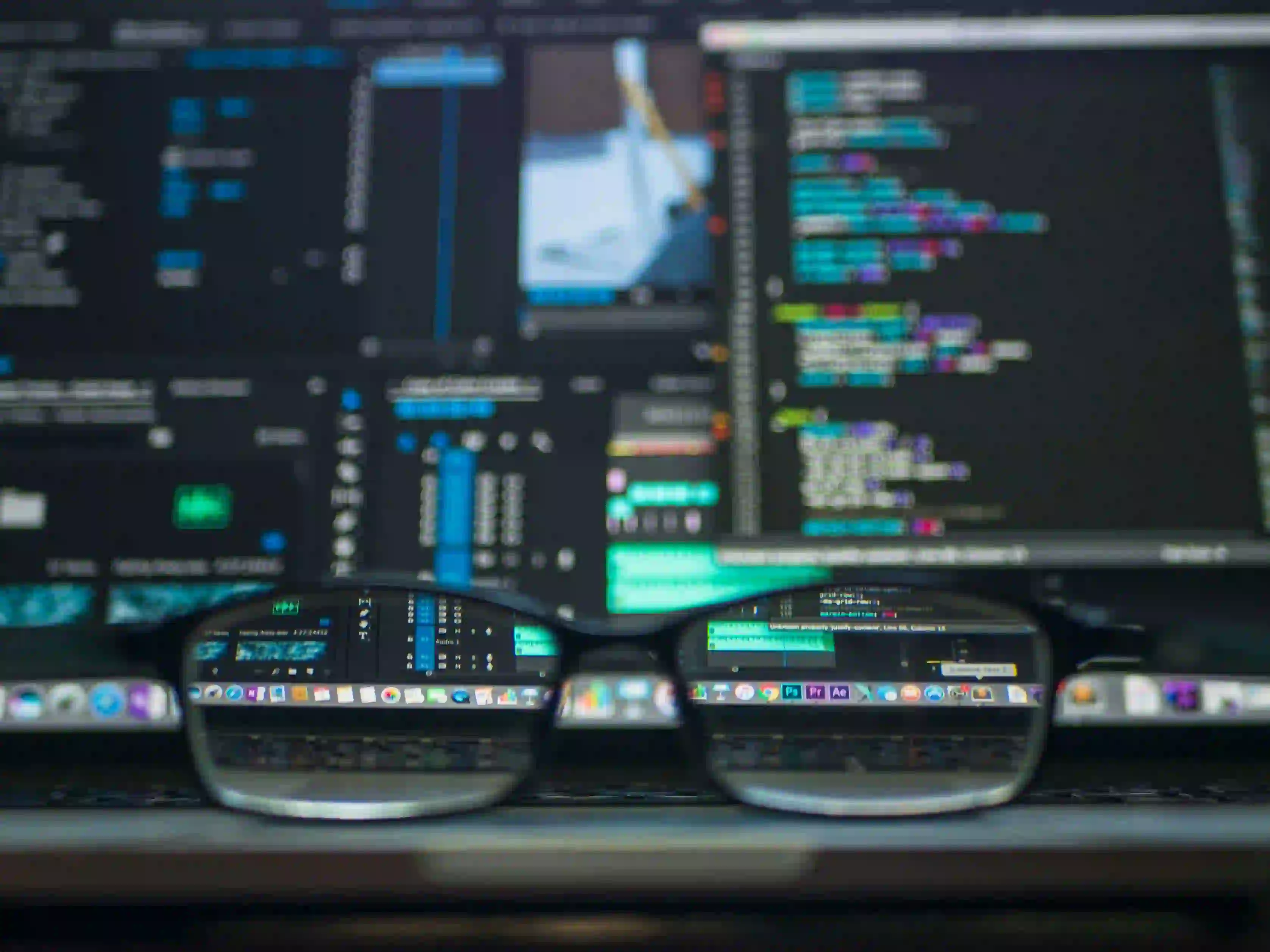
Common Pitfalls When Starting with Apache Thrift in Java
Apache Thrift is a powerful framework designed for scalable cross-language services development. If you're new to Thrift and Java, diving in can be a bit daunting. While it provides robust tools for communication between different programming languages, a few common pitfalls can hinder the development process. In this blog post, we’ll identify these pitfalls and provide you with strategies to avoid them, ensuring a smooth journey into using Apache Thrift with Java.
What is Apache Thrift?
Before delving into the pitfalls, let’s clarify what Apache Thrift is. Apache Thrift is a framework that allows you to define data types and service interfaces in a simple definition file. It compiles this file to generate the necessary code, allowing for easy communication between various programming languages like Java, C++, Python, and more. The complexity of Thrift lies in its configuration and usage, so understanding these pitfalls can help streamline your development experience.
