Struggling with Java Name Initials? Here's the Fix!
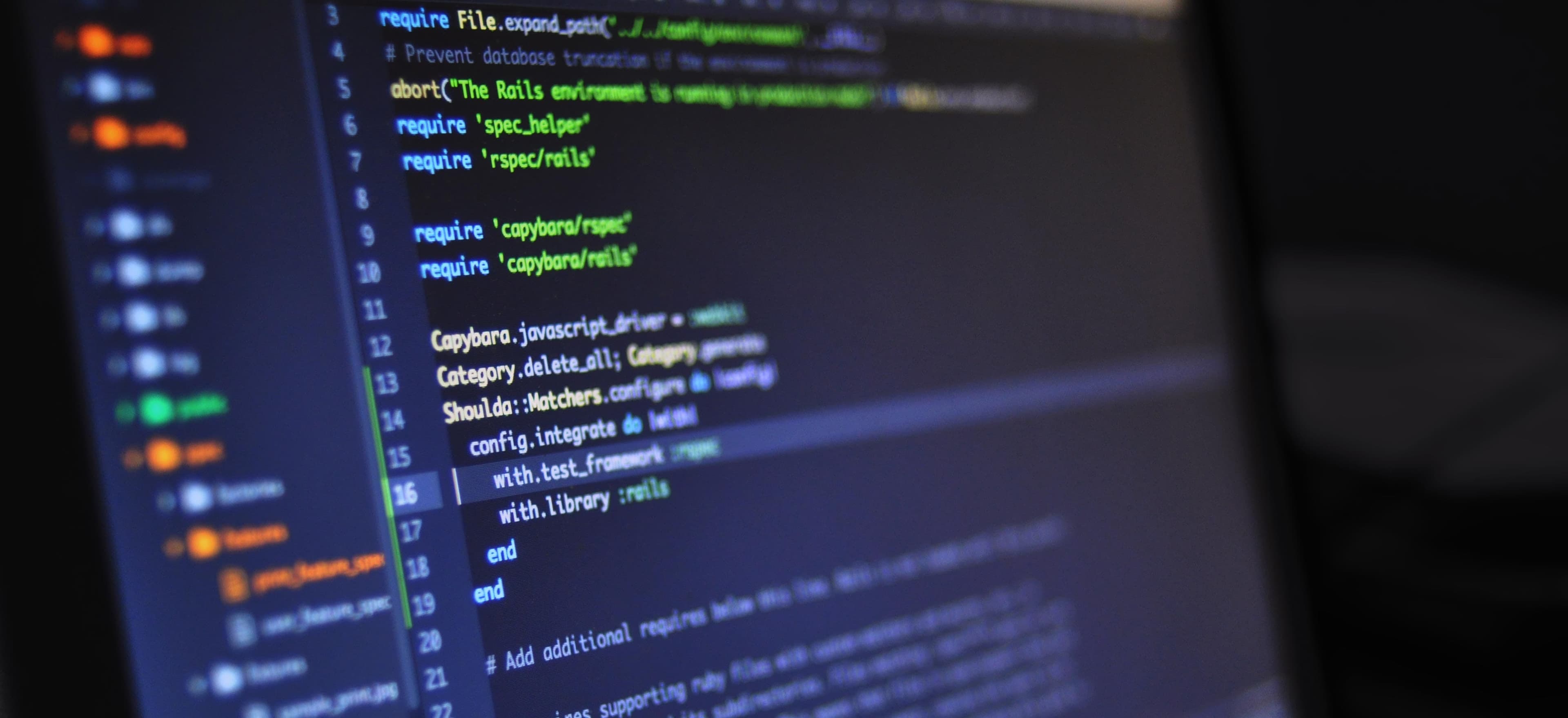
- Published on
Struggling with Java Name Initials? Here's the Fix!
Java is a versatile and powerful programming language, widely used for developing applications. A common task many developers might encounter is generating initials from names—an operation that may seem trivial but has its quirks and nuances. If you've found yourself puzzled when trying to extract initials from a string in Java, you are in the right place. Let’s dive in and explore effective ways to solve this issue.
