Tackling Misfire Issues in Quartz Scheduler Effectively
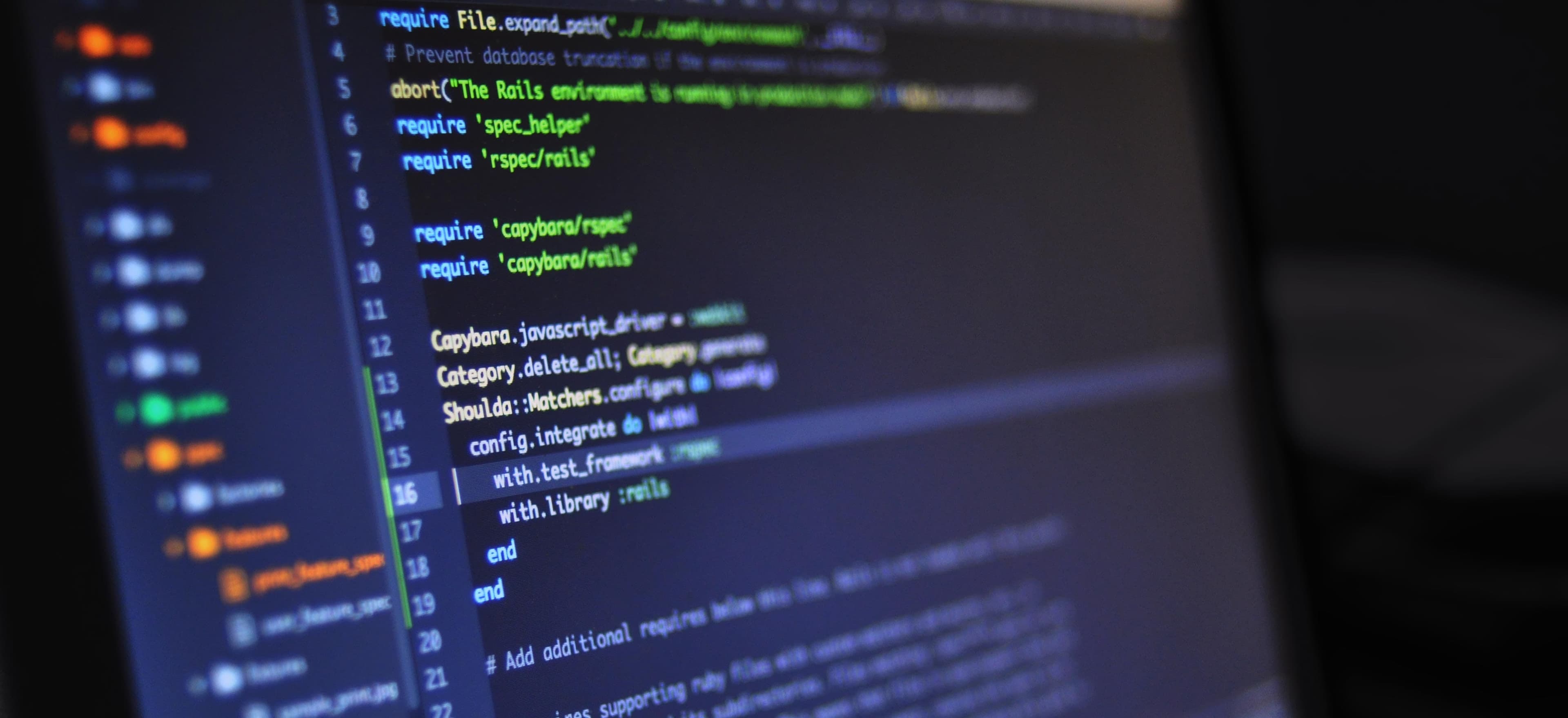
- Published on
Tackling Misfire Issues in Quartz Scheduler Effectively
Quartz Scheduler is a powerful and flexible open-source job scheduling library in Java. It's used extensively in enterprise applications to manage scheduled tasks efficiently. However, one common challenge developers often face is handling misfires – situations when a scheduled job does not execute at the correct time. Understanding misfires is crucial for maintaining the reliability and performance of your applications.
In this blog post, we will delve into what misfires are, the causes of misfire issues, how to configure Quartz Scheduler to handle them, and best practices for ensuring smooth job execution.
Understanding Misfires in Quartz Scheduler
A "misfire" occurs when a job is unable to execute at its scheduled time. In Quartz, misfires can happen for various reasons, including:
- The system was down at the scheduled time.
- The job took longer to complete than anticipated.
- Resource constraints, such as memory or processing power.
How Misfires Impact Your Applications
When jobs misfire, the implications can be significant, including:
- Delayed processing of critical tasks.
- Increased backlog of queued jobs.
- Unexpected behavior that may lead to data integrity issues.
To tackle these challenges head-on, it’s essential to understand how to properly configure Quartz Scheduler to handle misfires effectively.
