Common MyBatis Configuration Mistakes in Spring MVC 3
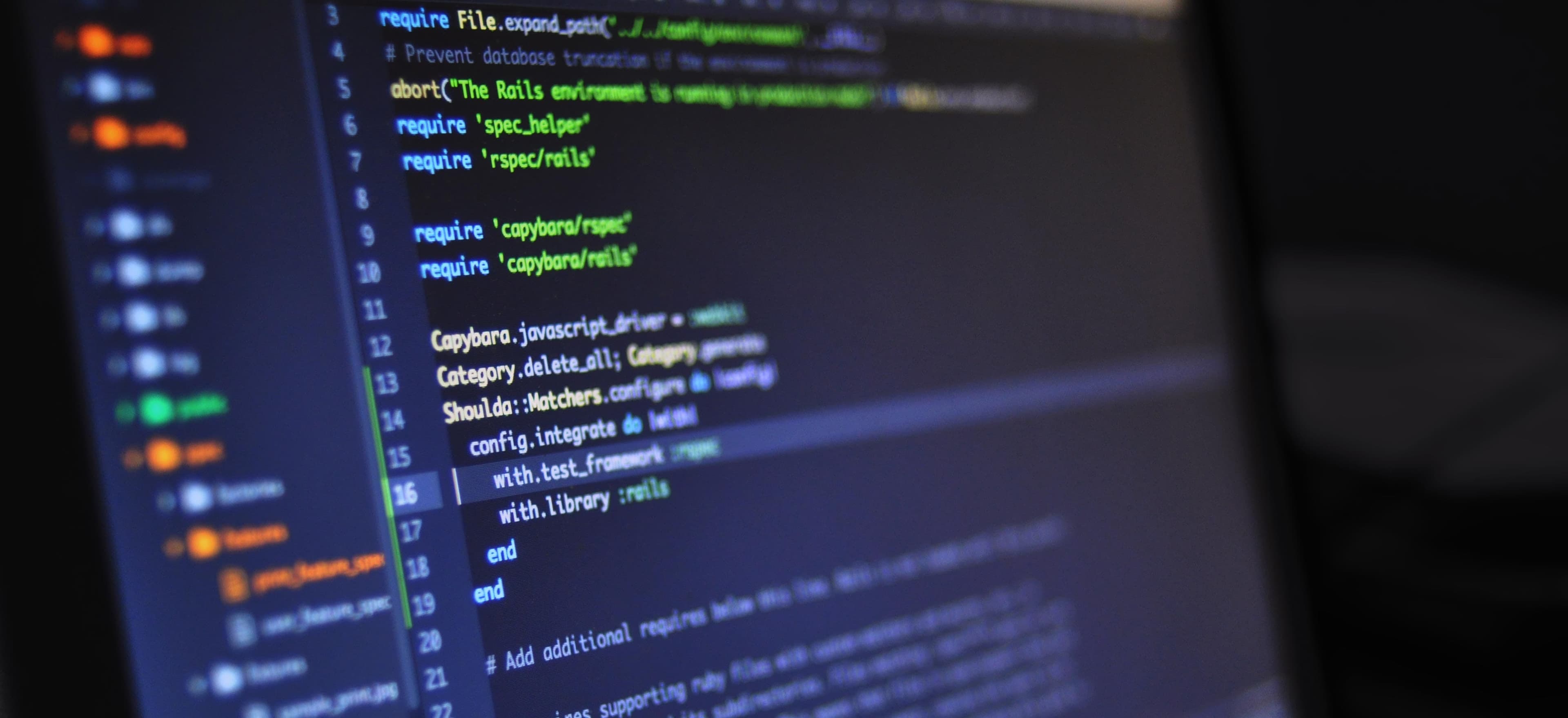
- Published on
Common MyBatis Configuration Mistakes in Spring MVC 3
MyBatis is a popular persistence framework that facilitates the interaction between Java applications and relational databases, while Spring MVC provides a comprehensive web framework for building Java applications. Combining these two powerful tools can lead to high-performing applications. Nonetheless, developers often encounter pitfalls during configuration. In this blog post, we will discuss common MyBatis configuration mistakes in Spring MVC 3 and how to avoid them.
