Common Pitfalls When Developing RESTful Services with Apache CXF
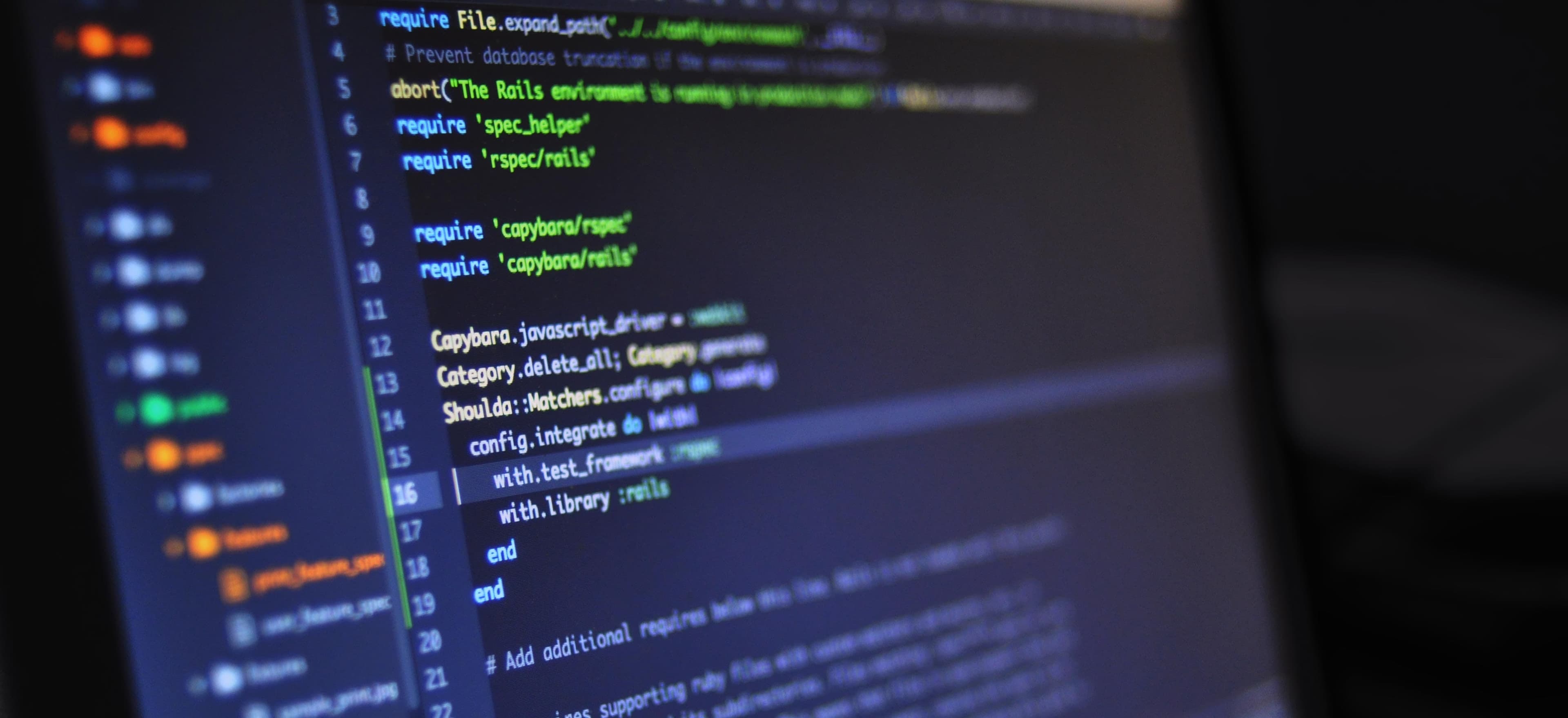
- Published on
Common Pitfalls When Developing RESTful Services with Apache CXF
Creating robust RESTful services is an essential component of modern software development. Apache CXF is a widely adopted framework that helps streamline the process of building these services. However, as with any technology, there are common pitfalls that developers may encounter. In this blog post, we will explore some of these pitfalls and how to effectively navigate them, ensuring that your RESTful services are efficient, maintainable, and scalable.
Understanding REST and Apache CXF
Before we dive into the pitfalls, let’s briefly understand what REST and Apache CXF are.
REST (Representational State Transfer) is an architectural style for designing networked applications. It relies on a stateless communication protocol, usually HTTP, and emphasizes the use of standard HTTP methods like GET, POST, PUT, and DELETE.
Apache CXF is an open-source framework that helps you build services using frontend programming APIs, such as JAX-WS and JAX-RS. It supports both SOAP and RESTful service development and provides a wealth of features to facilitate service creation, including data bindings, security, and configuration tools.
