Overcoming the Fear of Continuous Deployment Success
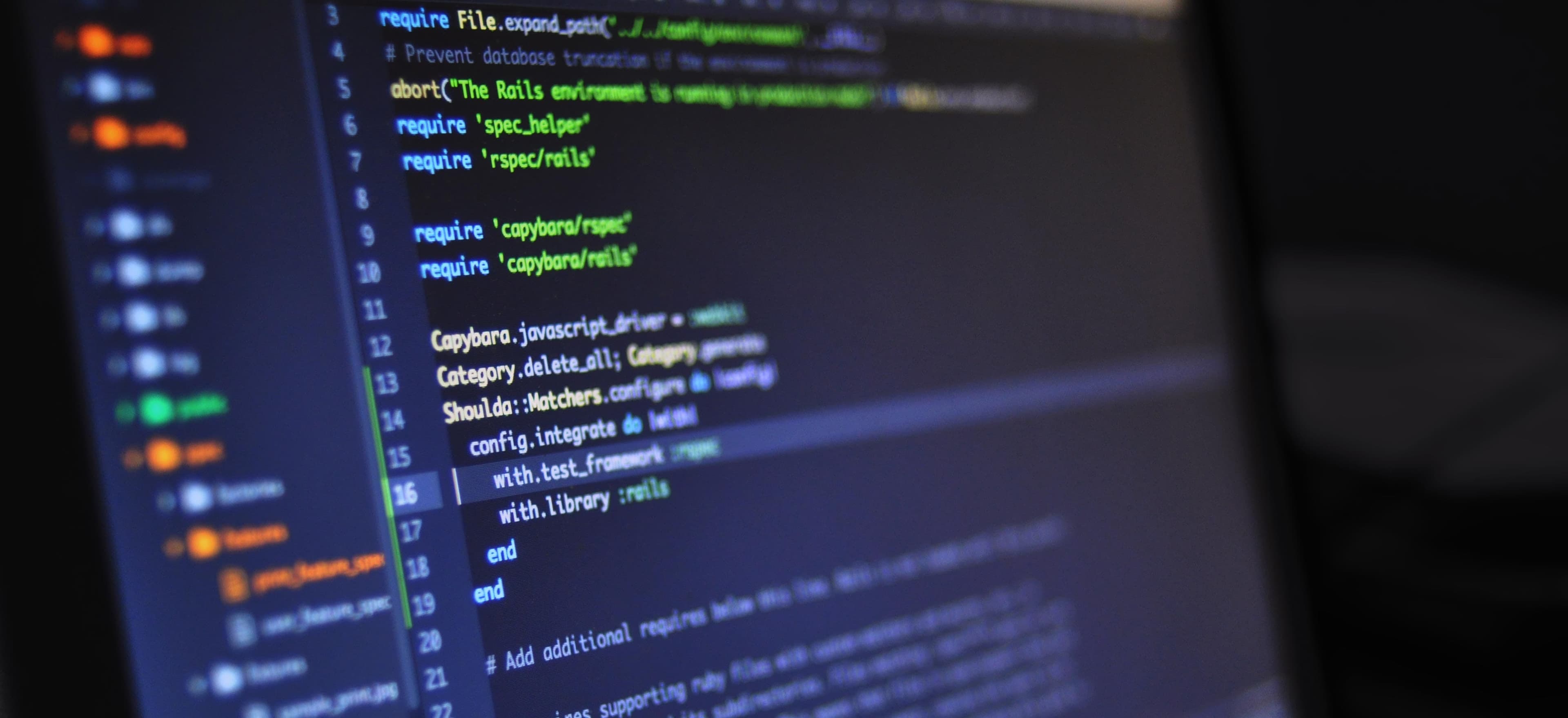
- Published on
Overcoming the Fear of Continuous Deployment Success
In today’s fast-paced software development environment, Continuous Deployment (CD) is a topic that garners both hope and fear. The idea of deploying code changes seamlessly to production may sound revolutionary, but it often invokes anxiety among developers and stakeholders alike. This post aims to demystify Continuous Deployment, tackle the associated fears, and illustrate how its successful implementation can lead to a more agile, responsive development process.
Understanding Continuous Deployment
Continuous Deployment is a software development practice where every code change that passes automated tests is automatically deployed to production. Unlike Continuous Integration (CI), where code changes are merged into a central repository and built upon, CD takes it a step further by releasing every change as soon as it is ready.
