Common Pitfalls When Using JpaRepository in Spring Boot
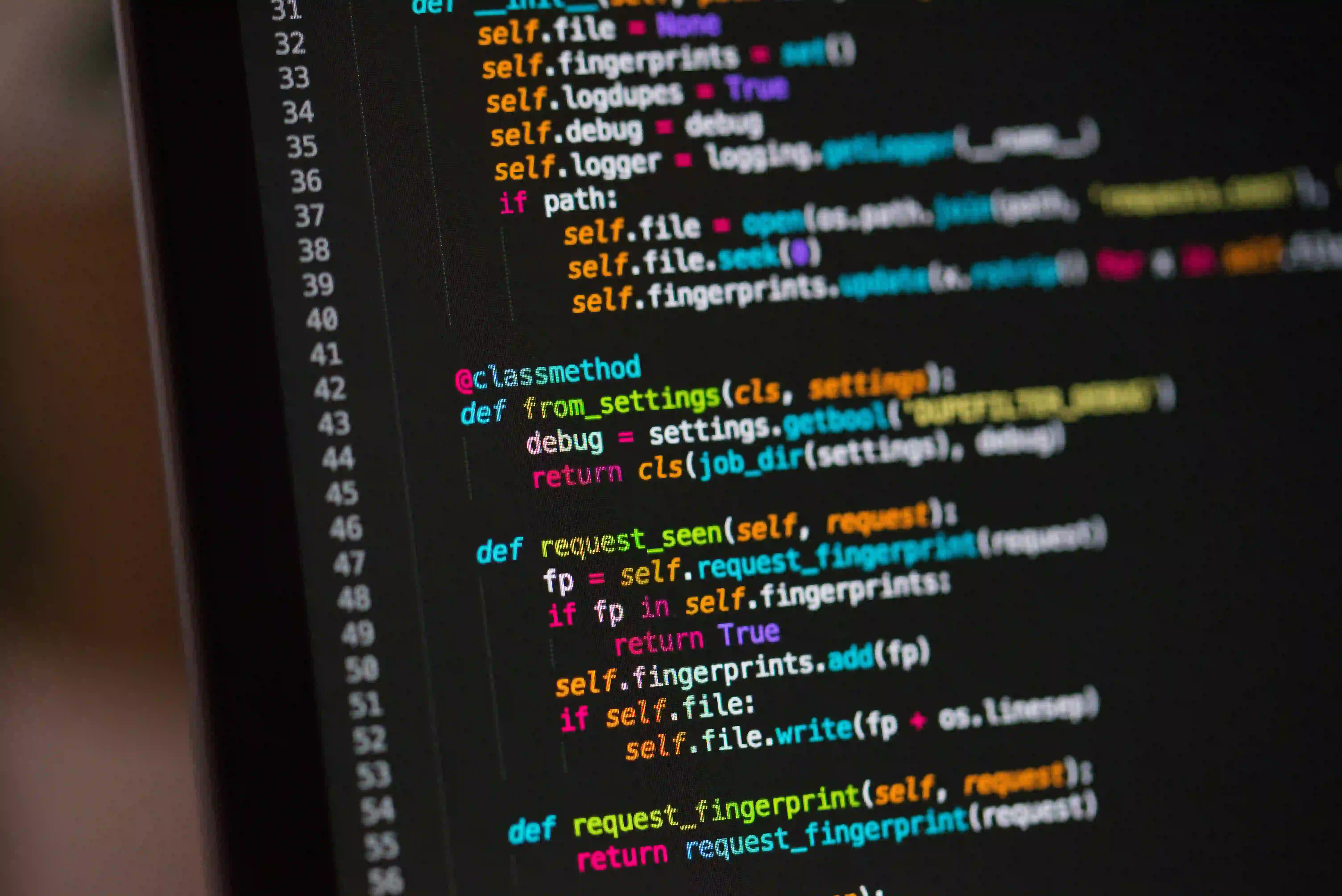
Common Pitfalls When Using JpaRepository in Spring Boot
Spring Data JPA is a powerful framework that makes it easy to work with databases in Java applications. One of its most significant features is the JpaRepository
interface, which provides a simple way to interact with your database. However, while it simplifies development, there are common pitfalls that can hinder performance or lead to unexpected behavior. In this blog post, we will explore these pitfalls and offer solutions to enhance your development process.