Understanding the Problem
Suppose you have a full name, such as "John Doe", and you want to extract the initials "J.D." from it. Sounds simple, right? However, names can vary greatly. They might have more than two parts (first name, middle name, last name) or even include suffixes.
Key Challenges
- Multiple Names: How do you handle names like "Mary Jane Smith"?
- Handling Edge Cases: What if the string is empty, null, or just whitespace? How does your code respond?
- Custom Formatting: Different conventions for initials—some people prefer periods while others don’t.
A Java Solution
Let's break this down into manageable pieces with code snippets to illustrate each step.
Step 1: Basic Setup
First, let's create a simple Java method to extract initials from a name. We will have to split the name into individual components, usually based on spaces.
public class InitialsExtractor {
public static String getInitials(String fullName) {
if (fullName == null || fullName.trim().isEmpty()) {
return "";
}
StringBuilder initials = new StringBuilder();
String[] nameParts = fullName.split(" ");
for (String namePart : nameParts) {
if (!namePart.isEmpty()) {
initials.append(namePart.charAt(0)).append(".");
}
}
// Remove the last period
initials.setLength(initials.length() - 1);
return initials.toString().toUpperCase();
}
public static void main(String[] args) {
String name = "Mary Jane Smith";
System.out.println("Initials: " + getInitials(name));
}
}
Explanation of the Code
-
Null and Empty Check: We first check if the input string is null or empty. If it is, we return an empty string. This helps avoid
NullPointerExceptions
or unwanted outputs. -
Splitting the Name: We use
String.split(" ")
to divide the name into components. This method returns an array of substrings. By iterating over this array, we can access each name part. -
Building the Initials: For each part, we append the first character to the
StringBuilder
object followed by a period. -
Final Touch: Finally, we remove the last period that has been appended after the last initial.
-
Uppercase Transformation: To maintain consistency, we convert the initials to uppercase.
Sample Output
Running the above code with Mary Jane Smith
would output:
Initials: M.J.S
Handling More Complex Names
In reality, names may have additional complexity. Let’s consider cases like middle names or the use of prefixes and suffixes.
Step 2: Enhanced Functionality
Here’s how we can enhance our method to accommodate some additional scenarios.
public static String getEnhancedInitials(String fullName) {
if (fullName == null || fullName.trim().isEmpty()) {
return "";
}
StringBuilder initials = new StringBuilder();
String[] nameParts = fullName.trim().split("\\s+"); // Handle multiple spaces
for (String namePart : nameParts) {
if (!namePart.isEmpty() && Character.isLetter(namePart.charAt(0))) {
initials.append(namePart.charAt(0)).append(".");
}
}
initials.setLength(Math.max(initials.length() - 1, 0)); // Handle case if initials were added.
return initials.toString().toUpperCase();
}
What's New?
-
Trimming and Splitting: We use
fullName.trim().split("\\s+")
to handle strings with multiple spaces between names. -
Character Check: Checking if the character is a letter helps filter out any erroneous inputs.
Usage Scenario
You can use this method anywhere you need initials, from creating user profiles to displaying names in UI.
public static void main(String[] args) {
String[] names = {
"John Doe",
" Mary Jane Smith ",
" Sir Ian McKellen ",
"",
null,
"Dr. John H. Watson"
};
for (String name : names) {
System.out.println("Initials: " + getEnhancedInitials(name));
}
}
Sample Output
Initials: J.D
Initials: M.J.S
Initials: I.M
Initials:
Initials:
Initials: J.W
The Closing Argument
Extracting initials in Java is a common task that can be handled with basic string manipulation techniques. By considering various edge cases and enhancing our initial implementation, we've developed a robust solution for a seemingly simple challenge.
For further reading on best practices in Java string manipulation, consider visiting Oracle's Java Documentation or Java String Manipulation Guide.
Mastering such fundamental tasks can greatly improve code readability and reduce complexity, making it easier to maintain and develop further functionalities in your Java applications.
If you have further questions about Java programming or extracting initials, feel free to comment below! Happy coding!
Checkout our other articles