Why Continuous Deployment?
- Faster Time to Market: Teams can respond to user needs more rapidly.
- Immediate Feedback: Direct user feedback allows for quicker adjustments.
- Higher Quality: Since code is tested at every stage, quality improves continuously.
However, many organizations still hesitate to adopt CD due to fears and concerns that stem from uncertainty.
Common Fears Ahead of Continuous Deployment
-
Fear of Broken Deployments
Many developers worry about deploying unfinished or flawed features. The thought of breaking the live application can be daunting. Even companies heavily invested in CD have faced deployment failures, leading to lost revenue and customer trust.
-
Fear of Increased Complexity
Implementing Continuous Deployment can introduce complexity to your workflow. From orchestrating deployments to maintaining staging environments, the overhead can seem overwhelming.
-
Fear of Resource Allocation
CD requires significant investment in automated testing and deployment pipelines. Organizations may fear the high upfront costs without a guaranteed return on investment.
-
Cultural Resistance
Your team may be accustomed to traditional deployment practices. Changing the mindset of a team can be one of the hardest hurdles to overcome.
Strategies to Overcome These Fears
Here we outline effective strategies and best practices to overcome the fears surrounding Continuous Deployment.
1. Implement a Comprehensive Testing Suite
A solid testing regimen is the backbone of Continuous Deployment. It instills confidence that code changes are reliable and minimizes the chances of failure.
Example of a Simple Test Case in Java using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
In this example, we are asserting that adding 2 and 3 returns 5. Automated tests like this should be a regular part of your CI/CD pipeline. Why? It ensures that as you deploy changes, you have a safety net that catches issues before they reach production.
2. Use Feature Flags
Feature flags allow you to deploy code that can be toggled on or off without affecting end users immediately. This mechanism can help you test features in production without creating a mess.
Example of Implementing a Feature Flag:
public class FeatureToggle {
private boolean isNewFeatureEnabled;
public FeatureToggle(boolean isEnabled) {
this.isNewFeatureEnabled = isEnabled;
}
public void executeFeature() {
if (isNewFeatureEnabled) {
// Execute new feature code
} else {
// Execute old feature code
}
}
}
With this approach, you can safely deploy the new feature. If anything goes wrong, you can switch it off without rolling back the entire deployment. Why? It allows for experimentation and gradual rollout without endangering your production environment.
3. Continuous Monitoring and Rollbacks
Monitoring tools collect real-time data on your application, catching any discrepancies early. By employing these, you make informed decisions and can quickly revert changes if something goes wrong.
Example of a Safe Rollback Using Git:
# Check the last few commits
git log --oneline
# Reset to a previous version
git reset --hard <commit-hash>
This process allows you to revert to a known stable state if a deployment causes issues. Why? This safety net can alleviate fears surrounding deployment, as you know you can quickly recover from errors.
4. Foster a Culture of Continuous Improvement
Building a DevOps culture takes time but is essential for successful Continuous Deployment. Encourage team members to embrace failure as a learning opportunity. Conduct post-mortems on failed deployments to improve for the future.
How To Implement This Culture:
- Regularly hold retrospectives.
- Promote open discussions about deployment fears.
- Reward experimentation and innovation.
5. Get Stakeholder Buy-In
It is crucial to ensure that business stakeholders understand the value of Continuous Deployment. Educate them on how rapid deployment can benefit the business. Use case studies from successful companies leveraging CD.
For more insight on how organizations like Netflix successfully implemented Continuous Deployment, you can visit Netflix’s Technology Blog.
My Closing Thoughts on the Matter
Overcoming the fear of Continuous Deployment is not a simple task, but it is entirely doable. By relying on a strong testing framework, feature flags, continuous monitoring, and fostering a solid culture, organizations can deploy with confidence. Embracing this mindset can help unlock the full potential of your development process.
By breaking down barriers and building a cooperative environment between teams, you can transform Continuous Deployment from a source of anxiety to a powerful tool for rapid innovation.
References
- Continuous Delivery: Reliable Software Releases through Build, Test, and Deployment Automation
- Implementing DevOps: Building a Continuous Delivery Pipeline
Are you ready to embrace Continuous Deployment? Remember, the key is not the number of deployments but the robustness of your process. Happy coding!
Checkout our other articles