Overcoming Parquet Read Speed Issues with SparkSQL and Alluxio
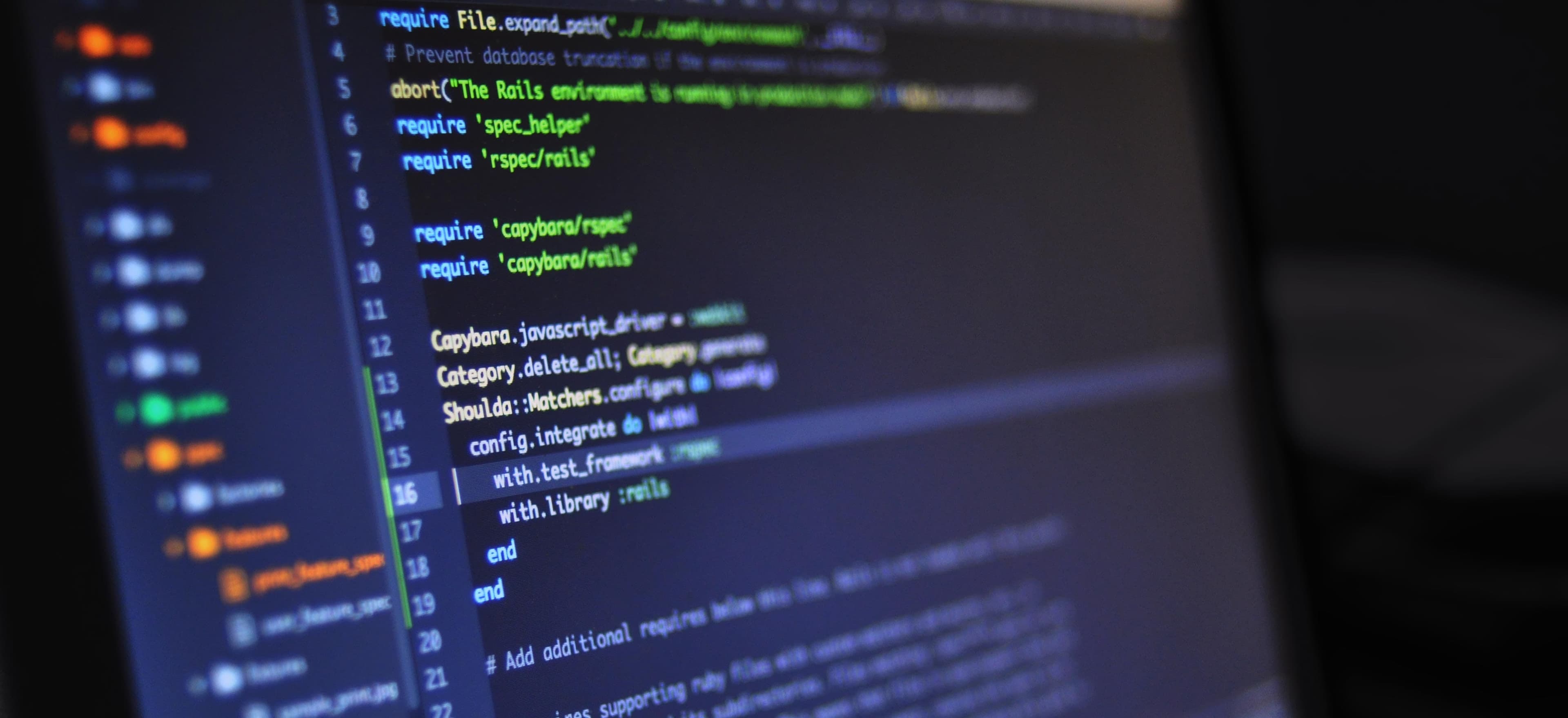
- Published on
Overcoming Parquet Read Speed Issues with SparkSQL and Alluxio
In today's data-centric world, efficient data retrieval is paramount. The Apache Parquet format has gained popularity for its columnar storage capabilities, which optimize big data processing. However, using SparkSQL to read Parquet files can sometimes be slow, particularly when dealing with large datasets. This is where Alluxio, a memory-centric virtual distributed storage system, comes into play to enhance performance.
