Eliminating Null Parameters: A Spring Aspect Guide
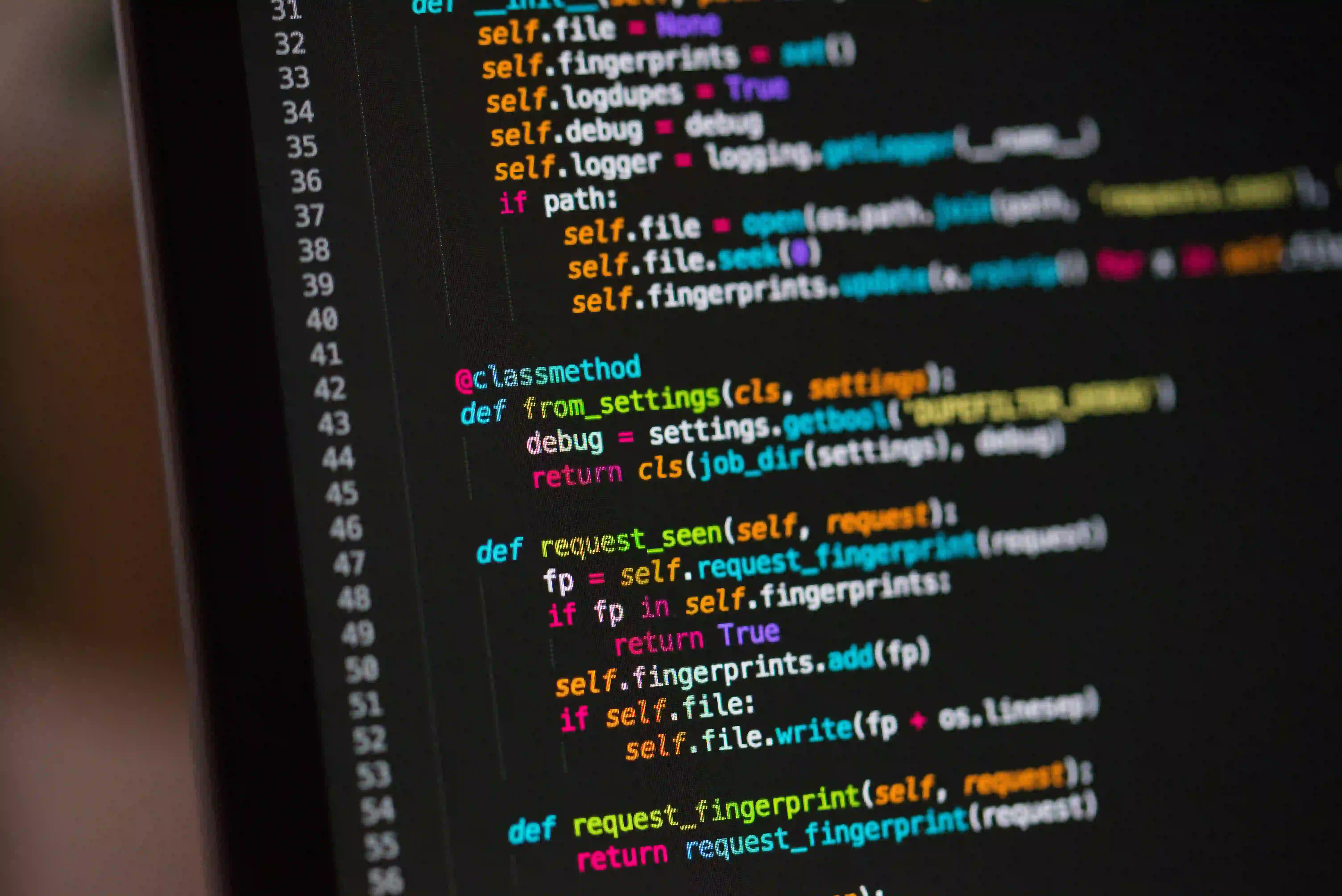
Eliminating Null Parameters: A Spring Aspect Guide
As modern application development continues to evolve, maintaining clean and efficient code is more crucial than ever. One common pitfall in programming, especially in Java-based applications, is the presence of null parameters. A null value can lead to a multitude of errors, ranging from simple exceptions to significant application failures. In this blog post, we will explore how to eliminate null parameters using Spring AOP (Aspect-Oriented Programming).
What is AOP?
Aspect-Oriented Programming (AOP) is a programming paradigm that allows you to separate cross-cutting concerns from the main business logic. This enhances modularity and reusability of code. AOP is particularly useful for logging, transaction management, and, as we will see, parameter validation.