Common Pitfalls
1. Not Understanding the Thrift Data Model
One of the first pitfalls to avoid is not thoroughly understanding how the Thrift data model works. Thrift uses a specific way to define data types, including structures, enums, and exceptions. Not comprehending these can lead to unexpected results in your program.
Example
// Define a simple user structure
struct User {
1: i32 id,
2: string name,
3: string email
}
The code above defines a basic User
struct. If you were to omit any field or misinterpret the type, it could result in runtime errors. Make it a point to read the Thrift documentation thoroughly to understand how to use these data models effectively.
2. Misconfiguring Thrift Services
Another shortcoming often encountered is the misconfiguration of Thrift services. The service configuration dictates how different components interact. When starting, you might overlook aspects such as server types, transport protocols, or serialization methods, leading to inefficient communication.
Configuration Example
// Define a simple service
service UserService {
User getUser(1: i32 id),
void createUser(1: User user)
}
This Thrift service allows you to retrieve and create users. To ensure smooth operations, you should utilize the TNonblockingServer
for async calls effectively. An incorrect server configuration could block service calls or affect performance negatively.
3. Ignoring Cross-language Compatibility
Thrift’s key feature is its cross-language support. A common pitfall is to assume that a service will behave the same way in every language. This assumption can lead to mismatches in data handling and types, producing bugs that are difficult to trace.
When communicating between languages, always check the generated code for conformity. For instance, int
in Java corresponds to i32
in Thrift, but in C++, it might have different limitations.
To mitigate this, always test the service in multiple languages after defining it.
4. Skipping Extensive Error Handling
Error handling is vital in any distributed system, yet developers often underestimate its importance in Thrift applications. Every thrift service has the potential to throw exceptions. If not managed correctly, these exceptions can propagate and lead to system failures.
Exception Handling Example
public User getUser(int id) throws UserNotFoundException {
User user = userRepository.find(id);
if (user == null) {
throw new UserNotFoundException("User not found for ID: " + id);
}
return user;
}
In the example above, effective error handling was put in place. Not handling exceptions gracefully can lead to service failure and complicate debugging.
For comprehensive guidelines on error handling, check out the Apache Thrift Error Handling documentation.
5. Neglecting Version Management
When using Thrift, especially in a team setting, managing versioning of your service definitions is crucial. A change in the service definition might not be compatible with existing clients, leading to hard-to-debug failures.
To handle this keep a clear versioning strategy. Using semantic versioning for service definitions can help:
- Major changes break compatibility.
- Minor changes add functionality in a compatible manner.
- Patch versions bug fixes.
6. Not Utilizing Code Generation Features
Thrift provides powerful code generation features. Failing to leverage these can lead to redundant code and wasted effort. You should always generate code from your Thrift files to maintain a single source of truth and minimize discrepancies.
Command Example
To generate a Java class from a Thrift definition file, you can use the following command:
thrift -r --gen java user_service.thrift
Utilizing this command can automatically create the necessary Java classes, thus saving you time and reducing human error.
7. Forgetting to Optimize Performance
Performance considerations are paramount, especially in a microservices architecture. With Thrift, while it's designed to be efficient, not optimizing your servers and clients can lead to latency and throughput issues.
- Make use of
TBufferedTransport
to reduce the number of IO calls. - For serialization, consider
TCompactProtocol
to reduce the size of data transmitted.
8. Poor Documentation Practice
When developing protocols and services, many teams don't take the time to create effective documentation. This leads to confusion for new team members or external consumers of your services.
Always document your Thrift files and any deviations you make from standard service usage. Consider the following structure for documentation:
# UserService Definition
## Methods
- **getUser**: Retrieves the user by ID.
- **createUser**: Creates a new user.
## Exceptions
- **UserNotFoundException**: Thrown when the user ID does not exist.
Having well-structured documentation helps everyone involved understand the service better and reduces onboarding time.
Closing the Chapter
Starting with Apache Thrift in Java comes with its own set of challenges. By being aware of these common pitfalls, you can ensure your development process is not only smoother but also more efficient. From mastering the Thrift data model to effectively managing error handling and service versioning, each of these elements plays a critical role.
Remember, the key to mastering Thrift is to understand and respect its design philosophy. Embrace its features fully, keep performance in mind, and always strive for clarity in your code and documentation. For more advanced techniques and options, consider exploring the Apache Thrift documentation.
By navigating these potential pitfalls, you'll be better equipped to harness the full power of Apache Thrift for your Java applications, leading to more robust, scalable, and maintainable code. Happy coding!