Common Pitfalls When Moving Java Code Outside of Functions
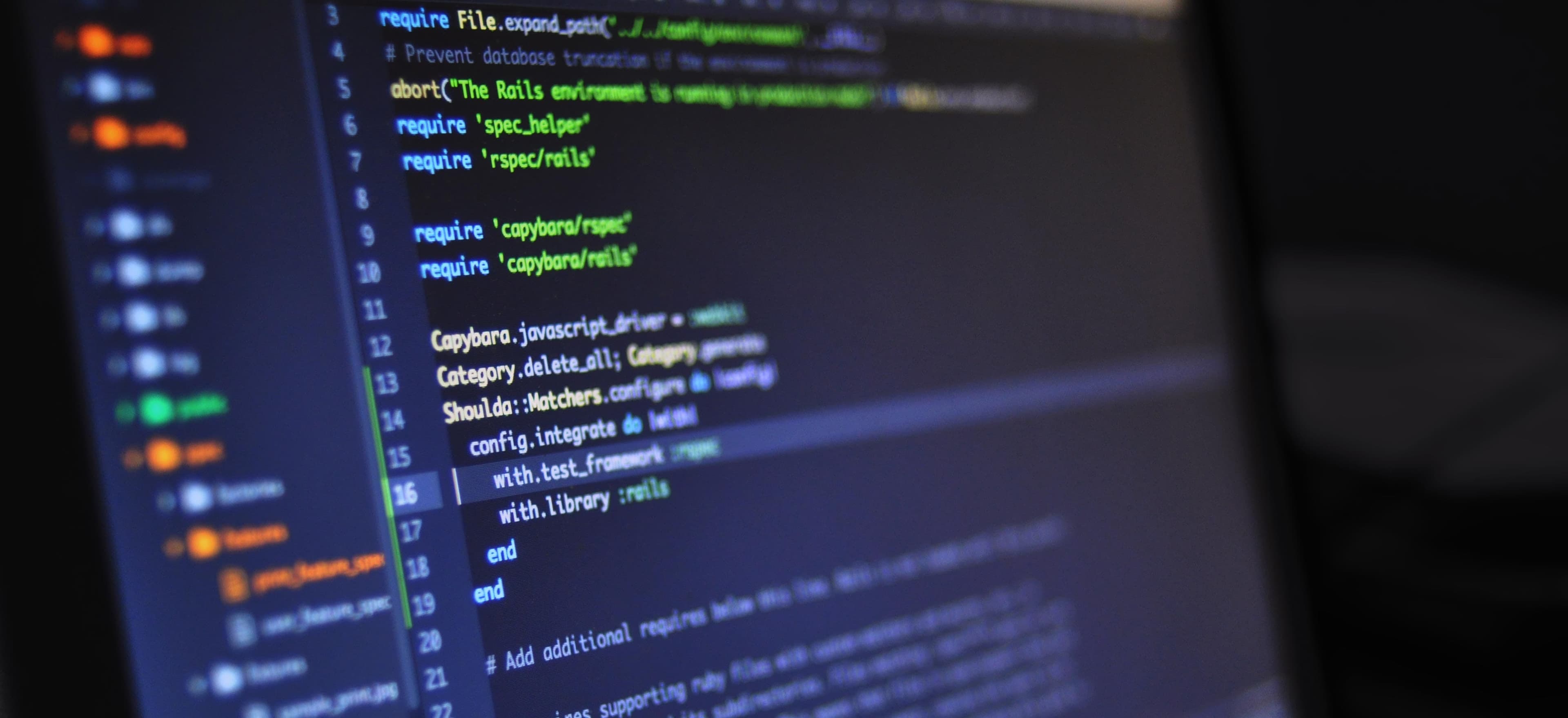
- Published on
Common Pitfalls When Moving Java Code Outside of Functions
Java is a versatile and powerful programming language. However, as with any tool, there are common pitfalls developers may encounter. One of these occurs when moving code outside of functions, a practice that may seem harmless but can introduce subtle bugs and readability issues. In this blog post, we will explore the pitfalls of moving Java code outside of functions, provide illustrative code snippets, and discuss best practices to maintain clean and efficient code.
Why Use Functions?
Functions, also known as methods in Java, are reusable blocks of code that perform a specific task. Using functions has several advantages:
- Code Reusability: Functions can be called multiple times throughout a program.
