Mastering CompletableFuture: Tackling Race Conditions in REST Calls
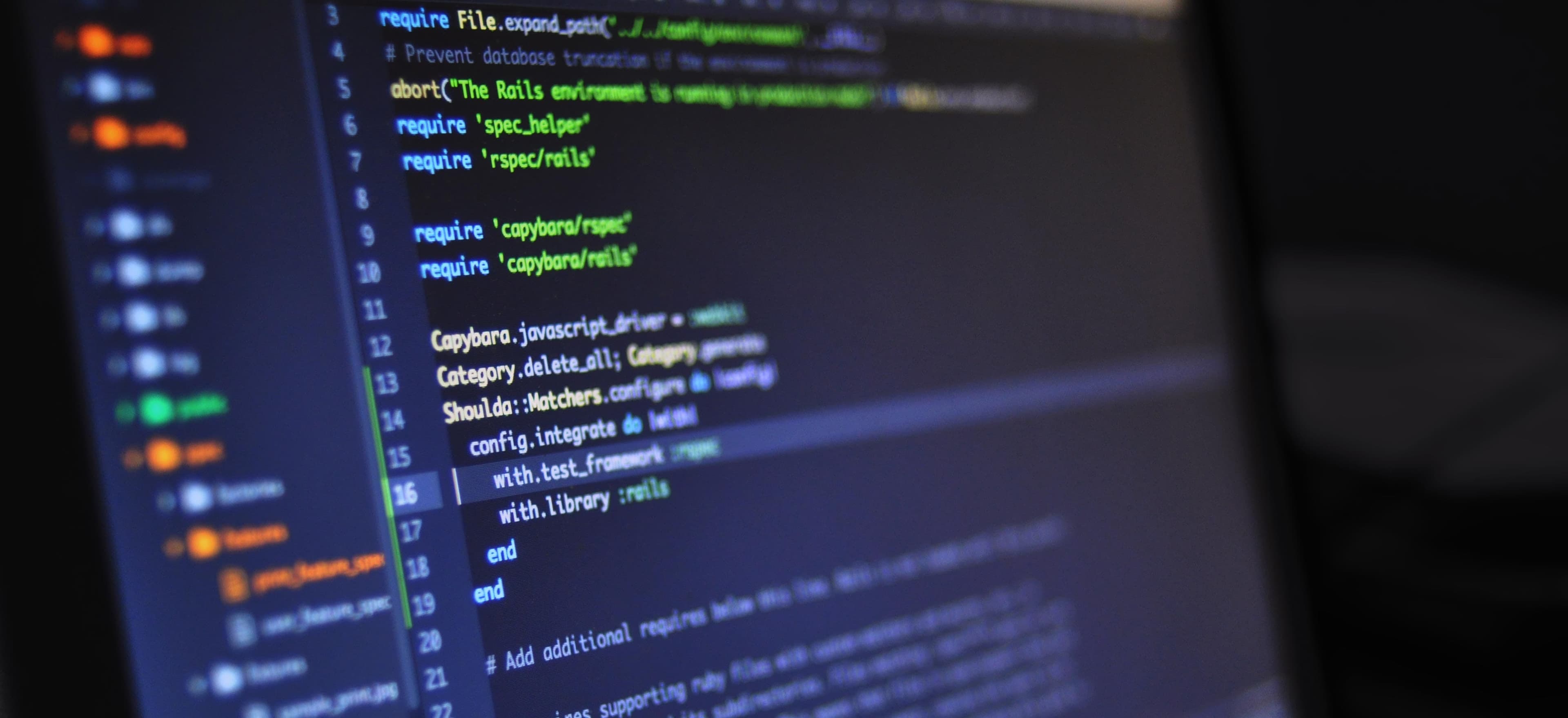
- Published on
Mastering CompletableFuture: Tackling Race Conditions in REST Calls
Java has evolved significantly over the years, and one of its most powerful features is the CompletableFuture
API introduced in Java 8. With asynchronous programming becoming the norm in modern applications, understanding how to effectively use CompletableFuture
to manage REST calls and tackle race conditions is crucial. In this post, we'll delve into how CompletableFuture
can enhance your Java applications by managing asynchronous processing and avoiding common pitfalls like race conditions.
Understanding CompletableFuture
CompletableFuture
is a significant leap forward from traditional threading methods in Java. It provides a way to write non-blocking code that can improve application performance by making better use of system resources.
Here’s a quick definition:
What is CompletableFuture?