Understanding JpaRepository
Before diving into potential pitfalls, let’s clarify what JpaRepository
is. It is an interface that simplifies data access and cache management for Java Persistence API (JPA) entities. The interface provides methods for CRUD operations and allows for more complex queries through method naming conventions, derived queries, or the use of SQL statements.
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByLastName(String lastName);
}
In this code snippet, UserRepository
will provide basic CRUD operations and a custom query to find users by their last names.
Pitfall 1: Over-reliance on Derived Query Methods
While derived query methods are convenient, they can sometimes lead to performance issues, especially as your application scales. When using derived methods, JPA must create queries in real-time based on method names, which can lead to inefficient SQL.
Solution
Always consider the underlying SQL generated by your query. You can do this by enabling SQL logging in your application.properties
:
spring.jpa.show-sql=true
If performance is critical, prefer using the @Query
annotation for complex queries. This way, you can write optimized JPQL or native SQL.
@Query("SELECT u FROM User u WHERE u.lastName = ?1")
List<User> customFindByLastName(String lastName);
By explicitly defining your query, you gain more control over its performance.
Pitfall 2: Not Using Optional
for Fetching Single Entities
When retrieving a single entity, many developers perform their fetch operations without considering the possibility that the entity may not exist. This can lead to a NoSuchElementException
.
Solution
Use Optional<T>
to wrap your return values. This allows you to handle absent values gracefully.
Optional<User> findById(Long id);
When you call this method, you can check if the user exists before proceeding:
Optional<User> userOpt = userRepository.findById(1L);
if (userOpt.isPresent()) {
User user = userOpt.get();
// proceed with user
} else {
// handle user not found
}
Pitfall 3: Inefficient Use of Fetching Strategies
In JPA, you can define how to fetch related entities: either eagerly or lazily. Overusing eager fetching can lead to performance issues and increased memory consumption.
Solution
Default to lazy loading unless you are certain that the relationships will always be accessed. This prevents unnecessary joins at query time.
@Entity
public class User {
@ManyToOne(fetch = FetchType.LAZY)
private Role role;
}
If you must use eager fetching, consider fetching only the relationships you need, using the @EntityGraph
annotation.
@EntityGraph(attributePaths = {"role"})
Optional<User> findByIdWithRole(Long id);
Pitfall 4: Ignoring Transactions
Another common oversight is neglecting transaction management. By default, Spring's repositories operate within a transactional context. However, certain operations, such as batch inserts or updates, might need explicit transaction management.
Solution
Use the @Transactional
annotation correctly to manage transactions.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void saveAllUsers(List<User> users) {
userRepository.saveAll(users);
}
}
Pitfall 5: Not Handling N+1 Query Issues
The N+1 problem arises when a query retrieves a list of entities, followed by one query for each entity to load related entities. This can lead to performance degradation.
Solution
To avoid N+1 issues, ensure that you fetch related entities in a single query whenever possible. This can be achieved through joins in JPQL or using the JOIN FETCH
syntax.
@Query("SELECT u FROM User u JOIN FETCH u.role")
List<User> findAllWithRoles();
By using this approach, you will reduce the number of database calls, improving your application's efficiency.
Pitfall 6: Incorrectly Configuring Entity Relationships
Misconfiguring relationships can lead to data inconsistency or even runtime exceptions. For example, forgetting to annotate bidirectional relationships can create issues when trying to persist or retrieve entities.
Solution
Always clearly define your relationships. Use the mappedBy
attribute correctly in bidirectional relationships:
@Entity
public class User {
@OneToMany(mappedBy = "user")
private List<Order> orders;
}
@Entity
public class Order {
@ManyToOne
private User user;
}
Final Thoughts
While JpaRepository
provides a straightforward way to handle data access in Spring Boot applications, it is essential to understand its underlying mechanics to avoid common pitfalls. By implementing solutions such as utilizing Optional
correctly, optimizing queries, managing transactional boundaries, and properly configuring relationships, you can improve performance and maintainability.
For further reading on Spring Data JPA, check out the official documentation and explore additional best practices in handling entities.
By keeping these pitfalls in mind and applying best practices during development, you’ll ensure that your Spring Boot applications are efficient, maintainable, and scalable for future growth. Happy coding!