Struggling with Guice? Simplify Your Rich Domain Model!
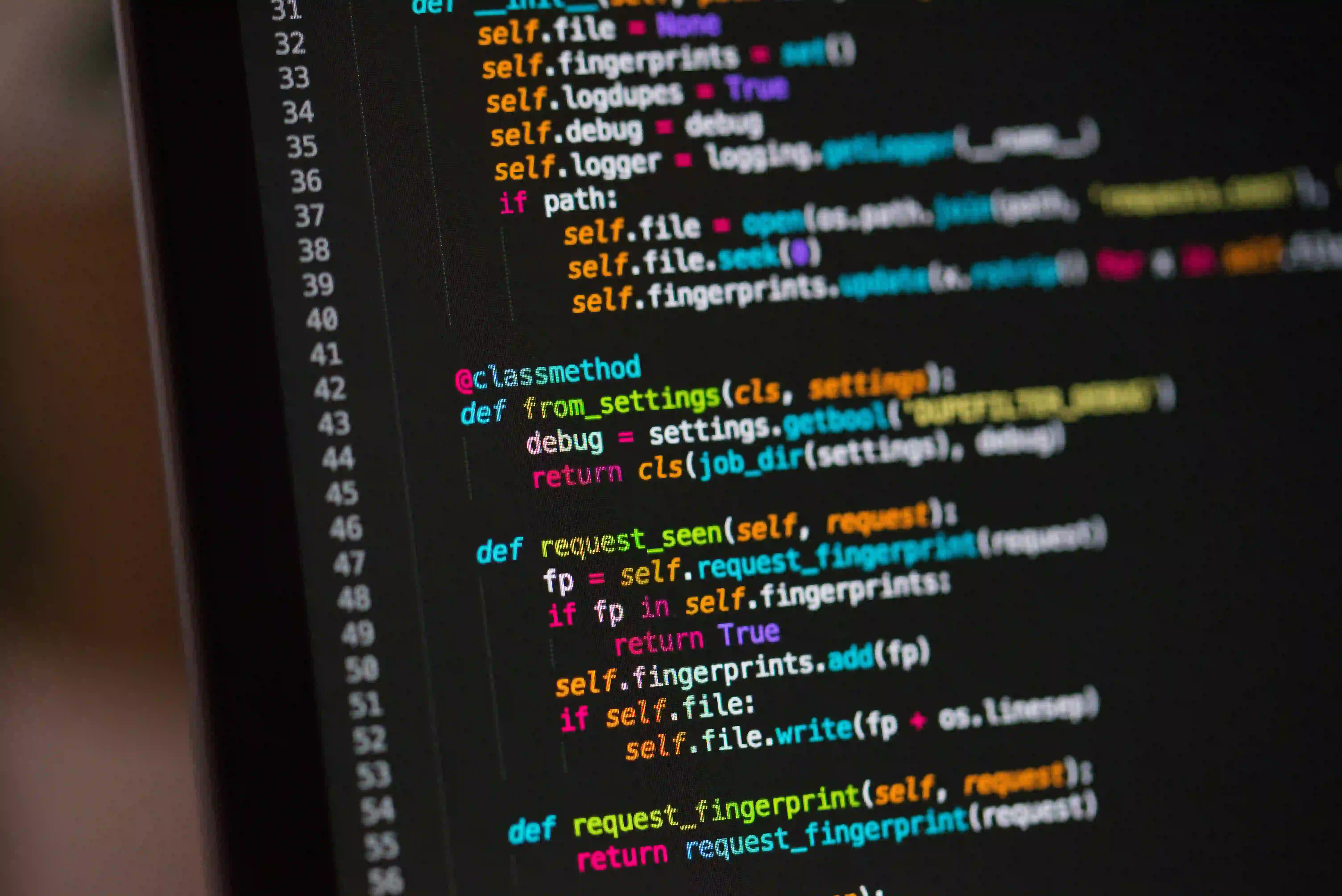
Struggling with Guice? Simplify Your Rich Domain Model!
In the vast world of Java development, many programmers encounter the challenge of managing dependencies in a way that remains clean and scalable. Google Guice, a lightweight dependency injection framework, simplifies this task. However, it’s not uncommon to feel overwhelmed by the intricacies that accompany building a rich domain model while leveraging Guice. In this blog post, we will break down some of these concepts, providing you with practical examples and clarifying the rationale behind them.