Common Pitfalls
1. Overlooking the Importance of HATEOAS
One key principle of REST architectural style is HATEOAS (Hypermedia as the Engine of Application State). This principle states that clients should be able to navigate the API dynamically using hyperlinks.
Pitfall: Many developers neglect to implement HATEOAS, causing their APIs to be less discoverable and more challenging to use.
How to Avoid: Make sure to include hypermedia links in your responses. For instance, if you have a resource representing a book, you might include links to its author or related reviews.
@Path("/books")
public class BookResource {
@GET
@Path("/{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response getBook(@PathParam("id") String id) {
Book book = bookService.findBook(id);
// Adding HATEOAS links
book.addLink(new Link("self", "/books/" + id));
book.addLink(new Link("author", "/authors/" + book.getAuthorId()));
return Response.ok(book).build();
}
}
The above code illustrates how to include links within the book resource, making the API more navigable. This enhances client usability and adheres to REST principles.
2. Ignoring Error Handling
Proper error handling and messaging in REST services are critical for client interaction. Not providing precise error messages can lead to confusion and poor user experiences.
Pitfall: Developers may overlook the need for comprehensive error handling or fail to standardize error responses.
How to Avoid: Use custom exceptions and response entities to handle errors gracefully. Here's an example of how to create a structured error response.
@Provider
public class CustomExceptionHandler implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable exception) {
ErrorResponse errorResponse = new ErrorResponse("server_error", exception.getMessage());
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity(errorResponse)
.build();
}
}
// Custom error response model
public class ErrorResponse {
private String errorType;
private String message;
public ErrorResponse(String errorType, String message) {
this.errorType = errorType;
this.message = message;
}
// Getters and setters...
}
By implementing a custom exception handler, you ensure consistent error reporting across your API. This not only aids developers but also enhances client applications consuming your service.
3. Underestimating Request Validations
Not validating incoming requests could lead to many issues, including unexpected server behavior, data integrity problems, or vulnerabilities.
Pitfall: Failing to validate request parameters or payloads can result in corrupted data being processed and stored.
How to Avoid: It is critical to validate request parameters and body content through annotations or manual checks.
@POST
@Path("/books")
@Consumes(MediaType.APPLICATION_JSON)
public Response addBook(@Valid Book book) {
if (book.getTitle() == null) {
return Response.status(Response.Status.BAD_REQUEST)
.entity("Book title cannot be null").build();
}
bookService.save(book);
return Response.status(Response.Status.CREATED).build();
}
In the above example, we are using the @Valid
annotation to assure the incoming data is correct. This keeps your service stable and reliable while promoting good data quality practices.
4. Mismanagement of Content Negotiation
Content negotiation is a core principle that allows clients to specify the format of the response. Ignoring this can lead to misunderstandings and decrease usability.
Pitfall: Developers often hard-code response formats instead of supporting multiple formats based on HTTP headers.
How to Avoid: Enable content negotiation in your services.
@GET
@Path("/books/{id}")
@Produces({MediaType.APPLICATION_JSON, MediaType.APPLICATION_XML})
public Response getBookById(@PathParam("id") String id, @Context HttpHeaders headers) {
Book book = bookService.findBook(id);
if (book == null) {
return Response.status(Response.Status.NOT_FOUND).build();
}
return Response.ok(book).build();
}
This snippet ensures that the response can be served in either JSON or XML format, based on what the client prefers. By doing so, you cater to a wider range of client needs.
5. Forgetting About Security Considerations
Security in RESTful services is often underestimated. With sensitive data being transmitted, failure to implement security measures can have severe consequences.
Pitfall: Many services lack authentication and authorization mechanisms, making them vulnerable to attacks.
How to Avoid: Integrate security solutions, such as OAuth2 or JWT tokens, to manage access to resources.
@GET
@Path("/secure/book")
@RolesAllowed("ADMIN")
public Response getSecureBook() {
// Only accessible to users with ADMIN role
return Response.ok().entity("This is a secure book resource").build();
}
Implementing security at the endpoint level ensures that only authorized users can access sensitive data or actions.
To Wrap Things Up
Navigating the common pitfalls when developing RESTful services with Apache CXF can significantly enhance your service's reliability, usability, and security. Remember, careful consideration of principles like HATEOAS, error handling, request validations, content negotiation, and security measures will help you create a more robust and maintainable API.
As you continue to develop RESTful services, keeping these best practices in mind will not only improve your current projects but will also pave the way for better software design principles in future endeavors.
For further reading on building RESTful services, consider checking the official Apache CXF documentation and the book RESTful Java with JAX-RS 2.0 by Leonard Richardson.
Happy coding!