- Readability: Functions give a name to a block of code, making your intent clear.
- Maintainability: Changing one part of the code is easier when it's encapsulated within a function.
Given these benefits, it's critical to understand the implications of moving code outside of these functions.
Pitfall 1: Breaking Scope and Context
When you remove code from its original function, you may sever its scope context. This can lead to NullPointerException
or VariableScopeError
if the code relies on local variables or method parameters.
Example: Variable Scope Issue
public class Example {
private int counter = 0;
public void incrementCounter() {
counter++;
}
// The following code snippet illustrates moving the counter increment outside of its function.
// Moving the following line outside causes the counter increment to not function correctly.
// counter++;
}
Why this matters: By moving counter++
outside the function, you lose the control of incrementing the counter based on business logic. It becomes harder to manage user interactions over time.
To rectify this, always ensure that code retaining state-related context remains within functions, where the necessary variables are accessible.
Pitfall 2: Increased Complexity
Placing logic directly in the body of a class can complicate your program. This structure can lead to procedural mindset, making code tougher to follow.
Example: Adding Complexity:
public class Employee {
private String name;
public Employee(String name) {
this.name = name;
}
// Code block placed outside of functions
// public static void displayEmployeeName() {
// System.out.println("Employee Name: " + this.name);
// }
// This will cause a compilation error since `this` is not accessible in a static context.
}
Why this matters: Trying to access instance variables from a static context will lead to errors. While static methods are useful for certain functionalities, they should not access instance data directly.
Instead, consider using instance methods for accessing the variable name
:
public void displayEmployeeName() {
System.out.println("Employee Name: " + this.name);
}
This enhances readability, maintains the integrity of the instance variable, and makes your code manageable.
Pitfall 3: Dependency and Coupling Issues
Moving code around may inadvertently increase coupling between classes. High coupling between components can reduce flexibility and testability.
Example: Increased Coupling
public class Order {
private ShoppingCart cart;
public Order(ShoppingCart cart) {
this.cart = cart;
}
// Directly manipulating the cart variable outside of a function can lead to issues
// cart.clearItems();
}
Why this matters: If clearItems()
is called outside of its designed methods, you could unintentionally clear the cart at inappropriate times.
To avoid this, keep interactions encapsulated within methods:
public void processOrder() {
cart.clearItems();
}
This method represents a complete workflow on how items should be managed, preserving the ability to maintain and modify the logic more efficiently.
Pitfall 4: Testing and Debugging Challenges
Code that lacks structure is often harder to test. Having business logic outside functions can lead to frustration during unit testing or debugging.
Example: Testing Difficulty
public class Calculator {
public static int add(int a, int b) {
// Adding logic outside any function
// return a + b; // This works,
// but messy scopes need to be fixed.
}
}
Why this matters: The reliance on ‘static’ context requires you to wrap logical flows in methods, detracting from clean testing structures.
By defining structured methods, you make it easier to write tests.
public int add(int a, int b) {
return a + b;
}
This method can then be tested in isolation.
Best Practices to Avoid Common Pitfalls
-
Encapsulate Logic: Always place code that manipulates state within functions or methods. This maintains the right context and scope.
-
Use Meaningful Names: When defining functions, use names that convey purpose. This leads to better readability.
-
Keep Classes Focused: Each class should have a single responsibility. If methods begin to diverge from the class's main purpose, consider refactoring.
-
Write Tests: To ensure code remains functional and errors are caught early, write unit tests. This reinforces good structure and helps identify pitfalls created by code rearrangements.
-
Refactor: Regularly assess your code structure. Refactor when necessary to retain clarity and performance.
Closing the Chapter
Moving Java code outside of functions may seem benign, but it can introduce several challenges concerning scope, complexity, coupling, and testing. Understanding these pitfalls is essential for writing maintainable and efficient code. Emphasizing encapsulation, meaningful naming, and the use of functions can mitigate these issues.
By adhering to best practices and fostering a habit of checking for potential issues during code refactoring, developers can assure their Java applications remain robust, readable, and efficient. Happy coding!
For more information on Java best practices, consider visiting the Oracle Java Documentation and explore Effective Java for insightful programming guidelines.
Hope this blog post sheds light on the common pitfalls when moving Java code outside functions and provides actionable insights for sound programming practices!