CompletableFuture
is a class in the java.util.concurrent
package that represents a future result of an asynchronous computation. It allows you to write asynchronous, non-blocking code more easily than using traditional threads.
Why Use CompletableFuture?
- Asynchronous Programming: It allows methods to return immediately without waiting for the result.
- Composability: You can chain multiple computations together easily.
- Error Handling: It offers a cleaner mechanism for handling exceptions compared to the traditional
try-catch
blocks.
Simple CompletableFuture Example
Let's consider a basic example of creating a CompletableFuture
:
import java.util.concurrent.*;
public class SimpleCFExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulating a long-running task
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Hello, CompletableFuture!";
});
// This will not block and will return immediately
System.out.println("Doing other work while waiting for the result...");
// Get the result of the asynchronous computation
try {
String result = future.get(); // This call will block until the result is available
System.out.println("Result from CompletableFuture: " + result);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
}
Code Explanation
-
supplyAsync:
- We are simulating a long-running task using
Thread.sleep()
. - This method is executed in a separate thread, freeing up the main thread for other tasks.
- We are simulating a long-running task using
-
get() Method:
- This blocks the main thread until the result is available, which helps maintain synchronization when needed.
Tackling Race Conditions in REST Calls
In distributed systems, such as microservices architectures, race conditions can occur during REST calls when two or more requests modify the same resource simultaneously. This can lead to inconsistent states and unpredictable behavior.
Let’s explore how to use CompletableFuture
to avoid such conflicts by ensuring that we handle RESTful calls properly.
Making Concurrent REST Calls
Assuming we have two REST endpoints that need to be accessed concurrently, we can manage them with CompletableFuture
.
Example REST Service
import org.springframework.web.client.RestTemplate;
import java.util.concurrent.*;
public class RestServiceExample {
private RestTemplate restTemplate = new RestTemplate();
public CompletableFuture<String> makeFirstCall() {
return CompletableFuture.supplyAsync(() -> restTemplate.getForObject("https://api.example.com/first", String.class));
}
public CompletableFuture<String> makeSecondCall() {
return CompletableFuture.supplyAsync(() -> restTemplate.getForObject("https://api.example.com/second", String.class));
}
}
Executing REST Calls Concurrently
Using the aforementioned service, we can execute both calls concurrently:
public static void main(String[] args) {
RestServiceExample service = new RestServiceExample();
CompletableFuture<String> firstCall = service.makeFirstCall();
CompletableFuture<String> secondCall = service.makeSecondCall();
CompletableFuture<Void> combinedFuture = CompletableFuture.allOf(firstCall, secondCall);
// Wait for all futures to complete
combinedFuture.join();
try {
System.out.println("First Call Result: " + firstCall.get());
System.out.println("Second Call Result: " + secondCall.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
Combating Race Conditions
When dealing with REST calls that modify shared resources, it's essential to ensure that race conditions are avoided. One way to achieve this is through the use of thenCompose
:
public CompletableFuture<String> safeUpdate(String data) {
return CompletableFuture.supplyAsync(() -> {
// Imagine this represents a synchronous REST call
return restTemplate.postForObject("https://api.example.com/update", data, String.class);
}).thenApply(result -> {
// Process the result further if needed
return "Data updated with result: " + result;
});
}
Explanation
- safeUpdate method:
- It handles the shared resource update within a
CompletableFuture
. - It ensures the processing of data after the REST call completes, ensuring that updates are managed correctly to avoid conflicts.
- It handles the shared resource update within a
Handling Errors Gracefully
A comprehensive application must handle exceptions that arise from REST calls. Here’s an improved example that captures errors using exceptionally
:
public CompletableFuture<String> makeCallAndHandleError() {
return CompletableFuture.supplyAsync(() -> {
return restTemplate.getForObject("https://api.example.com/data", String.class);
}).exceptionally(ex -> {
System.out.println("Error occurred: " + ex.getMessage());
return "Default Value"; // Fallback value
});
}
Explanation
- exceptionally method:
- It captures any exceptions during the execution of the async call and provides a fallback value.
The Closing Argument
The CompletableFuture
API is a powerful tool that can help you tackle race conditions in REST calls while making your Java applications more robust and responsive.
By following these patterns and best practices, you can ensure safe concurrent processing when dealing with shared resources.
For further reading on CompletableFuture in Java and better practices in REST calls, explore the official documentation and guidelines.
Embracing asynchronous programming in Java using CompletableFuture
will greatly enhance your application’s efficiency and stability, empowering you to build modern, high-performance applications.
Checkout our other articles