Configuring Misfire Policies
Quartz Scheduler provides several strategies for dealing with misfires through its MisfireInstruction
options. Let’s discuss the main types of misfire instructions:
1. Misfire Policy Options
Each job can specify how Quartz should handle misfires by setting a misfire instruction. Here are common options:
MISFIRE_INSTRUCTION_SIMPLE
: Triggers the job immediately upon detecting a misfire.MISFIRE_INSTRUCTION_JOB_DEFAULT
: Uses the default job misfire instruction.MISFIRE_INSTRUCTION_CRON_TRIGGER
: For cron-based triggers, this instructs the trigger to skip the misfired execution and re-schedule based on the next execution time.
Here’s an example of how to set a misfire instruction in a Quartz job:
import org.quartz.JobBuilder;
import org.quartz.JobDetail;
import org.quartz.Trigger;
import org.quartz.TriggerBuilder;
import org.quartz.SimpleScheduleBuilder;
public class MisfireExample {
public void createJob() {
JobDetail job = JobBuilder.newJob(MyJob.class)
.withIdentity("myJob", "group1")
.build();
// Setting up the trigger with a misfire instruction
Trigger trigger = TriggerBuilder.newTrigger()
.withIdentity("myTrigger", "group1")
.startNow()
.withSchedule(SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(10)
.repeatForever()
.withMisfireHandlingInstructions(SimpleTrigger.MISFIRE_INSTRUCTION_RESCHEDULE_NEXT_WITH_REMAINING_COUNT))
.build();
// Schedule the job and trigger
Scheduler scheduler = StdSchedulerFactory.getDefaultScheduler();
scheduler.start();
scheduler.scheduleJob(job, trigger);
}
}
Why This Code Matters
In the above snippet, we configured a simple job with a trigger that repeats every 10 seconds. The misfire handling instruction chosen (MISFIRE_INSTRUCTION_RESCHEDULE_NEXT_WITH_REMAINING_COUNT
) ensures that if a misfire occurs, it will try to execute the job as soon as possible without ignoring missed counts.
Understanding Run-Time vs. Misfire Criteria
When scheduling jobs, different types of triggers use different criteria to determine what constitutes a misfire. Additionally, jobs can be set for different execution contexts based on runtime parameters and misfire criteria.
2. Complex Trigger Configurations
For jobs that are scheduled using Cron triggers, the misfire strategy is slightly different. Here’s a simple cron job configuration:
import org.quartz.CronTrigger;
import org.quartz.CronScheduleBuilder;
// Define Cron Trigger with misfire support
CronTrigger cronTrigger = TriggerBuilder.newTrigger()
.withIdentity("cronTrigger", "group1")
.withSchedule(CronScheduleBuilder.cronSchedule("0/15 * * * * ?") // every 15 seconds
.withMisfireHandlingInstructionDoNothing())
.build();
Why Choose Do Nothing
?
Choosing withMisfireHandlingInstructionDoNothing()
ensures that if the job is missed during scheduled execution, Quartz will not execute it immediately but will wait until the next scheduled time (cronSchedule
). This is particularly useful in scenarios where running the job multiple times is not desired and you want to maintain a controlled execution flow.
Best Practices for Handling Misfires
1. Evaluate Job Requirements
Before implementing any misfire policy, ask yourself: "Do I want to re-execute missed jobs?" If your job is critical, re-running might be essential. For non-critical jobs, it could lead to duplication and further issues.
2. Monitor Application Performance
Always monitor the performance of your Quartz Scheduler implementation. Misfires can sometimes be symptomatic of a larger system issue, such as resource exhaustion or poor job design.
3. Evaluate Real-Time Data Needs
Ensure each job is designed with its data dependencies in mind. If data is real-time dependent, consider utilizing better resource management to avoid job delays.
4. Test Misfire Handling
When deploying code changes, ensure to test scenarios with misfires. Use testing frameworks to simulate system downtime or job duration to see how your configurations perform.
5. Leverage Logging
Integrate logging mechanisms to monitor misfire events. This data can provide valuable insights into when and why misfires are occurring so you can refine your configurations as needed.
Additional Resources
To dive deeper into Quartz Scheduler configuration and usage, have a look at the Quartz Scheduler documentation and consider this Java tutorial on scheduling tasks with Quartz.
The Bottom Line
Handling misfires in Quartz Scheduler is not just about configuration; it’s about understanding business needs and system capabilities. By evaluating misfire policies, monitoring applications, and adjusting job configurations, you can create a robust scheduling system that minimizes disruptions and improves reliability.
Tackling misfire issues head-on can significantly enhance your application's performance, ensuring timely execution of all scheduled jobs. As you implement these strategies, you’ll find that managing Quartz Scheduler becomes a seamless part of your development process. Happy scheduling!
Checkout our other articles