Overcoming Challenges in Effective Performance Monitoring
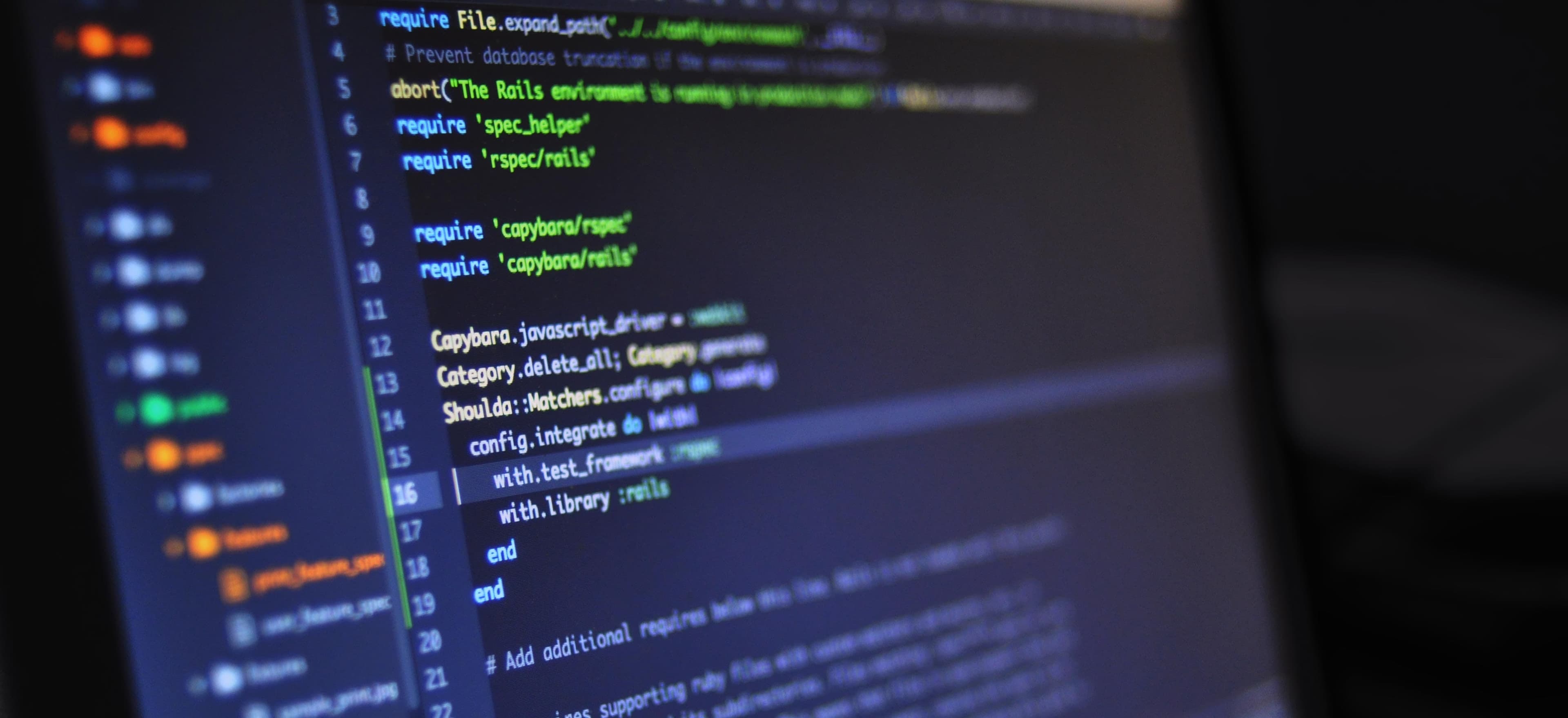
- Published on
Overcoming Challenges in Effective Performance Monitoring
Performance monitoring is a critical aspect of software development and IT operations. The ability to consistently gather and analyze performance data can significantly enhance the efficiency, reliability, and user satisfaction of applications. Many organizations, however, face various challenges in implementing effective performance monitoring strategies. In this blog post, we will explore these challenges and provide solutions to overcome them.
The Importance of Performance Monitoring
Before diving into the challenges, let's understand why performance monitoring is vital.
- Identifying Bottlenecks: Performance monitoring helps in identifying bottlenecks in an application, enabling teams to refine and optimize their code.
- Enhancing User Experience: By keeping performance in check, organizations can deliver a smoother, more responsive user experience, leading to customer satisfaction and retention.