Table of Contents
- Understanding MyBatis and Spring MVC
- Common Configuration Mistakes
- Mistake 1: Incorrect XML Configuration
- Mistake 2: Missing Component Scanning
- Mistake 3: Improper Data Source Configuration
- Mistake 4: Issues with Mappers
- Mistake 5: Mismanagement of Transactions
- Best Practices for MyBatis with Spring MVC
- Conclusion
Understanding MyBatis and Spring MVC
MyBatis simplifies database interactions through mapping SQL statements to Java methods. On the other hand, Spring MVC facilitates a robust request-handling framework for creating web applications. When integrated correctly, they can create applications that are both efficient and easy to maintain. However, misconfigurations can lead to hard-to-debug issues.
Common Configuration Mistakes
Mistake 1: Incorrect XML Configuration
One of the most common mistakes in configuring MyBatis is an improperly set up XML configuration file. This file defines the database connection pool and the mapper files.
Example:
<configuration>
<settings>
<setting name="cacheEnabled" value="true"/>
</settings>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/mydatabase"/>
<property name="username" value="root"/>
<property name="password" value="password"/>
</dataSource>
</environment>
</environments>
</configuration>
Why is this a problem?
Failing to include essential properties or misspelling them can cause the MyBatis framework to throw exceptions. It's crucial to validate that all properties are correctly set, especially the database credentials.
Mistake 2: Missing Component Scanning
When integrating MyBatis with Spring MVC, missing component scanning in the Spring configuration files is another common mistake.
Example:
<context:component-scan base-package="com.example.myapp"/>
<mybatis:scan base-package="com.example.myapp.mappers"/>
Why is this a problem?
Component scanning is essential for Spring to detect your services, repositories, and configurations. If MyBatis mappers are not scanned, Spring will not autowire the necessary beans, leading to NullPointerExceptions
when your application runs.
Mistake 3: Improper Data Source Configuration
Configuring the data source incorrectly can lead to issues such as connection failures.
Example:
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/mydatabase");
dataSource.setUsername("root");
dataSource.setPassword("password");
return dataSource;
}
Why is this a problem?
Ensure the driver class name and URL format are correct. Minor oversights can lead to significant downtime. Always double-check your connection details.
Mistake 4: Issues with Mappers
Another frequent issue arises when attempting to use mapper files. Incorrectly defining the namespace or SQL queries can cause application failures.
Example:
<mapper namespace="com.example.myapp.mappers.UserMapper">
<select id="getUser" resultType="com.example.myapp.models.User">
SELECT * FROM users WHERE id = #{id}
</select>
</mapper>
Why is this a problem?
If the namespace does not match the interface name, MyBatis cannot find the corresponding SQL query. Pay close attention to spelling and ensure the mapper files are picked up during the configuration process.
Mistake 5: Mismanagement of Transactions
Transactional management in a MyBatis + Spring MVC setup can be another source of issues. Make sure to configure transaction management correctly.
Example:
<tx:annotation-driven transaction-manager="transactionManager"/>
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
Why is this a problem?
Failing to manage transactions properly can lead to data integrity issues. Ensure that the @Transactional annotations are applied to your service methods to manage transactions explicitly.
Best Practices for MyBatis with Spring MVC
-
Use Annotation-Based Configuration: Instead of relying solely on XML configuration, consider adopting Java-based configuration. It offers better IDE support and compile-time checks.
-
Regularly Validate Your Configuration: Make it a habit to review and validate your MyBatis and Spring MVC configurations to ensure they are error-free.
-
Logging: Enable logging for MyBatis to troubleshoot SQL related issues effectively. Set the logging level to DEBUG to see the generated SQL and parameters.
<properties>
<property name="logImpl" value="LOG4J"/>
</properties>
-
Utilize the MyBatis generator: This tool can help streamline the creation of mapper interfaces and SQL statements automatically.
-
Maintain a Clear Project Structure: Keep mappers, services, and models in distinctly organized packages. This clarity will facilitate easier debugging and enhance maintainability.
Closing the Chapter
Integrating MyBatis with Spring MVC can drastically simplify data access in your Java applications. However, it's crucial to avoid common configuration mistakes. Paying attention to XML configurations, component scanning, data source settings, mapper definitions, and transaction management can prevent many headaches down the line.
For more in-depth learning, consider visiting the official MyBatis Documentation and Spring Framework Documentation.
By addressing these common pitfalls and adhering to best practices, you’ll position yourself to harness the full potential of MyBatis and Spring MVC for your Java applications. Happy coding!