Mastering Timeout Issues in Async JAX-RS Requests
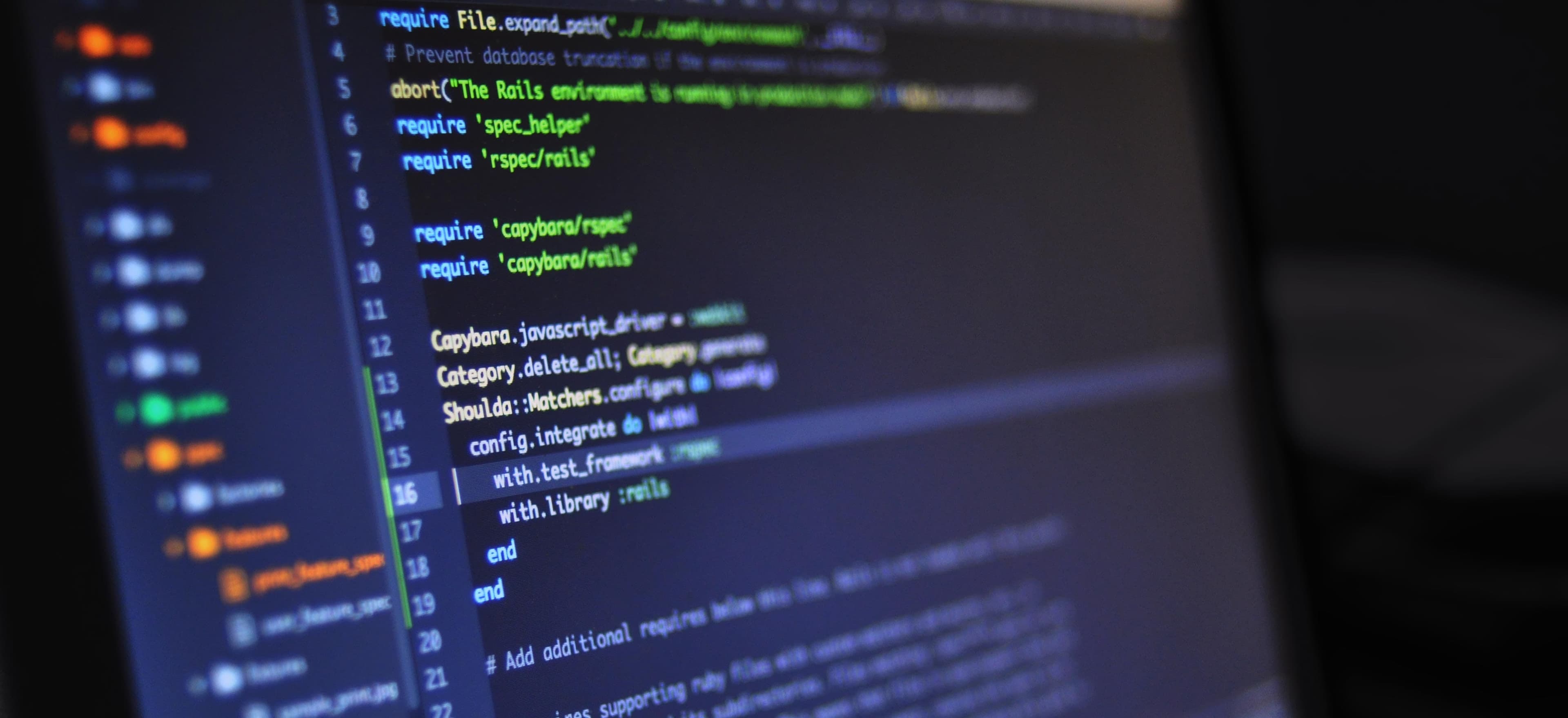
- Published on
Mastering Timeout Issues in Async JAX-RS Requests
As applications grow, performance becomes crucial, especially when dealing with RESTful services. When using JAX-RS (Java API for RESTful Web Services), asynchronous requests can significantly enhance the responsiveness of your web applications. However, handling timeouts in these asynchronous requests is vital to maintaining application reliability and user experience. In this article, we will delve into managing timeout issues effectively in async JAX-RS requests.