Understanding the Challenge
Reading large datasets stored in Parquet format using SparkSQL can present several issues:
- I/O Bottlenecks: The read speed can be significantly affected by network latency and disk I/O.
- Data Serialization: Converting Parquet to DataFrames can introduce overhead.
- Cluster Resource Contention: Unoptimized queries can lead to resource saturation on the cluster.
The Alluxio Advantage
Alluxio serves as an abstraction layer between storage and compute engines, allowing faster access to datasets. Alluxio caches data in memory, which helps alleviate the I/O bottleneck. Thus, even if your Parquet files are stored in a slower, persistent storage system like HDFS or S3, Alluxio can speed up data retrieval.
Benefits of Using Alluxio
- Improved Performance: By caching frequently accessed data in memory, the read times can drop significantly.
- Flexible Storage Layers: Alluxio allows you to combine various storage solutions seamlessly, providing great agility.
- Easy Integration: It integrates well with existing systems like SparkSQL, reducing the friction usually experienced during setup.
Setup Instructions
To exploit Alluxio in your SparkSQL workflows, you need to follow these steps:
-
Installing Alluxio: Download and set up Alluxio on your cluster. Refer to the official Alluxio documentation for comprehensive guidance.
-
Configuring SparkSQL: Ensure that Spark is configured to work with Alluxio by adding the necessary dependencies in your Spark application.
Sample Configuration
In your spark-defaults.conf
, include:
spark.jars.packages=org.alluxio:alluxio-client:2.8.0
Code Snippet: Connecting SparkSQL to Alluxio
Below is an example of how to connect SparkSQL to Alluxio and read a Parquet file.
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class SparkAlluxioExample {
public static void main(String[] args) {
// Create a Spark session
SparkSession spark = SparkSession.builder()
.appName("Alluxio Spark Integration")
.master("local[*]")
.getOrCreate();
// Load a Parquet file from Alluxio
String alluxioPath = "alluxio:///path/to/your/data.parquet";
Dataset<Row> parquetData = spark.read().parquet(alluxioPath);
// Show the content of the DataFrame
parquetData.show();
// Perform some transformations or actions...
// parquetData.filter("columnName > value").show();
// Stop Spark session
spark.stop();
}
}
Explanation of the Code:
- Spark Session: An entry point to your Spark application, providing the configuration and enabling the use of DataFrames.
- Reading Parquet: The
sparksession.read().parquet()
method is being used to load Parquet data from Alluxio efficiently. - Displaying Data: The
show()
method displays a sample of the data for quick verification.
Optimization Techniques
Once you have set up your system, consider the following optimization techniques to get the most out of your SparkSQL and Alluxio combination:
1. Caching DataFrame
If certain datasets are used frequently, cache them to prevent repeated I/O operations.
parquetData.cache();
2. Partitioning
Utilize Parquet's inherent ability to support partitioned datasets. When saving your DataFrame, specify the partition columns:
parquetData.write()
.partitionBy("year")
.parquet("alluxio:///path/to/output/");
3. Predicate Pushdown
To improve read speed, use predicate pushdown to read only the necessary data from Parquet files. When executing a query:
Dataset<Row> filteredData = parquetData.filter("column_name = 'desired_value'");
Performance Metrics
To quantify improvements, measure the read times before and after integrating Alluxio. You can use Spark's built-in metrics or external monitoring tools like Grafana for deeper insights into your Spark environment.
The Bottom Line
By leveraging Alluxio with Apache SparkSQL, you can significantly mitigate Parquet read speed issues. The sum of caching, optimization techniques, and proper configuration can lead to robust data processing pipelines capable of handling large datasets seamlessly.
For further reading, consider checking out these resources:
Integrating Alluxio with SparkSQL not only enhances the data processing speed but also improves the overall efficiency of your data architecture. So, whether you're developing data analytics workflows or building data-driven applications, ditch the sluggish parsing times and embrace the performance boost with Alluxio.
Feel free to adapt any of the configurations or methodologies discussed based on your specific use case. Happy coding!