- Resource Management: Effective monitoring aids in understanding resource utilization, ensuring that hardware and software resources are used optimally.
Common Challenges in Performance Monitoring
1. Data Overload
With numerous metrics to track, organizations often grapple with data overload. This can lead to confusion, making it difficult to identify critical issues.
Solution: Implement a focused approach by determining key performance indicators (KPIs) relevant to your application.
// Example of defining KPIs in Java
public class KPIMetrics {
private int responseTime; // in milliseconds
private int errorRate; // percentage of errors
private int throughput; // requests per second
// Constructor to initialize KPI metrics
public KPIMetrics(int responseTime, int errorRate, int throughput) {
this.responseTime = responseTime;
this.errorRate = errorRate;
this.throughput = throughput;
}
public void displayMetrics() {
System.out.println("Response Time: " + responseTime + "ms");
System.out.println("Error Rate: " + errorRate + "%");
System.out.println("Throughput: " + throughput + " requests/sec");
}
}
// Usage
KPIMetrics metrics = new KPIMetrics(200, 1, 100);
metrics.displayMetrics();
Why this works: By creating a class that encapsulates all essential KPIs, it allows teams to focus on the most important performance metrics without getting lost in the noise.
2. Real-time Monitoring Challenges
Real-time performance monitoring is essential for responding to issues as they arise. However, achieving this can be technically and logistically complex.
Solution: Utilize automated monitoring tools that support real-time data collection and analysis.
Tools such as Prometheus and Grafana can be integrated into your application to provide real-time insights.
- Prometheus: It scrapes metrics from applications at specified intervals.
- Grafana: It visualizes the data collected to help teams quickly understand application performance.
3. Lack of Integration with Existing Tools
Another major hurdle is the lack of integration of monitoring tools with existing systems. Many organizations rely on a variety of tools for development, testing, and production, and a disconnect between them can lead to inconsistencies.
Solution: Choose performance monitoring solutions that are designed to integrate seamlessly with existing DevOps tools. For instance, New Relic provides extensive API support for integrating performance data into development workflows and CI/CD pipelines.
4. Understanding and Interpreting Data
Having access to data is one thing, but understanding and interpreting the data is another challenge entirely. Teams often lack the analytical skills to draw actionable insights from performance data.
Solution: Provide training for team members in data interpretation and use analytics tools effectively.
Here’s how you might implement a simple logging mechanism in Java that can help capture performance data, which can later be analyzed:
import java.util.logging.*;
// Logger setup
public class PerformanceLogger {
private static final Logger logger = Logger.getLogger(PerformanceLogger.class.getName());
public static void logPerformanceData(String methodName, long executionTime) {
logger.log(Level.INFO, "Method: {0} took {1}ms to execute.", new Object[]{methodName, executionTime});
}
}
// Usage example
public class SampleService {
public void sampleMethod() {
long startTime = System.currentTimeMillis();
// Method logic here
long endTime = System.currentTimeMillis();
PerformanceLogger.logPerformanceData("sampleMethod", endTime - startTime);
}
}
Why this works: Logging execution time allows teams to track method performance over time and identify slow-performing methods for optimization.
5. Scalability of Monitoring Solutions
As the application grows, the performance monitoring solutions need to scale accordingly. Performance issues that may not be evident in a smaller scale can become major concerns as user load and data throughput increase.
Solution: Implement microservices architecture where necessary and leverage distributed monitoring solutions.
For instance, tools like Elastic Stack can be employed to monitor applications across multiple services without losing performance insights.
6. Handling Non-Functional Requirements
Performance monitoring must encompass not only functional but also non-functional requirements, such as load time and availability.
Solution: Use synthetic monitoring to simulate user interactions and assess application performance from an end-user perspective.
With synthetic monitoring tools, you can script user journeys and monitor performance at various stages, from making an API call to rendering a webpage.
Wrapping Up
Effective performance monitoring is an essential practice for any organization developing and maintaining applications. By overcoming the challenges outlined in this blog post -- data overload, real-time monitoring complexity, integration issues, data interpretation difficulties, scalability needs, and ensuring non-functional requirements -- organizations can optimize their applications for the best user experience.
Remember, these practices are not just about maintaining performance but continuously improving it. Always adapt and refine your monitoring strategies based on real-time insights obtained.
If you're looking for a deeper dive into specific performance monitoring tools, check out this comprehensive guide on monitoring tools.
Feel free to share your thoughts and experiences related to performance monitoring challenges and successes in the comments below. Happy coding!
Checkout our other articles