Understanding Asynchronous JAX-RS
JAX-RS provides a way to create RESTful web services in Java, and its asynchronous capabilities allow you to serve requests in a non-blocking manner. This means a server can handle multiple requests without getting blocked by long-running processes, enhancing scalability.
To utilize asynchronous processing in JAX-RS, you can implement the @Suspended
annotation, which allows you to suspend the response until the processing is complete. Let’s explore this further with an example.
Example of Asynchronous Handling in JAX-RS
Here’s a basic example of how to implement asynchronous processing in JAX-RS:
import javax.ws.rs.*;
import javax.ws.rs.core.*;
import javax.ws.rs.container.AsyncResponse;
import javax.ws.rs.container.Suspended;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Executors;
@Path("/async")
public class AsyncService {
@GET
@Path("/process")
public void processRequest(@Suspended AsyncResponse response) {
Executors.newCachedThreadPool().submit(() -> {
try {
Thread.sleep(5000); // Simulate long processing time
response.resume("Processing done!");
} catch (InterruptedException e) {
response.resume(e);
}
});
}
}
Explanation of Code
- @Suspended Annotation: This is crucial for asynchronous requests as it tells JAX-RS to suspend the response.
- Executors: Used to manage the logic in a separate thread, allowing the server to handle other requests concurrently.
- Thread.sleep: Simulates a lengthy operation. In a real scenario, this would be replaced by actual business logic like database access or external API calls.
- Response Handling: The response is resumed once processing is complete or an error occurs.
The Importance of Timeouts
While asynchronous processing improves performance, it can lead to issues if the processing takes too long. Users might experience delays, and system resources may be under pressure. This is where timeout management comes into play.
Timeout Strategies
- Client-Side Timeout: The client could set a timeout for how long they are willing to wait for a response.
- Server-Side Timeout: The server can enforce a timeout to prevent exhaustion of resources.
Let’s delve deeper into implementing server-side timeouts in JAX-RS.
Implementing Server-Side Timeouts
To control timeout behavior in your JAX-RS application, you can use the AsyncResponse
interface, which provides a method setTimeout
. It allows you to specify a timeout interval. Here’s how you can do that.
Setting a Timeout For AsyncResponse
import java.util.concurrent.*;
@Path("/timeout")
public class TimeoutService {
@GET
@Path("/process")
public void processRequest(@Suspended AsyncResponse response) {
// Set a timeout of 3 seconds
response.setTimeout(3, TimeUnit.SECONDS);
Executors.newCachedThreadPool().submit(() -> {
try {
Thread.sleep(5000); // Simulate long processing time
response.resume("Processing done!");
} catch (InterruptedException e) {
response.resume(e);
}
});
response.setTimeoutHandler(res -> {
res.resume(Response.status(Response.Status.REQUEST_TIMEOUT)
.entity("Processing timeout occurred!")
.build());
});
}
}
Explanation of Timeout Implementation
- setTimeout: This method is invoked on
AsyncResponse
to specify a delay before timing out. - TimeoutHandler: This function runs if the timeout occurs. It allows you to customize the response, which in this case sends a timeout message back to the client.
Best Practices for Handling Timeouts
- Avoid Long Processing Times: If possible, split complex operations into smaller tasks.
- Graceful Degradation: Consider providing alternative responses if a timeout occurs, like cached data or a message indicating that the service is busy.
- Logging and Monitoring: Keep track of instances when timeouts occur. This can aid in diagnosing slow operations or potential issues in the back-end.
- Use Circuit Breakers: When working in a microservices architecture, incorporating circuit breaker patterns can help manage failures in downstream services effectively. You can explore the Resilience4j library for such patterns.
Lessons Learned
Mastering timeout issues in asynchronous JAX-RS requests is fundamental for building robust web applications. Understanding both client-side and server-side timeout management provides you with the necessary tools to enhance user experience and application reliability.
By leveraging asynchronous capabilities with effective timeout strategies, your services can perform better under load and provide a smoother experience, ultimately leading to user satisfaction and better resource utilization.
For additional reading, consider checking the official JAX-RS documentation for more details and advanced features.
With mindful implementation of timeouts, you can ensure that your application not only performs well but also handles potential failures gracefully.
Feel free to reach out if you have any questions or need further assistance on handling asynchronous requests in JAX-RS!
Checkout our other articles