Why Eliminate Null Parameters?
Null parameters can lead to NullPointerExceptions
(NPE) at runtime, which can be difficult to trace and debug. This might cause applications to crash, affecting user experience and reliability. By implementing a strategy to check for null parameters, we can:
- Improve code robustness.
- Enhance maintainability.
- Prevent runtime exceptions.
- Make our APIs more user-friendly.
Setting Up a Spring Project
Before we dive into eliminating null parameters using AOP, we need to ensure we have a Spring project set up. You can create a Spring Boot application following these steps:
- Go to Spring Initializr.
- Select your preferred project metadata (group, artifact, name).
- Choose dependencies such as Spring Web and Spring AOP.
- Click on "Generate," and then download the zip file.
- Extract the files and import the project into your favorite IDE.
Implementing AOP for Null Parameter Checks
Step 1: Create an Aspect Class
We will create an aspect class which contains the logic to check for null parameters. Here’s how you can implement it.
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class ParameterValidationAspect {
@Around("execution(* com.example.service.*.*(..))")
public Object validateParameters(ProceedingJoinPoint joinPoint) throws Throwable {
Object[] args = joinPoint.getArgs();
for (Object arg : args) {
if (arg == null) {
throw new IllegalArgumentException("Null parameter not allowed: " + arg);
}
}
return joinPoint.proceed();
}
}
Explanation of the Code
- @Aspect: This annotation indicates that the class is an aspect in Spring AOP.
- @Around: This pointcut expression targets all methods within the package
com.example.service
. You can adjust the package according to your application's structure. - ProceedingJoinPoint: This allows us to join the method execution and proceed with it after validation.
- validateParameters method: This method checks each parameter for null values, and if any are found, it throws an
IllegalArgumentException
.
Step 2: Create a Sample Service
Next, we will create a sample service to demonstrate how our aspect works.
import org.springframework.stereotype.Service;
@Service
public class SampleService {
public void processData(String data) {
System.out.println("Processing: " + data);
}
}
Step 3: Testing the Aspect
It’s crucial to test whether our aspect effectively catches null parameters. We will create a simple controller.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class SampleController {
@Autowired
private SampleService sampleService;
@PostMapping("/process")
public String process(@RequestParam(required = false) String data) {
sampleService.processData(data);
return "Data processed successfully!";
}
}
What Happens Here?
When you invoke the endpoint /api/process
with a null parameter:
- The
processData
method inSampleService
is intercepted byParameterValidationAspect
. - The aspect checks every parameter.
- On finding
data
to be null, it throws anIllegalArgumentException
.
Testing with Postman
To test this implementation, you can use Postman or any API testing tool. Send a POST request to http://localhost:8080/api/process
without a parameter or with data=null
. You should receive an error response stating that null parameters are not allowed.
Advanced Null Parameter Validation
The basic aspect shown above checks for null parameters. However, for enhanced flexibility, we can design it to ignore certain parameters or provide more granularity in error handling. Consider the following modifications:
- Custom Annotations: Create a custom annotation that can specify which parameters can be null.
- Logging: Add logging for better traceability of where the null value originated.
Example of a custom annotation:
import java.lang.annotation.*;
@Target(ElementType.PARAMETER)
@Retention(RetentionPolicy.RUNTIME)
public @interface NullableParam {
}
Example on Applying the Annotation
Modify the SampleService
method to use the custom annotation:
public void processData(@NullableParam String data) {
System.out.println("Processing: " + data);
}
Update the aspect to respect this annotation:
import java.lang.reflect.Parameter;
public Object validateParameters(ProceedingJoinPoint joinPoint) throws Throwable {
Parameter[] parameters = joinPoint.getSignature().getDeclaringType().getMethod(joinPoint.getSignature().getName()).getParameters();
Object[] args = joinPoint.getArgs();
for (int i = 0; i < args.length; i++) {
if (args[i] == null && !parameters[i].isAnnotationPresent(NullableParam.class)) {
throw new IllegalArgumentException("Null parameter not allowed: " + parameters[i].getName());
}
}
return joinPoint.proceed();
}
Final Thoughts
Using Spring AOP to eliminate null parameters is a robust approach to improving application reliability and maintainability. The aspect we developed provides a flexible and reusable way of validating parameters, reducing the risk of NullPointerExceptions
in your Spring applications.
For further reading, consider checking out the following resources:
- Spring AOP Documentation
- Effective Java, 3rd Edition by Joshua Bloch, which covers best practices in Java.
By implementing these techniques, you can keep your applications clean, efficient, and user-friendly. Happy coding!