What is Dependency Injection?
Dependency Injection (DI) is a design pattern that enables a class to receive its dependencies from external sources rather than creating them internally. DI promotes loose coupling, making code easier to test, maintain, and scale.
Key Benefits of Dependency Injection:
- Decoupled Code: Classes are less dependent on each other.
- Easier Testing: Mock dependencies can be easily injected.
- Greater Maintainability: Changes in dependencies require less code change.
Introducing Guice
Google Guice helps implement DI in a straightforward manner. Using Guice, you define your dependencies in a configuration module, then let the framework handle the instantiation and injection. Let’s explore how to set up a simple project with Guice.
Setting Up Guice
To get started, ensure that you have Guice included in your Maven or Gradle project.
Maven Dependency:
<dependency>
<groupId>com.google.inject</groupId>
<artifactId>guice</artifactId>
<version>5.0.1</version>
</dependency>
Gradle Dependency:
implementation 'com.google.inject:guice:5.0.1'
Building Your Domain Model
Let’s create a simple domain model where we have a User
class and a service that handles user operations, UserService
.
Step 1: Create the User Class
public class User {
private String id;
private String name;
public User(String id, String name) {
this.id = id;
this.name = name;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
}
Step 2: Create the UserService Class
Now, let’s define the UserService
class, which will depend on a repository to fetch user data.
public interface UserRepository {
User findUserById(String id);
}
public class UserService {
private final UserRepository userRepository;
@Inject
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUser(String userId) {
return userRepository.findUserById(userId);
}
}
Why Use Interfaces? Using an interface for the repository ensures you can easily swap out implementations, which simplifies testing and maintenance.
Step 3: Create a Repository Implementation
Let’s create an implementation for our repository.
public class InMemoryUserRepository implements UserRepository {
private final Map<String, User> users = new HashMap<>();
public InMemoryUserRepository() {
users.put("1", new User("1", "John Doe"));
users.put("2", new User("2", "Jane Smith"));
}
@Override
public User findUserById(String id) {
return users.get(id);
}
}
Step 4: Setting Up the Guice Module
To bind our classes with their dependencies, we define a module:
import com.google.inject.AbstractModule;
public class UserModule extends AbstractModule {
@Override
protected void configure() {
bind(UserRepository.class).to(InMemoryUserRepository.class);
bind(UserService.class);
}
}
Step 5: Using Guice for Dependency Injection
Now that we have set up our classes and the module, we can use Guice’s injector to create instances.
import com.google.inject.Guice;
import com.google.inject.Injector;
public class Main {
public static void main(String[] args) {
Injector injector = Guice.createInjector(new UserModule());
UserService userService = injector.getInstance(UserService.class);
User user = userService.getUser("1");
System.out.println("Fetched User: " + user.getName());
}
}
Understanding the Flow
Here's how our code flows:
- The
Main
class creates an injector usingGuice.createInjector()
, passing an instance of ourUserModule
. - It retrieves the instance of
UserService
from the injector. - The
UserService
is constructed with an instance ofUserRepository
, which is actually an instance ofInMemoryUserRepository
.
Testing Your Code with Guice
When it comes to testing, you can mock dependencies seamlessly since Guice allows you to bind different classes easily.
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testGetUser() {
UserRepository mockRepository = mock(UserRepository.class);
when(mockRepository.findUserById("1")).thenReturn(new User("1", "Mock User"));
UserService userService = new UserService(mockRepository);
User user = userService.getUser("1");
assertEquals("Mock User", user.getName());
}
}
Why Guice?
Using Guice results in cleaner, more manageable code. It abstracts away much of the boilerplate code associated with manual dependency management. Additionally, Guice integrates well with other Java frameworks, making it a solid choice for modern applications.
Additional Resources
If you would like to dive deeper into dependency injection with Guice or explore its more advanced features, consider checking out these resources:
Final Thoughts
Google Guice can significantly simplify managing dependencies in a rich domain model, enabling cleaner, more maintainable, and testable code. By understanding its core components and leveraging them effectively, you can overcome initial struggles and harness the full potential of this powerful framework.
Start integrating Guice into your projects today, and simplify your development process!
Comments and Feedback
If you have any questions, tips, or experiences with Google Guice, feel free to share in the comments below!